licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 36525 | @doc raw"""
DenseLQDynamicModel(dnlp::LQDynamicData; implicit = false) -> DenseLQDynamicModel
DenseLQDynamicModel(s0, A, B, Q, R, N; implicit = false ...) -> DenseLQDynamicModel
A constructor for building a `DenseLQDynamicModel <: QuadraticModels.AbstractQuadraticModel`
Input data is for the problem of the form
```math
\begin{aligned}
\min \frac{1}{2} &\; \sum_{i = 0}^{N - 1}(s_i^T Q s_i + 2 u_i^T S^T x_i + u_i^T R u_i) + \frac{1}{2} s_N^T Q_f s_N \\
\textrm{s.t.} &\; s_{i+1} = A s_i + B u_i + w_i \quad \forall i=0, 1, ..., N-1 \\
&\; u_i = Kx_i + v_i \quad \forall i = 0, 1, ..., N - 1 \\
&\; gl \le E s_i + F u_i \le gu \quad \forall i = 0, 1, ..., N-1\\
&\; sl \le s \le su \\
&\; ul \le u \le uu \\
&\; s_0 = s0
\end{aligned}
```
---
Data is converted to the form
```math
\begin{aligned}
\min &\; \frac{1}{2} z^T H z \\
\textrm{s.t.} &\; \textrm{lcon} \le Jz \le \textrm{ucon}\\
&\; \textrm{lvar} \le z \le \textrm{uvar}
\end{aligned}
```
Resulting `H`, `J`, `h`, and `h0` matrices are stored within `QuadraticModels.QPData` as `H`, `A`, `c`, and `c0` attributes respectively
If `K` is defined, then `u` variables are replaced by `v` variables. The bounds on `u` are transformed into algebraic constraints,
and `u` can be queried by `get_u` and `get_s` within `DynamicNLPModels.jl`
Keyword argument `implicit = false` determines how the Jacobian is stored within the `QPData`. If `implicit = false`, the full, dense
Jacobian matrix is stored. If `implicit = true`, only the first `nu` columns of the Jacobian are stored with the Linear Operator `LQJacobianOperator`.
"""
function DenseLQDynamicModel(
dnlp::LQDynamicData{T, V, M};
implicit::Bool = false,
) where {
T,
V <: AbstractVector{T},
M <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix{T}},
}
if implicit
_build_implicit_dense_lq_dynamic_model(dnlp)
else
_build_dense_lq_dynamic_model(dnlp)
end
end
function DenseLQDynamicModel(
s0::V,
A::M,
B::M,
Q::M,
R::M,
N;
Qf::M = Q,
S::M = _init_similar(Q, size(Q, 1), size(R, 1), T),
E::M = _init_similar(Q, 0, length(s0), T),
F::M = _init_similar(Q, 0, size(R, 1), T),
K::MK = nothing,
w::V = _init_similar(s0, length(s0) * N, T),
sl::V = (similar(s0) .= -Inf),
su::V = (similar(s0) .= Inf),
ul::V = (similar(s0, size(R, 1)) .= -Inf),
uu::V = (similar(s0, size(R, 1)) .= Inf),
gl::V = (similar(s0, size(E, 1)) .= -Inf),
gu::V = (similar(s0, size(F, 1)) .= Inf),
implicit = false,
) where {
T,
V <: AbstractVector{T},
M <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix{T}},
}
dnlp = LQDynamicData(
s0,
A,
B,
Q,
R,
N;
Qf = Qf,
S = S,
E = E,
F = F,
K = K,
w = w,
sl = sl,
su = su,
ul = ul,
uu = uu,
gl = gl,
gu = gu,
)
DenseLQDynamicModel(dnlp; implicit = implicit)
end
function _build_dense_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: Nothing}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
bool_vec_s = (su .!= Inf .|| sl .!= -Inf)
num_real_bounds_s = sum(bool_vec_s)
dense_blocks = _build_block_matrices(A, B, K, N, w, nc)
block_A = dense_blocks.A
block_B = dense_blocks.B
block_d = dense_blocks.d
block_Aw = dense_blocks.Aw
block_dw = dense_blocks.dw
H_blocks = _build_H_blocks(Q, R, block_A, block_B, block_Aw, S, Qf, K, s0, N)
H = H_blocks.H
c0 = H_blocks.c0
dense_blocks.h .= H_blocks.block_h
dense_blocks.h01 .= H_blocks.block_h01
dense_blocks.h02 .= H_blocks.block_h02
dense_blocks.h_constant .= H_blocks.h_constant
dense_blocks.h0_constant = H_blocks.h0_constant
G = _init_similar(Q, nc * N, nu, T)
J = _init_similar(Q, nc * N + num_real_bounds_s * N, nu * N, T)
As0_bounds = _init_similar(s0, num_real_bounds_s * N, T)
dl = repeat(gl, N)
du = repeat(gu, N)
_set_G_blocks!(G, dl, du, block_B, block_A, block_d, block_Aw, block_dw, s0, E, F, K, N)
_set_J1_dense!(J, G, N)
As0 = _init_similar(s0, ns * (N + 1), T)
LinearAlgebra.mul!(As0, block_A, s0)
lvar = repeat(ul, N)
uvar = repeat(uu, N)
# Convert state variable constraints to algebraic constraints
offset_s = N * nc
if num_real_bounds_s == length(sl)
As0_bounds .= As0[(1 + ns):(ns * (N + 1))] .+ block_Aw[(1 + ns):(ns * (N + 1))]
for i = 1:N
J[
(offset_s + 1 + (i - 1) * ns):(offset_s + ns * N),
(1 + nu * (i - 1)):(nu * i),
] = @view(block_B[1:(ns * (N - i + 1)), :])
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_s):(i * num_real_bounds_s)
As0_bounds[row_range] .=
As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .+
block_Aw[(1 + ns * i):(ns * (i + 1))][bool_vec_s]
for j = 1:(N - i + 1)
J[
(offset_s + 1 + (i + j - 2) * num_real_bounds_s):(offset_s + (i + j - 1) * num_real_bounds_s),
(1 + nu * (i - 1)):(nu * i),
] = @view(block_B[(1 + (j - 1) * ns):(j * ns), :][bool_vec_s, :])
end
end
sl = sl[bool_vec_s]
su = su[bool_vec_s]
end
lcon2 = repeat(sl, N)
ucon2 = repeat(su, N)
LinearAlgebra.axpy!(-1, As0_bounds, ucon2)
LinearAlgebra.axpy!(-1, As0_bounds, lcon2)
lcon = _init_similar(s0, length(dl) + length(lcon2), T)
ucon = _init_similar(s0, length(du) + length(ucon2), T)
lcon[1:length(dl)] = dl
ucon[1:length(du)] = du
if length(lcon2) > 0
lcon[(1 + length(dl)):(length(dl) + num_real_bounds_s * N)] = lcon2
ucon[(1 + length(du)):(length(du) + num_real_bounds_s * N)] = ucon2
end
nvar = nu * N
nnzj = size(J, 1) * size(J, 2)
nh = size(H, 1)
nnzh = div(nh * (nh + 1), 2)
ncon = size(J, 1)
c = _init_similar(s0, nvar, T)
c .= H_blocks.c
DenseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
dense_blocks,
)
end
function _build_dense_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: AbstractMatrix{T}}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
dense_blocks = _build_block_matrices(A, B, K, N, w, nc)
block_A = dense_blocks.A
block_B = dense_blocks.B
block_d = dense_blocks.d
block_Aw = dense_blocks.Aw
block_dw = dense_blocks.dw
block_KAw = dense_blocks.KAw
H_blocks = _build_H_blocks(Q, R, block_A, block_B, block_Aw, S, Qf, K, s0, N)
H = H_blocks.H
c0 = H_blocks.c0
dense_blocks.h .= H_blocks.block_h
dense_blocks.h01 .= H_blocks.block_h01
dense_blocks.h02 .= H_blocks.block_h02
dense_blocks.h_constant .= H_blocks.h_constant
dense_blocks.h0_constant = H_blocks.h0_constant
bool_vec_s = (su .!= Inf .|| sl .!= -Inf)
num_real_bounds_s = sum(bool_vec_s)
bool_vec_u = (ul .!= -Inf .|| uu .!= Inf)
num_real_bounds_u = sum(bool_vec_u)
G = _init_similar(Q, nc * N, nu, T)
J = _init_similar(Q, (nc + num_real_bounds_s + num_real_bounds_u) * N, nu * N, T)
As0 = _init_similar(s0, ns * (N + 1), T)
As0_bounds = _init_similar(s0, num_real_bounds_s * N, T)
KAs0_bounds = _init_similar(s0, num_real_bounds_u * N, T)
KBI = _init_similar(Q, nu * N, nu, T)
KAs0 = _init_similar(s0, nu * N, T)
KAs0_block = _init_similar(s0, nu, T)
KB = _init_similar(Q, nu, nu, T)
I_mat = _init_similar(Q, nu, nu, T)
I_mat[LinearAlgebra.diagind(I_mat)] .= T(1)
dl = repeat(gl, N)
du = repeat(gu, N)
_set_G_blocks!(G, dl, du, block_B, block_A, block_d, block_Aw, block_dw, s0, E, F, K, N)
_set_J1_dense!(J, G, N)
LinearAlgebra.mul!(As0, block_A, s0)
# Convert state variable constraints to algebraic constraints
offset_s = nc * N
if num_real_bounds_s == length(sl)
As0_bounds .= As0[(1 + ns):(ns * (N + 1))] .+ block_Aw[(1 + ns):(ns * (N + 1))]
for i = 1:N
J[
(offset_s + 1 + (i - 1) * ns):(offset_s + ns * N),
(1 + nu * (i - 1)):(nu * i),
] = @view(block_B[1:(ns * (N - i + 1)), :])
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_s):(i * num_real_bounds_s)
As0_bounds[row_range] =
As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .+
block_Aw[(1 + ns * i):(ns * (i + 1))][bool_vec_s]
for j = 1:(N - i + 1)
J[
(offset_s + 1 + (i + j - 2) * num_real_bounds_s):(offset_s + (i + j - 1) * num_real_bounds_s),
(1 + nu * (i - 1)):(nu * i),
] = @view(block_B[(1 + (j - 1) * ns):(j * ns), :][bool_vec_s, :])
end
end
sl = sl[bool_vec_s]
su = su[bool_vec_s]
end
# Convert bounds on u to algebraic constraints
for i = 1:N
if i == 1
KB = I_mat
else
B_row_range = (1 + (i - 2) * ns):((i - 1) * ns)
B_sub_block = view(block_B, B_row_range, :)
LinearAlgebra.mul!(KB, K, B_sub_block)
end
KBI[(1 + nu * (i - 1)):(nu * i), :] = KB
LinearAlgebra.mul!(KAs0_block, K, As0[(1 + ns * (i - 1)):(ns * i)])
KAs0[(1 + nu * (i - 1)):(nu * i)] = KAs0_block
end
offset_u = nc * N + num_real_bounds_s * N
if num_real_bounds_u == length(ul)
KAs0_bounds .= KAs0 .+ block_KAw
for i = 1:N
J[
(offset_u + 1 + (i - 1) * nu):(offset_u + nu * N),
(1 + nu * (i - 1)):(nu * i),
] = @view(KBI[1:(nu * (N - i + 1)), :])
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_u):(i * num_real_bounds_u)
KAs0_bounds[row_range] =
KAs0[(1 + nu * (i - 1)):(nu * i)][bool_vec_u] .+
block_KAw[(1 + nu * (i - 1)):(nu * i)][bool_vec_u]
for j = 1:(N - i + 1)
J[
(offset_u + 1 + (i + j - 2) * num_real_bounds_u):(offset_u + (i + j - 1) * num_real_bounds_u),
(1 + nu * (i - 1)):(nu * i),
] = @view(KBI[(1 + (j - 1) * nu):(j * nu), :][bool_vec_u, :])
end
end
ul = ul[bool_vec_u]
uu = uu[bool_vec_u]
end
lcon2 = repeat(sl, N)
ucon2 = repeat(su, N)
lcon3 = repeat(ul, N)
ucon3 = repeat(uu, N)
LinearAlgebra.axpy!(-1, As0_bounds, lcon2)
LinearAlgebra.axpy!(-1, As0_bounds, ucon2)
LinearAlgebra.axpy!(-1, KAs0_bounds, lcon3)
LinearAlgebra.axpy!(-1, KAs0_bounds, ucon3)
lcon = _init_similar(s0, size(J, 1), T)
ucon = _init_similar(s0, size(J, 1), T)
lcon[1:length(dl)] = dl
ucon[1:length(du)] = du
if length(lcon2) > 0
lcon[(length(dl) + 1):(length(dl) + length(lcon2))] = lcon2
ucon[(length(du) + 1):(length(du) + length(ucon2))] = ucon2
end
if length(lcon3) > 0
lcon[(length(dl) + length(lcon2) + 1):(length(dl) + length(lcon2) + length(
lcon3,
))] = lcon3
ucon[(length(du) + length(ucon2) + 1):(length(du) + length(ucon2) + length(
ucon3,
))] = ucon3
end
nvar = nu * N
nnzj = size(J, 1) * size(J, 2)
nh = size(H, 1)
nnzh = div(nh * (nh + 1), 2)
ncon = size(J, 1)
c = _init_similar(s0, nvar, T)
c .= H_blocks.c
DenseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
dense_blocks,
)
end
function _build_implicit_dense_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: Nothing}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
nvar = nu * N
bool_vec_s = (su .!= Inf .|| sl .!= -Inf)
num_real_bounds_s = sum(bool_vec_s)
G = _init_similar(Q, nc * N, nu, T)
Jac1 = _init_similar(Q, nc, nu, N, T)
Jac2 = _init_similar(Q, num_real_bounds_s, nu, N, T)
Jac3 = _init_similar(Q, 0, nu, N, T)
As0 = _init_similar(s0, ns * (N + 1), T)
As0_bounds = _init_similar(s0, num_real_bounds_s * N, T)
c = _init_similar(s0, nvar, T)
x0 = _init_similar(s0, nvar, T)
lcon = _init_similar(s0, nc * N + num_real_bounds_s * N, T)
ucon = _init_similar(s0, nc * N + num_real_bounds_s * N, T)
x1 = _init_similar(Q, nc, 1, N, T)
x2 = _init_similar(Q, num_real_bounds_s, 1, N, T)
x3 = _init_similar(Q, 0, 1, N, T)
y = _init_similar(Q, nu, 1, N, T)
SJ1 = _init_similar(Q, nc, nu, T)
SJ2 = _init_similar(Q, num_real_bounds_s, nu, T)
SJ3 = _init_similar(Q, 0, nu, T)
H_sub_block = _init_similar(Q, nu, nu, T)
dense_blocks = _build_block_matrices(A, B, K, N, w, nc)
block_A = dense_blocks.A
block_B = dense_blocks.B
block_d = dense_blocks.d
block_Aw = dense_blocks.Aw
block_dw = dense_blocks.dw
H_blocks = _build_H_blocks(Q, R, block_A, block_B, block_Aw, S, Qf, K, s0, N)
H = H_blocks.H
c0 = H_blocks.c0
dense_blocks.h .= H_blocks.block_h
dense_blocks.h01 .= H_blocks.block_h01
dense_blocks.h02 .= H_blocks.block_h02
dense_blocks.h_constant .= H_blocks.h_constant
dense_blocks.h0_constant = H_blocks.h0_constant
dl = repeat(gl, N)
du = repeat(gu, N)
_set_G_blocks!(G, dl, du, block_B, block_A, block_d, block_Aw, block_dw, s0, E, F, K, N)
for i = 1:N
Jac1[:, :, i] = @view G[(1 + nc * (i - 1)):(nc * i), :]
end
LinearAlgebra.mul!(As0, block_A, s0)
lvar = repeat(ul, N)
uvar = repeat(uu, N)
# Convert state variable constraints to algebraic constraints
if num_real_bounds_s == length(sl)
As0_bounds .= As0[(1 + ns):(ns * (N + 1))] .+ block_Aw[(1 + ns):(ns * (N + 1))]
for i = 1:N
Jac2[:, :, i] = @view block_B[(1 + ns * (i - 1)):(ns * i), :]
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_s):(i * num_real_bounds_s)
As0_bounds[row_range] .=
As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .+
block_Aw[(1 + ns * i):(ns * (i + 1))][bool_vec_s]
Jac2[:, :, i] = @view block_B[(1 + (i - 1) * ns):(i * ns), :][bool_vec_s, :]
end
sl = sl[bool_vec_s]
su = su[bool_vec_s]
end
lcon2 = repeat(sl, N)
ucon2 = repeat(su, N)
LinearAlgebra.axpy!(-1, As0_bounds, ucon2)
LinearAlgebra.axpy!(-1, As0_bounds, lcon2)
lcon[1:length(dl)] = dl
ucon[1:length(du)] = du
if length(lcon2) > 0
lcon[(1 + length(dl)):(length(dl) + num_real_bounds_s * N)] = lcon2
ucon[(1 + length(du)):(length(du) + num_real_bounds_s * N)] = ucon2
end
ncon = (nc + num_real_bounds_s) * N
nnzj = ncon * size(H, 2)
nh = size(H, 1)
nnzh = div(nh * (nh + 1), 2)
J = LQJacobianOperator{T, M, AbstractArray{T}}(
Jac1,
Jac2,
Jac3,
N,
nu,
nc,
num_real_bounds_s,
0,
x1,
x2,
x3,
y,
SJ1,
SJ2,
SJ3,
H_sub_block,
)
DenseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = x0,
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
dense_blocks,
)
end
function _build_implicit_dense_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: AbstractMatrix{T}}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
dense_blocks = _build_block_matrices(A, B, K, N, w, nc)
block_A = dense_blocks.A
block_B = dense_blocks.B
block_d = dense_blocks.d
block_Aw = dense_blocks.Aw
block_dw = dense_blocks.dw
block_KAw = dense_blocks.KAw
H_blocks = _build_H_blocks(Q, R, block_A, block_B, block_Aw, S, Qf, K, s0, N)
H = H_blocks.H
c0 = H_blocks.c0
dense_blocks.h .= H_blocks.block_h
dense_blocks.h01 .= H_blocks.block_h01
dense_blocks.h02 .= H_blocks.block_h02
dense_blocks.h_constant .= H_blocks.h_constant
dense_blocks.h0_constant = H_blocks.h0_constant
bool_vec_s = (su .!= Inf .|| sl .!= -Inf)
num_real_bounds_s = sum(bool_vec_s)
bool_vec_u = (ul .!= -Inf .|| uu .!= Inf)
num_real_bounds_u = sum(bool_vec_u)
G = _init_similar(Q, nc * N, nu, T)
Jac1 = _init_similar(Q, nc, nu, N, T)
Jac2 = _init_similar(Q, num_real_bounds_s, nu, N, T)
Jac3 = _init_similar(Q, num_real_bounds_u, nu, N, T)
As0 = _init_similar(s0, ns * (N + 1), T)
As0_bounds = _init_similar(s0, num_real_bounds_s * N, T)
KAs0_bounds = _init_similar(s0, num_real_bounds_u * N, T)
KBI = _init_similar(Q, nu * N, nu, T)
KAs0 = _init_similar(s0, nu * N, T)
KAs0_block = _init_similar(s0, nu, T)
KB = _init_similar(Q, nu, nu, T)
lcon = _init_similar(s0, (nc + num_real_bounds_s + num_real_bounds_u) * N, T)
ucon = _init_similar(s0, (nc + num_real_bounds_s + num_real_bounds_u) * N, T)
I_mat = _init_similar(Q, nu, nu, T)
x1 = _init_similar(Q, nc, 1, N, T)
x2 = _init_similar(Q, num_real_bounds_s, 1, N, T)
x3 = _init_similar(Q, num_real_bounds_u, 1, N, T)
y = _init_similar(Q, nu, 1, N, T)
SJ1 = _init_similar(Q, nc, nu, T)
SJ2 = _init_similar(Q, num_real_bounds_s, nu, T)
SJ3 = _init_similar(Q, num_real_bounds_u, nu, T)
H_sub_block = _init_similar(Q, nu, nu, T)
I_mat[LinearAlgebra.diagind(I_mat)] .= T(1)
dl = repeat(gl, N)
du = repeat(gu, N)
_set_G_blocks!(G, dl, du, block_B, block_A, block_d, block_Aw, block_dw, s0, E, F, K, N)
for i = 1:N
Jac1[:, :, i] = @view G[(1 + nc * (i - 1)):(nc * i), :]
end
LinearAlgebra.mul!(As0, block_A, s0)
# Convert state variable constraints to algebraic constraints
offset_s = nc * N
if num_real_bounds_s == length(sl)
As0_bounds .= As0[(1 + ns):(ns * (N + 1))] .+ block_Aw[(1 + ns):(ns * (N + 1))]
for i = 1:N
Jac2[:, :, i] = @view block_B[(1 + ns * (i - 1)):(ns * i), :]
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_s):(i * num_real_bounds_s)
As0_bounds[row_range] .=
As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .+
block_Aw[(1 + ns * i):(ns * (i + 1))][bool_vec_s]
Jac2[:, :, i] = @view block_B[(1 + (i - 1) * ns):(i * ns), :][bool_vec_s, :]
end
sl = sl[bool_vec_s]
su = su[bool_vec_s]
end
# Convert bounds on u to algebraic constraints
for i = 1:N
if i == 1
KB = I_mat
else
B_row_range = (1 + (i - 2) * ns):((i - 1) * ns)
B_sub_block = view(block_B, B_row_range, :)
LinearAlgebra.mul!(KB, K, B_sub_block)
end
KBI[(1 + nu * (i - 1)):(nu * i), :] = KB
LinearAlgebra.mul!(KAs0_block, K, As0[(1 + ns * (i - 1)):(ns * i)])
KAs0[(1 + nu * (i - 1)):(nu * i)] = KAs0_block
end
offset_u = nc * N + num_real_bounds_s * N
if num_real_bounds_u == length(ul)
KAs0_bounds .= KAs0 .+ block_KAw
for i = 1:N
Jac3[:, :, i] = @view KBI[(1 + (i - 1) * nu):(i * nu), :]
end
else
for i = 1:N
row_range = (1 + (i - 1) * num_real_bounds_u):(i * num_real_bounds_u)
KAs0_bounds[row_range] =
KAs0[(1 + nu * (i - 1)):(nu * i)][bool_vec_u] .+
block_KAw[(1 + nu * (i - 1)):(nu * i)][bool_vec_u]
Jac3[:, :, i] = @view KBI[(1 + (i - 1) * nu):(i * nu), :][bool_vec_u, :]
end
ul = ul[bool_vec_u]
uu = uu[bool_vec_u]
end
lcon2 = repeat(sl, N)
ucon2 = repeat(su, N)
lcon3 = repeat(ul, N)
ucon3 = repeat(uu, N)
LinearAlgebra.axpy!(-1, As0_bounds, lcon2)
LinearAlgebra.axpy!(-1, As0_bounds, ucon2)
LinearAlgebra.axpy!(-1, KAs0_bounds, lcon3)
LinearAlgebra.axpy!(-1, KAs0_bounds, ucon3)
lcon[1:length(dl)] = dl
ucon[1:length(du)] = du
if length(lcon2) > 0
lcon[(length(dl) + 1):(length(dl) + length(lcon2))] = lcon2
ucon[(length(du) + 1):(length(du) + length(ucon2))] = ucon2
end
if length(lcon3) > 0
lcon[(length(dl) + length(lcon2) + 1):(length(dl) + length(lcon2) + length(
lcon3,
))] = lcon3
ucon[(length(du) + length(ucon2) + 1):(length(du) + length(ucon2) + length(
ucon3,
))] = ucon3
end
nvar = nu * N
ncon = (nc + num_real_bounds_s + num_real_bounds_u) * N
nnzj = ncon * size(H, 1)
nh = size(H, 1)
nnzh = div(nh * (nh + 1), 2)
c = _init_similar(s0, nvar, T)
c .= H_blocks.c
J = LQJacobianOperator{T, M, AbstractArray{T}}(
Jac1,
Jac2,
Jac3,
N,
nu,
nc,
num_real_bounds_s,
num_real_bounds_u,
x1,
x2,
x3,
y,
SJ1,
SJ2,
SJ3,
H_sub_block,
)
DenseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
dense_blocks,
)
end
function _build_block_matrices(
A::M,
B::M,
K,
N,
w::V,
nc,
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}}
ns = size(A, 2)
nu = size(B, 2)
if K == nothing
K = _init_similar(A, nu, ns, T)
end
# Define block matrices
block_A = _init_similar(A, ns * (N + 1), ns, T)
block_B = _init_similar(B, ns * N, nu, T)
block_Aw = _init_similar(w, ns * (N + 1), T)
block_h = _init_similar(A, nu * N, ns, T)
block_h01 = _init_similar(A, ns, ns, T)
block_h02 = _init_similar(w, ns, T)
h_const = _init_similar(w, nu * N, T)
h0_const = T(0)
block_d = _init_similar(A, nc * N, ns, T)
block_dw = _init_similar(w, nc * N, T)
block_KA = _init_similar(A, nu * N, ns, T)
block_KAw = _init_similar(w, nu * N, T)
A_k = copy(A)
BK = _init_similar(A, ns, ns, T)
KA = _init_similar(A, nu, ns, T)
KAw = _init_similar(w, nu, T)
Aw = _init_similar(A, ns, T)
AB_klast = _init_similar(A, size(B, 1), size(B, 2), T)
AB_k = _init_similar(A, size(B, 1), size(B, 2), T)
block_B[1:ns, :] = B
block_A[LinearAlgebra.diagind(block_A)] .= T(1)
LinearAlgebra.mul!(BK, B, K)
LinearAlgebra.axpy!(1, BK, A_k)
A_klast = copy(A_k)
A_knext = copy(A_k)
block_A[(ns + 1):(ns * 2), :] = A_k
LinearAlgebra.mul!(AB_k, A_k, B, 1, 0)
block_B[(1 + ns):(2 * ns), :] = AB_k
AB_klast = copy(AB_k)
# Fill the A and B matrices
LinearAlgebra.mul!(KA, K, A_k)
block_KA[1:nu, :] .= K
block_KA[(1 + nu):(2 * nu), :] .= KA
for i = 2:(N - 1)
LinearAlgebra.mul!(AB_k, A_k, AB_klast)
LinearAlgebra.mul!(A_knext, A_k, A_klast)
block_A[(ns * i + 1):(ns * (i + 1)), :] = A_knext
block_B[(1 + (i) * ns):((i + 1) * ns), :] = AB_k
LinearAlgebra.mul!(KA, K, A_knext)
block_KA[(1 + nu * i):(nu * (i + 1)), :] .= KA
AB_klast = copy(AB_k)
A_klast = copy(A_knext)
end
LinearAlgebra.mul!(A_knext, A_k, A_klast)
block_A[(ns * N + 1):(ns * (N + 1)), :] = A_knext
for i = 1:N
A_view = @view block_A[(1 + (i - 1) * ns):(i * ns), :]
for j = (i + 1):(N + 1)
LinearAlgebra.mul!(Aw, A_view, w[(1 + (j - i - 1) * ns):((j - i) * ns)])
block_Aw[(1 + (j - 1) * ns):(j * ns)] .+= Aw
end
end
for i = 1:N
Aw_view = @view block_Aw[(1 + (i - 1) * ns):(i * ns)]
LinearAlgebra.mul!(KAw, K, Aw_view)
block_KAw[(1 + (i - 1) * nu):(i * nu)] .= KAw
end
DenseLQDynamicBlocks{T, V, M}(
block_A,
block_B,
block_Aw,
block_h,
block_h01,
block_h02,
h_const,
h0_const,
block_d,
block_dw,
block_KA,
block_KAw,
)
end
function _build_H_blocks(
Q,
R,
block_A::M,
block_B::M,
Aw,
S,
Qf,
K,
s0,
N,
) where {T, M <: AbstractMatrix{T}}
ns = size(Q, 1)
nu = size(R, 1)
if K == nothing
K = _init_similar(Q, nu, ns, T)
end
H = _init_similar(block_A, nu * N, nu * N, T)
# block_h01, block_h02, and block_h are stored in DenseLQDynamicBlocks to provide quick updates when redefining s0
# block_h01 = A^T((Q + KTRK + 2 * SK))A where Q, K, R, S, and A are block matrices
# block_h02 = A^T((Q + KTRK + 2 * SK))block_matrix_A w
# block_h = (QB + SKB + K^T R K B + K^T S^T B)^T A + (S + K^T R)^T A
block_h01 = _init_similar(Q, ns, ns, T)
block_h02 = _init_similar(s0, ns, T)
block_h = _init_similar(block_A, nu * N, ns, T)
h_constant = _init_similar(s0, nu * N, T)
h0_constant = T(0)
# quad term refers to the summation of Q, K^T RK, SK, and K^T S^T that is left and right multiplied by B in the Hessian
quad_term = _init_similar(Q, ns, ns, T)
quad_term_B = _init_similar(block_B, size(block_B, 1), size(block_B, 2), T)
QfB = _init_similar(block_B, size(block_B, 1), size(block_B, 2), T)
quad_term_AB = _init_similar(block_A, ns, nu, T)
QfAB = _init_similar(block_A, ns, nu, T)
RK_STB = _init_similar(block_B, nu, nu, T)
BQB = _init_similar(block_B, nu, nu, T)
BQfB = _init_similar(block_B, nu, nu, T)
SK = _init_similar(Q, ns, ns, T)
RK = _init_similar(Q, nu, ns, T)
KTRK = _init_similar(Q, ns, ns, T)
RK_ST = _init_similar(Q, nu, ns, T)
QB_block_vec = _init_similar(quad_term_B, ns * (N + 1), nu, T)
h = _init_similar(s0, nu * N, T)
BTQA = _init_similar(Q, nu, ns, T)
RK_STA = _init_similar(Q, nu, ns, T)
BTQAw = _init_similar(s0, nu, T)
RK_STAw = _init_similar(s0, nu, T)
QA = _init_similar(Q, ns, ns, T)
KTRKA = _init_similar(Q, ns, ns, T)
SKA = _init_similar(Q, ns, ns, T)
KTSTA = _init_similar(Q, ns, ns, T)
QAw = _init_similar(s0, ns, T)
KTRKAw = _init_similar(s0, ns, T)
SKAw = _init_similar(s0, ns, T)
KTSTAw = _init_similar(s0, ns, T)
AQAs0 = _init_similar(s0, ns, T)
LinearAlgebra.mul!(SK, S, K)
LinearAlgebra.mul!(RK, R, K)
LinearAlgebra.mul!(KTRK, K', RK)
LinearAlgebra.axpy!(1.0, Q, quad_term)
LinearAlgebra.axpy!(1.0, SK, quad_term)
# axpy!(1.0, SK', quad_term) includes scalar operations because of the adjoint
# .+= is more efficient with adjoint
quad_term .+= SK'
LinearAlgebra.axpy!(1.0, KTRK, quad_term)
LinearAlgebra.copyto!(RK_ST, RK)
RK_ST .+= S'
for i = 1:N
B_row_range = (1 + (i - 1) * ns):(i * ns)
B_sub_block = view(block_B, B_row_range, :)
LinearAlgebra.mul!(quad_term_AB, quad_term, B_sub_block)
LinearAlgebra.mul!(QfAB, Qf, B_sub_block)
quad_term_B[(1 + (i - 1) * ns):(i * ns), :] = quad_term_AB
QfB[(1 + (i - 1) * ns):(i * ns), :] = QfAB
for j = 1:(N + 1 - i)
right_block = block_B[(1 + (j - 1 + i - 1) * ns):((j + i - 1) * ns), :]
LinearAlgebra.mul!(BQB, quad_term_AB', right_block)
LinearAlgebra.mul!(BQfB, QfAB', right_block)
for k = 1:(N - j - i + 2)
row_range = (1 + nu * (k + (j - 1) - 1)):(nu * (k + (j - 1)))
col_range = (1 + nu * (k - 1)):(nu * k)
if k == N - j - i + 2
view(H, row_range, col_range) .+= BQfB
else
view(H, row_range, col_range) .+= BQB
end
end
end
LinearAlgebra.mul!(RK_STB, RK_ST, B_sub_block)
for m = 1:(N - i)
row_range = (1 + nu * (m - 1 + i)):(nu * (m + i))
col_range = (1 + nu * (m - 1)):(nu * m)
view(H, row_range, col_range) .+= RK_STB
end
view(H, (1 + nu * (i - 1)):(nu * i), (1 + nu * (i - 1)):(nu * i)) .+= R
end
for i = 1:N
# quad_term_B = QB + SKB + KTRKB + KTSTB = (Q + SK + KTRK + KTST) B
fill!(QB_block_vec, T(0))
rows_QB = 1:(ns * (N - i))
rows_QfB = (1 + ns * (N - i)):(ns * (N - i + 1))
QB_block_vec[(1 + ns * i):(ns * N), :] = quad_term_B[rows_QB, :]
QB_block_vec[(1 + ns * N):(ns * (N + 1)), :] = QfB[rows_QfB, :]
LinearAlgebra.mul!(BTQA, QB_block_vec', block_A)
LinearAlgebra.mul!(RK_STA, RK_ST, block_A[(ns * (i - 1) + 1):(ns * i), :])
LinearAlgebra.mul!(BTQAw, QB_block_vec', Aw)
LinearAlgebra.mul!(RK_STAw, RK_ST, Aw[(1 + ns * (i - 1)):(ns * i)])
h_view = @view block_h[(1 + nu * (i - 1)):(nu * i), :]
LinearAlgebra.axpy!(1, BTQA, h_view)
LinearAlgebra.axpy!(1, RK_STA, h_view)
h_constant_view = @view h_constant[(1 + nu * (i - 1)):(nu * i)]
LinearAlgebra.axpy!(1, BTQAw, h_constant_view)
LinearAlgebra.axpy!(1, RK_STAw, h_constant_view)
A_view = @view block_A[(1 + ns * (i - 1)):(ns * i), :]
Aw_view = @view Aw[(1 + ns * (i - 1)):(ns * i)]
LinearAlgebra.mul!(QA, Q, A_view)
LinearAlgebra.mul!(KTRKA, KTRK, A_view)
LinearAlgebra.mul!(SKA, SK, A_view)
LinearAlgebra.mul!(KTSTA, SK', A_view)
LinearAlgebra.mul!(QAw, Q, Aw_view)
LinearAlgebra.mul!(KTRKAw, KTRK, Aw_view)
LinearAlgebra.mul!(SKAw, SK, Aw_view)
LinearAlgebra.mul!(KTSTAw, SK', Aw_view)
LinearAlgebra.mul!(block_h01, A_view', QA, 1, 1)
LinearAlgebra.mul!(block_h01, A_view', KTRKA, 1, 1)
LinearAlgebra.mul!(block_h01, A_view', SKA, 1, 1)
LinearAlgebra.mul!(block_h01, A_view', KTSTA, 1, 1)
LinearAlgebra.mul!(block_h02, A_view', QAw, 1, 1)
LinearAlgebra.mul!(block_h02, A_view', KTRKAw, 1, 1)
LinearAlgebra.mul!(block_h02, A_view', SKAw, 1, 1)
LinearAlgebra.mul!(block_h02, A_view', KTSTAw, 1, 1)
h0_constant += LinearAlgebra.dot(Aw_view, QAw)
h0_constant += LinearAlgebra.dot(Aw_view, KTRKAw)
h0_constant += LinearAlgebra.dot(Aw_view, SKAw)
h0_constant += LinearAlgebra.dot(Aw_view, KTSTAw)
end
A_view = @view block_A[(1 + ns * N):(ns * (N + 1)), :]
Aw_view = @view Aw[(1 + ns * N):(ns * (N + 1))]
LinearAlgebra.mul!(QA, Qf, A_view)
LinearAlgebra.mul!(block_h01, A_view', QA, 1, 1)
LinearAlgebra.mul!(QAw, Qf, Aw_view)
LinearAlgebra.mul!(block_h02, A_view', QAw, 1, 1)
h0_constant += LinearAlgebra.dot(Aw_view, QAw)
#LinearAlgebra.mul!(h0_constant, Aw_view', QAw, 1, 1)
LinearAlgebra.mul!(h, block_h, s0)
LinearAlgebra.mul!(AQAs0, block_h01, s0)
h0 = LinearAlgebra.dot(AQAs0, s0)
h0 += h0_constant
h0 += LinearAlgebra.dot(block_h02, s0) * T(2)
h += h_constant
return (
H = H,
c = h,
c0 = h0 / T(2),
block_h = block_h,
block_h01 = block_h01,
block_h02 = block_h02,
h_constant = h_constant,
h0_constant = h0_constant / T(2),
)
end
function _set_G_blocks!(
G,
dl,
du,
block_B::M,
block_A::M,
block_d::M,
block_Aw,
block_dw,
s0,
E,
F,
K::MK,
N,
) where {T, M <: AbstractMatrix{T}, MK <: Nothing}
ns = size(E, 2)
nu = size(F, 2)
nc = size(E, 1)
G[1:nc, :] = F
EB = _init_similar(block_B, nc, nu, T)
EA = _init_similar(block_B, nc, ns, T)
d = _init_similar(block_dw, nc, T)
for i = 1:N
if i != N
B_row_range = (1 + (i - 1) * ns):(i * ns)
B_sub_block = view(block_B, B_row_range, :)
LinearAlgebra.mul!(EB, E, B_sub_block)
G[(1 + nc * i):(nc * (i + 1)), :] = EB
end
A_view = @view block_A[(1 + ns * (i - 1)):(ns * i), :]
Aw_view = @view block_Aw[(1 + ns * (i - 1)):(ns * i)]
LinearAlgebra.mul!(EA, E, A_view)
LinearAlgebra.mul!(d, E, Aw_view)
block_d[(1 + nc * (i - 1)):(nc * i), :] .= EA
block_dw[(1 + nc * (i - 1)):(nc * i)] .= d
end
LinearAlgebra.mul!(dl, block_d, s0, -1, 1)
LinearAlgebra.mul!(du, block_d, s0, -1, 1)
LinearAlgebra.axpy!(-1, block_dw, dl)
LinearAlgebra.axpy!(-1, block_dw, du)
end
function _set_G_blocks!(
G,
dl,
du,
block_B,
block_A,
block_d,
block_Aw,
block_dw,
s0,
E,
F,
K::MK,
N,
) where {T, MK <: AbstractMatrix{T}}
ns = size(E, 2)
nu = size(F, 2)
nc = size(E, 1)
G[1:nc, :] = F
E_FK = _init_similar(E, nc, ns, T)
E_FKA = _init_similar(E, nc, ns, T)
FK = _init_similar(E, nc, ns, T)
EB = _init_similar(E, nc, nu, T)
d = _init_similar(s0, nc, T)
LinearAlgebra.copyto!(E_FK, E)
LinearAlgebra.mul!(FK, F, K)
LinearAlgebra.axpy!(1.0, FK, E_FK)
for i = 1:N
if i != N
B_row_range = (1 + (i - 1) * ns):(i * ns)
B_sub_block = view(block_B, B_row_range, :)
LinearAlgebra.mul!(EB, E_FK, B_sub_block)
G[(1 + nc * i):(nc * (i + 1)), :] = EB
end
A_view = @view block_A[(1 + ns * (i - 1)):(ns * i), :]
Aw_view = @view block_Aw[(1 + ns * (i - 1)):(ns * i)]
LinearAlgebra.mul!(E_FKA, E_FK, A_view)
LinearAlgebra.mul!(d, E_FK, Aw_view)
block_d[(1 + nc * (i - 1)):(nc * i), :] .= E_FKA
block_dw[(1 + nc * (i - 1)):(nc * i)] .= d
end
LinearAlgebra.mul!(dl, block_d, s0, -1, 1)
LinearAlgebra.mul!(du, block_d, s0, -1, 1)
LinearAlgebra.axpy!(-1, block_dw, dl)
LinearAlgebra.axpy!(-1, block_dw, du)
end
function _set_J1_dense!(J1, G, N)
# Only used for explicit Jacobian, not implicit Jacobian
nu = size(G, 2)
nc = Int(size(G, 1) / N)
for i = 1:N
col_range = (1 + nu * (i - 1)):(nu * i)
J1[(1 + nc * (i - 1)):(nc * N), col_range] = G[1:((N - i + 1) * nc), :]
end
end
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 42029 | @doc raw"""
SparseLQDynamicModel(dnlp::LQDynamicData) -> SparseLQDynamicModel
SparseLQDynamicModel(s0, A, B, Q, R, N; ...) -> SparseLQDynamicModel
A constructor for building a `SparseLQDynamicModel <: QuadraticModels.AbstractQuadraticModel`
Input data is for the problem of the form
```math
\begin{aligned}
\min \frac{1}{2} &\; \sum_{i = 0}^{N - 1}(s_i^T Q s_i + 2 u_i^T S^T x_i + u_i^T R u_i) + \frac{1}{2} s_N^T Q_f s_N \\
\textrm{s.t.} &\; s_{i+1} = A s_i + B u_i + w_i \quad \forall i=0, 1, ..., N-1 \\
&\; u_i = Kx_i + v_i \quad \forall i = 0, 1, ..., N - 1 \\
&\; gl \le E s_i + F u_i \le gu \quad \forall i = 0, 1, ..., N-1\\
&\; sl \le s \le su \\
&\; ul \le u \le uu \\
&\; s_0 = s0
\end{aligned}
```
---
Data is converted to the form
```math
\begin{aligned}
\min &\; \frac{1}{2} z^T H z \\
\textrm{s.t.} &\; \textrm{lcon} \le Jz \le \textrm{ucon}\\
&\; \textrm{lvar} \le z \le \textrm{uvar}
\end{aligned}
```
Resulting `H` and `J` matrices are stored as `QuadraticModels.QPData` within the `SparseLQDynamicModel` struct and
variable and constraint limits are stored within `NLPModels.NLPModelMeta`
If `K` is defined, then `u` variables are replaced by `v` variables, and `u` can be queried by `get_u` and `get_s` within `DynamicNLPModels.jl`
"""
function SparseLQDynamicModel(
dnlp::LQDynamicData{T, V, M},
) where {
T,
V <: AbstractVector{T},
M <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix{T}},
}
_build_sparse_lq_dynamic_model(dnlp)
end
function SparseLQDynamicModel(
s0::V,
A::M,
B::M,
Q::M,
R::M,
N;
Qf::M = Q,
S::M = _init_similar(Q, size(Q, 1), size(R, 1), T),
E::M = _init_similar(Q, 0, length(s0), T),
F::M = _init_similar(Q, 0, size(R, 1), T),
K::MK = nothing,
w::V = _init_similar(s0, length(s0) * N, T),
sl::V = (similar(s0) .= -Inf),
su::V = (similar(s0) .= Inf),
ul::V = (similar(s0, size(R, 1)) .= -Inf),
uu::V = (similar(s0, size(R, 1)) .= Inf),
gl::V = (similar(s0, size(E, 1)) .= -Inf),
gu::V = (similar(s0, size(F, 1)) .= Inf),
) where {
T,
V <: AbstractVector{T},
M <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix{T}},
}
dnlp = LQDynamicData(
s0,
A,
B,
Q,
R,
N;
Qf = Qf,
S = S,
E = E,
F = F,
K = K,
w = w,
sl = sl,
su = su,
ul = ul,
uu = uu,
gl = gl,
gu = gu,
)
SparseLQDynamicModel(dnlp)
end
function _build_sparse_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: Nothing}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
H_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
H_rowval = zeros(Int, (ns + nu) * N * ns + (ns + nu) * N * nu + ns * ns)
H_nzval = zeros(T, (ns + nu) * N * ns + (ns + nu) * N * nu + ns * ns)
J_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
J_rowval = zeros(Int, N * (ns^2 + ns * nu + ns) + N * (nc * ns + nc * nu))
J_nzval = zeros(T, N * (ns^2 + ns * nu + ns) + N * (nc * ns + nc * nu))
_set_sparse_H!(H_colptr, H_rowval, H_nzval, Q, R, N; Qf = Qf, S = S)
H = SparseArrays.SparseMatrixCSC(
(N + 1) * ns + nu * N,
(N + 1) * ns + nu * N,
H_colptr,
H_rowval,
H_nzval,
)
_set_sparse_J!(J_colptr, J_rowval, J_nzval, A, B, E, F, K, N)
J = SparseArrays.SparseMatrixCSC(
(nc + ns) * N,
(N + 1) * ns + nu * N,
J_colptr,
J_rowval,
J_nzval,
)
SparseArrays.dropzeros!(H)
SparseArrays.dropzeros!(J)
c0 = zero(T)
nvar = ns * (N + 1) + nu * N
c = _init_similar(s0, nvar, T)
lvar = _init_similar(s0, nvar, T)
uvar = _init_similar(s0, nvar, T)
lvar[1:ns] = s0
uvar[1:ns] = s0
lcon = _init_similar(s0, ns * N + N * nc, T)
ucon = _init_similar(s0, ns * N + N * nc, T)
ncon = size(J, 1)
nnzj = length(J.rowval)
nnzh = length(H.rowval)
lcon[1:(ns * N)] .= -w
ucon[1:(ns * N)] .= -w
for i = 1:N
lvar[(i * ns + 1):((i + 1) * ns)] = sl
uvar[(i * ns + 1):((i + 1) * ns)] = su
lcon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gl
ucon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gu
end
for j = 1:N
lvar[((N + 1) * ns + (j - 1) * nu + 1):((N + 1) * ns + j * nu)] = ul
uvar[((N + 1) * ns + (j - 1) * nu + 1):((N + 1) * ns + j * nu)] = uu
end
SparseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
)
end
function _build_sparse_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, MK <: AbstractMatrix}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
bool_vec = (ul .!= -Inf .|| uu .!= Inf)
num_real_bounds = sum(bool_vec)
# Transform u variables to v variables
new_Q = _init_similar(Q, size(Q, 1), size(Q, 2), T)
new_S = _init_similar(S, size(S, 1), size(S, 2), T)
new_A = _init_similar(A, size(A, 1), size(A, 2), T)
new_E = _init_similar(E, size(E, 1), size(E, 2), T)
KTR = _init_similar(Q, size(K, 2), size(R, 2), T)
SK = _init_similar(Q, size(S, 1), size(K, 2), T)
KTRK = _init_similar(Q, size(K, 2), size(K, 2), T)
BK = _init_similar(Q, size(B, 1), size(K, 2), T)
FK = _init_similar(Q, size(F, 1), size(K, 2), T)
H_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
H_rowval = zeros(Int, (ns + nu) * N * ns + (ns + nu) * N * nu + ns * ns)
H_nzval = zeros(T, (ns + nu) * N * ns + (ns + nu) * N * nu + ns * ns)
J_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
J_rowval = zeros(
Int,
N * (ns^2 + ns * nu + ns) +
N * (nc * ns + nc * nu) +
N * (ns * num_real_bounds + num_real_bounds),
)
J_nzval = zeros(
T,
N * (ns^2 + ns * nu + ns) +
N * (nc * ns + nc * nu) +
N * (ns * num_real_bounds + num_real_bounds),
)
LinearAlgebra.copyto!(new_Q, Q)
LinearAlgebra.copyto!(new_S, S)
LinearAlgebra.copyto!(new_A, A)
LinearAlgebra.copyto!(new_E, E)
LinearAlgebra.mul!(KTR, K', R)
LinearAlgebra.axpy!(1, KTR, new_S)
LinearAlgebra.mul!(SK, S, K)
LinearAlgebra.mul!(KTRK, KTR, K)
LinearAlgebra.axpy!(1, SK, new_Q)
LinearAlgebra.axpy!(1, SK', new_Q)
LinearAlgebra.axpy!(1, KTRK, new_Q)
LinearAlgebra.mul!(BK, B, K)
LinearAlgebra.axpy!(1, BK, new_A)
LinearAlgebra.mul!(FK, F, K)
LinearAlgebra.axpy!(1, FK, new_E)
# Get H and J matrices from new matrices
_set_sparse_H!(H_colptr, H_rowval, H_nzval, new_Q, R, N; Qf = Qf, S = new_S)
H = SparseArrays.SparseMatrixCSC(
(N + 1) * ns + nu * N,
(N + 1) * ns + nu * N,
H_colptr,
H_rowval,
H_nzval,
)
_set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
new_A,
B,
new_E,
F,
K,
bool_vec,
N,
num_real_bounds,
)
J = SparseArrays.SparseMatrixCSC(
ns * N + nc * N + num_real_bounds * N,
(N + 1) * ns + nu * N,
J_colptr,
J_rowval,
J_nzval,
)
SparseArrays.dropzeros!(H)
SparseArrays.dropzeros!(J)
# Remove algebraic constraints if u variable is unbounded on both upper and lower ends
lcon3 = _init_similar(ul, nu * N, T)
ucon3 = _init_similar(ul, nu * N, T)
ul = ul[bool_vec]
uu = uu[bool_vec]
lcon3 = repeat(ul, N)
ucon3 = repeat(uu, N)
nvar = ns * (N + 1) + nu * N
lvar = similar(s0, nvar)
fill!(lvar, -Inf)
uvar = similar(s0, nvar)
fill!(uvar, Inf)
lvar[1:ns] = s0
uvar[1:ns] = s0
lcon = _init_similar(s0, ns * N + N * length(gl) + length(lcon3))
ucon = _init_similar(s0, ns * N + N * length(gl) + length(lcon3))
lcon[1:(ns * N)] .= -w
ucon[1:(ns * N)] .= -w
ncon = size(J, 1)
nnzj = length(J.rowval)
nnzh = length(H.rowval)
for i = 1:N
lvar[(i * ns + 1):((i + 1) * ns)] = sl
uvar[(i * ns + 1):((i + 1) * ns)] = su
lcon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gl
ucon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gu
end
if length(lcon3) > 0
lcon[(1 + ns * N + N * nc):(ns * N + nc * N + num_real_bounds * N)] = lcon3
ucon[(1 + ns * N + N * nc):(ns * N + nc * N + num_real_bounds * N)] = ucon3
end
c0 = zero(T)
c = _init_similar(s0, nvar, T)
SparseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
)
end
function _build_sparse_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: SparseMatrixCSC{T}, MK <: Nothing}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
SparseArrays.dropzeros!(A)
SparseArrays.dropzeros!(B)
SparseArrays.dropzeros!(Q)
SparseArrays.dropzeros!(R)
SparseArrays.dropzeros!(Qf)
SparseArrays.dropzeros!(E)
SparseArrays.dropzeros!(F)
SparseArrays.dropzeros!(S)
H_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
H_rowval = zeros(
Int,
length(Q.rowval) * N +
length(R.rowval) * N +
2 * length(S.rowval) * N +
length(Qf.rowval),
)
H_nzval = zeros(
T,
length(Q.nzval) * N +
length(R.nzval) * N +
2 * length(S.nzval) * N +
length(Qf.nzval),
)
J_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
J_rowval = zeros(
Int,
length(A.rowval) * N +
length(B.rowval) * N +
length(E.rowval) * N +
length(F.rowval) * N +
ns * N,
)
J_nzval = zeros(
T,
length(A.nzval) * N +
length(B.nzval) * N +
length(E.nzval) * N +
length(F.nzval) * N +
ns * N,
)
_set_sparse_H!(H_colptr, H_rowval, H_nzval, Q, R, N; Qf = Qf, S = S)
H = SparseArrays.SparseMatrixCSC(
(N + 1) * ns + nu * N,
(N + 1) * ns + nu * N,
H_colptr,
H_rowval,
H_nzval,
)
_set_sparse_J!(J_colptr, J_rowval, J_nzval, A, B, E, F, K, N)
J = SparseArrays.SparseMatrixCSC(
(nc + ns) * N,
(N + 1) * ns + nu * N,
J_colptr,
J_rowval,
J_nzval,
)
c0 = zero(T)
nvar = ns * (N + 1) + nu * N
c = _init_similar(s0, nvar, T)
lvar = _init_similar(s0, nvar, T)
uvar = _init_similar(s0, nvar, T)
lvar[1:ns] = s0
uvar[1:ns] = s0
lcon = _init_similar(s0, ns * N + N * nc, T)
ucon = _init_similar(s0, ns * N + N * nc, T)
ncon = size(J, 1)
nnzj = length(J.rowval)
nnzh = length(H.rowval)
lcon[1:(ns * N)] .= -w
ucon[1:(ns * N)] .= -w
for i = 1:N
lvar[(i * ns + 1):((i + 1) * ns)] = sl
uvar[(i * ns + 1):((i + 1) * ns)] = su
lcon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gl
ucon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gu
end
for j = 1:N
lvar[((N + 1) * ns + (j - 1) * nu + 1):((N + 1) * ns + j * nu)] = ul
uvar[((N + 1) * ns + (j - 1) * nu + 1):((N + 1) * ns + j * nu)] = uu
end
SparseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
)
end
function _build_sparse_lq_dynamic_model(
dnlp::LQDynamicData{T, V, M, MK},
) where {T, V <: AbstractVector{T}, M <: SparseMatrixCSC{T}, MK <: SparseMatrixCSC{T}}
s0 = dnlp.s0
A = dnlp.A
B = dnlp.B
Q = dnlp.Q
R = dnlp.R
N = dnlp.N
Qf = dnlp.Qf
S = dnlp.S
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.F
K = dnlp.K
w = dnlp.w
sl = dnlp.sl
su = dnlp.su
ul = dnlp.ul
uu = dnlp.uu
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
SparseArrays.dropzeros!(A)
SparseArrays.dropzeros!(B)
SparseArrays.dropzeros!(Q)
SparseArrays.dropzeros!(R)
SparseArrays.dropzeros!(Qf)
SparseArrays.dropzeros!(E)
SparseArrays.dropzeros!(F)
SparseArrays.dropzeros!(S)
SparseArrays.dropzeros!(K)
bool_vec = (ul .!= -Inf .|| uu .!= Inf)
num_real_bounds = sum(bool_vec)
# Transform u variables to v variables
new_Q = _init_similar(Q, size(Q, 1), size(Q, 2), T)
new_S = _init_similar(S, size(S, 1), size(S, 2), T)
new_A = _init_similar(A, size(A, 1), size(A, 2), T)
new_E = _init_similar(E, size(E, 1), size(E, 2), T)
KTR = _init_similar(Q, size(K, 2), size(R, 2), T)
SK = _init_similar(Q, size(S, 1), size(K, 2), T)
KTRK = _init_similar(Q, size(K, 2), size(K, 2), T)
BK = _init_similar(Q, size(B, 1), size(K, 2), T)
FK = _init_similar(Q, size(F, 1), size(K, 2), T)
H_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
H_rowval = zeros(
Int,
length(Q.rowval) * N +
length(R.rowval) * N +
2 * length(S.rowval) * N +
length(Qf.rowval),
)
H_nzval = zeros(
T,
length(Q.nzval) * N +
length(R.nzval) * N +
2 * length(S.nzval) * N +
length(Qf.nzval),
)
LinearAlgebra.copyto!(new_Q, Q)
LinearAlgebra.copyto!(new_S, S)
LinearAlgebra.copyto!(new_A, A)
LinearAlgebra.copyto!(new_E, E)
LinearAlgebra.mul!(KTR, K', R)
LinearAlgebra.axpy!(1, KTR, new_S)
LinearAlgebra.mul!(SK, S, K)
LinearAlgebra.mul!(KTRK, KTR, K)
LinearAlgebra.axpy!(1, SK, new_Q)
LinearAlgebra.axpy!(1, SK', new_Q)
LinearAlgebra.axpy!(1, KTRK, new_Q)
LinearAlgebra.mul!(BK, B, K)
LinearAlgebra.axpy!(1, BK, new_A)
LinearAlgebra.mul!(FK, F, K)
LinearAlgebra.axpy!(1, FK, new_E)
SparseArrays.dropzeros!(new_Q)
SparseArrays.dropzeros!(new_A)
SparseArrays.dropzeros!(new_E)
SparseArrays.dropzeros!(new_S)
K_sparse = K[bool_vec, :]
H_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
H_rowval = zeros(
Int,
length(Q.rowval) * N +
length(R.rowval) * N +
2 * length(new_S.rowval) * N +
length(Qf.rowval),
)
H_nzval = zeros(
T,
length(Q.nzval) * N +
length(R.nzval) * N +
2 * length(new_S.nzval) * N +
length(Qf.nzval),
)
J_colptr = zeros(Int, ns * (N + 1) + nu * N + 1)
J_rowval = zeros(
Int,
length(new_A.rowval) * N +
length(B.rowval) * N +
length(new_E.rowval) * N +
length(F.rowval) * N +
ns * N +
length(K_sparse.rowval) * N +
num_real_bounds * N,
)
J_nzval = zeros(
T,
length(new_A.nzval) * N +
length(B.nzval) * N +
length(new_E.nzval) * N +
length(F.nzval) * N +
ns * N +
length(K_sparse.nzval) * N +
num_real_bounds * N,
)
# Get H and J matrices from new matrices
_set_sparse_H!(H_colptr, H_rowval, H_nzval, new_Q, R, N; Qf = Qf, S = new_S)
H = SparseArrays.SparseMatrixCSC(
(N + 1) * ns + nu * N,
(N + 1) * ns + nu * N,
H_colptr,
H_rowval,
H_nzval,
)
_set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
new_A,
B,
new_E,
F,
K,
bool_vec,
N,
num_real_bounds,
)
J = SparseArrays.SparseMatrixCSC(
ns * N + nc * N + num_real_bounds * N,
(N + 1) * ns + nu * N,
J_colptr,
J_rowval,
J_nzval,
)
# Remove algebraic constraints if u variable is unbounded on both upper and lower ends
lcon3 = _init_similar(ul, nu * N, T)
ucon3 = _init_similar(ul, nu * N, T)
ul = ul[bool_vec]
uu = uu[bool_vec]
lcon3 = repeat(ul, N)
ucon3 = repeat(uu, N)
nvar = ns * (N + 1) + nu * N
lvar = similar(s0, nvar)
fill!(lvar, -Inf)
uvar = similar(s0, nvar)
fill!(uvar, Inf)
lvar[1:ns] = s0
uvar[1:ns] = s0
lcon = _init_similar(s0, ns * N + N * length(gl) + length(lcon3))
ucon = _init_similar(s0, ns * N + N * length(gl) + length(lcon3))
ncon = size(J, 1)
nnzj = length(J.rowval)
nnzh = length(H.rowval)
lcon[1:(ns * N)] .= -w
ucon[1:(ns * N)] .= -w
for i = 1:N
lvar[(i * ns + 1):((i + 1) * ns)] = sl
uvar[(i * ns + 1):((i + 1) * ns)] = su
lcon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gl
ucon[(ns * N + 1 + (i - 1) * nc):(ns * N + i * nc)] = gu
end
if length(lcon3) > 0
lcon[(1 + ns * N + N * nc):(ns * N + nc * N + num_real_bounds * N)] = lcon3
ucon[(1 + ns * N + N * nc):(ns * N + nc * N + num_real_bounds * N)] = ucon3
end
c0 = zero(T)
c = _init_similar(s0, nvar, T)
SparseLQDynamicModel(
NLPModels.NLPModelMeta(
nvar,
x0 = _init_similar(s0, nvar, T),
lvar = lvar,
uvar = uvar,
ncon = ncon,
lcon = lcon,
ucon = ucon,
nnzj = nnzj,
nnzh = nnzh,
lin = 1:ncon,
islp = (ncon == 0);
),
NLPModels.Counters(),
QuadraticModels.QPData(c0, c, H, J),
dnlp,
)
end
#set the data needed to build a SparseArrays.SparseMatrixCSC matrix. H_colptr, H_rowval, and H_nzval
#are set so that they can be passed to SparseMatrixCSC() to obtain the `H` matrix such that
# z^T H z = sum_{i=1}^{N-1} s_i^T Q s + sum_{i=1}^{N-1} u^T R u + s_N^T Qf s_n .
function _set_sparse_H!(
H_colptr,
H_rowval,
H_nzval,
Q::M,
R::M,
N;
Qf::M = Q,
S::M = zeros(T, size(Q, 1), size(R, 1)),
) where {T, M <: AbstractMatrix{T}}
ns = size(Q, 1)
nu = size(R, 1)
for i = 1:N
for j = 1:ns
H_nzval[(1 + (i - 1) * (ns^2 + nu * ns) + (j - 1) * (ns + nu)):(ns * j + nu * (j - 1) + (i - 1) * (ns^2 + nu * ns))] =
@view Q[:, j]
H_nzval[(1 + (i - 1) * (ns^2 + nu * ns) + j * ns + (j - 1) * nu):((i - 1) * (ns^2 + nu * ns) + j * (ns + nu))] =
@view S[j, :]
H_rowval[(1 + (i - 1) * (ns^2 + nu * ns) + (j - 1) * ns + (j - 1) * nu):(ns * j + nu * (j - 1) + (i - 1) * (ns^2 + nu * ns))] =
(1 + (i - 1) * ns):(ns * i)
H_rowval[(1 + (i - 1) * (ns^2 + nu * ns) + j * ns + (j - 1) * nu):((i - 1) * (ns^2 + nu * ns) + j * (ns + nu))] =
(1 + (N + 1) * ns + nu * (i - 1)):((N + 1) * ns + nu * i)
H_colptr[((i - 1) * ns + j)] =
1 + (ns + nu) * (j - 1) + (i - 1) * (ns * nu + ns * ns)
end
end
for j = 1:ns
H_nzval[(1 + N * (ns^2 + nu * ns) + (j - 1) * ns):(ns * j + N * (ns^2 + nu * ns))] =
@view Qf[:, j]
H_rowval[(1 + N * (ns^2 + nu * ns) + (j - 1) * ns):(ns * j + N * (ns^2 + nu * ns))] =
(1 + N * ns):((N + 1) * ns)
H_colptr[(N * ns + j)] = 1 + ns * (j - 1) + N * (ns * nu + ns * ns)
end
offset = ns^2 * (N + 1) + ns * nu * N
for i = 1:N
for j = 1:nu
H_nzval[(1 + offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * (nu + ns)):(offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * nu + j * ns)] =
@view S[:, j]
H_nzval[(1 + offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * nu + j * ns):(offset + (i - 1) * (nu^2 + ns * nu) + j * (ns + nu))] =
@view R[:, j]
H_rowval[(1 + offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * (nu + ns)):(offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * nu + j * ns)] =
(1 + (i - 1) * ns):(i * ns)
H_rowval[(1 + offset + (i - 1) * (nu^2 + ns * nu) + (j - 1) * nu + j * ns):(offset + (i - 1) * (nu^2 + ns * nu) + j * (ns + nu))] =
(1 + (N + 1) * ns + (i - 1) * nu):((N + 1) * ns + i * nu)
H_colptr[(N + 1) * ns + (i - 1) * nu + j] =
1 + offset + (ns + nu) * (j - 1) + (nu^2 + ns * nu) * (i - 1)
end
end
H_colptr[ns * (N + 1) + nu * N + 1] = length(H_nzval) + 1
end
function _set_sparse_H!(
H_colptr,
H_rowval,
H_nzval,
Q::M,
R::M,
N;
Qf::M = Q,
S::M = spzeros(T, size(Q, 1), size(R, 1)),
) where {T, M <: SparseMatrixCSC{T}}
ST = SparseArrays.sparse(S')
ns = size(Q, 1)
nu = size(R, 1)
H_colptr[1] = 1
for i = 1:N
for j = 1:ns
Q_offset = length(Q.colptr[j]:(Q.colptr[j + 1] - 1))
H_nzval[(H_colptr[ns * (i - 1) + j]):(H_colptr[ns * (i - 1) + j] + Q_offset - 1)] =
Q.nzval[Q.colptr[j]:(Q.colptr[j + 1] - 1)]
H_rowval[(H_colptr[ns * (i - 1) + j]):(H_colptr[ns * (i - 1) + j] + Q_offset - 1)] =
Q.rowval[Q.colptr[j]:(Q.colptr[j + 1] - 1)] .+ ns * (i - 1)
ST_offset = length(ST.colptr[j]:(ST.colptr[j + 1] - 1))
H_nzval[(H_colptr[ns * (i - 1) + j] + Q_offset):(H_colptr[ns * (i - 1) + j] + Q_offset + ST_offset - 1)] =
ST.nzval[ST.colptr[j]:(ST.colptr[j + 1] - 1)]
H_rowval[(H_colptr[ns * (i - 1) + j] + Q_offset):(H_colptr[ns * (i - 1) + j] + Q_offset + ST_offset - 1)] =
ST.rowval[ST.colptr[j]:(ST.colptr[j + 1] - 1)] .+
(nu * (i - 1) + ns * (N + 1))
H_colptr[ns * (i - 1) + j + 1] =
H_colptr[ns * (i - 1) + j] + Q_offset + ST_offset
end
end
for j = 1:ns
Qf_offset = length(Qf.colptr[j]:(Qf.colptr[j + 1] - 1))
H_nzval[(H_colptr[N * ns + j]):(H_colptr[N * ns + j] + Qf_offset - 1)] =
Qf.nzval[Qf.colptr[j]:(Qf.colptr[j + 1] - 1)]
H_rowval[(H_colptr[N * ns + j]):(H_colptr[N * ns + j] + Qf_offset - 1)] =
Qf.rowval[Qf.colptr[j]:(Qf.colptr[j + 1] - 1)] .+ (ns * N)
H_colptr[ns * N + j + 1] = H_colptr[ns * N + j] + Qf_offset
end
for i = 1:N
for j = 1:nu
S_offset = length(S.colptr[j]:(S.colptr[j + 1] - 1))
H_nzval[(H_colptr[ns * (N + 1) + nu * (i - 1) + j]):(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset - 1)] =
S.nzval[S.colptr[j]:(S.colptr[j + 1] - 1)]
H_rowval[(H_colptr[ns * (N + 1) + nu * (i - 1) + j]):(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset - 1)] =
S.rowval[S.colptr[j]:(S.colptr[j + 1] - 1)] .+ ((i - 1) * ns)
R_offset = length(R.colptr[j]:(R.colptr[j + 1] - 1))
H_nzval[(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset):(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset + R_offset - 1)] =
R.nzval[R.colptr[j]:(R.colptr[j + 1] - 1)]
H_rowval[(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset):(H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset + R_offset - 1)] =
R.rowval[R.colptr[j]:(R.colptr[j + 1] - 1)] .+ ((i - 1) * nu + ns * (N + 1))
H_colptr[ns * (N + 1) + nu * (i - 1) + j + 1] =
H_colptr[ns * (N + 1) + nu * (i - 1) + j] + S_offset + R_offset
end
end
end
# set the data needed to build a SparseArrays.SparseMatrixCSC matrix. J_colptr, J_rowval, and J_nzval
# are set so that they can be passed to SparseMatrixCSC() to obtain the Jacobian, `J`. The Jacobian
# contains the data for the following constraints:
# As_i + Bu_i = s_{i + 1}
# gl <= Es_i + Fu_i <= get_u
# If `K` is defined, then this matrix also contains the constraints
# ul <= Kx_i + v_i <= uu
function _set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
A,
B,
E,
F,
K::MK,
bool_vec,
N,
nb,
) where {T, MK <: AbstractMatrix{T}}
# nb = num_real_bounds
ns = size(A, 2)
nu = size(B, 2)
nc = size(E, 1)
I_mat = _init_similar(A, nu, nu)
I_mat[LinearAlgebra.diagind(I_mat)] .= T(1)
# Set the first block column of A, E, and K
for j = 1:ns
J_nzval[(1 + (j - 1) * (ns + nc + nb)):((j - 1) * (nc + nb) + j * ns)] =
@view A[:, j]
J_nzval[(1 + (j - 1) * (nc + nb) + j * ns):(j * (ns + nc) + (j - 1) * nb)] =
@view E[:, j]
J_nzval[(1 + j * (ns + nc) + (j - 1) * nb):(j * (ns + nc + nb))] =
@view K[:, j][bool_vec]
J_rowval[(1 + (j - 1) * (ns + nc + nb)):((j - 1) * (nc + nb) + j * ns)] = 1:ns
J_rowval[(1 + (j - 1) * (nc + nb) + j * ns):(j * (ns + nc) + (j - 1) * nb)] =
(1 + ns * N):(nc + ns * N)
J_rowval[(1 + j * (ns + nc) + (j - 1) * nb):(j * (ns + nc + nb))] =
(1 + (ns + nc) * N):((ns + nc) * N + nb)
J_colptr[j] = 1 + (j - 1) * (ns + nc + nb)
end
# Set the remaining block columns corresponding to states: -I, A, E, K
for i = 2:N
offset = (i - 1) * ns * (ns + nc + nb) + (i - 2) * ns
for j = 1:ns
J_nzval[1 + offset + (j - 1) * (ns + nc + nb + 1)] = T(-1)
J_nzval[(1 + offset + (j - 1) * (ns + nc + nb) + j):(offset + j * ns + (j - 1) * (nc + nb) + j)] =
@view A[:, j]
J_nzval[(1 + offset + j * ns + (j - 1) * (nc + nb) + j):(offset + j * (ns + nc) + (j - 1) * nb + j)] =
@view E[:, j]
J_nzval[(1 + offset + j * (ns + nc) + (j - 1) * nb + j):(offset + j * (ns + nc + nb) + j)] =
@view K[:, j][bool_vec]
J_rowval[1 + offset + (j - 1) * (ns + nc + nb + 1)] = ns * (i - 2) + j
J_rowval[(1 + offset + (j - 1) * (ns + nc + nb) + j):(offset + j * ns + (j - 1) * (nc + nb) + j)] =
(1 + (i - 1) * ns):(i * ns)
J_rowval[(1 + offset + j * ns + (j - 1) * (nc + nb) + j):(offset + j * (ns + nc) + (j - 1) * nb + j)] =
(1 + N * ns + (i - 1) * nc):(N * ns + i * nc)
J_rowval[(1 + offset + j * (ns + nc) + (j - 1) * nb + j):(offset + j * (ns + nc + nb) + j)] =
(1 + N * (ns + nc) + (i - 1) * nb):(N * (ns + nc) + i * nb)
J_colptr[(i - 1) * ns + j] = 1 + (j - 1) * (ns + nc + nb + 1) + offset
end
end
# Set the column corresponding to states at N + 1, which are a single block of -I
for j = 1:ns
J_nzval[j + ns * (ns + nc + nb + 1) * N - ns] = T(-1)
J_rowval[j + ns * (ns + nc + nb + 1) * N - ns] = j + (N - 1) * ns
J_colptr[ns * N + j] = 1 + ns * (ns + nc + nb + 1) * N - ns + (j - 1)
end
# Set the remaining block columns corresponding to inputs: B, F, I
nscol_offset = N * (ns^2 + nc * ns + nb * ns + ns)
for i = 1:N
offset = (i - 1) * (nu * ns + nu * nc + nb) + nscol_offset
bool_offset = 0
for j = 1:nu
J_nzval[(1 + offset + (j - 1) * (ns + nc) + bool_offset):(offset + j * ns + (j - 1) * nc + bool_offset)] =
@view B[:, j]
J_nzval[(1 + offset + j * ns + (j - 1) * nc + bool_offset):(offset + j * (ns + nc) + bool_offset)] =
@view F[:, j]
if bool_vec[j]
J_nzval[1 + offset + j * (ns + nc) + bool_offset] = T(1)
J_rowval[1 + offset + j * (ns + nc) + bool_offset] =
(N * (ns + nc) + (i - 1) * nb + 1 + (bool_offset))
end
J_rowval[(1 + offset + (j - 1) * (ns + nc) + bool_offset):(offset + j * ns + (j - 1) * nc + bool_offset)] =
(1 + (i - 1) * ns):(i * ns)
J_rowval[(1 + offset + j * ns + (j - 1) * nc + bool_offset):(offset + j * (ns + nc) + bool_offset)] =
(1 + N * ns + (i - 1) * nc):(N * ns + i * nc)
J_colptr[(ns * (N + 1) + (i - 1) * nu + j)] =
1 + offset + (j - 1) * (ns + nc) + bool_offset
bool_offset += bool_vec[j]
end
end
J_colptr[ns * (N + 1) + nu * N + 1] = length(J_nzval) + 1
end
function _set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
A::M,
B::M,
E,
F,
K::MK,
N,
) where {T, M <: AbstractMatrix{T}, MK <: Nothing}
# nb = num_real_bounds
ns = size(A, 2)
nu = size(B, 2)
nc = size(E, 1)
# Set the first block column of A, E, and K
for j = 1:ns
J_nzval[(1 + (j - 1) * (ns + nc)):((j - 1) * nc + j * ns)] = @view A[:, j]
J_nzval[(1 + (j - 1) * nc + j * ns):(j * (ns + nc))] = @view E[:, j]
J_rowval[(1 + (j - 1) * (ns + nc)):((j - 1) * nc + j * ns)] = 1:ns
J_rowval[(1 + (j - 1) * nc + j * ns):(j * (ns + nc))] = (1 + ns * N):(nc + ns * N)
J_colptr[j] = 1 + (j - 1) * (ns + nc)
end
# Set the remaining block columns corresponding to states: -I, A, E, K
for i = 2:N
offset = (i - 1) * ns * (ns + nc) + (i - 2) * ns
for j = 1:ns
J_nzval[1 + offset + (j - 1) * (ns + nc + 1)] = T(-1)
J_nzval[(1 + offset + (j - 1) * (ns + nc) + j):(offset + j * ns + (j - 1) * nc + j)] =
@view A[:, j]
J_nzval[(1 + offset + j * ns + (j - 1) * nc + j):(offset + j * (ns + nc) + j)] =
@view E[:, j]
J_rowval[1 + offset + (j - 1) * (ns + nc + 1)] = ns * (i - 2) + j
J_rowval[(1 + offset + (j - 1) * (ns + nc) + j):(offset + j * ns + (j - 1) * nc + j)] =
(1 + (i - 1) * ns):(i * ns)
J_rowval[(1 + offset + j * ns + (j - 1) * nc + j):(offset + j * (ns + nc) + j)] =
(1 + N * ns + (i - 1) * nc):(N * ns + i * nc)
J_colptr[(i - 1) * ns + j] = 1 + (j - 1) * (ns + nc + 1) + offset
end
end
# Set the column corresponding to states at N + 1, which are a single block of -I
for j = 1:ns
J_nzval[j + ns * (ns + nc + 1) * N - ns] = T(-1)
J_rowval[j + ns * (ns + nc + 1) * N - ns] = j + (N - 1) * ns
J_colptr[ns * N + j] = 1 + ns * (ns + nc + 1) * N - ns + (j - 1)
end
# Set the remaining block columns corresponding to inputs: B, F, I
nscol_offset = N * (ns^2 + nc * ns + ns)
for i = 1:N
offset = (i - 1) * (nu * ns + nu * nc) + nscol_offset
for j = 1:nu
J_nzval[(1 + offset + (j - 1) * (ns + nc)):(offset + j * ns + (j - 1) * nc)] =
@view B[:, j]
J_nzval[(1 + offset + j * ns + (j - 1) * nc):(offset + j * (ns + nc))] =
@view F[:, j]
J_rowval[(1 + offset + (j - 1) * (ns + nc)):(offset + j * ns + (j - 1) * nc)] =
(1 + (i - 1) * ns):(i * ns)
J_rowval[(1 + offset + j * ns + (j - 1) * nc):(offset + j * (ns + nc))] =
(1 + N * ns + (i - 1) * nc):(N * ns + i * nc)
J_colptr[(ns * (N + 1) + (i - 1) * nu + j)] = 1 + offset + (j - 1) * (ns + nc)
end
end
J_colptr[ns * (N + 1) + nu * N + 1] = length(J_nzval) + 1
end
function _set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
A::M,
B::M,
E::M,
F::M,
K::MK,
bool_vec,
N,
nb,
) where {T, M <: SparseMatrixCSC{T}, MK <: SparseMatrixCSC{T}}
ns = size(A, 2)
nu = size(B, 2)
nc = size(E, 1)
I_mat = _init_similar(K, nu, nu)
I_mat[LinearAlgebra.diagind(I_mat)] .= T(1)
KI = I_mat[bool_vec, :]
K_sparse = K[bool_vec, :]
J_colptr[1] = 1
# Set the first block column of A, E, and K
for j = 1:ns
A_offset = length(A.colptr[j]:(A.colptr[j + 1] - 1))
J_nzval[(J_colptr[j]):(J_colptr[j] + A_offset - 1)] =
A.nzval[A.colptr[j]:(A.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j]):(J_colptr[j] + A_offset - 1)] =
A.rowval[A.colptr[j]:(A.colptr[j + 1] - 1)]
E_offset = length(E.colptr[j]:(E.colptr[j + 1] - 1))
J_nzval[(J_colptr[j] + A_offset):(J_colptr[j] + A_offset + E_offset - 1)] =
E.nzval[E.colptr[j]:(E.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j] + A_offset):(J_colptr[j] + A_offset + E_offset - 1)] =
E.rowval[E.colptr[j]:(E.colptr[j + 1] - 1)] .+ (ns * N)
K_offset = length(K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[j] + A_offset + E_offset):(J_colptr[j] + A_offset + E_offset + K_offset - 1)] =
K_sparse.nzval[K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[j] + A_offset + E_offset):(J_colptr[j] + A_offset + E_offset + K_offset - 1)] =
K_sparse.rowval[K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1)] .+
((ns + nc) * N)
)
J_colptr[j + 1] = J_colptr[j] + A_offset + E_offset + K_offset
end
# Set the remaining block columns corresponding to states: -I, A, E, K
for i = 2:N
for j = 1:ns
J_nzval[J_colptr[j + (i - 1) * ns]] = T(-1)
J_rowval[J_colptr[j + (i - 1) * ns]] = ns * (i - 2) + j
A_offset = length(A.colptr[j]:(A.colptr[j + 1] - 1))
J_nzval[(J_colptr[j + (i - 1) * ns] + 1):(J_colptr[j + (i - 1) * ns] + 1 + A_offset - 1)] =
A.nzval[A.colptr[j]:(A.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j + (i - 1) * ns] + 1):(J_colptr[j + (i - 1) * ns] + 1 + A_offset - 1)] =
A.rowval[A.colptr[j]:(A.colptr[j + 1] - 1)] .+ (ns * (i - 1))
E_offset = length(E.colptr[j]:(E.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset - 1)] =
E.nzval[E.colptr[j]:(E.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset - 1)] =
E.rowval[E.colptr[j]:(E.colptr[j + 1] - 1)] .+ (ns * N + nc * (i - 1))
)
K_offset = length(K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset + K_offset - 1)] =
K_sparse.nzval[K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset + K_offset - 1)] =
K_sparse.rowval[K_sparse.colptr[j]:(K_sparse.colptr[j + 1] - 1)] .+
((ns + nc) * N + nb * (i - 1))
)
J_colptr[ns * (i - 1) + j + 1] =
J_colptr[ns * (i - 1) + j] + 1 + A_offset + E_offset + K_offset
end
end
# Set the column corresponding to states at N + 1, which are a single block of -I
for j = 1:ns
J_nzval[J_colptr[ns * N + j]] = T(-1)
J_rowval[J_colptr[ns * N + j]] = ns * (N - 1) + j
J_colptr[ns * N + j + 1] = J_colptr[ns * N + j] + 1
end
# Set the remaining block columns corresponding to inputs: B, F, I
for i = 1:N
offset = ns * (N + 1) + nu * (i - 1)
for j = 1:nu
B_offset = length(B.colptr[j]:(B.colptr[j + 1] - 1))
J_nzval[(J_colptr[offset + j]):(J_colptr[offset + j] + B_offset - 1)] =
B.nzval[B.colptr[j]:(B.colptr[j + 1] - 1)]
J_rowval[(J_colptr[offset + j]):(J_colptr[offset + j] + B_offset - 1)] =
B.rowval[B.colptr[j]:(B.colptr[j + 1] - 1)] .+ (ns * (i - 1))
F_offset = length(F.colptr[j]:(F.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[offset + j] + B_offset):(J_colptr[offset + j] + B_offset + F_offset - 1)] =
F.nzval[F.colptr[j]:(F.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[offset + j] + B_offset):(J_colptr[offset + j] + B_offset + F_offset - 1)] =
F.rowval[F.colptr[j]:(F.colptr[j + 1] - 1)] .+ (ns * N + nc * (i - 1))
)
KI_offset = length(KI.colptr[j]:(KI.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[offset + j] + B_offset + F_offset):(J_colptr[offset + j] + B_offset + F_offset + KI_offset - 1)] =
KI.nzval[KI.colptr[j]:(KI.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[offset + j] + B_offset + F_offset):(J_colptr[offset + j] + B_offset + F_offset + KI_offset - 1)] =
KI.rowval[KI.colptr[j]:(KI.colptr[j + 1] - 1)] .+
((ns + nc) * N + nb * (i - 1))
)
J_colptr[offset + j + 1] =
J_colptr[offset + j] + B_offset + F_offset + KI_offset
end
end
end
function _set_sparse_J!(
J_colptr,
J_rowval,
J_nzval,
A::M,
B::M,
E::M,
F::M,
K::MK,
N,
) where {T, M <: SparseMatrixCSC{T}, MK <: Nothing}
ns = size(A, 2)
nu = size(B, 2)
nc = size(E, 1)
J_colptr[1] = 1
# Set the first block column of A, E, and K
for j = 1:ns
A_offset = length(A.colptr[j]:(A.colptr[j + 1] - 1))
J_nzval[(J_colptr[j]):(J_colptr[j] + A_offset - 1)] =
A.nzval[A.colptr[j]:(A.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j]):(J_colptr[j] + A_offset - 1)] =
A.rowval[A.colptr[j]:(A.colptr[j + 1] - 1)]
E_offset = length(E.colptr[j]:(E.colptr[j + 1] - 1))
J_nzval[(J_colptr[j] + A_offset):(J_colptr[j] + A_offset + E_offset - 1)] =
E.nzval[E.colptr[j]:(E.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j] + A_offset):(J_colptr[j] + A_offset + E_offset - 1)] =
E.rowval[E.colptr[j]:(E.colptr[j + 1] - 1)] .+ (ns * N)
J_colptr[j + 1] = J_colptr[j] + A_offset + E_offset
end
# Set the remaining block columns corresponding to states: -I, A, E
for i = 2:N
for j = 1:ns
J_nzval[J_colptr[j + (i - 1) * ns]] = T(-1)
J_rowval[J_colptr[j + (i - 1) * ns]] = ns * (i - 2) + j
A_offset = length(A.colptr[j]:(A.colptr[j + 1] - 1))
J_nzval[(J_colptr[j + (i - 1) * ns] + 1):(J_colptr[j + (i - 1) * ns] + 1 + A_offset - 1)] =
A.nzval[A.colptr[j]:(A.colptr[j + 1] - 1)]
J_rowval[(J_colptr[j + (i - 1) * ns] + 1):(J_colptr[j + (i - 1) * ns] + 1 + A_offset - 1)] =
A.rowval[A.colptr[j]:(A.colptr[j + 1] - 1)] .+ (ns * (i - 1))
E_offset = length(E.colptr[j]:(E.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset - 1)] =
E.nzval[E.colptr[j]:(E.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[j + (i - 1) * ns] + 1 + A_offset):(J_colptr[j + (i - 1) * ns] + 1 + A_offset + E_offset - 1)] =
E.rowval[E.colptr[j]:(E.colptr[j + 1] - 1)] .+ (ns * N + nc * (i - 1))
)
J_colptr[ns * (i - 1) + j + 1] =
J_colptr[ns * (i - 1) + j] + 1 + A_offset + E_offset
end
end
# Set the column corresponding to states at N + 1, which are a single block of -I
for j = 1:ns
J_nzval[J_colptr[ns * N + j]] = T(-1)
J_rowval[J_colptr[ns * N + j]] = ns * (N - 1) + j
J_colptr[ns * N + j + 1] = J_colptr[ns * N + j] + 1
end
# Set the remaining block columns corresponding to inputs: B, F
for i = 1:N
offset = ns * (N + 1) + nu * (i - 1)
for j = 1:nu
B_offset = length(B.colptr[j]:(B.colptr[j + 1] - 1))
J_nzval[(J_colptr[offset + j]):(J_colptr[offset + j] + B_offset - 1)] =
B.nzval[B.colptr[j]:(B.colptr[j + 1] - 1)]
J_rowval[(J_colptr[offset + j]):(J_colptr[offset + j] + B_offset - 1)] =
B.rowval[B.colptr[j]:(B.colptr[j + 1] - 1)] .+ (ns * (i - 1))
F_offset = length(F.colptr[j]:(F.colptr[j + 1] - 1))
(
J_nzval[(J_colptr[offset + j] + B_offset):(J_colptr[offset + j] + B_offset + F_offset - 1)] =
F.nzval[F.colptr[j]:(F.colptr[j + 1] - 1)]
)
(
J_rowval[(J_colptr[offset + j] + B_offset):(J_colptr[offset + j] + B_offset + F_offset - 1)] =
F.rowval[F.colptr[j]:(F.colptr[j + 1] - 1)] .+ (ns * N + nc * (i - 1))
)
J_colptr[offset + j + 1] = J_colptr[offset + j] + B_offset + F_offset
end
end
end
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 30656 | """
get_u(solution_ref, lqdm::SparseLQDynamicModel) -> u <: vector
get_u(solution_ref, lqdm::DenseLQDynamicModel) -> u <: vector
Query the solution `u` from the solver. If `K = nothing`, the solution for `u` is queried from `solution_ref.solution`
If `K <: AbstractMatrix`, `solution_ref.solution` returns `v`, and `get_u` solves for `u` using the `K` matrix (and the `A` and `B` matrices if `lqdm <: DenseLQDynamicModel`)
"""
function get_u(
solver_status,
lqdm::SparseLQDynamicModel{T, V, M1, M2, M3, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
MK <: AbstractMatrix{T},
}
solution = solver_status.solution
ns = lqdm.dynamic_data.ns
nu = lqdm.dynamic_data.nu
N = lqdm.dynamic_data.N
K = lqdm.dynamic_data.K
u = zeros(T, nu * N)
for i = 1:N
start_v = (i - 1) * nu + 1
end_v = i * nu
start_s = (i - 1) * ns + 1
end_s = i * ns
Ks = zeros(T, size(K, 1), 1)
s = solution[start_s:end_s]
v = solution[(ns * (N + 1) + start_v):(ns * (N + 1) + end_v)]
LinearAlgebra.mul!(Ks, K, s)
LinearAlgebra.axpy!(1, v, Ks)
u[start_v:end_v] = Ks
end
return u
end
function get_u(
solver_status,
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
M4 <: AbstractMatrix{T},
MK <: AbstractMatrix{T},
}
dnlp = lqdm.dynamic_data
N = dnlp.N
ns = dnlp.ns
nu = dnlp.nu
K = dnlp.K
block_A = lqdm.blocks.A
block_B = lqdm.blocks.B
block_Aw = lqdm.blocks.Aw
v = solver_status.solution
As0 = zeros(T, ns * (N + 1))
Bv = zeros(T, ns)
s = zeros(T, ns * (N + 1))
for i = 1:N
B_row_range = (1 + (i - 1) * ns):(i * ns)
B_sub_block = view(block_B, B_row_range, :)
for j = 1:(N - i + 1)
v_sub_vec = v[(1 + nu * (j - 1)):(nu * j)]
LinearAlgebra.mul!(Bv, B_sub_block, v_sub_vec)
s[(1 + ns * (i + j - 1)):(ns * (i + j))] .+= Bv
end
end
LinearAlgebra.mul!(As0, block_A, dnlp.s0)
LinearAlgebra.axpy!(1, As0, s)
LinearAlgebra.axpy!(1, block_Aw, s)
Ks = _init_similar(dnlp.s0, size(K, 1), T)
u = copy(v)
for i = 1:N
LinearAlgebra.mul!(Ks, K, s[(1 + ns * (i - 1)):(ns * i)])
u[(1 + nu * (i - 1)):(nu * i)] .+= Ks
end
return u
end
function get_u(
solver_status,
lqdm::SparseLQDynamicModel{T, V, M1, M2, M3, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
MK <: Nothing,
}
solution = solver_status.solution
ns = lqdm.dynamic_data.ns
nu = lqdm.dynamic_data.nu
N = lqdm.dynamic_data.N
u = solution[(ns * (N + 1) + 1):end]
return u
end
function get_u(
solver_status,
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
M4 <: AbstractMatrix{T},
MK <: Nothing,
}
return copy(solver_status.solution)
end
"""
get_s(solution_ref, lqdm::SparseLQDynamicModel) -> s <: vector
get_s(solution_ref, lqdm::DenseLQDynamicModel) -> s <: vector
Query the solution `s` from the solver. If `lqdm <: SparseLQDynamicModel`, the solution is queried directly from `solution_ref.solution`
If `lqdm <: DenseLQDynamicModel`, then `solution_ref.solution` returns `u` (if `K = nothing`) or `v` (if `K <: AbstactMatrix`), and `s` is found form
transforming `u` or `v` into `s` using `A`, `B`, and `K` matrices.
"""
function get_s(
solver_status,
lqdm::SparseLQDynamicModel{T, V, M1, M2, M3, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix},
}
solution = solver_status.solution
ns = lqdm.dynamic_data.ns
N = lqdm.dynamic_data.N
s = solution[1:(ns * (N + 1))]
return s
end
function get_s(
solver_status,
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
) where {
T,
V <: AbstractVector{T},
M1 <: AbstractMatrix{T},
M2 <: AbstractMatrix{T},
M3 <: AbstractMatrix{T},
M4 <: AbstractMatrix{T},
MK <: Union{Nothing, AbstractMatrix},
}
dnlp = lqdm.dynamic_data
N = dnlp.N
ns = dnlp.ns
nu = dnlp.nu
block_A = lqdm.blocks.A
block_B = lqdm.blocks.B
block_Aw = lqdm.blocks.Aw
v = solver_status.solution
As0 = zeros(T, ns * (N + 1))
Bv = zeros(T, ns)
s = zeros(T, ns * (N + 1))
for i = 1:N
B_row_range = (1 + (i - 1) * ns):(i * ns)
B_sub_block = view(block_B, B_row_range, :)
for j = 1:(N - i + 1)
v_sub_vec = v[(1 + nu * (j - 1)):(nu * j)]
LinearAlgebra.mul!(Bv, B_sub_block, v_sub_vec)
s[(1 + ns * (i + j - 1)):(ns * (i + j))] .+= Bv
end
end
LinearAlgebra.mul!(As0, block_A, dnlp.s0)
LinearAlgebra.axpy!(1, As0, s)
LinearAlgebra.axpy!(1, block_Aw, s)
return s
end
for field in fieldnames(LQDynamicData)
method = Symbol("get_", field)
@eval begin
@doc """
$($method)(LQDynamicData)
$($method)(SparseLQDynamicModel)
$($method)(DenseLQDynamicModel)
Return the value of $($(QuoteNode(field))) from `LQDynamicData` or `SparseLQDynamicModel.dynamic_data` or `DenseLQDynamicModel.dynamic_data`
"""
$method(dyn_data::LQDynamicData) = getproperty(dyn_data, $(QuoteNode(field)))
end
@eval $method(dyn_model::SparseLQDynamicModel) = $method(dyn_model.dynamic_data)
@eval $method(dyn_model::DenseLQDynamicModel) = $method(dyn_model.dynamic_data)
@eval export $method
end
for field in [:A, :B, :Q, :R, :Qf, :E, :F, :S, :K]
method = Symbol("set_", field, "!")
@eval begin
@doc """
$($method)(LQDynamicData, row, col, val)
$($method)(SparseLQDynamicModel, row, col, val)
$($method)(DenseLQDynamicModel, row, col, val)
Set the value of entry $($(QuoteNode(field)))[row, col] to val for `LQDynamicData`, `SparseLQDynamicModel.dynamic_data`, or `DenseLQDynamicModel.dynamic_data`
"""
$method(dyn_data::LQDynamicData, row, col, val) = (dyn_data.$field[row, col] = val)
end
@eval $method(dyn_model::SparseLQDynamicModel, row, col, val) =
(dyn_model.dynamic_data.$field[row, col] = val)
@eval $method(dyn_model::DenseLQDynamicModel, row, col, val) =
(dyn_model.dynamic_data.$field[row, col] = val)
@eval export $method
end
for field in [:s0, :sl, :su, :ul, :uu, :gl, :gu]
method = Symbol("set_", field, "!")
@eval begin
@doc """
$($method)(LQDynamicData, index, val)
$($method)(SparseLQDynamicModel, index, val)
$($method)(DenseLQDynamicModel, index, val)
Set the value of entry $($(QuoteNode(field)))[index] to val for `LQDynamicData`, `SparseLQDynamicModel.dynamic_data`, or `DenseLQDynamicModel.dynamic_data`
"""
$method(dyn_data::LQDynamicData, index, val) = (dyn_data.$field[index] = val)
end
@eval $method(dyn_model::SparseLQDynamicModel, index, val) =
(dyn_model.dynamic_data.$field[index] = val)
@eval $method(dyn_model::DenseLQDynamicModel, index, val) =
(dyn_model.dynamic_data.$field[index] = val)
@eval export $method
end
function fill_structure!(S::SparseMatrixCSC, rows, cols)
count = 1
@inbounds for col = 1:size(S, 2), k = S.colptr[col]:(S.colptr[col + 1] - 1)
rows[count] = S.rowval[k]
cols[count] = col
count += 1
end
end
function fill_coord!(S::SparseMatrixCSC, vals, obj_weight)
count = 1
@inbounds for col = 1:size(S, 2), k = S.colptr[col]:(S.colptr[col + 1] - 1)
vals[count] = obj_weight * S.nzval[k]
count += 1
end
end
function NLPModels.hess_structure!(
qp::SparseLQDynamicModel{T, V, M1, M2, M3},
rows::AbstractVector{<:Integer},
cols::AbstractVector{<:Integer},
) where {T, V, M1 <: SparseMatrixCSC, M2 <: SparseMatrixCSC, M3 <: AbstractMatrix}
fill_structure!(qp.data.H, rows, cols)
return rows, cols
end
function NLPModels.hess_structure!(
qp::DenseLQDynamicModel{T, V, M1, M2, M3},
rows::AbstractVector{<:Integer},
cols::AbstractVector{<:Integer},
) where {T, V, M1 <: Matrix, M2 <: Matrix, M3 <: Matrix}
count = 1
for j = 1:(qp.meta.nvar)
for i = j:(qp.meta.nvar)
rows[count] = i
cols[count] = j
count += 1
end
end
return rows, cols
end
function NLPModels.hess_coord!(
qp::SparseLQDynamicModel{T, V, M1, M2, M3},
x::AbstractVector{T},
vals::AbstractVector{T};
obj_weight::Real = one(eltype(x)),
) where {T, V, M1 <: SparseMatrixCSC, M2 <: SparseMatrixCSC, M3 <: AbstractMatrix}
NLPModels.increment!(qp, :neval_hess)
fill_coord!(qp.data.H, vals, obj_weight)
return vals
end
function NLPModels.hess_coord!(
qp::DenseLQDynamicModel{T, V, M1, M2, M3},
x::AbstractVector{T},
vals::AbstractVector{T};
obj_weight::Real = one(eltype(x)),
) where {T, V, M1 <: Matrix, M2 <: Matrix, M3 <: Matrix}
NLPModels.increment!(qp, :neval_hess)
count = 1
for j = 1:(qp.meta.nvar)
for i = j:(qp.meta.nvar)
vals[count] = obj_weight * qp.data.H[i, j]
count += 1
end
end
return vals
end
NLPModels.hess_coord!(
qp::SparseLQDynamicModel,
x::AbstractVector,
y::AbstractVector,
vals::AbstractVector;
obj_weight::Real = one(eltype(x)),
) = NLPModels.hess_coord!(qp, x, vals, obj_weight = obj_weight)
NLPModels.hess_coord!(
qp::DenseLQDynamicModel,
x::AbstractVector,
y::AbstractVector,
vals::AbstractVector;
obj_weight::Real = one(eltype(x)),
) = NLPModels.hess_coord!(qp, x, vals, obj_weight = obj_weight)
function NLPModels.jac_structure!(
qp::SparseLQDynamicModel{T, V, M1, M2, M3},
rows::AbstractVector{<:Integer},
cols::AbstractVector{<:Integer},
) where {T, V, M1 <: SparseMatrixCSC, M2 <: SparseMatrixCSC, M3 <: AbstractMatrix}
fill_structure!(qp.data.A, rows, cols)
return rows, cols
end
function NLPModels.jac_structure!(
qp::DenseLQDynamicModel{T, V, M1, M2, M3},
rows::AbstractVector{<:Integer},
cols::AbstractVector{<:Integer},
) where {T, V, M1 <: Matrix, M2 <: Matrix, M3 <: Matrix}
count = 1
for j = 1:(qp.meta.nvar)
for i = 1:(qp.meta.ncon)
rows[count] = i
cols[count] = j
count += 1
end
end
return rows, cols
end
function NLPModels.jac_coord!(
qp::SparseLQDynamicModel{T, V, M1, M2, M3},
x::AbstractVector,
vals::AbstractVector,
) where {T, V, M1 <: SparseMatrixCSC, M2 <: SparseMatrixCSC, M3 <: AbstractMatrix}
NLPModels.increment!(qp, :neval_jac)
fill_coord!(qp.data.A, vals, one(T))
return vals
end
function NLPModels.jac_coord!(
qp::DenseLQDynamicModel{T, V, M1, M2, M3},
x::AbstractVector,
vals::AbstractVector,
) where {T, V, M1 <: Matrix, M2 <: Matrix, M3 <: Matrix}
NLPModels.increment!(qp, :neval_jac)
count = 1
for j = 1:(qp.meta.nvar)
for i = 1:(qp.meta.ncon)
vals[count] = qp.data.A[i, j]
count += 1
end
end
return vals
end
function _dnlp_unsafe_wrap(
tensor::A,
dims::Tuple,
shift = 1,
) where {T, A <: AbstractArray{T}}
return unsafe_wrap(Matrix{T}, pointer(tensor, shift), dims)
end
function _dnlp_unsafe_wrap(
tensor::A,
dims::Tuple,
shift = 1,
) where {T, A <: CUDA.CuArray{T, 3, CUDA.Mem.DeviceBuffer}}
return unsafe_wrap(
CUDA.CuArray{T, 2, CUDA.Mem.DeviceBuffer},
pointer(tensor, shift),
dims,
)
end
function LinearAlgebra.mul!(
y::V,
Jac::LQJacobianOperator{T, M, A},
x::V,
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
fill!(y, zero(T))
J1 = Jac.truncated_jac1
J2 = Jac.truncated_jac2
J3 = Jac.truncated_jac3
N = Jac.N
nu = Jac.nu
nc = Jac.nc
nsc = Jac.nsc
nuc = Jac.nuc
for i = 1:N
sub_B1 = _dnlp_unsafe_wrap(J1, (nc, nu), (1 + (i - 1) * (nc * nu)))
sub_B2 = _dnlp_unsafe_wrap(J2, (nsc, nu), (1 + (i - 1) * (nsc * nu)))
sub_B3 = _dnlp_unsafe_wrap(J3, (nuc, nu), (1 + (i - 1) * (nuc * nu)))
for j = 1:(N - i + 1)
sub_x = view(x, (1 + (j - 1) * nu):(j * nu))
LinearAlgebra.mul!(
view(y, (1 + nc * (j + i - 2)):(nc * (j + i - 1))),
sub_B1,
sub_x,
1,
1,
)
LinearAlgebra.mul!(
view(y, (1 + nc * N + nsc * (j + i - 2)):(nc * N + nsc * (j + i - 1))),
sub_B2,
sub_x,
1,
1,
)
LinearAlgebra.mul!(
view(
y,
(1 + nc * N + nsc * N + nuc * (j + i - 2)):(nc * N + nsc * N + nuc * (j + i - 1)),
),
sub_B3,
sub_x,
1,
1,
)
end
end
end
function LinearAlgebra.mul!(
x::V,
Jac::LQJacobianOperator{T, M, A},
y::V,
) where {
T,
V <: CUDA.CuArray{T, 1, CUDA.Mem.DeviceBuffer},
M <: AbstractMatrix{T},
A <: AbstractArray{T},
}
J1 = Jac.truncated_jac1
J2 = Jac.truncated_jac2
J3 = Jac.truncated_jac3
N = Jac.N
nu = Jac.nu
nc = Jac.nc
nsc = Jac.nsc
nuc = Jac.nuc
x1 = Jac.x1
x2 = Jac.x2
x3 = Jac.x3
y1 = Jac.y
fill!(x1, zero(T))
fill!(x2, zero(T))
fill!(x3, zero(T))
for i = 1:N
y1 .= y[(1 + (i - 1) * nu):(i * nu)]
x1_view = view(x1, :, :, i:N)
x2_view = view(x2, :, :, i:N)
x3_view = view(x3, :, :, i:N)
J1_view = view(J1, :, :, 1:(N - i + 1))
J2_view = view(J2, :, :, 1:(N - i + 1))
J3_view = view(J3, :, :, 1:(N - i + 1))
y1_view = view(y1, :, :, i:N)
CUBLAS.gemm_strided_batched!('N', 'N', 1, J1_view, y1_view, 1, x1_view)
CUBLAS.gemm_strided_batched!('N', 'N', 1, J2_view, y1_view, 1, x2_view)
CUBLAS.gemm_strided_batched!('N', 'N', 1, J3_view, y1_view, 1, x3_view)
end
x[1:(nc * N)] .= reshape(x1, nc * N)
x[(1 + nc * N):((nc + nsc) * N)] .= reshape(x2, nsc * N)
x[(1 + (nc + nsc) * N):((nc + nsc + nuc) * N)] .= reshape(x3, nuc * N)
end
function LinearAlgebra.mul!(
y::V,
Jac::LinearOperators.AdjointLinearOperator{T, LQJacobianOperator{T, M, A}},
x::V,
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
fill!(y, zero(T))
jac_op = get_jacobian(Jac)
J1 = jac_op.truncated_jac1
J2 = jac_op.truncated_jac2
J3 = jac_op.truncated_jac3
N = jac_op.N
nu = jac_op.nu
nc = jac_op.nc
nsc = jac_op.nsc
nuc = jac_op.nuc
for i = 1:N
sub_B1 = _dnlp_unsafe_wrap(J1, (nc, nu), (1 + (i - 1) * (nc * nu)))
sub_B2 = _dnlp_unsafe_wrap(J2, (nsc, nu), (1 + (i - 1) * (nsc * nu)))
sub_B3 = _dnlp_unsafe_wrap(J3, (nuc, nu), (1 + (i - 1) * (nuc * nu)))
for j = 1:(N - i + 1)
x1 = view(x, (1 + (j + i - 2) * nc):((j + i - 1) * nc))
x2 = view(x, (1 + nc * N + (j + i - 2) * nsc):(nc * N + (j + i - 1) * nsc))
x3 = view(
x,
(1 + nc * N + nsc * N + (j + i - 2) * nuc):(nc * N + nsc * N + (j + i - 1) * nuc),
)
LinearAlgebra.mul!(view(y, (1 + nu * (j - 1)):(nu * j)), sub_B1', x1, 1, 1)
LinearAlgebra.mul!(view(y, (1 + nu * (j - 1)):(nu * j)), sub_B2', x2, 1, 1)
LinearAlgebra.mul!(view(y, (1 + nu * (j - 1)):(nu * j)), sub_B3', x3, 1, 1)
end
end
end
function LinearAlgebra.mul!(
y::V,
Jac::LinearOperators.AdjointLinearOperator{T, LQJacobianOperator{T, M, A}},
x::V,
) where {
T,
V <: CUDA.CuArray{T, 1, CUDA.Mem.DeviceBuffer},
M <: AbstractMatrix{T},
A <: AbstractArray{T},
}
fill!(y, zero(T))
jac_op = get_jacobian(Jac)
J1 = jac_op.truncated_jac1
J2 = jac_op.truncated_jac2
J3 = jac_op.truncated_jac3
N = jac_op.N
nu = jac_op.nu
nc = jac_op.nc
nsc = jac_op.nsc
nuc = jac_op.nuc
x1 = jac_op.x1
x2 = jac_op.x2
x3 = jac_op.x3
y1 = jac_op.y
x1 .= reshape(x[1:(nc * N)], (nc, 1, N))
x2 .= reshape(x[(1 + nc * N):((nc + nsc) * N)], (nsc, 1, N))
x3 .= reshape(x[(1 + (nc + nsc) * N):((nc + nsc + nuc) * N)], (nuc, 1, N))
for i = 1:N
fill!(y1, zero(T))
y1_view = view(y1, :, :, 1:(N - i + 1))
x1_view = view(x1, :, :, i:N)
x2_view = view(x2, :, :, i:N)
x3_view = view(x3, :, :, i:N)
J1_view = view(J1, :, :, 1:(N - i + 1))
J2_view = view(J2, :, :, 1:(N - i + 1))
J3_view = view(J3, :, :, 1:(N - i + 1))
CUBLAS.gemm_strided_batched!('T', 'N', 1, J1_view, x1_view, 1, y1_view)
CUBLAS.gemm_strided_batched!('T', 'N', 1, J2_view, x2_view, 1, y1_view)
CUBLAS.gemm_strided_batched!('T', 'N', 1, J3_view, x3_view, 1, y1_view)
view(y, (1 + (i - 1) * nu):(i * nu)) .= sum(y1_view, dims = (2, 3))
end
end
"""
get_jacobian(lqdm::DenseLQDynamicModel) -> LQJacobianOperator
get_jacobian(Jac::AdjointLinearOpeartor{T, LQJacobianOperator}) -> LQJacobianOperator
Gets the `LQJacobianOperator` from `DenseLQDynamicModel` (if the `QPdata` contains a `LQJacobian Operator`)
or returns the `LQJacobian Operator` from the adjoint of the `LQJacobianOperator`
"""
function get_jacobian(
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
) where {T, V, M1, M2, M3, M4, MK}
return lqdm.data.A
end
function get_jacobian(
Jac::LinearOperators.AdjointLinearOperator{T, LQJacobianOperator{T, M, A}},
) where {T, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
return Jac'
end
function Base.length(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
return length(Jac.truncated_jac1) +
length(Jac.truncated_jac2) +
length(Jac.truncated_jac3)
end
function Base.size(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
return (
size(Jac.truncated_jac1, 1) +
size(Jac.truncated_jac2, 1) +
size(Jac.truncated_jac3, 1),
size(Jac.truncated_jac1, 2),
)
end
function Base.eltype(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractMatrix{T}}
return T
end
function Base.isreal(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractMatrix{T}}
return isreal(Jac.truncated_jac1) &&
isreal(Jac.truncated_jac2) &&
isreal(Jac.truncated_jac3)
end
function Base.show(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractMatrix{T}}
show(Jac.truncated_jac1)
end
function Base.display(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractMatrix{T}}
display(Jac.truncated_jac1)
end
"""
LinearOperators.reset!(Jac::LQJacobianOperator{T, V, M})
Resets the values of attributes `SJ1`, `SJ2`, and `SJ3` to zero
"""
function LinearOperators.reset!(
Jac::LQJacobianOperator{T, M, A},
) where {T, M <: AbstractMatrix{T}, A <: AbstractMatrix{T}}
fill!(Jac.SJ1, T(0))
fill!(Jac.SJ2, T(0))
fill!(Jac.SJ3, T(0))
end
function NLPModels.jac_op(
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
x::V,
) where {T, V <: AbstractVector{T}, M1, M2 <: LQJacobianOperator, M3, M4, MK}
return lqdm.data.A
end
"""
add_jtsj!(H::M, Jac::LQJacobianOperator{T, V, M}, Σ::V, alpha::Number = 1, beta::Number = 1)
Generates `Jac' Σ Jac` and adds it to the matrix `H`.
`alpha` and `beta` are scalar multipliers such `beta H + alpha Jac' Σ Jac` is stored in `H`, overwriting the existing value of `H`
"""
function add_jtsj!(
H::M,
Jac::LQJacobianOperator{T, M, A},
Σ::V,
alpha::Number = 1,
beta::Number = 1,
) where {T, V <: AbstractVector{T}, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
J1 = Jac.truncated_jac1
J2 = Jac.truncated_jac2
J3 = Jac.truncated_jac3
N = Jac.N
nu = Jac.nu
nc = Jac.nc
nsc = Jac.nsc
nuc = Jac.nuc
ΣJ1 = Jac.SJ1
ΣJ2 = Jac.SJ2
ΣJ3 = Jac.SJ3
LinearAlgebra.lmul!(beta, H)
for i = 1:N
left_block1 = _dnlp_unsafe_wrap(J1, (nc, nu), (1 + (i - 1) * (nc * nu)))
left_block2 = _dnlp_unsafe_wrap(J2, (nsc, nu), (1 + (i - 1) * (nsc * nu)))
left_block3 = _dnlp_unsafe_wrap(J3, (nuc, nu), (1 + (i - 1) * (nuc * nu)))
for j = 1:(N + 1 - i)
Σ_range1 = (1 + (N - j) * nc):((N - j + 1) * nc)
Σ_range2 = (1 + nc * N + (N - j) * nsc):(nc * N + (N - j + 1) * nsc)
Σ_range3 =
(1 + (nc + nsc) * N + (N - j) * nuc):((nc + nsc) * N + (N - j + 1) * nuc)
ΣJ1 .= left_block1 .* view(Σ, Σ_range1)
ΣJ2 .= left_block2 .* view(Σ, Σ_range2)
ΣJ3 .= left_block3 .* view(Σ, Σ_range3)
for k = 1:(N - j - i + 2)
right_block1 =
_dnlp_unsafe_wrap(J1, (nc, nu), (1 + (k + i - 2) * (nc * nu)))
right_block2 =
_dnlp_unsafe_wrap(J2, (nsc, nu), (1 + (k + i - 2) * (nsc * nu)))
right_block3 =
_dnlp_unsafe_wrap(J3, (nuc, nu), (1 + (k + i - 2) * (nuc * nu)))
row_range = (1 + nu * (N - i - j + 1)):(nu * (N - i - j + 2))
col_range = (1 + nu * (N - i - k - j + 2)):(nu * (N - i - k - j + 3))
LinearAlgebra.mul!(
view(H, row_range, col_range),
ΣJ1',
right_block1,
alpha,
1,
)
LinearAlgebra.mul!(
view(H, row_range, col_range),
ΣJ2',
right_block2,
alpha,
1,
)
LinearAlgebra.mul!(
view(H, row_range, col_range),
ΣJ3',
right_block3,
alpha,
1,
)
end
end
end
end
function add_jtsj!(
H::M,
Jac::LQJacobianOperator{T, M, A},
Σ::V,
alpha::Number = 1,
beta::Number = 1,
) where {T, V <: CUDA.CuVector, M <: AbstractMatrix{T}, A <: AbstractArray{T}}
J1 = Jac.truncated_jac1
J2 = Jac.truncated_jac2
J3 = Jac.truncated_jac3
N = Jac.N
nu = Jac.nu
nc = Jac.nc
nsc = Jac.nsc
nuc = Jac.nuc
ΣJ1 = Jac.SJ1
ΣJ2 = Jac.SJ2
ΣJ3 = Jac.SJ3
H_sub_block = Jac.H_sub_block
LinearAlgebra.lmul!(beta, H)
for i = 1:N
left_block1 = view(J1, :, :, i)
left_block2 = view(J2, :, :, i)
left_block3 = view(J3, :, :, i)
for j = 1:(N + 1 - i)
Σ_range1 = (1 + (N - j) * nc):((N - j + 1) * nc)
Σ_range2 = (1 + nc * N + (N - j) * nsc):(nc * N + (N - j + 1) * nsc)
Σ_range3 =
(1 + (nc + nsc) * N + (N - j) * nuc):((nc + nsc) * N + (N - j + 1) * nuc)
ΣJ1 .= left_block1 .* view(Σ, Σ_range1)
ΣJ2 .= left_block2 .* view(Σ, Σ_range2)
ΣJ3 .= left_block3 .* view(Σ, Σ_range3)
for k = 1:(N - j - i + 2)
right_block1 = view(J1, :, :, (k + i - 1))
right_block2 = view(J2, :, :, (k + i - 1))
right_block3 = view(J3, :, :, (k + i - 1))
row_range = (1 + nu * (N - i - j + 1)):(nu * (N - i - j + 2))
col_range = (1 + nu * (N - i - k - j + 2)):(nu * (N - i - k - j + 3))
LinearAlgebra.mul!(H_sub_block, ΣJ1', right_block1)
H[row_range, col_range] .+= alpha .* H_sub_block
LinearAlgebra.mul!(H_sub_block, ΣJ2', right_block2)
H[row_range, col_range] .+= alpha .* H_sub_block
LinearAlgebra.mul!(H_sub_block, ΣJ3', right_block3)
H[row_range, col_range] .+= alpha .* H_sub_block
end
end
end
end
"""
reset_s0!(lqdm::SparseLQDynamicModel, s0)
reset_s0!(lqdm::DenseLQDynamicModel, s0)
Resets `s0` within `lqdm.dynamic_data`. For a `SparseLQDynamicModel`, this updates the variable bounds which fix the value of `s0`.
For a `DenseLQDynamicModel`, also resets the constraint bounds on the Jacobian and resets the linear and constant terms within the
objective function (i.e., `lqdm.data.c` and `lqdm.data.c0`). This provides a way to update the model after each sample period.
"""
function reset_s0!(
lqdm::SparseLQDynamicModel{T, V, M1, M2, M3, MK},
s0::V,
) where {T, V <: AbstractVector{T}, M1, M2, M3, MK}
dnlp = lqdm.dynamic_data
ns = dnlp.ns
lqdm.dynamic_data.s0 .= s0
lqdm.meta.lvar[1:ns] .= s0
lqdm.meta.uvar[1:ns] .= s0
end
function reset_s0!(
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
s0::V,
) where {T, V <: AbstractVector{T}, M1, M2, M3, M4, MK <: Nothing}
dnlp = lqdm.dynamic_data
dense_blocks = lqdm.blocks
N = dnlp.N
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.E
ul = dnlp.ul
uu = dnlp.uu
sl = dnlp.sl
su = dnlp.su
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
# Get matrices for multiplying by s0
block_A = dense_blocks.A
block_Aw = dense_blocks.Aw
block_h = dense_blocks.h
block_h0 = dense_blocks.h01
block_d = dense_blocks.d
block_dw = dense_blocks.dw
block_h02 = dense_blocks.h02
h_constant = dense_blocks.h_constant
h0_constant = dense_blocks.h0_constant
lcon = lqdm.meta.lcon
ucon = lqdm.meta.ucon
# Reset s0
lqdm.dynamic_data.s0 .= s0
As0 = _init_similar(s0, ns * (N + 1), T)
Qs0 = _init_similar(s0, ns, T)
dl = repeat(gl, N)
du = repeat(gu, N)
bool_vec_s = (sl .!= -Inf .|| su .!= Inf)
nsc = sum(bool_vec_s)
sl = sl[bool_vec_s]
su = su[bool_vec_s]
LinearAlgebra.mul!(dl, block_d, s0, -1, 1)
LinearAlgebra.mul!(du, block_d, s0, -1, 1)
# Reset constraint bounds corresponding to E and F matrices
lcon[1:(nc * N)] .= dl
ucon[1:(nc * N)] .= du
lcon[1:(nc * N)] .-= block_dw
ucon[1:(nc * N)] .-= block_dw
LinearAlgebra.mul!(As0, block_A, s0)
# reset linear term
LinearAlgebra.mul!(lqdm.data.c, block_h, s0)
lqdm.data.c += h_constant
# reset constant term
LinearAlgebra.mul!(Qs0, block_h0, s0)
lqdm.data.c0 = LinearAlgebra.dot(s0, Qs0) / T(2)
lqdm.data.c0 += h0_constant
lqdm.data.c0 += LinearAlgebra.dot(s0, block_h02)
for i = 1:N
# Reset bounds on constraints from state variable bounds
lcon[(1 + nc * N + nsc * (i - 1)):(nc * N + nsc * i)] .=
sl .- As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .-
block_Aw[(1 + ns * i):((i + 1) * ns)][bool_vec_s]
ucon[(1 + nc * N + nsc * (i - 1)):(nc * N + nsc * i)] .=
su .- As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .-
block_Aw[(1 + ns * i):((i + 1) * ns)][bool_vec_s]
end
end
function reset_s0!(
lqdm::DenseLQDynamicModel{T, V, M1, M2, M3, M4, MK},
s0::V,
) where {T, V <: AbstractVector{T}, M1, M2, M3, M4, MK <: AbstractMatrix{T}}
dnlp = lqdm.dynamic_data
dense_blocks = lqdm.blocks
N = dnlp.N
ns = dnlp.ns
nu = dnlp.nu
E = dnlp.E
F = dnlp.E
K = dnlp.K
ul = dnlp.ul
uu = dnlp.uu
sl = dnlp.sl
su = dnlp.su
gl = dnlp.gl
gu = dnlp.gu
nc = size(E, 1)
# Get matrices for multiplying by s0
block_A = dense_blocks.A
block_Aw = dense_blocks.Aw
block_h = dense_blocks.h
block_h0 = dense_blocks.h01
block_d = dense_blocks.d
block_dw = dense_blocks.dw
block_KA = dense_blocks.KA
block_KAw = dense_blocks.KAw
block_h02 = dense_blocks.h02
h_constant = dense_blocks.h_constant
h0_constant = dense_blocks.h0_constant
lcon = lqdm.meta.lcon
ucon = lqdm.meta.ucon
# Reset s0
lqdm.dynamic_data.s0 .= s0
lqdm.data.c0 += LinearAlgebra.dot(s0, block_h02)
As0 = _init_similar(s0, ns * (N + 1), T)
Qs0 = _init_similar(s0, ns, T)
KAs0 = _init_similar(s0, nu * N, T)
dl = repeat(gl, N)
du = repeat(gu, N)
bool_vec_s = (sl .!= -Inf .|| su .!= Inf)
nsc = sum(bool_vec_s)
bool_vec_u = (ul .!= -Inf .|| uu .!= Inf)
nuc = sum(bool_vec_u)
sl = sl[bool_vec_s]
su = su[bool_vec_s]
ul = ul[bool_vec_u]
uu = uu[bool_vec_u]
LinearAlgebra.mul!(dl, block_d, s0, -1, 1)
LinearAlgebra.mul!(du, block_d, s0, -1, 1)
# Reset constraint bounds corresponding to E and F matrices
lcon[1:(nc * N)] .= dl
ucon[1:(nc * N)] .= du
lcon[1:(nc * N)] .-= block_dw
ucon[1:(nc * N)] .-= block_dw
LinearAlgebra.mul!(As0, block_A, s0)
LinearAlgebra.mul!(KAs0, block_KA, s0)
# reset linear term
LinearAlgebra.mul!(lqdm.data.c, block_h, s0)
lqdm.data.c += h_constant
# reset constant term
LinearAlgebra.mul!(Qs0, block_h0, s0)
lqdm.data.c0 = LinearAlgebra.dot(s0, Qs0) / T(2)
lqdm.data.c0 += h0_constant
lqdm.data.c0 += LinearAlgebra.dot(s0, block_h02)
for i = 1:N
# Reset bounds on constraints from state variable bounds
lcon[(1 + nc * N + nsc * (i - 1)):(nc * N + nsc * i)] .=
sl .- As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .-
block_Aw[(1 + i * ns):((i + 1) * ns)][bool_vec_s]
ucon[(1 + nc * N + nsc * (i - 1)):(nc * N + nsc * i)] .=
su .- As0[(1 + ns * i):(ns * (i + 1))][bool_vec_s] .-
block_Aw[(1 + i * ns):((i + 1) * ns)][bool_vec_s]
# Reset bounds on constraints from input variable bounds
lcon[(1 + (nc + nsc) * N + nuc * (i - 1)):((nc + nsc) * N + nuc * i)] .=
ul .- KAs0[(1 + nu * (i - 1)):(nu * i)][bool_vec_u] .-
block_KAw[(1 + nu * (i - 1)):(nu * i)][bool_vec_u]
ucon[(1 + (nc + nsc) * N + nuc * (i - 1)):((nc + nsc) * N + nuc * i)] .=
uu .- KAs0[(1 + nu * (i - 1)):(nu * i)][bool_vec_u] .-
block_KAw[(1 + nu * (i - 1)):(nu * i)][bool_vec_u]
end
end
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 6953 | function test_mul(lq_dense, lq_dense_imp)
dnlp = lq_dense.dynamic_data
N = dnlp.N
nu = dnlp.nu
J = get_jacobian(lq_dense)
J_imp = get_jacobian(lq_dense_imp)
Random.seed!(10)
x = rand(nu * N)
y = rand(size(J, 1))
x_imp = similar(lq_dense_imp.dynamic_data.s0, length(x))
y_imp = similar(lq_dense_imp.dynamic_data.s0, length(y))
LinearAlgebra.copyto!(x_imp, x)
LinearAlgebra.copyto!(y_imp, y)
LinearAlgebra.mul!(y, J, x)
LinearAlgebra.mul!(y_imp, J_imp, x_imp)
@test y ≈ Vector(y_imp) atol = 1e-14
x = rand(nu * N)
y = rand(size(J, 1))
x_imp = similar(lq_dense_imp.dynamic_data.s0, length(x))
y_imp = similar(lq_dense_imp.dynamic_data.s0, length(y))
LinearAlgebra.copyto!(x_imp, x)
LinearAlgebra.copyto!(y_imp, y)
LinearAlgebra.mul!(x, J', y)
LinearAlgebra.mul!(x_imp, J_imp', y_imp)
@test x ≈ Vector(x_imp) atol = 1e-14
end
function test_add_jtsj(lq_dense, lq_dense_imp)
dnlp = lq_dense.dynamic_data
N = dnlp.N
nu = dnlp.nu
H = zeros(nu * N, nu * N)
Random.seed!(10)
J = get_jacobian(lq_dense)
J_imp = get_jacobian(lq_dense_imp)
ΣJ = similar(J); fill!(ΣJ, 0)
x = rand(size(J, 1))
H_imp = similar(lq_dense_imp.data.H, nu * N, nu * N); fill!(H_imp, 0)
x_imp = similar(lq_dense_imp.dynamic_data.s0, length(x));
LinearAlgebra.copyto!(x_imp, x)
LinearAlgebra.mul!(ΣJ, Diagonal(x), J)
LinearAlgebra.mul!(H, J', ΣJ)
add_jtsj!(H_imp, J_imp, x_imp)
@test LowerTriangular(Array(H_imp)) ≈ LowerTriangular(H) atol = 1e-10
end
function dynamic_data_to_CUDA(dnlp::LQDynamicData)
s0c = CuVector{Float64}(undef, length(dnlp.s0))
Ac = CuArray{Float64}(undef, size(dnlp.A))
Bc = CuArray{Float64}(undef, size(dnlp.B))
Qc = CuArray{Float64}(undef, size(dnlp.Q))
Rc = CuArray{Float64}(undef, size(dnlp.R))
Sc = CuArray{Float64}(undef, size(dnlp.S))
Ec = CuArray{Float64}(undef, size(dnlp.E))
Fc = CuArray{Float64}(undef, size(dnlp.F))
wc = CuArray{Float64}(undef, length(dnlp.w))
Qfc = CuArray{Float64}(undef, size(dnlp.Qf))
glc = CuVector{Float64}(undef, length(dnlp.gl))
guc = CuVector{Float64}(undef, length(dnlp.gu))
ulc = CuVector{Float64}(undef, length(dnlp.ul))
uuc = CuVector{Float64}(undef, length(dnlp.uu))
slc = CuVector{Float64}(undef, length(dnlp.sl))
suc = CuVector{Float64}(undef, length(dnlp.su))
LinearAlgebra.copyto!(Ac, dnlp.A)
LinearAlgebra.copyto!(Bc, dnlp.B)
LinearAlgebra.copyto!(Qc, dnlp.Q)
LinearAlgebra.copyto!(Rc, dnlp.R)
LinearAlgebra.copyto!(s0c, dnlp.s0)
LinearAlgebra.copyto!(Sc, dnlp.S)
LinearAlgebra.copyto!(Ec, dnlp.E)
LinearAlgebra.copyto!(Fc, dnlp.F)
LinearAlgebra.copyto!(wc, dnlp.w)
LinearAlgebra.copyto!(Qfc, dnlp.Qf)
LinearAlgebra.copyto!(glc, dnlp.gl)
LinearAlgebra.copyto!(guc, dnlp.gu)
LinearAlgebra.copyto!(ulc, dnlp.ul)
LinearAlgebra.copyto!(uuc, dnlp.uu)
LinearAlgebra.copyto!(slc, dnlp.sl)
LinearAlgebra.copyto!(suc, dnlp.su)
if dnlp.K != nothing
Kc = CuArray{Float64}(undef, size(dnlp.K))
LinearAlgebra.copyto!(Kc, dnlp.K)
else
Kc = nothing
end
LQDynamicData(s0c, Ac, Bc, Qc, Rc, dnlp.N; Qf = Qfc, S = Sc,
E = Ec, F = Fc, K = Kc, sl = slc, su = suc, ul = ulc, uu = uuc, gl = glc, gu = guc, w = wc
)
end
function test_sparse_support(lqdm)
d = lqdm.dynamic_data
(lqdm_sparse_data = SparseLQDynamicModel(d.s0, sparse(d.A), sparse(d.B), sparse(d.Q), sparse(d.R), d.N;
sl = d.sl, ul = d.ul, su = d.su, uu = d.uu, Qf = sparse(d.Qf), K = (d.K == nothing ? nothing : sparse(d.K)),
S = sparse(d.S), E = sparse(d.E), F = sparse(d.F), gl = d.gl, gu = d.gu))
@test lqdm.data.H ≈ lqdm_sparse_data.data.H atol = 1e-10
@test lqdm.data.A ≈ lqdm_sparse_data.data.A atol = 1e-10
end
function test_dense_reset_s0(dnlp, lq_dense, new_s0)
lq_dense_test = DenseLQDynamicModel(dnlp)
dnlp.s0 .= new_s0
lq_dense_new_s0 = DenseLQDynamicModel(dnlp)
reset_s0!(lq_dense_test, new_s0)
@test lq_dense_test.data.H ≈ lq_dense_new_s0.data.H atol = 1e-10
@test lq_dense_test.data.A ≈ lq_dense_new_s0.data.A atol = 1e-10
@test lq_dense_test.data.c ≈ lq_dense_new_s0.data.c atol = 1e-10
@test lq_dense_test.data.c0 ≈ lq_dense_new_s0.data.c0 atol = 1e-10
@test lq_dense_test.meta.lcon ≈ lq_dense_new_s0.meta.lcon atol = 1e-8
@test lq_dense_test.meta.ucon ≈ lq_dense_new_s0.meta.ucon atol = 1e-8
@test lq_dense_test.dynamic_data.s0 == lq_dense_new_s0.dynamic_data.s0
end
function test_sparse_reset_s0(dnlp, lq_sparse, new_s0)
reset_s0!(lq_sparse, new_s0)
@test lq_sparse.dynamic_data.s0 == new_s0
end
function runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
optimize!(model)
solution_ref_sparse = madnlp(lq_sparse, max_iter=100)
solution_ref_dense = madnlp(lq_dense, max_iter=100)
solution_ref_sparse_from_data = madnlp(lq_sparse_from_data, max_iter=100)
solution_ref_dense_from_data = madnlp(lq_dense_from_data, max_iter=100)
@test objective_value(model) ≈ solution_ref_sparse.objective atol = 1e-7
@test objective_value(model) ≈ solution_ref_dense.objective atol = 1e-5
@test objective_value(model) ≈ solution_ref_sparse_from_data.objective atol = 1e-7
@test objective_value(model) ≈ solution_ref_dense_from_data.objective atol = 1e-5
@test solution_ref_sparse.solution[(ns * (N + 1) + 1):(ns * (N + 1) + nu*N)] ≈ solution_ref_dense.solution atol = 1e-5
@test solution_ref_sparse_from_data.solution[(ns * (N + 1) + 1):(ns * (N + 1) + nu*N)] ≈ solution_ref_dense_from_data.solution atol = 1e-5
# Test get_u and get_s functions with no K matrix
s_values = value.(all_variables(model)[1:(ns * (N + 1))])
u_values = value.(all_variables(model)[(1 + ns * (N + 1)):(ns * (N + 1) + nu * N)])
@test s_values ≈ get_s(solution_ref_sparse, lq_sparse) atol = 1e-6
@test u_values ≈ get_u(solution_ref_sparse, lq_sparse) atol = 1e-6
@test s_values ≈ get_s(solution_ref_dense, lq_dense) atol = 2e-5
@test u_values ≈ get_u(solution_ref_dense, lq_dense) atol = 2e-5
test_sparse_support(lq_sparse)
lq_dense_imp = DenseLQDynamicModel(dnlp; implicit = true)
imp_test_set = []
push!(imp_test_set, lq_dense_imp)
if CUDA.has_cuda_gpu()
dnlp_cuda = dynamic_data_to_CUDA(dnlp)
lq_dense_cuda = DenseLQDynamicModel(dnlp_cuda; implicit=true)
push!(imp_test_set, lq_dense_cuda)
end
@testset "Test mul and add_jtsj!" for lq_imp in imp_test_set
test_mul(lq_dense, lq_imp)
test_add_jtsj(lq_dense, lq_imp)
end
new_s0 = copy(dnlp.s0) .+ .5
test_dense_reset_s0(dnlp, lq_dense, new_s0)
new_s0 = copy(dnlp.s0) .+ 1
test_sparse_reset_s0(dnlp, lq_sparse, new_s0)
end
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 13163 | using Test, DynamicNLPModels, MadNLP, Random, JuMP, LinearAlgebra, SparseArrays, CUDA
include("sparse_lq_test.jl")
include("functions.jl")
N = 3 # number of time steps
ns = 2 # number of states
nu = 1 # number of inputs
# generate random Q, R, A, and B matrices
Random.seed!(10)
Q_rand = Random.rand(ns, ns)
Q = Q_rand * Q_rand' + I
R_rand = Random.rand(nu,nu)
R = R_rand * R_rand' + I
A_rand = rand(ns, ns)
A = A_rand * A_rand' + I
B = rand(ns, nu)
# generate upper and lower bounds
sl = rand(ns)
ul = fill(-15.0, nu)
su = sl .+ 4
uu = ul .+ 10
s0 = sl .+ 2
su_with_inf = copy(su)
sl_with_inf = copy(sl)
su_with_inf[1] = Inf
sl_with_inf[1] = -Inf
Qf_rand = Random.rand(ns,ns)
Qf = Qf_rand * Qf_rand' + I
E = rand(3, ns)
F = rand(3, nu)
gl = fill(-5.0, 3)
gu = fill(15.0, 3)
S = rand(ns, nu)
w = rand(0.0:.0001:.25, ns * N)
K = rand(nu, ns)
# Test with no bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test with lower bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test with upper bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, su = su, uu = uu, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; su = su, uu = uu, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; su = su, uu = uu, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; su = su, uu = uu, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test with upper and lower bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl=sl, ul=ul, su = su, uu = uu, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl=sl, ul=ul, su = su, uu = uu, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test with Qf matrix
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, Qf = Qf, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, Qf = Qf, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, Qf = Qf, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, Qf = Qf, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test with E and F matrix bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test edge case where one state is unbounded, other(s) is bounded
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl_with_inf, ul = ul, su = su_with_inf, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl_with_inf, ul = ul, su = su_with_inf, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl_with_inf, ul = ul, su = su_with_inf, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl_with_inf, ul = ul, su = su_with_inf, uu = uu, E = E, F = F, gl = gl, gu = gu, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
@test size(lq_dense.data.A, 1) == size(E, 1) * 3 + sum(su_with_inf .!= Inf .|| sl_with_inf .!= -Inf) * N
# Test S matrix case
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, S = S, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, S = S, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, S = S, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, S = S, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test K matrix case without S
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test K matrix case with S
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test K matrix case with S and with partial bounds on u
nu = 2 # number of inputs
# generate random Q, R, A, and B matrices
Random.seed!(3)
R_rand = Random.rand(nu,nu)
R = R_rand * transpose(R_rand) + I
B = rand(ns, nu)
# generate upper and lower bounds
ul = fill(-20.0, nu)
uu = ul .+ 30
ul_with_inf = copy(ul)
uu_with_inf = copy(uu)
uu_with_inf[1] = Inf
ul_with_inf[1] = -Inf
F = rand(3, nu)
S = rand(ns, nu)
K = rand(nu, ns)
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, sl = sl, ul = ul_with_inf, su = su, uu = uu_with_inf, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul_with_inf, su = su, uu = uu_with_inf, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul_with_inf, su = su, uu = uu_with_inf, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; sl = sl, ul = ul_with_inf, su = su, uu = uu_with_inf, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test K with no bounds
model = build_QP_JuMP_model(Q,R,A,B, N;s0=s0, E = E, F = F, gl = gl, gu = gu, K = K, w = w)
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; E = E, F = F, gl = gl, gu = gu, K = K, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
lq_sparse_from_data = SparseLQDynamicModel(s0, A, B, Q, R, N; E = E, F = F, gl = gl, gu = gu, K = K, w = w)
lq_dense_from_data = DenseLQDynamicModel(s0, A, B, Q, R, N; E = E, F = F, gl = gl, gu = gu, K = K, w = w)
runtests(model, dnlp, lq_sparse, lq_dense, lq_sparse_from_data, lq_dense_from_data, N, ns, nu)
# Test get_* and set_* functions
dnlp = LQDynamicData(copy(s0), A, B, Q, R, N; sl = sl, ul = ul, su = su, uu = uu, E = E, F = F, gl = gl, gu = gu, K = K, S = S, w = w)
lq_sparse = SparseLQDynamicModel(dnlp)
lq_dense = DenseLQDynamicModel(dnlp)
@test get_A(dnlp) == A
@test get_A(lq_sparse) == A
@test get_A(lq_dense) == A
rand_val = rand()
Qtest = copy(Q)
Qtest[1, 2] = rand_val
set_Q!(dnlp, 1,2, rand_val)
@test get_Q(dnlp) == Qtest
Qtest[1, 1] = rand_val
set_Q!(lq_sparse, 1, 1, rand_val)
@test get_Q(lq_sparse) == Qtest
Qtest[2, 1] = rand_val
set_Q!(lq_dense, 2, 1, rand_val)
@test get_Q(lq_dense) == Qtest
rand_val = rand()
gltest = copy(gl)
gltest[1] = rand_val
set_gl!(dnlp, 1, rand_val)
@test get_gl(dnlp) == gltest
gltest[2] = rand_val
set_gl!(lq_sparse, 2, rand_val)
@test get_gl(lq_sparse) == gltest
gltest[3] = rand_val
set_gl!(lq_dense, 3, rand_val)
@test get_gl(lq_dense) == gltest
# Test non-default vector/matrix on GenericArrays
s0 = randn(Float32,2)
A = randn(Float32,2,2)
B = randn(Float32,2,2)
Q = randn(Float32,2,2)
R = randn(Float32,2,2)
S = randn(Float32,2,2)
K = randn(Float32,2,2)
E = randn(Float32,2,2)
F = randn(Float32,2,2)
gl = randn(Float32,2)
gu = gl .+ 2
sl = s0 .- 1
su = s0 .+ 1
ul = randn(Float32,2)
uu = ul .+ 2
w = Float32.(rand(0.0:.0001:.25, ns * 10))
s0 = Test.GenericArray(s0)
A = Test.GenericArray(A)
B = Test.GenericArray(B)
Q = Test.GenericArray(Q)
R = Test.GenericArray(R)
S = Test.GenericArray(S)
K = Test.GenericArray(K)
E = Test.GenericArray(E)
F = Test.GenericArray(F)
gl = Test.GenericArray(gl)
gu = Test.GenericArray(gu)
sl = Test.GenericArray(sl)
su = Test.GenericArray(su)
ul = Test.GenericArray(ul)
uu = Test.GenericArray(uu)
w = Test.GenericArray(w)
@test (DenseLQDynamicModel(s0, A, B, Q, R, 10; S = S, E = E, F = F, gl = gl, gu = gu, ul = ul, uu = uu, sl = sl, su = su, w = w) isa
DenseLQDynamicModel{Float32, GenericArray{Float32, 1}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, Nothing})
@test (DenseLQDynamicModel(s0, A, B, Q, R, 10; K = K, S = S, E = E, F = F, gl = gl, gu = gu, ul = ul, uu = uu, sl = sl, su = su, w = w) isa
DenseLQDynamicModel{Float32, GenericArray{Float32, 1}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, GenericArray{Float32, 2}, GenericArray{Float32, 2}})
@test (SparseLQDynamicModel(s0, A, B, Q, R, 10; S = S, E = E, F = F, gl = gl, gu = gu, ul = ul, uu = uu, sl = sl, su = su, w = w) isa
SparseLQDynamicModel{Float32, GenericArray{Float32, 1}, SparseMatrixCSC{Float32, Int64}, SparseMatrixCSC{Float32, Int64}, GenericArray{Float32, 2}, Nothing})
@test (SparseLQDynamicModel(s0, A, B, Q, R, 10; K = K, S = S, E = E, F = F, gl = gl, gu = gu, ul = ul, uu = uu, sl = sl, su = su, w = w) isa
SparseLQDynamicModel{Float32, GenericArray{Float32, 1}, SparseMatrixCSC{Float32, Int64}, SparseMatrixCSC{Float32, Int64}, GenericArray{Float32, 2}, GenericArray{Float32, 2}})
# Test LQJacobianOperator APIs
lq_dense_imp = DenseLQDynamicModel(dnlp; implicit=true)
@test length(get_jacobian(lq_dense_imp)) == (length(get_jacobian(lq_dense_imp).truncated_jac1)
+ length(get_jacobian(lq_dense_imp).truncated_jac2) + length(get_jacobian(lq_dense_imp).truncated_jac3))
(@test size(get_jacobian(lq_dense_imp)) == (size(get_jacobian(lq_dense_imp).truncated_jac1, 1) + size(get_jacobian(lq_dense_imp).truncated_jac2, 1)
+ size(get_jacobian(lq_dense_imp).truncated_jac3, 1), size(get_jacobian(lq_dense_imp).truncated_jac1, 2)))
@test isreal(get_jacobian(lq_dense_imp)) == isreal(get_jacobian(lq_dense_imp).truncated_jac1)
@test eltype(get_jacobian(lq_dense_imp)) == eltype(get_jacobian(lq_dense_imp).truncated_jac1)
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | code | 3816 | """
build_QP_JuMP_model(Q,R,A,B,N;...) -> JuMP.Model(...)
Return a `JuMP.jl` Model for the quadratic problem
min 1/2 ( sum_{i=1}^{N-1} s_i^T Q s + sum_{i=1}^{N-1} u^T R u + s_N^T Qf s_n )
s.t. s_{i+1} = As_i + Bs_i for i = 1,..., N-1
Optional Arguments
- `Qf = []`: matrix multiplied by s_N in objective function (defaults to Q if not given)
- `c = zeros(N*size(Q,1) + N*size(R,1)`: linear term added to objective funciton, c^T z
- `sl = fill(-Inf, size(Q,1))`: lower bound on state variables
- `su = fill(Inf, size(Q,1))`: upper bound on state variables
- `ul = fill(-Inf, size(Q,1))`: lower bound on input variables
- `uu = fill(Inf, size(Q,1))`: upper bound on input variables
- `s0 = []`: initial state of the first state variables
"""
function build_QP_JuMP_model(
Q,R,A,B, N;
s0 = zeros(size(Q, 1)),
sl = [],
su = [],
ul = [],
uu = [],
Qf = Q,
E = [],
F = [],
gl = [],
gu = [],
S = zeros(size(Q, 1), size(R, 1)),
K = zeros(size(R, 1), size(Q, 1)),
w = zeros(size(Q, 1))
)
ns = size(Q,1) # Number of states
nu = size(R,1) # Number of inputs
NS = 1:ns # set of states
NN = 1:N # set of times
NU = 1:nu # set of inputs
model = Model(MadNLP.Optimizer) # define model
@variable(model, s[NS, 0:N]) # define states
@variable(model, u[NU, 0:(N-1)]) # define inputs
if !iszero(K)
@variable(model, v[NU, 0:(N-1)])
end
# Bound states/inputs
if length(sl) > 0
for i in NS
for j in 0:N
if sl[i] != -Inf
@constraint(model, s[i,j] >= sl[i])
end
end
end
end
if length(su) > 0
for i in NS
for j in 0:N
if su[i] != Inf
@constraint(model, s[i,j] <= su[i])
end
end
end
end
if length(ul) > 0
for i in NU
for j in 0:(N-1)
if ul[i] != -Inf
@constraint(model, u[i,j] >= ul[i])
end
end
end
end
if length(uu) > 0
for i in NU
for j in 0:(N-1)
if uu[i] != Inf
@constraint(model, u[i,j] <= uu[i])
end
end
end
end
if length(s0) >0
for i in NS
JuMP.fix(s[i,0], s0[i])
end
end
# Give constraints from A, B, matrices
for s1 in NS
for t in 0:(N - 1)
@constraint(model, s[s1, t + 1] == sum(A[s1, s2] * s[s2, t] for s2 in NS) + sum(B[s1, u1] * u[u1, t] for u1 in NU) + w[s1 + t * ns])
end
end
# Constraints for Kx + v = u
if !iszero(K)
for u1 in NU
@constraint(model, [t in 0:(N - 1)], u[u1, t] == v[u1, t] + sum( K[u1, s1] * s[s1,t] for s1 in NS))
end
end
# Add E, F constraints
if length(E) > 0
for i in 1:size(E,1)
@constraint(model,[t in 0:(N-1)], gl[i] <= sum(E[i, s1] * s[s1, t] for s1 in NS) + sum(F[i,u1] * u[u1, t] for u1 in NU))
@constraint(model,[t in 0:(N-1)], gu[i] >= sum(E[i, s1] * s[s1, t] for s1 in NS) + sum(F[i,u1] * u[u1, t] for u1 in NU))
end
end
# Give objective function as xT Q x + uT R u where x is summed over T and u is summed over T-1
@objective(model,Min, sum( 1/2 * Q[s1, s2]*s[s1,t]*s[s2,t] for s1 in NS, s2 in NS, t in 0:(N-1)) +
sum( 1/2 * R[u1,u2] * u[u1, t] * u[u2,t] for t in 0:(N-1) , u1 in NU, u2 in NU) +
sum( 1/2 * Qf[s1,s2] * s[s1,N] * s[s2, N] for s1 in NS, s2 in NS) +
sum( S[s1, u1] * s[s1, t] * u[u1, t] for s1 in NS, u1 in NU, t in 0:(N-1))
)
return model
end
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | docs | 4786 | # DynamicNLPModels.jl
| **Documentation** | **Build Status** | **Coverage** |
|:-----------------:|:----------------:|:----------------:|
| [](https://madnlp.github.io/DynamicNLPModels.jl/dev) | [](https://github.com/MadNLP/DynamicNLPModels.jl/actions) | [](https://codecov.io/gh/MadNLP/DynamicNLPModels.jl) |
DynamicNLPModels.jl is a package for [Julia](https://julialang.org/) designed for representing linear [model predictive control (MPC)](https://en.wikipedia.org/wiki/Model_predictive_control) problems. It includes an API for building a model from user defined data and querying solutions.
## Installation
To install this package, please use
```julia
using Pkg
Pkg.add(url="https://github.com/MadNLP/DynamicNLPModels.jl.git")
```
or
```julia
pkg> add https://github.com/MadNLP/DynamicNLPModels.jl.git
```
## Overview
DynamicNLPModels.jl can construct both sparse and condensed formulations for MPC problems based on user defined data. We use the methods discussed by [Jerez et al.](https://doi.org/10.1016/j.automatica.2012.03.010) to eliminate the states and condense the problem. DynamicNLPModels.jl constructs models that are subtypes of `AbstractNLPModel` from [NLPModels.jl](https://github.com/JuliaSmoothOptimizers/NLPModels.jl) enabling both the sparse and condensed models to be solved with a variety of different solver packages in Julia. DynamicNLPModels was designed in part with the goal of solving linear MPC problems on the GPU. This can be done within [MadNLP.jl](https://github.com/MadNLP/MadNLP.jl) using [MadNLPGPU.jl](https://github.com/MadNLP/MadNLP.jl/tree/master/lib/MadNLPGPU).
The general sparse formulation used within DynamicNLPModels.jl is
$$\begin{align*}
\min_{s, u, v} \quad & s_N^\top Q_f s_N + \frac{1}{2} \sum_{i = 0}^{N-1} \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]^\top \left[ \begin{array}{cc} Q & S \\ S^\top & R \end{array} \right] \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]\\
\textrm{s.t.} \quad & s_{i+1} = As_i + Bu_i + w_i \quad \forall i = 0, 1, \cdots, N - 1 \\
& u_i = Ks_i + v_i \quad \forall i = 0, 1, \cdots, N - 1 \\
& g^l \le E s_i + F u_i \le g^u \quad \forall i = 0, 1, \cdots, N - 1\\
& s^l \le s_i \le s^u \quad \forall i = 0, 1, \cdots, N \\
& u^l \le u_i \le u^u \quad \forall i = 0, 1, \cdots, N - 1\\
& s_0 = \bar{s}
\end{align*}$$
where $s_i$ are the states, $u_i$ are the inputs$, $N$ is the time horizon, $\bar{s}$ are the initial states, and $Q$, $R$, $A$, and $B$ are user defined data. The matrices $Q_f$, $S$, $K$, $E$, and $F$ and the vectors $w$, $g^l$, $g^u$, $s^l$, $s^u$, $u^l$, and $u^u$ are optional data. $v_t$ is only needed in the condensed formulation, and it arises when $K$ is defined by the user to ensure numerical stability of the condensed problem.
The condensed formulation used within DynamicNLPModels.jl is
$$\begin{align*}
\min_{\boldsymbol{v}} \quad & \frac{1}{2} \boldsymbol{v}^\top \boldsymbol{H} \boldsymbol{v} + \boldsymbol{h}^\top \boldsymbol{v} + \boldsymbol{h}_0\\
\textrm{s.t.} \quad & d^l \le \boldsymbol{J} \boldsymbol{v} \le d^u.
\end{align*}$$
## Getting Started
DynamicNLPModels.jl takes user defined data to form a `SparseLQDyanmicModel` or a `DenseLQDynamicModel`. The user can first create an object containing the `LQDynamicData`, or they can pass the data directly to the `SparseLQDynamicModel` or `DenseLQDynamicModel` constructors.
```julia
using DynamicNLPModels, Random, LinearAlgebra
Q = 1.5 * Matrix(I, (3, 3))
R = 2.0 * Matrix(I, (2, 2))
A = rand(3, 3)
B = rand(3, 2)
N = 5
s0 = [1.0, 2.0, 3.0]
lqdd = LQDynamicData(s0, A, B, Q, R, N; **kwargs)
sparse_lqdm = SparseLQDynamicModel(lqdd)
dense_lqdm = DenseLQDynamicModel(lqdd)
# or
sparse_lqdm = SparseLQDynamicModel(s0, A, B, Q, R, N; **kwargs)
dense_lqdm = DenseLQDynamicModel(s0, A, B, Q, R, N; **kwargs)
```
Optional data (such as $s^l$, $s^u$, $S$, or $Q_f$) can be passed as key word arguments. The models `sparse_lqdm` or `dense_lqdm` can be solved by different solvers such as MadNLP.jl or Ipopt (Ipopt requires the extension NLPModelsIpopt.jl). An example script under `\examples` shows how the dense problem can be solved on a GPU using MadNLPGPU.jl.
DynamicNLPModels.jl also includes an API for querying solutions and reseting data. Solutions can be queried using `get_u(solver_ref, dynamic_model)` and `get_s(solver_ref, dynamic_model)`. The problem can be reset with a new $s_0$ by calling `reset_s0!(dynamic_model, s0)`.
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | docs | 59 | # API Manual
```@autodocs
Modules = [DynamicNLPModels]
```
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | docs | 7295 |
# Getting Started
DynamicNLPModels.jl takes user defined data to construct a linear MPC problem of the form
```math
\begin{aligned}
\min_{s, u, v} &\; s_N^\top Q_f s_N + \frac{1}{2} \sum_{i = 0}^{N-1} \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]^\top \left[ \begin{array}{cc} Q & S \\ S^\top & R \end{array} \right] \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]\\
\textrm{s.t.} &\;s_{i+1} = As_i + Bu_i + w_i \quad \forall i = 0, 1, \cdots, N - 1 \\
&\; u_i = Ks_i + v_i \quad \forall i = 0, 1, \cdots, N - 1 \\
&\; g^l \le E s_i + F u_i \le g^u \quad \forall i = 0, 1, \cdots, N - 1\\
&\; s^l \le s_i \le s^u \quad \forall i = 0, 1, \cdots, N \\
&\; u^l \le u_i \le u^u \quad \forall i = 0, 1, \cdots, N - 1\\
&\; s_0 = \bar{s}.
\end{aligned}
```
This data is stored within the struct `LQDynamicData`, which can be created by passing the data `s0`, `A`, `B`, `Q`, `R` and `N` to the constructor as in the example below.
```julia
using DynamicNLPModels, Random, LinearAlgebra
Q = 1.5 * Matrix(I, (3, 3))
R = 2.0 * Matrix(I, (2, 2))
A = rand(3, 3)
B = rand(3, 2)
N = 5
s0 = [1.0, 2.0, 3.0]
lqdd = LQDynamicData(s0, A, B, Q, R, N; **kwargs)
```
`LQDynamicData` contains the following fields. All fields after `R` are keyword arguments:
* `ns`: number of states (determined from size of `Q`)
* `nu`: number of inputs (determined from size of `R`)
* `N` : number of time steps
* `s0`: a vector of initial states
* `A` : matrix that is multiplied by the states that corresponds to the dynamics of the problem. Number of columns is equal to `ns`
* `B` : matrix that is multiplied by the inputs that corresonds to the dynamics of the problem. Number of columns is equal to `nu`
* `Q` : objective function matrix for system states from ``0, 1, \cdots, (N - 1)``
* `R` : objective function matrix for system inputs from ``0, 1, \cdots, (N - 1)``
* `Qf`: objective function matrix for system states at time ``N``
* `S` : objective function matrix for system states and inputs
* `E` : constraint matrix multiplied by system states. Number of columns is equal to `ns`
* `F` : constraint matrix multiplied by system inputs. Number of columns is equal to `nu`
* `K` : feedback gain matrix. Used to ensure numerical stability of the condensed problem. Not necessary within the sparse problem
* `w` : constant term within dynamic constraints. At this time, this is the only data that is time varying. This vector must be length `ns` * `N`, where each set of `ns` entries corresponds to that time (i.e., entries `1:ns` correspond to time ``0``, entries `(ns + 1):(2 * ns)` corresond to time ``1``, etc.)
* `sl` : lower bounds on state variables
* `su` : upper bounds on state variables
* `ul` : lower bounds on ipnut variables
* `uu` : upper bounds on input variables
* `gl` : lower bounds on the constraints ``Es_i + Fu_i``
* `gu` : upper bounds on the constraints ``Es_i + Fu_i``
## `SparseLQDynamicModel`
A `SparseLQDynamicModel` can be created by either passing `LQDynamicData` to the constructor or passing the data itself, where the same keyword options exist which can be used for `LQDynamicData`.
```julia
sparse_lqdm = SparseLQDynamicModel(lqdd)
# or
sparse_lqdm = SparseLQDynamicModel(s0, A, B, Q, R, N; **kwargs)
```
The `SparseLQDynamicModel` contains four fields:
* `dynamic_data` which contains the `LQDynamicData`
* `data` which is the `QPData` from [QuadraticModels.jl](https://github.com/JuliaSmoothOptimizers/QuadraticModels.jl). This object also contains the following data:
- `H` which is the Hessian of the linear MPC problem
- `A` which is the Jacobian of the linear MPC problem such that ``\textrm{lcon} \le A z \le \textrm{ucon}``
- `c` which is the linear term of a quadratic objective function
- `c0` which is the constant term of a quadratic objective function
* `meta` which contains the `NLPModelMeta` for the problem from NLPModels.jl
* `counters` which is the `Counters` object from NLPModels.jl
!!! note
The `SparseLQDynamicModel` requires that all matrices in the `LQDynamicData` be the same type. It is recommended that the user be aware of how to most efficiently store their data in the `Q`, `R`, `A`, and `B` matrices as this impacts how efficiently the `SparseLQDynamicModel` is constructed. When `Q`, `R`, `A`, and `B` are sparse, building the `SparseLQDynamicModel` is much faster when these are passed as sparse rather than dense matrices.
## `DenseLQDynamicModel`
The `DenseLQDynamicModel` eliminates the states within the linear MPC problem to build an equivalent optimization problem that is only a function of the inputs. This can be particularly useful when the number of states is large compared to the number of inputs.
A `DenseLQDynamicModel` can be created by either passing `LQDynamicData` to the constructor or passing the data itself, where the same keyword options exist which can be used for `LQDynamicData`.
```julia
dense_lqdm = DenseLQDynamicModel(lqdd)
# or
dense_lqdm = DenseLQDynamicModel(s0, A, B, Q, R, N; **kwargs)
```
The `DenseLQDynamicModel` contains five fields:
* `dynamic_data` which contains the `LQDynamicData`
* `data` which is the `QPData` from [QuadraticModels.jl](https://github.com/JuliaSmoothOptimizers/QuadraticModels.jl). This object also contains the following data:
- `H` which is the Hessian of the condensed linear MPC problem
- `A` which is the Jacobian of the condensed linear MPC problem such that ``\textrm{lcon} \le A z \le \textrm{ucon}``
- `c` which is the linear term of the condensed linear MPC problem
- `c0` which is the constant term of the condensed linear MPC problem
* `meta` which contains the `NLPModelMeta` for the problem from NLPModels.jl
* `counters` which is the `Counters` object from NLPModels.jl
* `blocks` which contains the data needed to condense the model and then to update the condensed model when `s0` is reset.
The `DenseLQDynamicModel` is formed from dense matrices, and this dense system can be solved on a GPU using MadNLP.jl and MadNLPGPU.jl For an example script for performing this, please see the the [examples directory](https://github.com/MadNLP/DynamicNLPModels.jl/tree/main/examples) of the main repository.
## API functions
An API has been created for working with `LQDynamicData` and the sparse and dense models. All functions can be seen in the API Manual section. However, we give a short overview of these functions here.
* `reset_s0!(LQDynamicModel, new_s0)`: resets the model in place with a new `s0` value. This could be called after each sampling period in MPC to reset the model with a new measured value
* `get_s(solver_ref, LQDynamicModel)`: returns the optimal solution for the states from a given solver reference
* `get_u(solver_ref, LQDynamicModel)`: returns the optimal solution for the inputs from a given solver reference; when `K` is defined, the solver reference contains the optimal ``v`` values rather than optimal ``u`` values, adn this function converts ``v`` to ``u`` and returns the ``u`` values
* `get_*`: returns the data of `*` where `*` is an object within `LQDynamicData`
* `set_*!`: sets the value within the data of `*` for a given entry to a user defined value
| DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.0 | 4c035c67eee91a19afdc5db63eb71464c5db32ba | docs | 3275 | # Introduction
[DynamicNLPModels.jl](https://github.com/MadNLP/DynamicNLPModels.jl) is a package for [Julia](https://julialang.org/) designed for representing linear [model predictive control (MPC)](https://en.wikipedia.org/wiki/Model_predictive_control) problems. It includes an API for building a model from user defined data and querying solutions.
!!! note
This documentation is also available in [PDF format](DynamicNLPModels.pdf).
## Installation
To install this package, please use
```julia
using Pkg
Pkg.add(url="https://github.com/MadNLP/DynamicNLPModels.jl.git")
```
or
```julia
pkg> add https://github.com/MadNLP/DynamicNLPModels.jl.git
```
## Overview
DynamicNLPModels.jl can construct both sparse and condensed formulations for MPC problems based on user defined data. We use the methods discussed by [Jerez et al.](https://doi.org/10.1016/j.automatica.2012.03.010) to eliminate the states and condense the problem. DynamicNLPModels.jl constructs models that are subtypes of `AbstractNLPModel` from [NLPModels.jl](https://github.com/JuliaSmoothOptimizers/NLPModels.jl) enabling both the sparse and condensed models to be solved with a variety of different solver packages in Julia. DynamicNLPModels was designed in part with the goal of solving linear MPC problems on the GPU. This can be done within [MadNLP.jl](https://github.com/MadNLP/MadNLP.jl) using [MadNLPGPU.jl](https://github.com/MadNLP/MadNLP.jl/tree/master/lib/MadNLPGPU).
The general sparse formulation used within DynamicNLPModels.jl is
```math
\begin{aligned}
\min_{s, u, v} &\; s_N^\top Q_f s_N + \frac{1}{2} \sum_{i = 0}^{N-1} \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]^\top \left[ \begin{array}{cc} Q & S \\ S^\top & R \end{array} \right] \left[ \begin{array}{c} s_i \\ u_i \end{array} \right]\\
\textrm{s.t.} &\;s_{i+1} = As_i + Bu_i + w_i \quad \forall i = 0, 1, \cdots, N - 1 \\
&\; u_i = Ks_i + v_i \quad \forall i = 0, 1, \cdots, N - 1 \\
&\; g^l \le E s_i + F u_i \le g^u \quad \forall i = 0, 1, \cdots, N - 1\\
&\; s^l \le s_i \le s^u \quad \forall i = 0, 1, \cdots, N \\
&\; u^l \le u_t \le u^u \quad \forall i = 0, 1, \cdots, N - 1\\
&\; s_0 = \bar{s}
\end{aligned}
```
where ``s_i`` are the states, ``u_i`` are the inputs, ``N`` is the time horizon, ``\bar{s}`` are the initial states, and ``Q``, ``R``, ``A``, and ``B`` are user defined data. The matrices ``Q_f``, ``S``, ``K``, ``E``, and ``F`` and the vectors ``w``, ``g^l``, ``g^u``, ``s^l``, ``s^u``, ``u^l``, and ``u^u`` are optional data. ``v_t`` is only needed in the condensed formulation, and it arises when ``K`` is defined by the user to ensure numerical stability of the condensed problem.
The condensed formulation used within DynamicNLPModels.jl is
```math
\begin{aligned}
\min_{\boldsymbol{v}} &\;\; \frac{1}{2} \boldsymbol{v}^\top \boldsymbol{H} \boldsymbol{v} + \boldsymbol{h}^\top \boldsymbol{v} + \boldsymbol{h}_0\\
\textrm{s.t.} &\; d^l \le \boldsymbol{J} \boldsymbol{v} \le d^u.
\end{aligned}
```
# Bug reports and support
This package is new and still undergoing some development. If you encounter a bug, please report it through Github's [issue tracker](https://github.com/MadNLP/DynamicNLPModels.jl/issues). | DynamicNLPModels | https://github.com/MadNLP/DynamicNLPModels.jl.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 79 | using Documenter, TopologyPreprocessing
makedocs(sitename="My Documentation")
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 20085 | using Eirene
using Pipe
#%%
# ==============================
# ======== Tested code ========
#%%
# ================================
# ======== Untested code ========
"""
get_barcodes(results_eirene::Dict, max_dim::Integer; min_dim::Int=1)
Calls Eirene.barcode for 'dim' in range from `min_dim` up to 'max_dim' and
stack the resulting Arrays into a vector.
The returned value is a Vector of Arrays{Float64,2}. Each array is of
different size, because of different number of detected cycles. First column
of each array contains birth step, second column contains death step.
Arrays in returned vector correspond to Betti curve dimensions form range
`min_dim` up to 'max_dim'.
"""
function get_barcodes(results_eirene::Dict, max_dim::Integer; min_dim::Int = 1, sorted::Bool = false)
barcodes = Matrix{Float64}[]
for d = min_dim:max_dim
result = barcode(results_eirene, dim = d)
if isempty(result) && d > 1
result = zeros(size(barcodes[d-1]))
end
if sorted
result = result[sortperm(result[:, 1]), :]
end
push!(barcodes, result)
end
return barcodes
end
"""
plot_barcodes(barcodes; min_dim::Integer=1, betti_labels::Bool=true, default_labels::Bool=true kwargs...)
Creates a plot for set of barcodesstored in `barcodes` and return the
handler to the plot.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
"""
function plot_barcodes!(barcodes::Vector, plot_ref;
min_dim::Integer = 1,
barcodes_labels::Bool = true,
default_labels::Bool = true,
sort_by_birth::Bool = false,
alpha::Float64 = 1.0,
kwargs...)#; plot_size = (width=1200, height=800),
# TODO add change of x label based on x values- so it is either edge density for 0:1 range values or Filtration step otherwise
# TODO add ordering of bars to firstly birth time, then death time
# TODO dims should be given as range, not a single min dim
# barcodes = all_barcodes_geom
max_dim = size(barcodes, 1) - (1 - min_dim) # TODO not sure if this is correct solution
if min_dim > max_dim
throw(DomainError(
min_dim,
"\'min_dim\' must be greater that maximal dimension in \'bettis\'",
))
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 8
end
colors_set = get_bettis_color_palete(min_dim = min_dim)
dims_indices = 1:length(min_dim:max_dim)
all_sizes = [size(barcodes[k], 1) for k = dims_indices]
ranges_sums = vcat(0, [sum(all_sizes[1:k]) for k = dims_indices])
y_val_ranges = [ranges_sums[k]+1:ranges_sums[k+1] for k = dims_indices]
if sort_by_birth
sort_barcodes!(barcodes, min_dim, max_dim)
end
total_cycles = sum([size(barcodes[k], 1) for (k, dim) in enumerate(min_dim:max_dim)])
# for p = min_dim:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
for (p, dim) = enumerate(min_dim:max_dim)# 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# @info p, dim
args = (lc = colors_set[p], linewidth = lw)
# b = barcodes[p][1:1:(end-1),:]
b = barcodes[p][:, :]
all_infs = findall(x -> isinf(x), b)
for k in all_infs
b[k] = 1
end
# if dim == 0
# b = sort(b, dims = 1)
# end
total_bars = size(b, 1)
y_vals = [[k, k] for k in y_val_ranges[p]]
lc = colors_set[p]
for k = 1:total_bars
# TODO change label to empty one
plot!(b[k, :], y_vals[k]; label = "", lc = lc, alpha = alpha)#; args...)
end
if false && betti_labels
label = "β$(dim)"
end
# plot!(label=label)
end
# display(plot_ref)
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
all_labels = reshape(["β$(k)" for k in 1:max_dim], (1, max_dim))
plot!(label = all_labels)
plot!(legend = true)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Birth/Death")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Cycle")
end
# ylims!(0, 2*total_cycles)
ylims!(0, total_cycles + 2)
return plot_ref
end
function plot_barcodes(barcodes::Vector;
kwargs...)
plot_ref = plot(; kwargs...)
plot_barcodes!(barcodes, plot_ref;
kwargs...)
end
function sort_barcodes!(barcodes, min_dim, max_dim)
sorted_barcodes = copy(barcodes)
for (dim_index, dim) = enumerate(min_dim:max_dim)
# if dim == 0
# permutation = sortperm(barcodes[dim_index][:, 2])
# else
permutation = sortperm(barcodes[dim_index][:, 1])
# end
sorted_barcodes[dim_index] = barcodes[dim_index][permutation, :]
# end
end
barcodes = sorted_barcodes
# for (dim_index, dim) = enumerate(min_dim:max_dim)
# if dim == 0
# sort!(barcodes[dim_index], dims = 1)
# else
# sort!(barcodes[dim_index], dims = 1)
# end
# end
end
# TODO This has to be imported from other file
# function get_bettis_color_palete(; min_dim = 1, use_set::Integer = 1)
# """
# function get_bettis_color_palete()
#
# Generates vector with colours used for Betti plots. Designed for Betti plots consistency.
# """
# # TODO what does the number in the function below is used for?
#
# if use_set == 1
# cur_colors = [Gray(bw) for bw = 0.0:0.025:0.5]
# if min_dim == 0
# colors_set = [RGB(87 / 256, 158 / 256, 0 / 256)]
# else
# colors_set = RGB[]
# end
# colors_set = vcat(
# colors_set,
# [
# RGB(255 / 256, 206 / 256, 0 / 256),
# RGB(248 / 256, 23 / 256, 0 / 256),
# RGB(97 / 256, 169 / 256, 255 / 256),
# RGB(163 / 256, 0 / 256, 185 / 256),
# RGB(33 / 256, 96 / 256, 45 / 256),
# RGB(4 / 256, 0 / 256, 199 / 256),
# RGB(135 / 256, 88 / 256, 0 / 256),
# ],
# cur_colors,
# )
# else
# use_set == 2
# cur_colors = get_color_palette(:auto, 1)
# cur_colors3 = get_color_palette(:lightrainbow, 1)
# cur_colors2 = get_color_palette(:cyclic1, 1)
# if min_dim == 0
# # colors_set = [cur_colors[3], cur_colors[5], [:red], cur_colors[1]] #cur_colors[7],
# colors_set = [cur_colors3[3], cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
# else
# colors_set = [cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
# # colors_set = [cur_colors[5], [:red], cur_colors[1], cur_colors[14]]
# end
# # for c = [collect(11:25);]
# # push!(colors_set, cur_colors2[c])
# # end
# colors_set = vcat(colors_set, [cur_colors2[c] for c in [collect(11:25);]])
# end
#
# return colors_set
# end
function get_birth_death_ratio(barcodes; max_dim::Integer = 3)
# birth_death_raio_π = [[all_barcodes_geom[k][m,2]/all_barcodes_geom[k][m,1] for m= 1:size(all_barcodes_geom[k],1)] for k in 1:max_dim]
birth_death_ratio_π = [barcodes[k][:, 2] ./ barcodes[k][:, 1] for k in 1:max_dim]
return birth_death_ratio_π
end
function get_barcode_lifetime(barcodes; max_dim::Integer = 3)
lifetime = [barcodes[k][:, 2] .- barcodes[k][:, 1] for k in 1:max_dim]
return lifetime
end
#%%
"""
get_barcode_max_lifetime(lifetimes, min_dim, max_dim)
Returns the maximal life times of barcode for all dimensions.
"""
function get_barcode_max_lifetime(lifetimes)
total_lifetimes = length(lifetimes)
all_max_lifetimes = zeros(total_lifetimes, 1)
for k in 1:total_lifetimes
all_max_lifetimes[k] = findmax(lifetimes[k])[1]
end
return all_max_lifetimes
end
"""
boxplot_birth_death(areas_matrix, min_dim::Integer, max_dim::Integer)
Plots the boxplot of area under betti curves.
"""
function boxplot_birth_death(birth_death_ratio_π, min_dim::Integer, max_dim::Integer)
bplot = StatsPlots.boxplot()
data_colors = get_bettis_color_palete()
total_plots = size(birth_death_ratio_π, 1)
for k in 1:total_plots
StatsPlots.boxplot!(bplot, birth_death_ratio_π[k], labels = "β$(k)", color = data_colors[k])
StatsPlots.dotplot!(bplot, birth_death_ratio_π[k], color = data_colors[k])
end
return bplot
end
"""
boxplot_birth_death(areas_matrix, min_dim::Integer, max_dim::Integer)
Plots the boxplot of area under betti curves.
"""
function boxplot_lifetime(barcode_lifetime, min_dim::Integer, max_dim::Integer)
bplot = StatsPlots.boxplot()
data_colors = get_bettis_color_palete()
total_plots = size(barcode_lifetime, 1)
for k in 1:total_plots
StatsPlots.boxplot!(bplot, barcode_lifetime[k], labels = "β$(k)", color = data_colors[k])
StatsPlots.dotplot!(bplot, barcode_lifetime[k], color = data_colors[k])
end
return bplot
end
#%%
"""
get_barcode_max_db_ratios(lifetimes, min_dim, max_dim)
Returns the maximal life times of barcode for all dimensions.
"""
function get_barcode_max_db_ratios(db_ratos)
total_db = length(db_ratos)
all_max_db = zeros(total_db, 1)
for k in 1:total_db
all_max_db[k] = findmax(db_ratos[k])[1]
end
return all_max_db
end
"""
get_normalised_barcodes(barcodes::Vector, betti_numbers::Array)
Returns the barcodes which values are within [0,1] range.
1. get corresponding bettis
2. get max number of steps
3. divide all values by total number of steps.
"""
function get_normalised_barcodes(barcodes, betti_numbers::Array)
if typeof(betti_numbers) == Vector
total_steps = size(betti_numbers[1], 1)
else
total_steps = size(betti_numbers, 1)
end
return barcodes ./ total_steps
end
"""
get_normalised_barcodes_collection(barcodes_collection, bettis_collection)
Applies get_normalised_barcodes to the collection of barcodes and corresponding
betti curves.
"""
function get_normalised_barcodes_collection(barcodes_collection, bettis_collection)
if size(barcodes_collection, 1) != size(bettis_collection, 1)
throw(BoundsError(barcodes_collection, bettis_collection,
"Both collections must have same number of elements",
))
else
total_collections = size(barcodes_collection, 1)
end
normed_collection = deepcopy(barcodes_collection)
for k = 1:total_collections
normed_collection[k] = get_normalised_barcodes(barcodes_collection[k], bettis_collection[k])
end
return normed_collection
end
"""
plot_bd_diagram(barcodes;
dims::Range,
use_js::Bool=false,
kwargs...)
Creates a birth/death diagram from `barcodes` and returns the handlers to
the plots.
By default, dims is set to range '1:length(barcodes)', which plots all of
the diagrams. If set to an integer, plots only 1 dimension.
If 'use_js' is set to true, plotly backend is used for plotting.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO dims are defined as if the dimensions always starts at 1- this has to be changed
"""
function plot_bd_diagram(barcodes; dims = 1:length(barcodes),
use_js::Bool = false,
class_sizes = [],
class_labels = [],
normilised_diagonal::Bool = true,
alpha=0.4,
kwargs...)
# TODO max min should be ready to use from input data- might be better to have better structures as an inupt
# max_dim = size(barcodes, 1)
# min_dim = findmin(dims)[1]
min_dim = dims[1]
max_dim = dims[end]
if max_dim > length(barcodes)
throw(DimensionMismatch("Can not have dims range larger than barcodes length"))
end
# all_dims = min_dim:max_dim
# if findmax(dims)[1] > max_dim
# throw(DomainError(
# min_dim,
# "\'dims\' must be less than maximal dimension in \'bettis\'",
# ))
# end
# lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
# if !isnothing(lw_pos)
# lw = kwargs[lw_pos]
# else
# lw = 2
# end
colors_set = TopologyPreprocessing.get_bettis_color_palete(min_dim = min_dim)
if use_js
plotly()
else
gr()
end
plot_ref = plot(; xlims = (0, 1), ylims = (0, 1), kwargs...)
# Add diagonal
if normilised_diagonal
max_coord = 1
else
max_x = max([k for k in vcat([barcodes[d][:,1] for (d, dim) in dims|> enumerate]...) if !isinf(k) ]...)
max_y = max([k for k in vcat([barcodes[d][:,2] for (d, dim) in dims|> enumerate]...) if !isinf(k) ]...)
max_coord = max(max_x, max_y)
end
scaling_factor = 1.05
min_val = -0.05
plot!([0, scaling_factor*max_coord], [0, scaling_factor*max_coord], label = "")
xlims!(min_val, scaling_factor*max_coord)
ylims!(min_val, scaling_factor*max_coord)
for (p, dim) in enumerate(dims)
# colors_set[p]
my_vec = barcodes[p]
# TODO class size is not a default and basic bd diagram property- should be factored out to other function
if class_labels != [] && class_sizes != []
labels = ["class/size $(class_labels[k])/$(class_sizes[class_labels[k]])" for k in 1:size(class_labels, 1)]
elseif class_sizes == []
labels = ["class: $(k)" for k in 1:size(my_vec, 1)]
else
labels = ["class/size $(k)/$(class_sizes[k])" for k in 1:size(my_vec, 1)]
end
args = (color = colors_set[p],
# linewidth = lw,
label = "β$(dim)",
aspect_ratio = 1,
size = (600, 600),
legend = :bottomright,
framestyle=:origin,
alpha=alpha,
# hover = labels,
kwargs...)
if class_labels != []
class_sizes != []
for x = class_labels
plot!(my_vec[Int(x), 1], my_vec[Int(x), 2], seriestype = :scatter; args...)
end
end
plot!(my_vec[:, 1], my_vec[:, 2], seriestype = :scatter; args...)
end
xlabel!("birth")
ylabel!("death")
return plot_ref
end
#
"""
plot_all_bd_diagrams(barcodes_collection;
min_dim::Integer=1,
betti_labels::Bool=true,
default_labels::Bool=true,
all_legend=false,
my_alpha=0.12,
aspect_ratio=1,
kwargs...)
Creates a set of birth/death diagrams from `barcodes_collection`
and returns a dictionary with the handlers to the plots.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
"""
function plot_all_bd_diagrams(barcodes_collection;
min_dim::Integer = 1,
betti_labels::Bool = true,
default_labels::Bool = true,
all_legend = false,
my_alpha = 0.12,
aspect_ratio = 1,
base_w = 600,
base_h = 600,
kwargs...)
total_dims = size(barcodes_collection[1], 1)
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
title_pos = findfirst(x -> x == :title, keys(kwargs))
if !isnothing(title_pos)
my_title = kwargs[title_pos]
else
my_title = "Birth death diagram"
end
colors_set = TopologyPreprocessing.get_bettis_color_palete(min_dim = min_dim)
plot_dict = Dict()
for b = 1:total_dims
args = (lc = colors_set[b],
linewidth = lw,
label = false,
aspect_ratio = aspect_ratio,
size = (base_w, base_h),
kwargs...)
plot_dict["β$(b)"] = scatter(; xlims = (0, 1), ylims = (0, 1), dpi = 300, args...)
for bars = barcodes_collection
barcode = bars[b]
scatter!(barcode[:, 1], barcode[:, 2],
markeralpha = my_alpha,
markercolor = colors_set[b],
dpi = 300)
end
plot!(legend = all_legend)
plot!(title = (my_title * ", β$(b)"))
# legend_pos = findfirst(x -> x == :legend, keys(kwargs))
# if !isnothing(legend_pos)
# plot!(legend = kwargs[legend_pos])
# else
# plot!(legend = betti_labels)
# end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Birth")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Death")
end
end
return plot_dict
end
## ===-
# Simpler plotting
"""
plot_bd_diagram(barcodes;
dims::Range,
use_js::Bool=false,
kwargs...)
Creates a birth/death diagram from `barcodes` and returns the handlers to
the plots.
By default, dims is set to range '1:length(barcodes)', which plots all of
the diagrams. If set to an integer, plots only 1 dimension.
If 'use_js' is set to true, plotly backend is used for plotting.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO dims are defined as if the dimensions always starts at 1- this has to be changed
"""
function plot_simple_bd_diagram(barcodes; dims = 1:length(barcodes), max_bd = 0, use_js::Bool = false,
kwargs...)
# TODO max min should be ready to use from input data- might be better to have better structures as an inupt
max_dim = size(barcodes, 1)
min_dim = findmin(dims)[1]
# all_dims = min_dim:max_dim
if findmax(dims)[1] > max_dim
throw(DomainError(
min_dim,
"\'dims\' must be less than maximal dimension in \'bettis\'",
))
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
colors_set = TopologyPreprocessing.get_bettis_color_palete(min_dim = 1)
if use_js
plotly()
else
gr()
end
plot_ref = plot(; kwargs...)
for (p, dim) in dims |> enumerate
# colors_set[p]
my_vec = barcodes[p]
args = (color = colors_set[p],
linewidth = lw,
aspect_ratio = 1,
size = (600, 600),
legend = :bottomright,
kwargs...)
# scatter!(my_vec[:, 1], my_vec[:, 2], args...)
plot!(my_vec[:, 1], my_vec[:, 2], seriestype = :scatter; args...)
end
# Add diagonal
if max_bd > 0
max_x = max_bd
max_y = max_bd
plot!([0, max_y], [0, max_y], label = "")
else
all_births = vcat([barcodes[d][:, 1] for d in dims]...)
all_deaths = vcat([barcodes[d][:, 2] for d in dims]...)
max_x = findmax(all_births)[1]
max_y = findmax(all_deaths)[1]
plot!([0, max_y], [0, max_y], label = "")
end
return plot_ref
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 28399 | # ==============================
# ======== Tested code ========
using Eirene
using Plots
using StatsPlots
#%%
"""
get_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int=1)
Calls Eirene.betticurve for 'dim' in range from `min_dim` up to 'max_dim' and
stack the resulting Arrays into a vector.
The returned value is a Vector of Arrays{Float64,2}. Each array is of size
(n,2), where n is the maximal number of steps taken to compute Betti curve of dimensions
ranging form `min_dim` to `max_dim`. First column of each array contains numbered steps.
Second column are the Betti curve values for corresponding step.
Arrays in returned vector correspond to Betti curve dimensions form range
`min_dim` up to 'max_dim'.
"""
function get_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int = 1)
bettis = Matrix{Float64}[]
for d = min_dim:max_dim
result = betticurve(results_eirene, dim = d)
if isempty(result) && d > 1
result = zeros(size(bettis[d-1]))
end
push!(bettis, result)
end
return bettis
end
# TODO add get_bettis_from_matrix, to wrap C= eirene...; get bettis
#%%
"""
normalise_bettis(bettis::Vector)
normalise_bettis(bettis::Array)
Normalise the number of steps for Betti curves. 'bettis' can be either vector of
arrays (each array contain Betti curve of different dimension) or an array
containing Betti curve of a single dimension.
"""
function normalise_bettis(bettis::Vector)
@debug "Vector version"
norm_bettis = copy(bettis)
@debug "norm_bettis size :" size(norm_bettis)[1][1]
max_dim = size(norm_bettis)[1]
@debug "typeof(max_dim) :" typeof(max_dim[1])
for d = 1:(max_dim)
if !isempty(norm_bettis[d])
norm_bettis[d][:, 1] /= findmax(norm_bettis[d][:, 1])[1]
end
end
return norm_bettis
end
#%%
function normalise_bettis(bettis::Array)
@debug "Array version"
norm_bettis = copy(bettis)
@debug "norm_bettis size :" size(norm_bettis)
if !isempty(norm_bettis)
norm_bettis[:, 1] /= findmax(norm_bettis[:, 1])[1]
end
return norm_bettis
end
#%%
# function vectorize_bettis(betti_curves::Array{Matrix{Float64,2}})
"""
vectorize_bettis(betti_curves::Matrix{Float64})
Reshapes the 'betti_curves' from type Array{Matrices{Float64,2}} into
Matrix{Float64}.
The resulting matrix size is (n, k), where 'n' is equal to the number of
rows in each matrix, 'k' is equal to the number of matrices.
TODO: Change the name- it takse vector and returns a matrix.
TODO: get bettis could have an arguent betti_type which would determine resulting type
"""
function vectorize_bettis(betti_curves::Vector{Array{Float64,2}})
first_betti = 1
last_betti = size(betti_curves,1)
return hcat([betti_curves[k][:, 2] for k = first_betti:last_betti]...)
end
#%%
@deprecate vectorize_bettis(eirene_results::Dict, maxdim::Integer, mindim::Integer) vectorize_bettis(betti_curves)
# ===
#%%
"""
get_vectorized_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int=1)
Takes the eirene result and computes Betti curves for dimensions in range
'mindim:maxdim'. Every Betti curve is stored in successive column of the
resulting array.
TODO: this should return a matrix, where first col are indices and rest are B values (1st col is missing now)
"""
function get_vectorized_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int = 1)
all_bettis = get_bettis(results_eirene, max_dim, min_dim = min_dim)
bettis_vector = vectorize_bettis(all_bettis)
return bettis_vector
end
# ==
#%%
"""
plot_bettis(bettis; min_dim::Integer=1, betti_labels::Bool=true, default_labels::Bool=true kwargs...)
Creates a plot for set of betti numbers stored in `bettis` and return the
handler to the plot.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO: min_dim is not included in all_dims variable
TODO: add change of x label based on x values- so it is either edge density for 0:1 range values or Filtration step otherwise
"""
function plot_bettis(bettis::Vector;
min_dim::Integer = 1,
use_edge_density::Bool=true,
betti_labels::Bool = true,
default_labels::Bool = true,
kwargs...)#; plot_size = (width=1200, height=800),
max_dim = size(bettis, 1)
all_dims = 1:max_dim
if min_dim > max_dim
throw(DomainError(
min_dim,
"\'min_dim\' must be greater that maximal dimension.",
))
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
# Create iterator for all loops
all_iterations = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
if use_edge_density
for p = all_iterations
max_step = findmax(bettis[p][:, 1])[1]
bettis[p][:, 1] ./= max_step
end
end
colors_set = get_bettis_color_palete(min_dim=min_dim)
plot_ref = plot(; kwargs...)
# for p = min_dim:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for p = all_iterations
for (index, p) in enumerate(min_dim:max_dim)
args = (lc = colors_set[index], linewidth = lw)
if betti_labels
args = (args..., label = "β$(p)")
end
plot!(bettis[index][:, 1], bettis[index][:, 2]; args...)
end
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
# set tlims to integer values
max_ylim = findmax(ceil.(Int, ylims(plot_ref)))[1]
if max_ylim <=3
ylims!((0, 3))
end
if use_edge_density
xlims!((0, 1))
end
return plot_ref
end
"""
plot_bettis(bettis::Array; min_dim::Integer=1, betti_labels::Bool=true, default_labels::Bool=true kwargs...)
Creates a plot for set of betti numbers stored in `bettis` and return the
handler to the plot.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO: add change of x label based on x values- so it is either edge density for 0:1 range values or Filtration step otherwise
"""
function plot_bettis(bettis::Array;
# min_dim::Integer = 1,
dims_range=1:size(bettis,2),
use_edge_density::Bool=true,
betti_labels::Bool = true,
default_labels::Bool = true,
normalised=true,
kwargs...)#; plot_size = (width=1200, height=800),
max_dim = dims_range[end]
min_dim = dims_range[1]
if min_dim > max_dim
throw(DomainError(
min_dim,
"\'min_dim\' must be greater that maximal dimension in \'bettis\'",
))
end
total_steps = size(bettis, 1)
if normalised
x_vals = range(0, stop=1, length=total_steps)
else
x_vals = range(0, stop=total_steps)
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
# if use_edge_density
# # for p = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for (index, p) in enumerate(min_dim:max_dim)
# max_step = findmax(bettis[:, 1])[1]
# bettis[p][:, 1] ./=max_step
# end
# end
colors_set = get_bettis_color_palete(min_dim=min_dim)
plot_ref = plot(; kwargs...)
# for p = min_dim:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for p = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
for (index, p) in enumerate(min_dim:max_dim)
args = (lc = colors_set[index], linewidth = lw)
if betti_labels
args = (args..., label = "β$(p)")
end
plot!(x_vals, bettis[:, index]; args...)
end
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
# set tlims to integer values
max_ylim = findmax(ceil.(Int, ylims(plot_ref)))[1]
if max_ylim <=3
ylims!((0, 3))
end
if use_edge_density
xlims!((0, 1))
end
return plot_ref
end
# ======= Untested code
# TODO add default kwargs paring function -> parse_kwargs()
"""
plot_all_bettis ...
"""
function plot_all_bettis(bettis_collection;
min_dim::Integer = 1,
betti_labels::Bool = true,
default_labels::Bool = true,
normalised=true,
kwargs...)#; plot_size = (width=1200, height=800),
total_dims = size(bettis_collection[1],2)
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
colors_set = get_bettis_color_palete(min_dim=min_dim)
max_y_val = find_max_betti(bettis_collection)
plot_ref = plot(; kwargs...)
for b = 1:total_dims
args = (lc = colors_set[b], linewidth = lw, alpha=0.12,label=false, ylims=(0,max_y_val))
for bettis = bettis_collection
betti_vals = bettis[:,b]
total_steps = size(bettis, 1)
x_vals = range(0, stop=1, length=total_steps)
plot!(x_vals, betti_vals; args...)
end
# my_label = "β$(b)"
# betti_vals = results_d["bettis_collection"][:hc][end]
# x_vals = range(0, stop=1, length=size(betti_vals, 1))
# plot!(x_vals, betti_vals; lc = colors_set[b], linewidth = 1, alpha=0.1,label=my_label, ylims=(0,max_y_val))
end
plot!(legend=true)
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
return plot_ref
end
"""
find_max_betti(bettis_collection::Array)
Returns the highest Betti curve value from all dimensions.
"""
function find_max_betti(bettis_collection::Array)
if typeof(bettis_collection) == Vector
bettis_collection = vectorize_bettis(bettis_collection)
end
max_y_val = 0
for betti_set in bettis_collection
local_max = findmax(betti_set)[1]
if local_max > max_y_val
max_y_val = local_max
end
end
return max_y_val
end
# ======= Untested code == end
#%%
"""
printready_plot_bettis(kwargs)
Creates a plot using 'plot_bettis' with arguments which were tested to be very
good for using them in prints. Used arguments are:
"""
function printready_plot_bettis(kwargs)
return nothing
end
#%%
"""
function get_bettis_color_palete()
Generates vector with colours used for Betti plots. Designed for Betti plots consistency.
"""
function get_bettis_color_palete(; min_dim = 1, use_set::Integer = 1)
# TODO what does the number in the function below is used for?
if use_set == 1
cur_colors = [Gray(bw) for bw = 0.0:0.025:0.5]
if min_dim == 0
colors_set = [RGB(87 / 256, 158 / 256, 0 / 256)]
else
colors_set = []
end
max_RGB = 256
colors_set = vcat(
colors_set,
[
RGB(255 / max_RGB, 206 / max_RGB, 0 / max_RGB),
RGB(248 / max_RGB, 23 / max_RGB, 0 / max_RGB),
RGB(97 / max_RGB, 169 / max_RGB, 255 / max_RGB),
RGB(163 / max_RGB, 0 / max_RGB, 185 / max_RGB),
RGB(33 / max_RGB, 96 / max_RGB, 45 / max_RGB),
RGB(4 / max_RGB, 0 / max_RGB, 199 / max_RGB),
RGB(135 / max_RGB, 88 / max_RGB, 0 / max_RGB),
],
cur_colors,
)
else
use_set == 2
cur_colors = get_color_palette(:auto, 1)
cur_colors3 = get_color_palette(:lightrainbow, 1)
cur_colors2 = get_color_palette(:cyclic1, 1)
if min_dim == 0
# colors_set = [cur_colors[3], cur_colors[5], [:red], cur_colors[1]] #cur_colors[7],
colors_set = [cur_colors3[3], cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
else
colors_set = [cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
# colors_set = [cur_colors[5], [:red], cur_colors[1], cur_colors[14]]
end
# for c = [collect(11:25);]
# push!(colors_set, cur_colors2[c])
# end
colors_set = vcat(colors_set, [cur_colors2[c] for c in [collect(11:25);]])
end
return colors_set
end
# ==============================
# ======= Untested code =======
# using Measures
# using Plots.PlotMeasures
#
# # Source: https://github.com/JuliaPlots/Plots.jl/issues/897
# function setdefaultplottingparams(;upscale=2)
# #8x upscaling in resolution
# fntsm = Plots.font("sans-serif", pointsize=round(12.0*upscale))
# fntlg = Plots.font("sans-serif", pointsize=round(18.0*upscale))
# default(titlefont=fntlg, guidefont=fntlg, tickfont=fntsm, legendfont=fntsm)
# default(size=(800*upscale,600*upscale)) #Plot canvas size
# default(dpi=500) #Only for PyPlot - presently broken
# end
#%%
"""
plot_bettis_collection(bettis_collection, bett_num; step=1, show_plt=true, R=0., G=0.4, B=1.0)
PLots collection of Betti curves of rank 'bett-num'. Every successive plot has
lower opacity than predecessor. 'step' defines step between collection elements
that are ploted. By default, plot is displayed after carteation. This can be
disabled by setting 'show_plt' to false.
Color of the plot can be set with 'R', 'G', 'B' parameters.
"""
function plot_bettis_collection(bettis_collection,
bett_num,
max_rank;
step = 1,
show_plt = true,
R = 0.0,
G = 0.4,
B = 1.0)
step > 0 || error("Steps should be natural number!")
bettis_total = size(bettis_collection, 1)
colors_set = zeros(Float64, bettis_total, 4)
colors_set[:, 1] .= R
colors_set[:, 2] .= G
colors_set[:, 3] .= B
max_betti = get_max_betti_from_collection(bettis_collection)
@info "max_betti" max_betti
x = 0
y = bettis_total * 0.1
va_range = collect(range(bettis_total + x, y, length = bettis_total))
colors_set[:, 4] .= va_range / findmax(va_range)[1]
rgba_set = RGBA[]
for k = 1:size(colors_set, 1)
push!(
rgba_set,
RGBA(colors_set[k, 1], colors_set[k, 2], colors_set[k, 3], colors_set[k, 4]),
)
end
plt_reference = plot(1, title = "Betti curves collection, rank $(bett_num)", label = "")
for b = 1:step:bettis_total
betti = bettis_collection[b]
x_vals_1 = (1:size(betti[:, bett_num], 1)) / size(betti[:, bett_num], 1)
plot!(x_vals_1, betti[:, bett_num], lc = rgba_set[b], label = "rank=$(max_rank-b)")
plot!(ylim = (0, max_betti))
end
xlabel!("Normalised steps")
ylabel!("Number of cycles")
plot!(legend = true)
show_plt && display(plt_reference)
return plt_reference
end
#%%
"""
get_max_bettis(bettis)
Returns the maximal bettis of Betti curves for all dimensions.
"""
function get_max_bettis(bettis)
all_max_bettis = findmax(bettis, dims=1)[1]
return all_max_bettis
end
# TODO change name
# TODO check what for dim is used, change to min dim
function get_max_betti_from_collection(bettis_collection; dim = 1)
max_betti = 0
for betti in bettis_collection
# global max_betti
local_max = findmax(betti)[1]
if (local_max > max_betti)
max_betti = local_max
end
end
return max_betti
end
#%%
"""
plot_and_save_bettis(eirene_results, plot_title::String,
results_path::String; extension = ".png",
data_size::String="", do_save=true,
extend_title=true, do_normalise=true, max_dim=3,
legend_on=true)
Plot Betti curves from 0 up to `max_dim` using `eirene_results` from Eirene library and
returns handler for figure. Optionally, if `do_save` is set, saves the figure
or if `do_normalise` is set, sets the steps range to be normalised to the
horizontal axis maximal value.
"""
function plot_and_save_bettis(bettis,
plot_title::String,
results_path::String;
file_name = "",
extension = ".png",
do_save = true,
do_normalise = true,
min_dim = 0,
max_dim = 3,
legend_on = true,
kwargs...)
bettis = get_bettis(eirene_results, max_dim)
if do_normalise
bettis = normalise_bettis(bettis)
end
plot_ref =
plot_bettis(bettis, plot_title, legend_on = legend_on, min_dim = min_dim, kwargs...)
if do_save
if isempty(file_name)
file_name = plot_title * extension
elseif isempty(findall(x -> x == extension[2:end], split(file_name, ".")))
#check for the extension in file name
file_name *= extension
end
save_figure_with_params(
plot_ref,
results_path;
extension = extension,
prefix = split(file_name, ".")[1],
)
end
return plot_ref
end
# TODO merge functions for getting betti curves
# Original function returns 2 different types of betti curves. If no default
# value parameters is given, it returns vector of matrices. If num of steps is
# given, then it return matrix maxdim x numsteps.
# """
# bettis_eirene(matr, maxdim; mintime=-Inf, maxtime=Inf, numofsteps=Inf, mindim=1)
#
# Takes the `matr` and computes Betti curves up to `maxdim`. Return matrix only
# with betti curve values
#
#
# Function taken from: https://github.com/alexyarosh/hyperbolic
# """
#%%
@deprecate bettis_eirene(matr, maxdim; mintime = -Inf, maxtime = Inf, numofsteps = Inf, mindim = 1) get_bettis(results_eirene, max_dim; min_dim = 1)
@deprecate get_bettis_from_image(img_name, plot_params; file_path = "", plot_heatmaps = true, save_heatmaps = false, plot_betti_figrues = true) get_bettis(results_eirene, max_dim; min_dim = 1)
@deprecate get_bettis_from_image2(img_name;file_path = "",plot_heatmaps = true, save_heatmaps = false, plot_betti_figrues = true) get_bettis_from_image(img_name, plot_params; file_path = "", plot_heatmaps = true, save_heatmaps = false, plot_betti_figrues = true)
@deprecate plot_and_save_bettis2(eirene_results, plot_title::String, results_path::String; file_name = "", extension = ".png", data_size::String = "", do_save = true, extend_title = true, do_normalise = true, min_dim = 0, max_dim = 3, legend_on = true) plot_and_save_bettis(bettis, plot_title::String, results_path::String; file_name = "", extension = ".png", do_save = true, do_normalise = true, min_dim = 0, max_dim = 3, legend_on = true, kwargs...)
@deprecate get_and_plot_bettis(eirene_results; max_dim = 3, min_dim = 1, plot_title = "", legend_on = false) get_bettis(results_eirene, max_dim; min_dim = 1)
#%%
"""
lower_ordmat_resolution(ordered_matrix::Array, total_bins::Int)
Takes ordered matrix 'input_matrix' and reduces the resolution of values in the
matrix into 'total_bins' bins.
"""
function lower_ordmat_resolution(ordered_matrix::Array, total_bins::Int)
new_ordered_matrix = zeros(size(ordered_matrix))
max_val = findmax(ordered_matrix)[1]
min_val = findmin(ordered_matrix)[1]
bin_step = max_val ÷ total_bins
old_bins = min_val:bin_step:max_val
for bin = 1:total_bins
@debug "First step threshold is $(old_bins[bin])"
indices = findall(x -> (x >= old_bins[bin]), ordered_matrix)
new_ordered_matrix[indices] .= bin - 1
end
@debug "Max_val in new matrix is " findmax(new_ordered_matrix)
@debug "And should be " total_bins - 1
return new_ordered_matrix
end
#%%
"""
average_bettis(bettis_matrix; up_factor=8)
Takes the average values of betti curves stored in 'bettis_matrix'.
'bettis_matrix' consist of different simulations(first index of the matrix),
different ranks (third index of the matrix). Second index of the matrices
(saples) may vary accross many simulations and for this reason, all betti curves
are upsampled by a factor of 'upsample_factor' and then the average for every
dimension is computed.
"""
function average_bettis(bettis_matrix::Matrix; up_factor = 8)
bettis_matrix_backup = copy(bettis_matrix)
simulations = size(bettis_matrix, 1)
dimensions = size(bettis_matrix[1], 1)
max_samples = 0
for k = 1:simulations
# global max_samples
current_len = length(bettis_matrix[k][1][:, 1])
if max_samples < current_len
max_samples = current_len
end
end
bettis_size = size(bettis_matrix)
total_upsamples = (max_samples - 1) * up_factor + 1
x_resampled = range(0, 1, step = total_upsamples)
avg_bettis = zeros(total_upsamples, dimensions)
std_bettis = copy(avg_bettis)
resampled_bettis = zeros(simulations, total_upsamples, dimensions)
# resample betti curves
for simulation = 1:simulations, betti = 1:dimensions
resampled_bettis[simulation, :, betti] =
upsample_vector2(bettis_matrix[simulation][betti][:, 2], total_upsamples)
end
# average and std Betti
for dimension = 1:dimensions
avg_bettis[:, dimension] = mean(resampled_bettis[:, :, dimension], dims = 1)
std_bettis[:, dimension] = mean(resampled_bettis[:, :, dimension], dims = 1)
end
return avg_bettis, std_bettis
end
#%%
function upsample_vector2(input_vector, total_upsamples)
total_orig_samples = size(input_vector, 1) - 1
x_vals = range(0, 1, length = total_orig_samples + 1)
spl = Spline1D(x_vals, input_vector)
x_upsampled = range(0, 1, length = total_upsamples)
y_upsampled = spl(x_upsampled)
# ref = plot(range(0, 1, length=total_orig_samples), input_vector);
# plot!(x_vals, y_upsampled);
# display(ref)
return y_upsampled
end
#%%
"""
upsample_vector(input_vector; upsample_factor::Int=8)
Takes an 'input_vector' and returns a vector which has 'upsample_factor' many
times more samples. New samples are interpolated with 'spl' function from
'Dierckx' package.
"""
function upsample_vector(input_vector; upsample_factor::Int = 8)
total_orig_samples = size(input_vector, 1) - 1
total_samples = upsample_factor * total_orig_samples + 1
x_vals = range(0, 1, length = total_orig_samples + 1)
spl = Spline1D(x_vals, input_vector)
x_upsampled = range(0, 1, length = total_samples)
y_upsampled = spl(x_upsampled)
# ref = plot(range(0, 1, length=total_orig_samples), input_vector);
# plot!(x_vals, y_upsampled);
# display(ref)
return y_upsampled
end
# =========--=======-========-==========-=======-
# From bettis areas
# Area under Betti curve functions
#%%
"""
get_area_under_betti_curve(betti_curves, min_dim, max_dim)
Computes the area under Betti curves stored in 'betti_curves', where each row is
a Betti curve and each column is a value.
"""
function get_area_under_betti_curve(betti_curves::Union{Matrix{Float64}, Array{Array{Float64,2}}};do_normalised::Bool=false)
#TODO check this part
if size(betti_curves,2) < 2
bettis_vector = vectorize_bettis(betti_curves)
else
bettis_vector = betti_curves
end
# @info sum(bettis_vector, dims=1)
bettis_area = sum(bettis_vector, dims=1)
if do_normalised
total_steps = size(bettis_vector,1)
bettis_area ./= total_steps
end
# @info bettis_area
return bettis_area
end
@deprecate get_area_under_betti_curve(C, min_dim, max_dim) get_area_under_betti_curve(betti_curves; do_normalised=false)
#%%
"""
get_dataset_bettis_areas(dataset; min_dim::Integer=1, max_dim::Integer=3, return_matrix::Bool=true)
Computes topology of every matrix in dataset, computes Betti curves for dimensions
min_dim up to max_dim and returns vector (or matrix) of areas under Betti curves.
"""
function get_dataset_bettis_areas(dataset; min_dim::Integer=1, max_dim::Integer=3, return_matrix::Bool=true)
areas_vector = Array[]
for data = dataset
@info "Computing topology."
C = eirene(data, maxdim=max_dim,)
matrix_bettis = get_bettis(C,max_dim, min_dim=min_dim)
push!(areas_vector, get_area_under_betti_curve(matrix_bettis))
end
if return_matrix
return vcat([areas_vector[k] for k=1:10]...)
else
return areas_vector
end
end
#%%
"""
get_area_boxes(areas_matrix, min_dim::Integer, max_dim::Integer)
Plots the boxplot of area under betti curves.
"""
function get_area_boxes(areas_matrix, min_dim::Integer, max_dim::Integer)
bplot = StatsPlots.boxplot()
data_colors = get_bettis_color_palete()
for (index, value) in enumerate(min_dim:max_dim)
StatsPlots.boxplot!(bplot, areas_matrix[:,index], labels="β$(value)", color=data_colors[value])
end
return bplot
end
# function get_bettis_collection_from_matrices(ordered_matrices_collection; max_dim::Int=3, min_dim::Int=1)
# bettis_collection = Array[]
#
# for matrix = ordered_matrices_collection
# @debug "Computing Bettis..."
# eirene_geom = eirene(matrix,maxdim=max_B_dim,model="vr")
#
# bettis = reshape_bettis(get_bettis(eirene_geom, max_B_dim))
# push!(bettis_collection, bettis)
# end
#
# return bettis_collection
# end
#
# #%%
# TODO find what are the alternative functions for the functions below
# @deprecate get_dataset_topology(dataset; min_dim::Integer=1, max_dim::Integer=3, get_curves::Bool=true, get_areas::Bool=true, get_persistence_diagrams::Bool=true, do_normalise::Bool=true)
# @deprecate get_bettis_collection(ordered_matrices_collection; max_B_dim=3)
# @deprecate reshape_bettis(bettis)
# @deprecate print_hmap_with_bettis(ordered_matrices_collection, bettis_collection, plot_data::PlottingData)
# @deprecate make_hm_and_betti_plot(ordered_geom_gr, bettis, title_hmap, title_bettis, max_betti)
# @deprecate matrix_analysis(test_data::PlottingData;generation_function=get_geom_matrix)
# @deprecate multiscale_matrix_testing(sample_space_dims = 3, maxsim = 5, min_B_dim = 1, max_B_dim = 3, size_start = 10, size_step = 5, size_stop = 50; do_random = true, control_saving = false, perform_eavl = false)
# @deprecate plot_betti_numbers(betti_numbers, edge_density, title="Geometric matrix"; stop=0.6)
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 23592 | using LinearAlgebra
import Plots.plot as plot
# using Plots
using Random
include("PlottingWrappers.jl")
include("PointsSubstitution.jl")
"""
function expand_matrix(input_matrix, expansion_size, last_components
Takes 'input_matrix' (an ordering matrix used for creating cliques) and and adds
2×'expansion_size' number of rows. 'last_components' are the values in original
matrix that are added last to the clique.
Results may be be plotted with same funtion with sufix "with_plot".
"""
function expand_matrix(input_matrix, expansion_size, last_components)
new_comp = last_components
matrix_size = size(input_matrix, 1)
for mat_sizes = matrix_size:2:(matrix_size+2expansion_size)
input_matrix, new_comp = add_step_to_matrix(input_matrix, new_comp)
end
return input_matrix
end
function expand_matrix_with_plot(args...)
input_matrix = expand_matrix_with_plot(args...)
expand_plt_ref = plot_square_heatmap(input_matrix, 1, size(input_matrix, 1);
plt_title="Original, size:$(matrix_size)",
color_palete=:lightrainbow)
display(expand_plt_ref)
return input_matrix, expand_plt_ref
end
# Shuffle matrix entries
"""
function shuffle_matrix(input_matrix, shuffles; do_plot=false)
Takes symmetric 'input_matrix' and randomly swaps rows 'shuffles' many times.
Results may be plotted by setting 'do_plot=true'.
"""
function shuffle_matrix(input_matrix, total_shuffles)
matrix_size = size(input_matrix, 1)
rows = randcycle(matrix_size)
shuffled_ord_mat = copy(input_matrix)
for k = 1:total_shuffles
# global shuffled_ord_mat, rows
srcs, trgts = rand(rows, 2)
swap_rows!(shuffled_ord_mat, srcs, trgts)
end
return shuffled_ord_mat
end
function shuffle_matrix_with_plotting(args...)
shuffled_ord_mat = shuffle_matrix(args...)
if do_plot
shuff_plt_ref = plot_square_heatmap(shuffled_ord_mat, 1, size(shuffled_ord_mat, 1);
plt_title="Shuffled, size:$(matrix_size)",
color_palete=:lightrainbow)
display(shuff_plt_ref)
end
return input_matrix, shuff_plt_ref
end
"""
function organize_shuff_matrix(input_matrix; do_plots=false)
Reorganizes 'input_matrix' so that values highest values in a row are positioned
next to the diagonal.
Results may be plotted by setting 'do_plot=true'.
"""
function organize_shuff_matrix(input_matrix)
unscrambled_matrix = copy(input_matrix)
matrix_size = size(input_matrix, 1)
for k = matrix_size:-2:2
max_row_val = findmax(unscrambled_matrix[k, :])[2]
# put to the previous last position
swap_rows!(unscrambled_matrix, max_row_val, k - 1)
# skip 1 row and work on next one
end
return unscrambled_matrix
end
function organize_shuff_matrix_with_plotting(input_matrix)
unscrambled_matrix = organize_shuff_matrix(input_matrix)
reorganized_plt_ref = plot_square_heatmap(unscrambled_matrix, 1, size(unscrambled_matrix, 1);
plt_title="unscrambled_matrix, size:$(matrix_size)",
color_palete=:lightrainbow
)
display(reorganized_plt_ref)
return unscrambled_matrix, reorganized_plt_ref
end
"""
function order_max_vals_near_diagonal(input_matrix; do_plots=false, direction=:descending)
Orders values in 'input_matrix' so that values next to diagonal are descending
(by default).
TODO- not working- Optionally, ascending order can be used by setting 'direction' to
':ascending'.
Results may be plotted by setting 'do_plot=true'.
"""
function order_max_vals_near_diagonal(input_matrix; direction=:descending)
# Find max values next to the diagonal
matrix_size = size(input_matrix, 1)
if direction == :descending
# ordering_function = findmax
new_ord_value = -1
iteration_values = matrix_size:-2:2
# iteration_values = 2:2:matrix_size
elseif direction == :ascending
# ordering_function = findmin
new_ord_value = findmax(input_matrix)[1] * 2
iteration_values = 2:2:matrix_size
else
# @error "Unknow ordering was given"
throw("Unknow ordering was given")
end
reordered_matrix = copy(input_matrix)
row_indices = 1:2:matrix_size
col_indices = 2:2:matrix_size
coord_set = [CartesianIndex(row_indices[k], col_indices[k]) for k = 1:matrix_size÷2]
diag_max_values = reordered_matrix[coord_set]
for k = iteration_values
max_val, max_ind = findmax(diag_max_values)
(direction == :descending) ? (position = floor(k ÷ 2)) : (position = floor(k ÷ 2))
diag_max_values[max_ind] = diag_max_values[position]
diag_max_values[position] = new_ord_value
max_ind *= 2
swap_rows!(reordered_matrix, k, max_ind)
swap_rows!(reordered_matrix, k - 1, max_ind - 1)
end
return reordered_matrix
end
function order_max_vals_near_diagonal_with_plotting(input_matrix; kwargs...)
reordered_matrix = order_max_vals_near_diagonal(input_matrix; kwargs...)
reorganized_plt_ref = plot_square_heatmap(reordered_matrix, 1, size(reordered_matrix, 1);
plt_title="reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
display(reorganized_plt_ref)
return reordered_matrix, reorganized_plt_ref
end
"""
function fine_tune_matrix(input_matrix; do_plots=false)
Check if velues next to the maximal values are organized in descending order.
"""
function fine_tune_matrix(input_matrix)
# Find max values next to the diagonal
matrix_size = size(input_matrix, 1)
fine_tune_matrix = copy(input_matrix)
# if direction == :descending
# # ordering_function = findmax
# new_ord_value = -1
# iteration_values = matrix_size:-2:2
# # iteration_values = 2:2:matrix_size
#
# elseif direction == :ascending
# # ordering_function = findmin
# new_ord_value = findmax(input_matrix)[1]*2
# iteration_values = 2:2:matrix_size
# else
# # @error "Unknow ordering was given"
# throw("Unknow ordering was given")
# end
for k = 2:2:matrix_size-1
if fine_tune_matrix[k-1, k+1] > fine_tune_matrix[k, k+1]
swap_rows!(fine_tune_matrix, k, k - 1)
end
end
return fine_tune_matrix
end
function fine_tune_matrix_with_ploting(input_matrix)
fine_tune_matrix = fine_tune_matrix(input_matrix)
fine_tuned_plt_ref = plot_square_heatmap(fine_tune_matrix, 1, size(reordered_matrix, 1);
plt_title="fine_tuned, size:$(matrix_size)",
color_palete=:lightrainbow)
display(fine_tuned_plt_ref)
return fine_tune_matrix, fine_tuned_plt_ref
end
# TODO separate plotting from processing
function order_max_vals_by_row_avg(input_matrix; do_plots=false)
# Find max values next to the diagonal
matrix_size = size(input_matrix,1)
# Row average
row_avg = reshape(mean(input_matrix, dims=1),(matrix_size, 1))
# Using funciton below, because sortperm is not working on Array{Float64,2}
sorted_rows_indexes = [findall(x->x==sort(row_avg, dims=1)[k], row_avg)[1][1] for k=1:matrix_size]
matrix_indices = collect(range(1,matrix_size))
# Sort indices by values (highest to lowest)
# Create a list of indices, which corresponding valeus are ordered
sorted_indices = sort!([1:matrix_size;],
by=i->(sorted_rows_indexes[i],matrix_indices[i]))
sorted_matrix = copy(input_matrix)
for k = 1:matrix_size÷2 #iteration_values
max_ind = sorted_indices[k]
sorted_indices[k] = k
sorted_indices[max_ind] = max_ind
swap_rows!(sorted_matrix, k, max_ind)
# swap_rows!(sorted_matrix, k-1, max_ind-1)
end
# TODO separate plotting from processing
reorganized_plt_ref = plot_square_heatmap(sorted_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
# TODO separate plotting from processing
input_mat_plt_ref = plot_square_heatmap(input_matrix, 1,size(reordered_matrix,1);
plt_title = "input_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
common_plot1 = plot(input_mat_plt_ref, reorganized_plt_ref, layout=(1,2),
size=(800,400))
# reordered_matrix = copy(input_matrix)
# row_indices = 1:2:matrix_size
# col_indices = 2:2:matrix_size
# coord_set = [CartesianIndex(row_indices[k], col_indices[k]) for k=1:matrix_size÷2]
#
#
# for k = iteration_values
# max_val, max_ind = findmax(diag_max_values)
# position = floor(k÷2)
# diag_max_values[max_ind] = diag_max_values[position]
# diag_max_values[position] = new_ord_value
# max_ind *= 2
#
# swap_rows!(reordered_matrix, k, max_ind)
# swap_rows!(reordered_matrix, k-1, max_ind-1)
# end
if do_plots
# TODO separate plotting from processing
reorganized_plt_ref = plot_square_heatmap(sorted_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
display(reorganized_plt_ref)
end
return reordered_matrix, reorganized_plt_ref
end
function order_max_vals_near_diagonal2(input_matrix; do_final_plot=false, do_all_plots = false, direction=:descending)
# Find max values next to the diagonal
matrix_size = size(input_matrix,1)
reordered_matrix = copy(input_matrix)
reorganized_plt_ref = []
# for every row in matrix
for k = 1:2:matrix_size-1
# global reordered_matrix
# TODO separate plotting from processing
reorganized_plt_ref_pt0 = plot_square_heatmap(reordered_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
max_val, max_ind = findmax(reordered_matrix[k:end, k:end])
# Take the smaller coordinate
# (max_ind[1] < max_ind[2]) ? (target_row = max_ind[1]) : (target_row = max_ind[2])
target_row = max_ind[1]+k-1
reordered_matrix = swap_rows(reordered_matrix, k, target_row)
# TODO separate plotting from processing
reorganized_plt_ref_pt1 = plot_square_heatmap(reordered_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
val, second_target = findmax(reordered_matrix[k,k:end])
second_target = second_target+k-1
reordered_matrix = swap_rows(reordered_matrix, k+1, second_target)
# end
#
#
if do_all_plots
# TODO separate plotting from processing
reorganized_plt_ref_pt2 = plot_square_heatmap(reordered_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
reorganized_plt_ref = plot(reorganized_plt_ref_pt0, reorganized_plt_ref_pt1, reorganized_plt_ref_pt2, layout=(1,3), size=(1400,400))
display(reorganized_plt_ref)
end
end
if do_final_plot
# TODO separate plotting from processing
reorganized_plt_ref = plot_square_heatmap(reordered_matrix, 1,size(reordered_matrix,1);
plt_title = "reordered_matrix, size:$(matrix_size)",
color_palete=:lightrainbow)
# display(reorganized_plt_ref)
else
reorganized_plt_ref=[]
end
return reordered_matrix, reorganized_plt_ref
end
"""
function get_key_for_value(d::Dict, target_value)
Returns key of the dictionary which corresponds to the given target value.
"""
function get_key_for_value(d::Dict, target_value)
for (key, value) in d
if value == target_value
return key
end
end
end
function order_max_vals_near_diagonal3(input_matrix, ordering; direction=:descending)
# Find max values next to the diagonal
matrix_size = size(input_matrix,1)
reordered_matrix = deepcopy(input_matrix)
new_ordering = Dict()
# for every row in matrix
for m = 1:2:matrix_size-1
# global reordered_matrix
max_val, max_ind = findmax(reordered_matrix[m:end, m:end])
# Take the smaller coordinate
first_target = max_ind[1]+m-1
reordered_matrix = swap_rows(reordered_matrix, m, first_target)
# check for duplicates
val, second_target = findmax(reordered_matrix[m,m:end])
second_target = second_target+m-1
if first_target == second_target
@debug "are same"
second_target -= 1
end
reordered_matrix = swap_rows(reordered_matrix, m+1, second_target)
# find key which initially had region = first_target
region1 = get_key_for_value(ordering, first_target)
region2 = get_key_for_value(ordering, second_target)
if region1 in keys(new_ordering)
@warn "repeated"
end
if region2 in keys(new_ordering)
@warn "repeated2"
end
new_ordering[region1] = m
new_ordering[region2] = m +1
println("Replaced $(region1) fom $(ordering[region1]) to $(m)")
println("Replaced $(region2) fom $(ordering[region2]) to $(m+1)")
end
return reordered_matrix, new_ordering
end
##
# """
# matrix_poling!(input_matrix; method = "avg_pooling")
#
# Takes a matrix and changes it's values to the same value, according to 'method'.
# Possible methods are:
# - 'max_pooling'- finds maximal value and replaces all values with the maximal
# value.
# - 'avg_pooling'- changes values to the average value
# - 'gauss_pooling'- uses gausian kernel as weights to the values in the matrix
# """
# function matrix_poling!(input_matrix::Array; method::String = "max_pooling")
# if method == "max_pooling"
# max_val = findmax(input_matrix)[1]
# input_matrix .= max_val
# end
# return input_matrix
# end
# matrix_poling(mat[1:3,1:3]; method = "gauss_pooling")
function matrix_poling(input_matrix::Array; method = "avg_pooling", kernel_size=3,gauss_sigma=1)
out_matrix = copy(input_matrix)
if method == "max_pooling"
max_val = findmax(out_matrix)[1]
out_matrix .= max_val
elseif method == "avg_pooling"
avg_val = mean(out_matrix)
out_matrix .= floor(Int,avg_val)
elseif method == "gauss_pooling"
@debug "Gauss pooling"
# gauss_kernel = ones(kernel_size,kernel_size)
# gauss_kernel[kernel_size÷2+1,kernel_size÷2+1] = 2
filtering_kernel = Kernel.gaussian(gauss_sigma)
# gauss_kernel2 = imfilter(gauss_kernel, filtering_kernel)
# gauss_kernel3 = gauss_kernel2.-findmin(gauss_kernel2)[1]/findmax(gauss_kernel2)[1]
# gauss_kernel3 = gauss_kernel3./sum(gauss_kernel3)
out_matrix = imfilter(out_matrix, filtering_kernel)
# out_matrix += out_matrix.*gauss_kernel3
out_matrix .= floor.(Int,out_matrix)
end
return out_matrix
end
function subsample_matrix(square_matrix::Array; subsamp_size::Int=2, method="max_pooling")
if !issymmetric(square_matrix)
error("Input matrix is not square")
return
end
if subsamp_size == 2
reorganize_matrix(square_matrix; subsamp_size=subsamp_size, method=method)
return reorganize_matrix(square_matrix; subsamp_size=subsamp_size, method=method)
elseif subsamp_size%2 == 0
new_matrix = reorganize_matrix(square_matrix; subsamp_size=subsamp_size, method=method)
return reorganize_matrix(new_matrix; subsamp_size=2, method=method)
else
new_matrix = reorganize_matrix(square_matrix; subsamp_size=subsamp_size, method=method)
return new_matrix
end
end
#= Should the result be overlapping or not? Options:
- filter it as a whole image, return diagonal
- filter subimages- the problem is that htere will be edge effect at every border of cells
- filter subimages and assign midde value to whole patch
- filter whole upper diagonal matrix
Plot gaussian kernel
Should we care about
=#
function reorganize_matrix(square_matrix::Array; subsamp_size::Int=2, method="max_pooling", overlap::Int=0,gauss_sigma=1)
if method == "gauss_pooling"
(subsamp_size >= 3) || error("Can not do gaussian pooling for area smaller than 3x3")
end
@debug method
# Subsample upper half
square_matrix2 = Float64.(copy(square_matrix))
total_rows, total_cols = size(square_matrix)
size_mismatch_flag = false
# if total_rows%2 != 0
# total_rows -= 1
# end
# if total_cols%2 != 0
# total_cols -= 1
# end
if method == "gauss_pooling"
square_matrix2 = zeros(Int,size(square_matrix))
square_matrix2[1:end-1,2:end] = UpperTriangular(square_matrix[1:end-1,2:end])
# flip matrix
do_matrix_flip = true
if do_matrix_flip
square_matrix3 = zeros(Float64,size(square_matrix))
for row in 0:total_rows-1
for col in 0:total_cols-1
square_matrix3[row+1,col+1] = square_matrix[end-row,end-col]
end
end
square_matrix3[1:end-1,:] = square_matrix3[2:end,:]
square_matrix3[:,2:end] = square_matrix3[:,1:end-1]
else
square_matrix3 = copy(square_matrix2)
square_matrix3[1:end-1,:] = square_matrix2[2:end,:]
square_matrix3[:,1:end-1] = square_matrix2[:,2:end]
end
for row in 1:total_rows
for col in 1:row
square_matrix2[row,col] = square_matrix3[row,col]
end
end
filtering_kernel = Kernel.gaussian(gauss_sigma)
square_matrix2 = imfilter(square_matrix2, filtering_kernel)
square_matrix2 .= Int.(floor.(Int,square_matrix2))
elseif method == "row_pooling"
function gauss_func(σ,len;μ=0)
maxv = len÷2
minv= -len÷2
if len%2 == 0
maxv-=1
end
x = collect(minv:1:maxv)
return exp.(-(((x.-μ)./σ)./2).^2)./(σ*sqrt(2π))
end
# Take 'subsamp_size'in total in horizontal and in vertical line from
# current matrix element
# subsamp_size = 5
val_range = subsamp_size÷2
r = (subsamp_size÷2)*2
# total_rows = 266
# total_cols = 266
# row = 3
for row = 1:1:(total_rows-1)
for col = (row+1):1:total_cols
if row < r && col <= r
row_range = row - 1
col_range = val_range + (val_range-row_range÷2)
else
row_range = val_range
end
if row > total_rows-r && col >= total_cols-r
col_range = total_cols - row -1
row_range = val_range + (val_range-col_range)
else
col_range = val_range
end
r_beg = row - row_range
r_end = row + row_range
c_beg = col - col_range
c_end = col + col_range
# if r_beg < 1 && r_end > total_rows
if r_beg < 1
r_end += abs(r_beg)+1
r_beg = 1
end
if r_end > col
r_beg -= abs(r_end-col)
if r_beg <1
r_beg=1
end
r_end = col-1
end
# end # if both
# if c_beg < row+1 && c_end > total_cols
if c_beg < row+1
c_end += abs(c_beg-(row+1))
c_beg = row+1
end
if c_end > total_cols
c_beg -= abs(total_rows-c_end)
c_end = total_cols
end
vrange = r_beg:r_end
try
square_matrix2[row,col] += sum(
square_matrix[vrange,col]
.* gauss_func(gauss_sigma,length(vrange))
)
vrange = c_beg:c_end
square_matrix2[row,col] += sum(
square_matrix[row,c_beg:c_end] .*
gauss_func(gauss_sigma,length(vrange))
)
catch e
@error "Failed to compute row pooling"
@error "row" row
@error "col" col
square_matrix2[row,col] = 0
break
# error(e)
end
end # for col
end # for rows
else
step = subsamp_size-overlap
for row = 1:step:(total_rows-2)
for col = (row+subsamp_size):step:total_cols
r_beg = row
r_end = row+subsamp_size-1
c_beg = col
c_end = col+subsamp_size-1
if r_end > total_rows || c_end > total_cols
size_mismatch_flag = true
continue
end
square_matrix2[r_beg:r_end,c_beg:c_end] =
matrix_poling(square_matrix[r_beg:r_end,c_beg:c_end]; method=method,kernel_size=subsamp_size, gauss_sigma=gauss_sigma)
end # for col
size_mismatch_flag && continue
end # for rows
end # if method
# Copy over lower half
for row in 2:total_rows
for col in 1:row-1
square_matrix2[row,col] = square_matrix2[col,row]
end
end
# keep same values on diagonal
for row in 1:total_rows
square_matrix2[row,row] = square_matrix[row,row]
end
return square_matrix2
end
function pool_matrix(square_matrix::Array; method="max_pooling")
out_matrix = copy(square_matrix)
pool_matrix!(square_matrix; method=method)
return out_matrix
end
"""
add_random_patch(input_matrix; patch_size=1, total_patches=1, locations)
Takes a matrix and replaces some values with random values. Returns a new matrix
with replaced values and indicies where replacement took place.
Values can be
replaced by setting 'patch_size' to values bigger than 1. If the input matrix
is symmetric, then output matrix will be symmetric as well (values from above
diagnoal will be copied over values from below diagonal).
"""
function add_random_patch(input_matrix::Matrix; patch_size=1, total_patches=1, locations=CartesianIndex(0))
total_rows, total_cols = size(input_matrix)
max_row = total_rows-patch_size+1
max_col = total_cols-patch_size+1
output_matrix = copy(input_matrix)
max_val = findmax(output_matrix)[1]
min_val = findmin(output_matrix)[1]
matrix_type = typeof(output_matrix[1])
if patch_size>total_rows || patch_size>total_cols
error(DimensionMismatch,": Patch size is bigger than the matrix!")
end
# ===
issymmetric(input_matrix) ? (symmetrize_matrix = true) : (symmetrize_matrix = false)
if locations == CartesianIndex(0)
@debug "Locations were not specified- random locations will be used"
if symmetrize_matrix
possible_indices = findall(x->true,UpperTriangular(output_matrix))
possible_indices = possible_indices[findall(x->x[1]<=x[2], possible_indices)]
possible_indices = possible_indices[findall(x->x[1]<=max_row, possible_indices)]
possible_indices = possible_indices[findall(x->x[2]<=max_col, possible_indices)]
else
possible_indices = possible_indices = findall(x->true,output_matrix)
end
tartget_indices = possible_indices[randcycle(length(possible_indices))]
else
wrong_indices = findall(x->x[1]>max_row || x[2]>max_col, locations)
if isempty(wrong_indices)
tartget_indices = locations
total_patches = size(locations)[1]
else
error(DimensionMismatch,": Given indices are bigger than the matrix dimensions!")
end
end
changed_indices = CartesianIndex[]
for replacement=1:total_patches
row = tartget_indices[replacement][1]
col = tartget_indices[replacement][2]
r_range = row:row+patch_size-1
c_range = col:col+patch_size-1
for ind in CartesianIndices((r_range,c_range))
push!(changed_indices,ind)
end
new_rand_matrix = floor.(matrix_type, rand(patch_size,patch_size) .* (max_val-min_val+1) .+ min_val)
output_matrix[r_range,c_range] .= new_rand_matrix
end
if symmetrize_matrix
# Inverse second column
changed_indices2 = [changed_indices changed_indices]
for ind = 1:size(changed_indices)[1]
c_ind = changed_indices2[ind,2]
changed_indices2[ind,2] = CartesianIndex(c_ind[2],c_ind[1])
end
# Copy over lower half
for row in 2:total_rows
for col in 1:row-1
output_matrix[row,col] = output_matrix[col,row]
end
end
@debug "Returned symmetric matrix" output_matrix
return output_matrix, changed_indices2
else
return output_matrix, changed_indices
end
end
function scramble_matrix(in_matrix::Array; k::Int=2, max_iterations=-1)
out_matrix = copy(in_matrix)
total_rows, total_cols = size(in_matrix)
counter = 0
if max_iterations < 1
max_iterations = (total_cols*(total_cols-1))/2
end
for row = 1:k:total_rows-k
# @info "row:" row
for col=total_cols:-k:row+1
# @info "col:" col
if row == col-k+1
# @info "shoulbreak"
continue
end
indices = collect(CartesianIndices((row:row+k-1,col-k+1:col)))
permut_indices = shuffle(indices)
out_matrix[indices] .= in_matrix[permut_indices]
counter +=1
if counter >= max_iterations
break
end
end
if counter >= max_iterations
break
end
end
return out_matrix
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 15396 | using LinearAlgebra
using StatsBase
"""
shift_to_non_negative(matrix::Array)
Returns a matrix in which values are non-negative. This is done by finding the
minimal value in the input matrix and adding its absolute value to the matrix
elements.
"""
function shift_to_non_negative(matrix::Array)
min_val = findmin(matrix)[1]
if min_val < 0
return matrix .-= min_val
else
return matrix
end
end
"""
normalize_to_01(matrix::Array; use_factor=false, norm_factor=256)
Returns a matrix which values are in range [0, 1]. If 'use_factor' is set to
'true' then values are normalized to 'norm_factor' (by default set to 256).
If the values in the input matrix are below 0, then they are shifted so that only positive numbers are in
the matrix (the values are normalized to new maximal value or norm_factor).
"""
function normalize_to_01(matrix::Array; use_factor = false, norm_factor = 256)
normalized_matrix = copy(matrix)
min_val = findmin(normalized_matrix)[1]
if min_val < 0
normalized_matrix .+= abs(min_val)
else
normalized_matrix .-= abs(min_val)
end
max_val = findmax(normalized_matrix)[1]
if use_factor
if max_val > norm_factor
@warn "Maximal values exceed \'norm_factor\'."
end
normalized_matrix = normalized_matrix ./ norm_factor
else
normalized_matrix = normalized_matrix ./ max_val
end
return normalized_matrix
end
# function symmetrize_image(image)
"""
function diagonal_symmetrize(image::Matrix; below_over_upper::Bool=false)
Takes an 'image' in the form of a matrix and return a copy which is symmetric
with respect to diagonal- values above diagonal are copied over values below the
diagonal. This can be inverted by setting 'below_over_upper=true'.
If the input matrix is not square, then square matrix is created by taking
matrix of k times 'k' elements, 'k=min(r,c)' where 'r' is number of rows and 'c'
is number of columns.
"""
function diagonal_symmetrize(image::Matrix; below_over_upper::Bool = false)
w, h = size(image)
mat_size = findmin([w, h])[1]
img = copy(image[1:mat_size, 1:mat_size])
# Get all cartesian indices from input matrix
matrix_indices = CartesianIndices((1:mat_size, 1:mat_size))
# Filter out indices below diagonal
if below_over_upper
matrix_indices = findall(x -> x[1] > x[2], matrix_indices)
else
matrix_indices = findall(x -> x[2] > x[1], matrix_indices)
end
# how many elements are above diagonal
repetition_number = Int(ceil((mat_size * (mat_size - 1)) / 2))
# Substitute elements
for k = 1:repetition_number
# n_pos = matrix_indices[k]
mat_ind = matrix_indices[k]
# ordered_matrix[mat_ind] = k
img[mat_ind[2], mat_ind[1]] = img[mat_ind]
end
try
checksquare(img)
catch err
if isa(err, DimensionMismatch)
@error "Resulting matrix is not a square matrix"
throw(err)
end
end
# issymmetric(Float64.(img))
return img
end
# =====
# matrix ordering
"""
function get_ordered_matrix(in_matrix::Matrix;
assign_same_values::Bool = false,
force_symmetry::Bool = false,
small_dist_grouping::Bool = false,
min_dist::Number = 1e-16,
total_dist_groups::Int = 0,
ordering_start::Int=1)
Takes a @input_matrix and returns ordered form of this matrix.
The ordered form is a matrix which elements represent ordering from smallest to
highest values in @input_matrix.
If @input_matrix is symmetric, then ordering happens only with upper diagonal.
Lower diagonal is symetrically copied from values above diagonal.
By default, if there is a geoup of entriess with the same value, they all are
assigned with the same ordering number. This can be changed with
@assign_same_values parameter.
Symetry ordering can be froced with @force_symmetry parameter.
By setting 'small_dist_grouping' to true, all the values that difference is
lower than 'min_dist', will be assigned with the same order number.
# Examples
```julia-repl
julia> a = [0 11 12;
11 0 13;
12 13 0];
julia> get_ordered_matrix(a)
3×3 Array{Int64,2}:
0 1 2
1 0 3
2 3 0
```
```julia-repl
julia> b = [38 37 36 30;
37 34 30 32;
36 30 31 30;
30 32 30 29]
julia> get_ordered_matrix(b; assign_same_values=false)
4×4 Array{Int64,2}:
0 6 5 2
6 0 1 4
5 1 0 3
2 4 3 0
julia> get_ordered_matrix(b; assign_same_values=true)
4×4 Array{Int64,2}:
0 4 3 1
4 0 1 2
3 1 0 1
1 2 1 0
```
"""
function get_ordered_matrix(in_matrix::Matrix;
assign_same_values::Bool = false,
force_symmetry::Bool = false,
small_dist_grouping::Bool = false,
min_dist::Number = 1e-16,
total_dist_groups::Int = 0,
ordering_start::Int=1)
# TODO Symmetry must be forced for matrix in which there are NaN elements- needs
# to be further investigated
# TODO not working for negative only values
# TODO check for square matrix
# ==
mat_size = size(in_matrix)
ord_mat = zeros(Int, mat_size)
# how many elements are above diagonal
if issymmetric(in_matrix) || force_symmetry
matrix_indices =
generate_indices(mat_size, symmetry_order = true, include_diagonal = false)
do_symmetry = true
else
matrix_indices = generate_indices(mat_size, symmetry_order = false)
do_symmetry = false
end
total_elements = length(matrix_indices)
# Collect vector of indices
all_ind_collected = arr_to_vec(matrix_indices)
# Sort indices vector according to inpu array
# TODO Cant this be done with sortperm? in_matrix > UpperTriangular |> sortperm
index_sorting = sort_indices_by_values(in_matrix, all_ind_collected)
ordering_number = ordering_start
for k = 1:total_elements
# global ordering_number
next_sorted_pos = index_sorting[k]
mat_ind = matrix_indices[next_sorted_pos]
if assign_same_values && k != 1
prev_sorted_pos = index_sorting[k-1]
prev_mat_ind = matrix_indices[prev_sorted_pos]
cond1 = in_matrix[prev_mat_ind] == in_matrix[mat_ind]
cond2 = small_dist_grouping
cond3 = abs(in_matrix[prev_mat_ind] - in_matrix[mat_ind]) < min_dist
if cond1 || (cond2 && cond3)
ordering_number -= 1
end
end
set_values!(ord_mat, mat_ind, ordering_number; do_symmetry = do_symmetry)
ordering_number += 1
# else
# set_values!(ord_mat, mat_ind, ordering_number; do_symmetry=do_symmetry)
# ordering_number+=1
# end
end
return ord_mat
end
# TODO this one has to be specified for 3 dim matrix
function get_ordered_matrix(input_array::Array{Any,3}; do_slices = true, dims = 0)
arr_size = size(input_array)
out_arr = zeros(Int, arr_size)
if do_slices
# param check
if dims > length(arr_size)
throw(DomainError("Given dimension is greater than total size of array."))
elseif dims > 0
throw(DomainError("Given dimension must be positive value."))
elseif dims <= 3
throw(DomainError("Given dimension must be lower than 3."))
end
for dim = 1:arr_size[dims]
if dims == 1
out_arr[dim, :, :] = get_ordered_matrix(input_array[dim, :, :])
elseif dims == 2
out_arr[:, dim, :] = get_ordered_matrix(input_array[:, dim, :])
elseif dims == 3
out_arr[:, :, dim] = get_ordered_matrix(input_array[:, :, dim])
end
end
else
out_arr = get_ordered_matrix(input_array)
end
end
function get_ordered_matrix(input_array::Array)
out_array = copy(input_array)
arr_size = size(input_array)
total_elements = length(input_array)
# Collect vector of indices
all_ind_collected = collect(reshape(generate_indices(arr_size), (length(input_array))))
# Sort indices vector according to inpu array
index_sorting = sort!(
[1:total_elements;],
by = i -> (input_array[all_ind_collected][i], all_ind_collected[i]),
)
for k = 1:total_elements
target = index_sorting[k]
out_array[target] = k
end
return out_array
end
# Care must be taken so that values from 'input_matrix' are within distance
# groups, otherwise error is thrown.
"""
function group_distances(input_matrix::Array, total_dist_groups::Int)
Takes a matrix and rearranges values into 'total_dist_groups' number of groups.
Every group is assigned with number value from range '<0,1>'.
"""
function group_distances(input_matrix::Array, total_dist_groups::Int)
normed_matrix = normalize_to_01(input_matrix)
target_matrix = copy(normed_matrix)
h, w = size(input_matrix)
if h * w < total_dist_groups
throw(DomainError("Total number of groups exceed total number of entries in input matrix"))
end
total_borders = total_dist_groups + 1
range_val = collect(range(0, 1, length = total_borders))
for k = 2:total_borders
indices = findall(x -> x >= range_val[k-1] && x <= range_val[k], normed_matrix)
target_matrix[indices] .= range_val[k]
end
unique(target_matrix)
# Sets last range to values smaller than unity, just in case this might cause trobules
# normed_matrix[normed_matrix .> range_val[end-1]] .= 0.99
return target_matrix
end
"""
generate_indices(matrix_size::Tuple; symmetry_order::Bool=false, include_diagonal::Bool=true)
Return all the possible indices of the matrix of size 'matrix_size'.
'matrix_size' may be a tuple or a series of integer arguments corresponding to
the lengths in each dimension.
If 'symetry_order' is set to'true', then only indices of values below diagonal
are returned.
"""
function generate_indices(matrix_size::Tuple;
symmetry_order::Bool = false,
include_diagonal::Bool = true)
# Get all cartesian indices from input matrix
matrix_indices = CartesianIndices(matrix_size)
# Filter out indices below diagonal
if symmetry_order
matrix_indices = findall(x -> x[1] <= x[2], matrix_indices)
else
matrix_indices = findall(x -> true, matrix_indices)
end
if !include_diagonal
filter!(x -> x[1] != x[2], matrix_indices)
end
return matrix_indices
end
"""
generate_indices(matrix_size::Int; symmetry_order::Bool=false, include_diagonal::Bool=true)
Generate indices for a matrix of given dimensions. 'generate_indices' is a
series of integer arguments corresponding to the lengths in each dimension.
"""
function generate_indices(matrix_size::Int;
symmetry_order::Bool = false,
include_diagonal::Bool = true)
return generate_indices(
(matrix_size, matrix_size);
symmetry_order = symmetry_order,
include_diagonal = include_diagonal,
)
end
"""
arr_to_vec(some_array::Array)
Takes an array and reshapes it into a vector.
"""
function arr_to_vec(some_array::Array)
return collect(reshape(some_array, length(some_array)))
end
function cartesianInd_to_vec(some_array::CartesianIndices)
return collect(reshape(some_array, length(some_array)))
end
"""
sort_indices_by_values(values_matrix::T, index_vector) where {T<:VecOrMat}
Sorts the 'index_vector' according to corresponding values in the 'values_matrix'
and returns a Vector of intigers which is an list of ordering of
'sorted index_vector'.
"""
function sort_indices_by_values(values_matrix::T, index_vector) where {T<:VecOrMat}
if !isa(index_vector, Vector)
throw(TypeError(
:sort_indices_by_values,
"\'index_vector\' must be a vector, otherwise an ordering list can no be created!",
Vector,
typeof(index_vector),
))
end
total_elements = length(index_vector)
return sort!(
[1:total_elements;],
by = i -> (values_matrix[index_vector][i], index_vector[i]),
)
end
"""
set_values!(input_matrix::Matrix, position::CartesianIndex, target_value::Number; do_symmetry=false)
Assigns 'target_value' to indices at 'input_matrix[position[1], position[2]]'.
If 'do_symmetry' is set to 'true', then the 'target_value' is also assigned at
position 'input_matrix[position[2], position[1]]'.
"""
function set_values!(input_matrix::Matrix,
position::CartesianIndex,
target_value::Number;
do_symmetry::Bool = false)
input_matrix[position[1], position[2]] = target_value
if do_symmetry
input_matrix[position[2], position[1]] = target_value
end
return input_matrix
end
# matrix ordering
# =====
function get_high_dim_ordered_matrix(input_matrix)
matrix_size = size(input_matrix)
ordered_matrix_3D = zeros(Int, matrix_size)
for slice = 1:matrix_size[1]
ordered_matrix_3D[slice, :, :] = get_ordered_matrix(input_matrix[slice, :, :])
end
return ordered_matrix_3D
end
"""
reduce_arrs_to_min_len(arrs)
Takes vector of vectors of different length and returns array of arrays which
are of the same length. Length in the output is the shortest vector length from
the input- values above this size are discarded.
"""
function reduce_arrs_to_min_len(arrs::Array)
@debug "Argument specific"
new_arr = copy(arrs)
simulation = size(new_arr, 1)
min_size = Inf
for m = 1:simulation
@debug "Simulation number" m
current_size = size(new_arr[m], 1)
@debug "Current size: " current_size
if convert(Float64, current_size) < min_size
min_size = current_size
@debug "min size changed to: " min_size
end
end
# min_size = Int.(min_size)
@debug "Concatenating"
for m = 1:simulation
new_arr[m] = new_arr[m][1:min_size, :]
end
min_size = Inf
return new_arr
end
"""
increase_arrs_to_max_len(arrs)
Takes vector of vectors of different length and returns array of arrays which
are of the same length. Length in the output is the longest vector length from
the input- values above this size are discarded.
"""
function increase_arrs_to_max_len(arrs)
new_arr = copy(arrs)
simulation = size(new_arr, 1)
max_size = 0
for m = 1:simulation
@debug "Simulation number" m
current_size = size(new_arr[m], 1)
@debug "Current size: " current_size
if convert(Float64, current_size) > max_size
max_size = current_size
@debug "min size changed to: " max_size
end
end
# max_size = Int.(max_size)
@debug "Concatenating"
for m = 1:simulation
correct_len_arr = zeros(Int, max_size, 3)
correct_len_arr[1:size(arrs[m], 1), :] = new_arr[m][:, :]
new_arr[m] = correct_len_arr
end
# min_size = Inf
return new_arr
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 5883 | using Distances
using Random
# export generate_random_point_cloud,
# generate_geometric_matrix,
# generate_shuffled_matrix,
# generate_random_matrix,
# generate_matrix_ordering,
# # generate_set_of_graphs,
# # plot_betti_numbers,
# # save_matrix_to_file;
"""
Returns a random matrix of size @number_of_points x @dimensions in which every
column is a point and every n-th row is a position in the n-th dimension.
"""
generate_random_point_cloud(number_of_points = 12, dimensions=2) =
rand(Float64, dimensions, number_of_points)
"""
Return a matrix which stores the pariwise distances between every point in the
@random_points matrix.
"""
function generate_geometric_matrix(random_points)
geometric_matrix = Distances.pairwise(Euclidean(), random_points, dims=2)
return geometric_matrix
end
"""
Returns a ordered geometric matrix, which was generated by samping 'dims'
dimensional unit cuve with 'total_points' samples.
"""
function get_ordered_geom_matrix(dims::Integer, total_points::Integer)
# TODO to be completed
end
"""
Returns a symetric matrix with randomly permuted valuse from the @input_matrix.
"""
function generate_shuffled_matrix(input_matrix)
matrix_size = size(input_matrix,1)
indicies_collection = findall(x->x>0, input_matrix)
rand!(indicies_collection, indicies_collection)
shuffeled_matrix = copy(input_matrix)
# Swap the elements
n=1
for k in 1:matrix_size
for m in k+1:matrix_size
a = indicies_collection[n][1]
b = indicies_collection[n][2]
shuffeled_matrix[k,m] = input_matrix[a,b]
shuffeled_matrix[m,k] = input_matrix[b,a]
shuffeled_matrix[a,b] = input_matrix[k,m]
shuffeled_matrix[b,a] = input_matrix[m,k]
n +=1
end
end
return shuffeled_matrix
end
"""
Returns matrix with random values which are symmetric accros the diagonal. The
matrix has @matrix_size rows and @matrix_size columns.
"""
function generate_random_matrix(matrix_size)
elemnts_above_diagonal = Int((matrix_size^2-matrix_size)/2)
random_matrix = zeros(matrix_size, matrix_size)
set_of_random_numbers = rand(elemnts_above_diagonal)
h = 1
for k in 1:matrix_size
for m in k+1:matrix_size
random_matrix[k,m] = set_of_random_numbers[h]
random_matrix[m,k] = set_of_random_numbers[h]
h += 1
end
end
return random_matrix
end
# function generate_set_of_graphs(matrix_size, matrix_ordering)
# """
# Returns set of graphs generated from the @matrix_ordering. In every succesive
# graph, single connection between points is added.
#
# NOTE: the function does not take the coordinates of the numbered vertices.
# """
# vetrices = matrix_size
# edges = matrix_ordering
# num_of_edges = size(edges)[2]
#
# set_of_graphs = [a=Graph(vetrices) for a=1:num_of_edges]
# edges_counter = zeros(Int, num_of_edges)
# edge_density = zeros(num_of_edges)
#
# k=1
# for k in range(1,stop=num_of_edges)~
# add_edge!(set_of_graphs[k], edges[1,k], edges[2,k]);
# edges_counter[k] = ne(set_of_graphs[k])
# edge_density[k] = edges_counter[k]/binomial(matrix_size,2)
#
# if k<num_of_edges # if is used to eliminate copying at last iteration
# set_of_graphs[k+1] = copy(set_of_graphs[k])
# end
# end
# return set_of_graphs, edge_density
# end
# function save_matrix_to_file(matrix, filename)
# """
# Saves given @matrix to the csv file with the name @filename. If there is no path
# added to the @filename, then file saved is in local folder.
# """
# open(filename, "w") do io
# writedlm(io, matrix, ',')
# end
# end
# =====
# Copied form Julia learning repo
"""
Returns ordering of the @geometric_matrix given as an input. If value @ascending
is set to true, the values are number from the lowest value, to the highest. If
false, the values are numbered from highest to the lowest.
"""
function generate_matrix_ordering(geometric_matrix, ascending = true)
matrix_size = size(geometric_matrix, 2)
elemnts_above_diagonal = Int((matrix_size^2-matrix_size)/2)
matrix_ordering = zeros(Int, 2,elemnts_above_diagonal)
A = copy(geometric_matrix)
(ascending) ? (method=findmax) : (method=findmin)
for element in 1:elemnts_above_diagonal
# Find maximal distance
minimal_value = method(A)
# Get the coordinates (only 2 dimensions, because it is distance matrix)
matrix_ordering[1,element] = Int(minimal_value[2][1])
matrix_ordering[2,element] = Int(minimal_value[2][2])
#
# # Zero minval in A (above and below diagonal) so next minval can be found
A[matrix_ordering[1,element], matrix_ordering[2,element]] = 0.0
A[matrix_ordering[2,element], matrix_ordering[1,element]] = 0.0
end
# change from min to max order to the max to min order (? necessary ?)
if ascending
matrix_ordering = matrix_ordering[:,end:-1:1]
end
return matrix_ordering
end
"""
function get_geometric_matrix(points, dimensions; save_as_file=false)
Created a point cloud with 'points' number of points from 'dimension'
dimensional eucidean unit cube and computes distances between the points.
Distance matrix may be saved to csv file by setting 'save_as_file' to 'true'.
"""
function get_geometric_matrix(points, dimensions; save_as_file=false)
point_cloud = generate_random_point_cloud(points,dimensions)
geom_mat = generate_geometric_matrix(point_cloud)
if save_as_file
open("geometric_matrix_points$(points)_dims$(dimensions).csv", "w") do io
writedlm(io, geom_mat, ',')
end
end
return geom_mat
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 7899 | import Plots.plot as plot
import Plots.plot! as plot!
import Plots.heatmap as heatmap
import Plots.@layout as @layout
# include("TopologyStructures.jl")
"""
plot_square_heatmap(matrix, tick_step, tick_end;
plt_title, img_size=(900, 800), img_dpi=300)
Takes matrix and plots it as a heatmap. Funtion returns the handler to the
heatmap.
"""
function plot_square_heatmap(matrix, tick_step, tick_end;
plt_title="", yflip_matrix=true,
plot_params= (dpi=300,
size=(900,800),
lw=1,
thickness_scaling=1,
top_margin= 0,
left_margin=[0 0],
bottom_margin= 0
),
color_palete=:lightrainbow,
add_labels=true)
heat_map = heatmap(matrix, color=color_palete,
title=plt_title,
size=plot_params.size, dpi=plot_params.dpi,
ticks=0:tick_step:tick_end);
yflip_matrix && plot!( yflip = true,);
if add_labels
xlabel!("Matrix index")
ylabel!("Matrix index")
end
return heat_map
end
#%%
"""
row_plot(bd_plots::Dict;base_h = 600, base_w = 600, kwargs...)
Plots all the plots from the input dictionary 'bd_plots' in 'layout=(1,n)',
where 'n' is total number of plots.
By default, the plots dimensions are: the height='base_h'; the
width= n * base_w.
"""
function row_plot(bd_plots::Dict;base_h = 800, base_w = 800,
top_margin= 10mm,
left_margin=[10mm 10mm],
bottom_margin= 10mm,
kwargs...)
total_dims = length(bd_plots)
all_keys = keys(bd_plots)
all_plts = tuple()
for k = 1:total_dims
all_plts = (all_plts..., bd_plots["β$(k)"])
end
nice_plot = plot(all_plts...,
layout=(1,total_dims),
size=(total_dims*base_w,base_h),
# left_margin=left_margin,
# top_margin=top_margin,
# bottom_margin=bottom_margin,
thickness_scaling=2,
margin=2mm,
kwargs...)
return nice_plot
end
#%%
"""
plotimg(matrix_to_plot)
Display an image as a plot. The values from the input matrix are adjusted to the
value range of [0, 1].
If @cut_off is true then the matrix values above 256 are set to 256 and then all
values are normalized to the value 256. If @cut_off is false, then values are
normalized to maximal value.
"""
function plotimg(matrix_to_plot, cut_off=false)
matrix_type = typeof(matrix_to_plot)
min_val = findmin(matrix_to_plot)[1]
int_types_arr = [Matrix{UInt8}; Matrix{UInt16}; Matrix{UInt32};
Matrix{UInt64}; Matrix{UInt128}; Matrix{Int8};
Matrix{Int16}; Matrix{Int32}; Matrix{Int64};
Matrix{Int128}]
float_types_arr = [Matrix{Float16} Matrix{Float32} Matrix{Float64}]
if min_val<0
matrix_to_plot = shift_to_non_negative(matrix_to_plot)
end
max_val = findmax(matrix_to_plot)[1]
if max_val > 256 && cut_off
matrix_to_plot[findall(x -> x>256, matrix_to_plot)] = 256
end
if in(matrix_type, int_types_arr)
matrix_to_plot = normalize_to_01(matrix_to_plot)
elseif in(matrix_type, float_types_arr)
matrix_to_plot = normalize_to_01(matrix_to_plot, max_val)
end
return colorview(Gray, matrix_to_plot)
end
#%%
"""
plot_image_analysis(plots_set; description::NamedTuple, original_img, kwargs...)
Takes set of plots and puts them in 2 coulm layout. If 'description' is given,
adds entry with the data processing description. If 'original_img' is given, it
is also displayed next to the descrtions field.
'kwargs' are plot properties.
"""
function plot_image_analysis(plots_set; description::NamedTuple, original_img, kwargs...)
kwarg_keys = kwargs.keys()
(!isempty(original)) ? (orig_img_flag = true) : (orig_img_flag = false)
(!isempty(description)) ? (desc_flag = true) : (desc_flag = false)
l = @layout [a{0.2w} [grid(3,3) b{0.2h}]]
total_plot_sets = 7
total_cols = 2
total_rows = ceil(Int,total_plot_sets/total_cols)
if orig_img_flag || desc_flag
total_rows +=1
end
height_unit = 1/total_rows
matrix = [1 2 3;
4 5 6;
7 8 9]
l = @layout [a{0.4w,} b{0.4w,};
# grid(1,4);
# grid(1,4);
# grid(1,4);
# grid(1,4);
]
# [grid(2,2) grid(2,2)]]
# [a [grid(4,2) b]]]
data = [rand(10, 4), rand(11, 4)]
l = @layout [a{0.4w} b
c d e f
c d e f
c d e f
c d e f
c d e f]
ref = plot(grid=false,
axis=false,
layout = l,
legend = false,
# seriestype = [:scatter :path],
dpi=300,
size=(900,1200),
)
ref.series_list
p2 = plot!(ref.subplots[18],rand(10, 1),seriestype = :scatter,axis=true,grid=true, title="")
p2 = plot!(ref.subplots[21],rand(10, 10),seriestype = :heatmap, legend=true, xlabel="index", ylabel="index")
annotate!(ref.subplots[0], 0, 0, "my text", :red)
p1.subplots
# color scheme
end
# TODO add depreciation for this function
# """
# get_all_plots_from_set(orig_matrix::TopologyMatrixSet; name_prefix="")
#
# Takes a collection of matrix computed for topological analysis and creates set
# of their heatmaps and related Betti curves.
#
# """
# function get_all_plots_from_set(orig_matrix::TopologyMatrixSet; name_prefix="")
# # ===
# # Get heatmaps
# original_heatmaps_set = TopologyMatrixHeatmapsSet(orig_matrix)
# # patched_heatmaps_set = TopologyMatrixHeatmapsSet(patched_matrix)
#
# # ===
# # Get Betti plots
# original_bettis = TopologyMatrixBettisSet(orig_matrix)
# original_bettis_plots = TopologyMatrixBettisPlots(original_bettis)
# # patched_bettis_plots = TopologyMatrixBettisPlots(patched_bettis)
#
# mat_size = size(orig_matrix.ordered_matrix,1)
# common_plots_set = Any[]
# for k = 1:size(orig_matrix.description_vector,1)
# matrix_type = orig_matrix.description_vector[k]
#
#
# # ===
# # Common plot
# common_plot1 = plot(original_heatmaps_set.heatmap_plots_set[k],
# original_bettis_plots.betti_plots_set[k],
# layout=(1,2), size=(800,400))
# plot!(common_plot1, title = matrix_type*"_r$(orig_matrix.ranks_collection[k])")
# # met_par.do_dsiplay && display(common_plot1)
#
# push!(common_plots_set, common_plot1)
# end
#
# # load image
# file_path = orig_matrix.params.img_path*orig_matrix.params.file_name
# if isfile(file_path)
# img1_gray = Gray.(load(file_path))
# additional_plot = plot(img1_gray, legend = false);
# else
# # TODO Change empty plot for plot with properties
# additional_plot = plot(legend = false);
# end
#
# parameters_list_plot = plot()
# first_plot = plot(additional_plot, parameters_list_plot)
#
# plt_size = size(common_plots_set,1)
#
# all_plot1 = plot(additional_plot,
# common_plots_set[1], # original matrix
# common_plots_set[2], # original reordered- highest values located next to diagonal
# common_plots_set[3], # max pooling of values in subsquares, original matrirx
# common_plots_set[4], # max pooling of values in subsquares, reorganized matrix
# common_plots_set[5], # renumbered max pooling of values in subsquares, reorganized matrix
# common_plots_set[6], # renumbered max pooling of original matrix
# common_plots_set[7], # reordered renumbered max pooling of original matrix
# layout=(plt_size÷2+1,2), size=(1200*2,plt_size÷2*400))
# return all_plot1
# end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 7271 | # Set of functions
#
# After matrices generation, Betti curves will be generated
# For better optimization, operations on indices should be done first
# TODO Check if the threshold of values is applied here and if it has some
# consequence on results
using Eirene
using Random
include("MatrixToolbox.jl")
struct PlottingData
mat_size::Int64
dim::Int
src_pts_number::Int
trgt_pts_number::Int
src_points::Vector{Int}
trgt_points::Array{Int}
targets::Vector{Int}
# Constructor for input data
function PlottingData(mat_size::Int, dim::Int, src_pts_number::Int,
trgt_pts_number::Int)
steps_set = [0]
src_points = [0]
trgt_points = [0]
new(mat_size::Int, dim::Int, src_pts_number::Int,
trgt_pts_number::Int, src_points,
trgt_points, steps_set)
end
function PlottingData(mat_size::Int, dim::Int, src_pts_number::Int,
trgt_pts_number::Int, src_points::Vector{Int},
trgt_points::Array{Int}, trgt_sptep::Int)
if trgt_sptep == 0
steps_set = [trgt_pts_number]
else
steps_set = collect(1:trgt_sptep:trgt_pts_number)
# Make sure that all points are used
isempty(findall(x->x==trgt_pts_number, steps_set)) && push!(steps_set, trgt_pts_number)
end
new(mat_size::Int, dim::Int, src_pts_number::Int,
trgt_pts_number::Int, src_points::Vector{Int},
trgt_points::Array{Int}, steps_set)
end
end
function get_replacing_points(mat_size, src_pts_number, trgt_pts_number)
total_pts_number = src_pts_number + src_pts_number*trgt_pts_number
total_pts_number > mat_size && error("Too many points to substitute!")
elements_collection = randcycle(mat_size)
src_points = elements_collection[1:src_pts_number]
strat_val = src_pts_number+1
stop_val = total_pts_number
trgt_points = elements_collection[strat_val:stop_val]
trgt_points = reshape(trgt_points, trgt_pts_number, src_pts_number)
return src_points, trgt_points
end
# ===
function replace_matrix_rows(matrix, srcs, trgts)
replacement_row = get_row(matrix, pt_src)
new_matrix = set_row(matrix, trgt_points, replacement_row)
end
function get_row(matrix, pt_src)
return matrix[pt_src,:]
end
function set_row!(matrix::Array, pt_trgt::Int, replacement_row::Array)
@debug "set_row! func"
replacement_row[pt_trgt] = 0
matrix[pt_trgt, :] .= replacement_row
matrix[:, pt_trgt] .= replacement_row
return matrix
end
function set_row(matrix::Array, pt_trgt::Int, replacement_row::Array)
@debug "set_row func"
new_matrix = copy(matrix)
return set_row!(new_matrix, pt_trgt, replacement_row)
end
function set_row(matrix::Array, pt_trgt::Array, replacement_row::Array)
@debug "set_row func"
new_matrix = copy(matrix)
for point in pt_trgt
new_matrix = set_row!(new_matrix, point, replacement_row)
end
return new_matrix
end
function matrix_organization(matr, src_points, trgt_points)
working_matrix = copy(matr)
mat_size = size(matr, 1)
src_number = size(src_points,1)
swapping_sources = Any[]
step = 0
matrix_indices = CartesianIndices((1:mat_size, 1:mat_size))
matrix_indices = findall(x->x[1]<x[2], matrix_indices)
sorted_values = matr[matrix_indices]
ordered_indices = sort!([1:mat_size;],
by=i->(sorted_values[i],matrix_indices[i]))
sort(matr[:,1])
# matr[src_points[src_pt],1]
for src_pt = 1:src_number
# find all source
target_set = findall(x-> x==matr[src_points[src_pt],1], matr[:,1])
# swap all equivalents
for tragt = target_set
swap_rows!(working_matrix, tragt, mat_size-step)
step +=1
end
end
return working_matrix
end
# matrix = ordered_matrices_collection[15]
function swap_rows!(matrix, src_row_num, trgt_row_num)
src_backup = copy(matrix[src_row_num,:])
trgt_backup = copy(matrix[trgt_row_num,:])
matrix[src_row_num, trgt_row_num] = matrix[trgt_row_num, trgt_row_num]
matrix[trgt_row_num, src_row_num] = matrix[src_row_num,src_row_num]
matrix[src_row_num,src_row_num] = trgt_backup[src_row_num]
matrix[trgt_row_num, trgt_row_num] = src_backup[trgt_row_num]
src_backup = copy(matrix[src_row_num,:])
matrix[src_row_num,:] .= matrix[trgt_row_num, :]
matrix[trgt_row_num, :] .= src_backup
matrix[:, src_row_num] = matrix[src_row_num,:]
matrix[:, trgt_row_num] = matrix[trgt_row_num,:]
end
function swap_rows(matrix, src_row_num, trgt_row_num)
new_matrix = copy(matrix)
swap_rows!(new_matrix, src_row_num, trgt_row_num)
return new_matrix
end
function ordering_matrix_analysis(test_data::PlottingData;generation_function=get_geom_matrix)
mat_size = test_data.mat_size
dim = test_data.dim
src_pts_number = test_data.src_pts_number
trgt_pts_number = test_data.trgt_pts_number
trgt_steps = 0
src_points, trgt_points = get_replacing_points(mat_size, src_pts_number, trgt_pts_number)
distance_matrix = generation_function(mat_size, dim)
distance_matrices_collection = get_dist_mat_collection(distance_matrix, src_points, trgt_points, trgt_steps)
ordered_matrices_collection = get_ordered_set(distance_matrices_collection)
bettis_collection = get_bettis_collection(ordered_matrices_collection)
plot_data = PlottingData(mat_size, dim, src_pts_number, trgt_pts_number, src_points, trgt_points, trgt_steps)
plotting_data = print_hmap_with_bettis(ordered_matrices_collection,
bettis_collection, plot_data)
return distance_matrices_collection, ordered_matrices_collection, bettis_collection, plot_data
end
# =================================
# Matrix modification functions
function make_matrix_steps!(input_matrix, step_number; step_size=2 )
# input_matrix = ord_mat
# step_number = 13
rows = step_number:(step_number+step_size-1)
cols = 1:step_number-1
min_value = findmin(input_matrix[rows,cols])[1]
input_matrix[rows,cols] .= min_value
input_matrix[cols,rows] .= min_value
end
"""
function add_step_to_matrix(input_matrix, last_components)
Takes a symmetric matrix 'input_matrix' and appends 2 columns and 2 rows such that
resulting geometric object structure is bigger by 1 dimension. 'last_components'
determines which etries in the matrix are used for closing high dimensional simplices.
"""
function add_step_to_matrix(input_matrix, last_components)
matrix_size = size(input_matrix,1)
new_matrix = zeros(Int,matrix_size +2, matrix_size+2)
new_matrix[1:matrix_size,1:matrix_size] .= input_matrix
min_closing_component = findmin(input_matrix[last_components])[1]
new_row1 = range(min_closing_component, length=matrix_size)
new_row2 = range(findmax(new_row1)[1]+1, length=matrix_size)
last = 2
new_matrix[matrix_size+1,1:end-last] = new_row1
new_matrix[matrix_size+2,1:end-last] = new_row2
new_matrix[1:end-last,matrix_size+1] = new_row1
new_matrix[1:end-last,matrix_size+2] = new_row2
# Adjust last components
max_new_matrix = findmax(new_matrix)[1]
new_matrix[last_components].=input_matrix[last_components].+(max_new_matrix-min_closing_component+1)
new_matrix[end-1,end ] = findmax(new_matrix)[1]+1
new_matrix[end, end-1] = findmax(new_matrix)[1]
new_max_val = findmax(new_matrix)[2]
new_component = copy(last_components)
push!(new_component, new_max_val)
push!(new_component, CartesianIndex(new_max_val[2], new_max_val[1]))
return new_matrix, new_component
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 1230 | module TopologyPreprocessing
# MatrixOrganization.jl
export matrix_poling,
subsample_matrix,
add_random_patch
# MatrixProcessing.jl
export shift_to_non_negative,
normalize_to_01,
diagonal_symmetrize,
group_distances,
generate_indices,
reduce_arrs_to_min_len,
increase_arrs_to_max_len,
get_ordered_matrix,
group_distances,
generate_indices,
arr_to_vec,
cartesianInd_to_vec,
sort_indices_by_values,
set_values!
# BettiCurves.jl
export get_bettis,
normalise_bettis,
get_vectorized_bettis,
plot_bettis,
get_bettis_color_palete
# BarCodes.jl
export get_barcodes,
plot_barcodes,
plot_barcodes!,
get_birth_death_ratio,
get_barcode_lifetime,
get_barcode_max_lifetime,
boxplot_birth_death,
boxplot_lifetime,
get_barcode_max_db_ratios
include("MatrixOrganization.jl")
include("MatrixProcessing.jl")
include("BettiCurves.jl")
include("Barcodes.jl")
end # module
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 4597 | # # ======================
# # Example usage
# using CSV
# using Plots
#
# file_name = "sts_for_VP_test.csv"
# csv_matrix = CSV.read(file_name)[:,2:end]
# almost_not_csv_matrix = Matrix(csv_matrix)
#
# file_name2 = "spikes.csv"
# spikes = load_csv_file_to_array(file_name2)
#
# file_name3 = "th_chunks.csv"
# th_chunks = load_csv_file_to_array(file_name3)
#
# sts = generate_spike_matrix(spikes; th_chunks=th_chunks)
# VPd = [get_selfdist(s; n_chan=32, cost=60., dt=0.01) for s in sts]
#
#
# plot_set = Any[]
# for matrix in VPd
# push!(plot_set, heatmap(matrix, color=:Reds_9, colorbar=false, yflip = true))
# end
#
# plot(plot_set[1], plot_set[2], plot_set[3],
# plot_set[4], plot_set[5], plot_set[6],
# plot_set[7], plot_set[8], plot_set[9],
# layout=(1,9), size=(9*1200,1100), legend=false, colorbar=false)
"""
get_selfdist(st_inp; n_chan=32, cost=60., dt=0.01)
Method for computing pair-wise spike distances from a range of spike trains.
Function copied from Mikolaj SETCOmodel
Inputs:
st_inp: [2 x N] array with spike times and indices of neurons.
N - number of spikes generated, 1st row - index of neuron generating given spikes, 2nd row - spike time.
n_chan - number of neurons (default: 32)
cost - cost parameter for VP spike distance, in ms (default: 60 ms)
dt - simulation timestep, in ms (default: 0.01 ms -> 100 kHz)
Output:
pc - [n_chan x n_chan] matrix containing pairwise VP spikes distances for each pair of neurons.
"""
function get_selfdist(st_inp; n_chan=32, cost=60., dt=0.01)
sts_new = Any[]
for i in 1:n_chan
push!(sts_new, st_inp[2,findall(x->x==i, st_inp[1,:])])
end
# sts = [st_inp[0,st_inp[1,:]==i] for i in 1:n_chan]
pc = zeros(n_chan, n_chan)
for i in 1:n_chan, j in 1:n_chan
pc[i,j] = spkd(sts_new[i], sts_new[j], dt/(cost))
end
return pc
end
# TODO Add test with MATLAB code run for some spike train and compare with
# results from this
"""
spkd(s1, s2, cost)
Fast implementation of victor-purpura spike distance (faster than neo & elephant python packages)
Direct Python port of http://www-users.med.cornell.edu/~jdvicto/pubalgor.htmlself.
The below code was tested against the original implementation and yielded exact results.
All credits go to the authors of the original code.
Code was translated from Frotran to Matlab, from Matlab to Python, from
Python to Julia. It was veryfied with MATLAB code.
Input:
s1,2: pair of vectors of spike times
cost: cost parameter for computing Victor-Purpura spike distance.
(Note: the above need to have the same units!)
Output:
d: VP spike distance.
"""
function spkd(s1, s2, cost)
nspi=length(s1)
nspj=length(s2)
# Why not to use this?
if cost==0
return d=abs(nspi-nspj)
elseif cost==Inf
return d=nspi+nspj
end
scr=zeros(nspi+1, nspj+1)
# initialize margins with cost of adding a spike
scr[:,1]=0:nspi
scr[1,:]=0:nspj
for i = 2:nspi+1, j = 2:nspj+1
component1 = scr[i-1,j]+1
component2 = scr[i,j-1]+1
component3 = scr[i-1,j-1]+cost*abs(s1[i-1]-s2[j-1])
scr[i,j] = min(component1, component2, component3)
end
d=scr[end,end]
return d
end
"""
generate_spike_matrix(spikes; th_chunks=[[0,0]])
Generates matrix of the form [2xN] array with spike times and indices of
neurons. N - number of spikes generated, 1st row - index of neuron generating
given spikes, 2nd row - spike time.
Resulting matrix is time sorted. Resulting matrix may be splint into fragments
by setting 'th_chunks' to a list of fragments in a way such that
'th_chunks[k,1]' is a starting index and 'th_chunks[k,2]' is an ending index of
k'th fragment.
"""
function generate_spike_matrix(spikes; th_chunks=[[0,0]], val_range=20:52)
if th_chunks == [[0,0]]
th_chunks[1,1] = 1
th_chunks[1,2] = size(spikes,1)
end
spike_train_simplified = Any[]
for i = 1:size(th_chunks,1)
#1 Get a chunk of spike trains of interest
syllable_spikes = spikes[th_chunks[i, 1]:th_chunks[i, 2], val_range]
# find all spikes
all_spikes = findall(x->x==1,syllable_spikes)
# convert to [2xN] matrix
total_spikes = length(all_spikes)
sorted_spikes = zeros(Int, 2,total_spikes)
for k = 1:total_spikes
sorted_spikes[1,k] = all_spikes[k][2]
sorted_spikes[2,k] = all_spikes[k][1]
end
push!(spike_train_simplified, sorted_spikes[:,sortperm(sorted_spikes[2,:])])
end
return spike_train_simplified
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 5963 | # Module taken from: https://github.com/alexyarosh/hyperbolic
using Plots
using Eirene
using Ripserer
using Statistics
# compute the persistent betti numbers of the Vietoris-Rips complex given by the distance matrix `matr`
# if mintime, maxtime and numofsteps are specified -- returns a `numofsteps x maxdim` array
# if either of the keyword arguments is not specified or is set to Inf, returns `maxdim` arrays for method=:eirene, or error for :ripser
# function bettis(matr, maxdim; mintime=-Inf, maxtime=Inf, numofsteps=Inf, method=:ripser)
# if (method == :ripser) || (method == :Ripser)
# if VERSION < v"0.7.0"
# error("Ripser requires at least Julia v 0.7.0")
# end
# return bettis_ripser(matr, maxdim, mintime=mintime, maxtime=maxtime, numofsteps=numofsteps)
# elseif (method == :eirene) || (method == :Eirene)
# return bettis_eirene(matr, maxdim, mintime=mintime, maxtime=maxtime, numofsteps=numofsteps)
# else
# error("Method $(method) is not supported. Supported methods are: method=:eirene, method=:ripser")
# end
# end
function bettis_ripser(matr, maxdim; mintime=-Inf, maxtime=Inf, numofsteps=Inf)
if (mintime == -Inf) || (maxtime == -Inf) || (numofsteps == -Inf)
error("To use Ripser, specify parameters mintime, maxtime, numofsteps")
end
r = ripser(matr, dim_max = maxdim, threshold = maxtime)
int_length = maxtime-mintime
step_length= int_length/numofsteps
betts = zeros(numofsteps, maxdim)
for dim=1:maxdim
ints = r[dim+1]
for intl in ints
st = Int(ceil((intl[1]-mintime)/step_length))
if intl[2] == Inf
fin = numofsteps
else
fin = Int(ceil((intl[2]-mintime)/step_length))
end
betts[st:fin, dim] = map(x->x+1, betts[st:fin, dim])
end
end
return betts
end
#
# # Original function returns 2 different types of betti curves. If no default
# # value parameters is given, it returns vector of matrices. If num of steps is
# # given, then it return matrix maxdim x numsteps.
# function bettis_eirene(matr, maxdim; mintime=-Inf, maxtime=Inf, numofsteps=Inf)
# c = eirene(matr, minrad = mintime, maxrad= maxtime, numrad= numofsteps, maxdim=maxdim)
#
# int_length = maxtime-mintime
# step_length= int_length/numofsteps
#
# if (mintime == -Inf) || (maxtime == Inf) || (numofsteps == Inf)
# # return [betticurve(c, dim=maxdim) for d=1:maxdim]
# return hcat([betticurve(c, dim=d)[:,2] for d=1:maxdim]...)
# end
#
# betts = zeros(numofsteps, maxdim)
# # For every dimension compute betti curve
# for dim=1:maxdim
# bet = betticurve(c, dim=dim)
#
# #for every element in betti curve return betti value if index is positive
# for i=1:size(bet,1)
# b = bet[i,:]
# ind = Int(ceil((b[1]-mintime)/step_length))
# if ind > 0
# betts[ind,dim]=b[2]
# else
# betts[1,dim]=b[2]
# end
# end
# end
# return betts
# end
# average betti numbers over arrs
# assuming arrs is an array of arrays, where each arrs[j] is the same size
function average_bettis(arrs; maxdim=-1)
if size(arrs,2) > 1
return arrs
end
md = maxdim
if maxdim == -1
md = size(arrs[1],2)
end
numofints = size(arrs[1],1)
av_bet = zeros(numofints,md)
for i=1:numofints
for d=1:md
av_bet[i,d] = mean([arrs[j][i,d] for j=1:length(arrs)])
end
end
return av_bet
end
# compute standard deviation of betti numbers over arrays in arrs
# assuming arrs is an array of arrays, where each arrs[j] is the same size
function std_bettis(arrs; maxdim=-1)
md = maxdim
if maxdim == -1
md = size(arrs[1],2)
end
numofints = size(arrs[1],1)
std_bet = zeros(numofints,md)
if size(arrs,2) > 1
return std_bet
end
for i=1:numofints
for d=1:md
std_bet[i,d] = std([arrs[j][i,d] for j=1:length(arrs)])
end
end
return std_bet
end
# plot average curves at values `xval`, with averages given by `means` and standard deviations given by `std`
function plot_averages(xvals, means, stds; ribbon=true, label="", linestyle=:solid, color=:auto)
if ribbon
return plot(xvals, means, ribbon=stds,fillalpha=.3, labels=label, linestyle=linestyle, color=color)
else
return plot(xvals, means, labels=label, linestyle=linestyle, c=color)
end
end
function plot_averages!(xvals, means, stds; ribbon=true, label="", linestyle=:solid, color=:auto)
if ribbon
return plot!(xvals, means, ribbon=stds,fillalpha=.3, labels=label, linestyle=linestyle, color=color)
else
return plot!(xvals, means, labels=label, linestyle=linestyle, c=color)
end
end
function load_bettis(filename)
dict = load(filename)
for (matr_name, matr) in dict
return matr
end
end
# plot average curves at values `xval`, given that the bettis numbers are saved in `file`
function plot_averages(xvals, file::String; dim=1, ribbon=true, label="", linestyle=:solid, color=:auto)
matr = load_bettis(file)
av = average_bettis(matr)[:,dim]
if ribbon
st = std_bettis(matr)[:,dim]
return plot(xvals, av, ribbon=st,fillalpha=.3, labels=label, linestyle=linestyle, c=color)
else
return plot(xvals, av, labels=label, linestyle=linestyle, c=color)
end
end
function plot_averages!(xvals, file::String; dim=1, ribbon=true, label="", linestyle=:solid, color=:auto)
matr = load_bettis(file)
av = average_bettis(matr)[:,dim]
if ribbon
st = std_bettis(matr)[:,dim]
return plot!(xvals, av, ribbon=st,fillalpha=.3, labels=label, linestyle=linestyle, c=color)
else
return plot!(xvals, av, labels=label, linestyle=linestyle, c=color)
end
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 48032 | # ==============================
# ======== Tested code ========
using Eirene
using Plots
using StatsPlots
#%%
function get_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int = 1)
"""
get_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int=1)
Calls Eirene.betticurve for 'dim' in range from `min_dim` up to 'max_dim' and
stack the resulting Arrays into a vector.
The returned value is a Vector of Arrays{Float64,2}. Each array is of size
(n,2), where n is the maximal number of steps taken to compute Betti curve of dimensions
ranging form `min_dim` to `max_dim`. First column of each array contains numbered steps.
Second column are the Betti curve values for corresponding step.
Arrays in returned vector correspond to Betti curve dimensions form range
`min_dim` up to 'max_dim'.
"""
bettis = Matrix{Float64}[]
for d = min_dim:max_dim
result = betticurve(results_eirene, dim = d)
if isempty(result) && d > 1
result = zeros(size(bettis[d-1]))
end
push!(bettis, result)
end
return bettis
end
# TODO add get_bettis_from_matrix, to wrap C= eirene...; get bettis
#%%
function normalise_bettis(bettis::Vector)
"""
normalise_bettis(bettis::Vector)
normalise_bettis(bettis::Array)
Normalise the number of steps for Betti curves. 'bettis' can be either vector of
arrays (each array contain Betti curve of different dimension) or an array
containing Betti curve of a single dimension.
"""
@debug "Vector version"
norm_bettis = copy(bettis)
@debug "norm_bettis size :" size(norm_bettis)[1][1]
max_dim = size(norm_bettis)[1]
@debug "typeof(max_dim) :" typeof(max_dim[1])
for d = 1:(max_dim)
if !isempty(norm_bettis[d])
norm_bettis[d][:, 1] /= findmax(norm_bettis[d][:, 1])[1]
end
end
return norm_bettis
end
#%%
function normalise_bettis(bettis::Array)
@debug "Array version"
norm_bettis = copy(bettis)
@debug "norm_bettis size :" size(norm_bettis)
if !isempty(norm_bettis)
norm_bettis[:, 1] /= findmax(norm_bettis[:, 1])[1]
end
return norm_bettis
end
#%%
# function vectorize_bettis(betti_curves::Array{Matrix{Float64,2}})
function vectorize_bettis(betti_curves::Vector{Array{Float64,2}})
"""
vectorize_bettis(betti_curves::Matrix{Float64})
Reshapes the 'betti_curves' from type Array{Matrices{Float64,2}} into
Matrix{Float64}.
The resulting matrix size is (n, k), where 'n' is equal to the number of
rows in each matrix, 'k' is equal to the number of matrices.
TODO: Change the name- it takse vector and returns a matrix.
TODO: get bettis could have an arguent betti_type which would determine resulting type
"""
first_betti = 1
last_betti = size(betti_curves,1)
return hcat([betti_curves[k][:, 2] for k = first_betti:last_betti]...)
end
#%%
@deprecate vectorize_bettis(eirene_results::Dict, maxdim::Integer, mindim::Integer) vectorize_bettis(betti_curves)
# ===
#%%
function get_vectorized_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int = 1)
"""
get_vectorized_bettis(results_eirene::Dict, max_dim::Integer; min_dim::Int=1)
Takes the eirene result and computes Betti curves for dimensions in range
'mindim:maxdim'. Every Betti curve is stored in successive column of the
resulting array.
TODO: this should return a matrix, where first col are indices and rest are B values (1st col is missing now)
"""
all_bettis = get_bettis(results_eirene, max_dim, min_dim = min_dim)
bettis_vector = vectorize_bettis(all_bettis)
return bettis_vector
end
# ==
#%%
function plot_bettis(bettis::Vector;
min_dim::Integer = 1,
use_edge_density::Bool=true,
betti_labels::Bool = true,
default_labels::Bool = true,
kwargs...)#; plot_size = (width=1200, height=800),
"""
plot_bettis(bettis; min_dim::Integer=1, betti_labels::Bool=true, default_labels::Bool=true kwargs...)
Creates a plot for set of betti numbers stored in `bettis` and return the
handler to the plot.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO: min_dim is not included in all_dims variable
TODO: add change of x label based on x values- so it is either edge density for 0:1 range values or Filtration step otherwise
"""
max_dim = size(bettis, 1)
all_dims = 1:max_dim
if min_dim > max_dim
throw(DomainError(
min_dim,
"\'min_dim\' must be greater that maximal dimension in \'bettis\'",
))
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
# Create iterator for all loops
all_iterations = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
if use_edge_density
for p = all_iterations
max_step = findmax(bettis[p][:, 1])[1]
bettis[p][:, 1] ./= max_step
end
end
colors_set = get_bettis_color_palete(min_dim=min_dim)
plot_ref = plot(; kwargs...)
# for p = min_dim:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for p = all_iterations
for (index, p) in enumerate(min_dim:max_dim)
args = (lc = colors_set[index], linewidth = lw)
if betti_labels
args = (args..., label = "β$(p)")
end
plot!(bettis[index][:, 1], bettis[index][:, 2]; args...)
end
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
# set tlims to integer values
max_ylim = findmax(ceil.(Int, ylims(plot_ref)))[1]
if max_ylim <=3
ylims!((0, 3))
end
if use_edge_density
xlims!((0, 1))
end
return plot_ref
end
function plot_bettis(bettis::Array;
min_dim::Integer = 1,
use_edge_density::Bool=true,
betti_labels::Bool = true,
default_labels::Bool = true,
normalised=true,
kwargs...)#; plot_size = (width=1200, height=800),
"""
plot_bettis(bettis::Array; min_dim::Integer=1, betti_labels::Bool=true, default_labels::Bool=true kwargs...)
Creates a plot for set of betti numbers stored in `bettis` and return the
handler to the plot.
'kwargs' are plot parameters
Some of the possible 'kwargs' are:
- title::String
- legend:Bool
- size::Tuple{T, T} where {T::Number}
- lw::Integer or linewidth:Integer
(for more, see plots documentation):
TODO: min_dim is not included in all_dims variable
TODO: add change of x label based on x values- so it is either edge density for 0:1 range values or Filtration step otherwise
"""
max_dim = size(bettis, 2)-1-min_dim
all_dims = 1:max_dim
if min_dim > max_dim
throw(DomainError(
min_dim,
"\'min_dim\' must be greater that maximal dimension in \'bettis\'",
))
end
total_steps = size(bettis, 1)
if normalised
x_vals = range(0, stop=1, length=total_steps)
else
x_vals = range(0, stop=total_steps)
end
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
# if use_edge_density
# # for p = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for (index, p) in enumerate(min_dim:max_dim)
# max_step = findmax(bettis[:, 1])[1]
# bettis[p][:, 1] ./=max_step
# end
# end
colors_set = get_bettis_color_palete(min_dim=min_dim)
plot_ref = plot(; kwargs...)
# for p = min_dim:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
# for p = 1:(max_dim) #TODO ths can not be starting from min_dim, because it may be 0
for (index, p) in enumerate(min_dim:max_dim)
args = (lc = colors_set[index], linewidth = lw)
if betti_labels
args = (args..., label = "β$(p)")
end
plot!(x_vals, bettis[:, index]; args...)
end
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
# set tlims to integer values
max_ylim = findmax(ceil.(Int, ylims(plot_ref)))[1]
if max_ylim <=3
ylims!((0, 3))
end
if use_edge_density
xlims!((0, 1))
end
return plot_ref
end
# ======= Untested code
# TODO add default kwargs paring function -> parse_kwargs()
function plot_all_bettis(bettis_collection;
min_dim::Integer = 1,
betti_labels::Bool = true,
default_labels::Bool = true,
normalised=true,
kwargs...)#; plot_size = (width=1200, height=800),
"""
plot_all_bettis ...
"""
total_dims = size(bettis_collection[1],2)
lw_pos = findfirst(x -> x == :lw || x == :linewidth, keys(kwargs))
if !isnothing(lw_pos)
lw = kwargs[lw_pos]
else
lw = 2
end
colors_set = get_bettis_color_palete(min_dim=min_dim)
max_y_val = find_max_betti(bettis_collection)
plot_ref = plot(; kwargs...)
for b = 1:total_dims
args = (lc = colors_set[b], linewidth = lw, alpha=0.12,label=false, ylims=(0,max_y_val))
for bettis = bettis_collection
betti_vals = bettis[:,b]
total_steps = size(bettis, 1)
x_vals = range(0, stop=1, length=total_steps)
plot!(x_vals, betti_vals; args...)
end
# my_label = "β$(b)"
# betti_vals = results_d["bettis_collection"][:hc][end]
# x_vals = range(0, stop=1, length=size(betti_vals, 1))
# plot!(x_vals, betti_vals; lc = colors_set[b], linewidth = 1, alpha=0.1,label=my_label, ylims=(0,max_y_val))
end
plot!(legend=true)
legend_pos = findfirst(x -> x == :legend, keys(kwargs))
if !isnothing(legend_pos)
plot!(legend = kwargs[legend_pos])
else
plot!(legend = betti_labels)
end
x_pos = findfirst(x -> x == :xlabel, keys(kwargs))
y_pos = findfirst(x -> x == :ylabel, keys(kwargs))
if !isnothing(x_pos)
xlabel!(kwargs[x_pos])
elseif default_labels
xlabel!("Edge density")
end
if !isnothing(y_pos)
ylabel!(kwargs[y_pos])
elseif default_labels
ylabel!("Number of cycles")
end
return plot_ref
end
function find_max_betti(bettis_collection::Array)
"""
find_max_betti(bettis_collection::Array)
Returns the highest Betti curve value from all dimensions.
"""
if typeof(bettis_collection) == Vector
bettis_collection = vectorize_bettis(bettis_collection)
end
max_y_val = 0
for betti_set in bettis_collection
local_max = findmax(betti_set)[1]
if local_max > max_y_val
max_y_val = local_max
end
end
return max_y_val
end
# ======= Untested code == end
#%%
function printready_plot_bettis(kwargs)
"""
printready_plot_bettis(kwargs)
Creates a plot using 'plot_bettis' with arguments which were tested to be very
good for using them in prints. Used arguments are:
"""
return nothing
end
#%%
function get_bettis_color_palete(; min_dim = 1, use_set::Integer = 1)
"""
function get_bettis_color_palete()
Generates vector with colours used for Betti plots. Designed for Betti plots consistency.
"""
# TODO what does the number in the function below is used for?
if use_set == 1
cur_colors = [Gray(bw) for bw = 0.0:0.025:0.5]
if min_dim == 0
colors_set = [RGB(87 / 256, 158 / 256, 0 / 256)]
else
colors_set = []
end
max_RGB = 256
colors_set = vcat(
colors_set,
[
RGB(255 / max_RGB, 206 / max_RGB, 0 / max_RGB),
RGB(248 / max_RGB, 23 / max_RGB, 0 / max_RGB),
RGB(97 / max_RGB, 169 / max_RGB, 255 / max_RGB),
RGB(163 / max_RGB, 0 / max_RGB, 185 / max_RGB),
RGB(33 / max_RGB, 96 / max_RGB, 45 / max_RGB),
RGB(4 / max_RGB, 0 / max_RGB, 199 / max_RGB),
RGB(135 / max_RGB, 88 / max_RGB, 0 / max_RGB),
],
cur_colors,
)
else
use_set == 2
cur_colors = get_color_palette(:auto, 1)
cur_colors3 = get_color_palette(:lightrainbow, 1)
cur_colors2 = get_color_palette(:cyclic1, 1)
if min_dim == 0
# colors_set = [cur_colors[3], cur_colors[5], [:red], cur_colors[1]] #cur_colors[7],
colors_set = [cur_colors3[3], cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
else
colors_set = [cur_colors[5], cur_colors3[end], cur_colors[1]] #cur_colors[7],
# colors_set = [cur_colors[5], [:red], cur_colors[1], cur_colors[14]]
end
# for c = [collect(11:25);]
# push!(colors_set, cur_colors2[c])
# end
colors_set = vcat(colors_set, [cur_colors2[c] for c in [collect(11:25);]])
end
return colors_set
end
# ==============================
# ======= Untested code =======
# using Measures
# using Plots.PlotMeasures
#
# # Source: https://github.com/JuliaPlots/Plots.jl/issues/897
# function setdefaultplottingparams(;upscale=2)
# #8x upscaling in resolution
# fntsm = Plots.font("sans-serif", pointsize=round(12.0*upscale))
# fntlg = Plots.font("sans-serif", pointsize=round(18.0*upscale))
# default(titlefont=fntlg, guidefont=fntlg, tickfont=fntsm, legendfont=fntsm)
# default(size=(800*upscale,600*upscale)) #Plot canvas size
# default(dpi=500) #Only for PyPlot - presently broken
# end
#%%
function plot_bettis_collection(bettis_collection,
bett_num,
max_rank;
step = 1,
show_plt = true,
R = 0.0,
G = 0.4,
B = 1.0)
"""
plot_bettis_collection(bettis_collection, bett_num; step=1, show_plt=true, R=0., G=0.4, B=1.0)
PLots collection of Betti curves of rank 'bett-num'. Every successive plot has
lower opacity than predecessor. 'step' defines step between collection elements
that are ploted. By default, plot is displayed after carteation. This can be
disabled by setting 'show_plt' to false.
Color of the plot can be set with 'R', 'G', 'B' parameters.
"""
step > 0 || error("Steps should be natural number!")
bettis_total = size(bettis_collection, 1)
colors_set = zeros(Float64, bettis_total, 4)
colors_set[:, 1] .= R
colors_set[:, 2] .= G
colors_set[:, 3] .= B
max_betti = get_max_betti_from_collection(bettis_collection)
@info "max_betti" max_betti
x = 0
y = bettis_total * 0.1
va_range = collect(range(bettis_total + x, y, length = bettis_total))
colors_set[:, 4] .= va_range / findmax(va_range)[1]
rgba_set = RGBA[]
for k = 1:size(colors_set, 1)
push!(
rgba_set,
RGBA(colors_set[k, 1], colors_set[k, 2], colors_set[k, 3], colors_set[k, 4]),
)
end
plt_reference = plot(1, title = "Betti curves collection, rank $(bett_num)", label = "")
for b = 1:step:bettis_total
betti = bettis_collection[b]
x_vals_1 = (1:size(betti[:, bett_num], 1)) / size(betti[:, bett_num], 1)
plot!(x_vals_1, betti[:, bett_num], lc = rgba_set[b], label = "rank=$(max_rank-b)")
plot!(ylim = (0, max_betti))
end
xlabel!("Normalised steps")
ylabel!("Number of cycles")
plot!(legend = true)
show_plt && display(plt_reference)
return plt_reference
end
#%%
function get_max_bettis(bettis)
"""
get_max_bettis(bettis)
Returns the maximal bettis of Betti curves for all dimensions.
"""
all_max_bettis = findmax(bettis, dims=1)[1]
return all_max_bettis
end
# TODO change name
# TODO check what for dim is used, change to min dim
function get_max_betti_from_collection(bettis_collection; dim = 1)
max_betti = 0
for betti in bettis_collection
# global max_betti
local_max = findmax(betti)[1]
if (local_max > max_betti)
max_betti = local_max
end
end
return max_betti
end
#%%
function plot_and_save_bettis(bettis,
plot_title::String,
results_path::String;
file_name = "",
extension = ".png",
do_save = true,
do_normalise = true,
min_dim = 0,
max_dim = 3,
legend_on = true,
kwargs...)
"""
plot_and_save_bettis(eirene_results, plot_title::String,
results_path::String; extension = ".png",
data_size::String="", do_save=true,
extend_title=true, do_normalise=true, max_dim=3,
legend_on=true)
Plot Betti curves from 0 up to `max_dim` using `eirene_results` from Eirene library and
returns handler for figure. Optionally, if `do_save` is set, saves the figure
or if `do_normalise` is set, sets the steps range to be normalised to the
horizontal axis maximal value.
"""
bettis = get_bettis(eirene_results, max_dim)
if do_normalise
bettis = normalise_bettis(bettis)
end
plot_ref =
plot_bettis(bettis, plot_title, legend_on = legend_on, min_dim = min_dim, kwargs...)
if do_save
if isempty(file_name)
file_name = plot_title * extension
elseif isempty(findall(x -> x == extension[2:end], split(file_name, ".")))
#check for the extension in file name
file_name *= extension
end
save_figure_with_params(
plot_ref,
results_path;
extension = extension,
prefix = split(file_name, ".")[1],
)
end
return plot_ref
end
# TODO merge functions for getting betti curves
# Original function returns 2 different types of betti curves. If no default
# value parameters is given, it returns vector of matrices. If num of steps is
# given, then it return matrix maxdim x numsteps.
# """
# bettis_eirene(matr, maxdim; mintime=-Inf, maxtime=Inf, numofsteps=Inf, mindim=1)
#
# Takes the `matr` and computes Betti curves up to `maxdim`. Return matrix only
# with betti curve values
#
#
# Function taken from: https://github.com/alexyarosh/hyperbolic
# """
#%%
@deprecate bettis_eirene(matr, maxdim; mintime = -Inf, maxtime = Inf, numofsteps = Inf, mindim = 1) get_bettis(results_eirene, max_dim; min_dim = 1)
#%%
function get_bettis_from_image(img_name,
plot_params;
file_path = "",
plot_heatmaps = true,
save_heatmaps = false,
plot_betti_figrues = true)
"""
function get_bettis_from_image(img_name)
Computes Betti curves for the image file indicated by @img_name. If the image is
not symmetric, then it is the elements below diagonal are copied over the
elmenents above the diagonal.
"""
file_n = split(img_name, ".")[1]
img1_gray = Gray.(load(file_path * img_name))
img_size = size(img1_gray)
C_ij = Float64.(img1_gray)
if !issymmetric(C_ij)
img1_gray = symmetrize_image(img1_gray)
C_ij = Float64.(img1_gray)
end
img_size = size(C_ij, 1)
# C_ij =-C_ij
# C_ij .+= 1
# ==============================================================================
# =============================== Ordered matrix ===============================
if size(C_ij, 1) > 80
@warn "Running Eirene for big matrix: " img_size
@warn "Eirene may have trobules with big matrices/images."
end
ordered_matrix = get_ordered_matrix(C_ij; assing_same_values = false)
# ==============================================================================
# ============================ Persistance homology ============================
C = eirene(ordered_matrix, maxdim = 3, model = "vr")
# ==============================================================================
# ================================ Plot results ================================
# TODO separate plotting from processing
if plot_heatmaps
full_ordered_matrix = get_ordered_matrix(C_ij; assing_same_values = false)
heat_map2 = plot_square_heatmap(
full_ordered_matrix,
10,
img_size;
plt_title = "Order matrix of $(file_n)",
plot_params = plot_params,
)
if save_heatmaps
heatm_details = "_heatmap_$(file_n)"
savefig(heat_map2, heatmaps_path * "ordering" * heatm_details)
end
end
if plot_betti_figrues
plot_title = "Betti curves of $(file_n), size=$(img_size) "
figure_name = "betti_$(file_n)_n$(img_size)"
ref = plot_and_save_bettis(C,
plot_title,
figure_path,;
file_name = figure_name,
plot_params = plot_params,
do_save = false,
extend_title = false,
do_normalise = false,
max_dim = 3,
legend_on = true,
min_dim = 1)
end
display(img1_gray)
display(heat_map2)
display(ref)
end
# ===============================================
@deprecate get_bettis_from_image2(img_name;file_path = "",plot_heatmaps = true, save_heatmaps = false, plot_betti_figrues = true) get_bettis_from_image(img_name, plot_params; file_path = "", plot_heatmaps = true, save_heatmaps = false, plot_betti_figrues = true)
@deprecate plot_and_save_bettis2(eirene_results, plot_title::String, results_path::String; file_name = "", extension = ".png", data_size::String = "", do_save = true, extend_title = true, do_normalise = true, min_dim = 0, max_dim = 3, legend_on = true) plot_and_save_bettis(bettis, plot_title::String, results_path::String; file_name = "", extension = ".png", do_save = true, do_normalise = true, min_dim = 0, max_dim = 3, legend_on = true, kwargs...)
#%%
function get_and_plot_bettis(eirene_results;
max_dim = 3,
min_dim = 1,
plot_title = "",
legend_on = false)
bettis = get_bettis(eirene_results, max_dim)
norm_bettis = normalise_bettis(bettis)
plot_ref =
plot_bettis2(norm_bettis, plot_title, legend_on = legend_on, min_dim = min_dim)
# display(plot_ref)
return plot_ref
end
#%%
function lower_ordmat_resolution(ordered_matrix::Array, total_bins::Int)
"""
lower_ordmat_resolution(ordered_matrix::Array, total_bins::Int)
Takes ordered matrix 'input_matrix' and reduces the resolution of values in the
matrix into 'total_bins' bins.
"""
new_ordered_matrix = zeros(size(ordered_matrix))
max_val = findmax(ordered_matrix)[1]
min_val = findmin(ordered_matrix)[1]
bin_step = max_val ÷ total_bins
old_bins = min_val:bin_step:max_val
for bin = 1:total_bins
@debug "First step threshold is $(old_bins[bin])"
indices = findall(x -> (x >= old_bins[bin]), ordered_matrix)
new_ordered_matrix[indices] .= bin - 1
end
@debug "Max_val in new matrix is " findmax(new_ordered_matrix)
@debug "And should be " total_bins - 1
return new_ordered_matrix
end
#%%
function average_bettis(bettis_matrix::Matrix; up_factor = 8)
"""
average_bettis(bettis_matrix; up_factor=8)
Takes the average values of betti curves stored in 'bettis_matrix'.
'bettis_matrix' consist of different simulations(first index of the matrix),
different ranks (third index of the matrix). Second index of the matrices
(saples) may vary accross many simulations and for this reason, all betti curves
are upsampled by a factor of 'upsample_factor' and then the average for every
dimension is computed.
"""
bettis_matrix_backup = copy(bettis_matrix)
simulations = size(bettis_matrix, 1)
dimensions = size(bettis_matrix[1], 1)
max_samples = 0
for k = 1:simulations
# global max_samples
current_len = length(bettis_matrix[k][1][:, 1])
if max_samples < current_len
max_samples = current_len
end
end
bettis_size = size(bettis_matrix)
total_upsamples = (max_samples - 1) * up_factor + 1
x_resampled = range(0, 1, step = total_upsamples)
avg_bettis = zeros(total_upsamples, dimensions)
std_bettis = copy(avg_bettis)
resampled_bettis = zeros(simulations, total_upsamples, dimensions)
# resample betti curves
for simulation = 1:simulations, betti = 1:dimensions
resampled_bettis[simulation, :, betti] =
upsample_vector2(bettis_matrix[simulation][betti][:, 2], total_upsamples)
end
# average and std Betti
for dimension = 1:dimensions
avg_bettis[:, dimension] = mean(resampled_bettis[:, :, dimension], dims = 1)
std_bettis[:, dimension] = mean(resampled_bettis[:, :, dimension], dims = 1)
end
return avg_bettis, std_bettis
end
#%%
function upsample_vector2(input_vector, total_upsamples)
total_orig_samples = size(input_vector, 1) - 1
x_vals = range(0, 1, length = total_orig_samples + 1)
spl = Spline1D(x_vals, input_vector)
x_upsampled = range(0, 1, length = total_upsamples)
y_upsampled = spl(x_upsampled)
# ref = plot(range(0, 1, length=total_orig_samples), input_vector);
# plot!(x_vals, y_upsampled);
# display(ref)
return y_upsampled
end
#%%
function upsample_vector(input_vector; upsample_factor::Int = 8)
"""
upsample_vector(input_vector; upsample_factor::Int=8)
Takes an 'input_vector' and returns a vector which has 'upsample_factor' many
times more samples. New samples are interpolated with 'spl' function from
'Dierckx' package.
"""
total_orig_samples = size(input_vector, 1) - 1
total_samples = upsample_factor * total_orig_samples + 1
x_vals = range(0, 1, length = total_orig_samples + 1)
spl = Spline1D(x_vals, input_vector)
x_upsampled = range(0, 1, length = total_samples)
y_upsampled = spl(x_upsampled)
# ref = plot(range(0, 1, length=total_orig_samples), input_vector);
# plot!(x_vals, y_upsampled);
# display(ref)
return y_upsampled
end
# =========--=======-========-==========-=======-
# From bettis areas
# Area under Betti curve functions
#%%
function get_area_under_betti_curve(betti_curves::Union{Matrix{Float64}, Array{Array{Float64,2}}};do_normalised::Bool=false)
"""
get_area_under_betti_curve(betti_curves, min_dim, max_dim)
Computes the area under Betti curves stored in 'betti_curves', where each row is
a Betti curve and each column is a value.
"""
#TODO check this part
if size(betti_curves,2) < 2
bettis_vector = vectorize_bettis(betti_curves)
else
bettis_vector = betti_curves
end
# @info sum(bettis_vector, dims=1)
bettis_area = sum(bettis_vector, dims=1)
if do_normalised
total_steps = size(bettis_vector,1)
bettis_area ./= total_steps
end
# @info bettis_area
return bettis_area
end
# function get_area_under_betti_curve(C, min_dim, max_dim)
# """
# get_area_under_betti_curve(C, min_dim, max_dim)
#
# Computes the Betti curves and returns their area under curve.
# """
# all_bettis = get_bettis(C,max_dim, min_dim=min_dim)
# bettis_vector = hcat([all_bettis[k][:,2] for k=min_dim:max_dim]...)
# # @info sum(bettis_vector, dims=1)
#
#
# total_steps = size(bettis_vector,1)
#
# bettis_area = sum(bettis_vector, dims=1) ./ total_steps
# # @info bettis_area
# return bettis_area
# end
#%%
function get_dataset_bettis_areas(dataset; min_dim::Integer=1, max_dim::Integer=3, return_matrix::Bool=true)
"""
get_dataset_bettis_areas(dataset; min_dim::Integer=1, max_dim::Integer=3, return_matrix::Bool=true)
Computes topology of every matrix in dataset, computes Betti curves for dimensions
min_dim up to max_dim and returns vector (or matrix) of areas under Betti curves.
"""
areas_vector = Array[]
for data = dataset
@info "Computing topology."
C = eirene(data, maxdim=max_dim,)
matrix_bettis = get_bettis(C,max_dim, min_dim=min_dim)
push!(areas_vector, get_area_under_betti_curve(matrix_bettis))
end
if return_matrix
return vcat([areas_vector[k] for k=1:10]...)
else
return areas_vector
end
end
# struct TopologyData
# min_dim::Integer
# max_dim::Integer
#
# do_normalise::Bool=true
#
# betti_curves
# normed_bettis
# betti_areas::Matrix{Int}
#
# # Constructor for input data
# function TopologyData(my_matrix::Matrix, max_dim::Int; min_dim::Int, do_normalise::Bool=true)
# min_dim = min_dim
# max_dim = max_dim
#
# @info "Computing topology for maxdim =" max_dim
# C = eirene(my_matrix, maxdim=max_dim)
# betti_curves = get_bettis(C, max_dim, min_dim=min_dim)
# normed_bettis = normalise_bettis(betti_curves)
# betti_areas = get_area_under_betti_curve(betti_curves; do_normalised=do_normalise)
# end
# end
#%%
function get_dataset_topology(dataset;
min_dim::Integer=1,
max_dim::Integer=3,
get_curves::Bool=true,
get_areas::Bool=true,
get_persistence_diagrams::Bool=true,
do_normalise::Bool=true)
topology_set = TopologyData[]
for some_matrix in dataset
resulting_topology = TopologyData(some_matrix, max_dim, min_dim=min_dim, do_normalise=do_normalise)
push!(topology_set, resulting_topology)
end
return topology_set
end
#%%
function get_area_boxes(areas_matrix, min_dim::Integer, max_dim::Integer)
"""
get_area_boxes(areas_matrix, min_dim::Integer, max_dim::Integer)
Plots the boxplot of area under betti curves.
"""
bplot = StatsPlots.boxplot()
data_colors = get_bettis_color_palete()
for (index, value) in enumerate(min_dim:max_dim)
StatsPlots.boxplot!(bplot, areas_matrix[:,index], labels="β$(value)", color=data_colors[value])
end
return bplot
end
function get_bettis_collection_from_matrices(ordered_matrices_collection; max_dim::Int=3, min_dim::Int=1)
bettis_collection = Array[]
for matrix = ordered_matrices_collection
@debug "Computing Bettis..."
eirene_geom = eirene(matrix,maxdim=max_B_dim,model="vr")
bettis = reshape_bettis(get_bettis(eirene_geom, max_B_dim))
push!(bettis_collection, bettis)
end
return bettis_collection
end
# =========--=======-========-==========-=======-
# Code from Points substitution:
# Compute series of betti curves
# function get_bettis_collection(ordered_matrices_collection; max_B_dim=3)
# bettis_collection = Array[]
#
# for matrix = ordered_matrices_collection
# @debug "Computing Bettis..."
# eirene_geom = eirene(matrix,maxdim=max_B_dim,model="vr")
#
# bettis = reshape_bettis(get_bettis(eirene_geom, max_B_dim))
# push!(bettis_collection, bettis)
# end
#
# return bettis_collection
# end
#
# # Plot series of betti curves with their heatmaps
# function reshape_bettis(bettis)
# bettis_count = size(bettis,1)
# output_betti = zeros(size(bettis[1],1), bettis_count)
#
# for betti = 1:bettis_count
# output_betti[:,betti] = bettis[betti][:,2]
# end
# return output_betti
# end
#
# function get_ord_mat_collection(matrix_collection)
# mat_size = size(matrix_collection[1],1)
# ordered_mat_coll = [zeros(Int, mat_size,mat_size) for k=1:length(matrix_collection)]
#
# size(matrix_collection)
# for matrix = 1:length(matrix_collection)
# ordered_mat_coll[matrix] = Int.(get_ordered_matrix(matrix_collection[matrix]))
# end
# return ordered_mat_coll
# end
#
#
#
#
#
# function print_hmap_with_bettis(ordered_matrices_collection, bettis_collection,
# plot_data::PlottingData)
# num_plots = size(ordered_matrices_collection,1)
# sources = 1:(plot_data.src_pts_number)
# targets = 1:(plot_data.trgt_pts_number)
# plot_set = Any[]
#
# max_betti = get_max_betti_from_collection(bettis_collection;dim=1)
#
# index = 1
# for src = 1:size(sources,1), trgt = 1:size(targets,1)
# # index = src * trgt
# ordered_geom_gr = ordered_matrices_collection[index]
# bettis = bettis_collection[index]
# title_hmap = "trgt:$(targets[trgt])_src:$(sources[src])_r:$(rank(ordered_geom_gr))"
# title_bettis = "gr_trg=$(targets[trgt])_src=$(sources[src])_steps=$(size(bettis,1))"
# push!(plot_set, make_hm_and_betti_plot(ordered_geom_gr, bettis, title_hmap, title_bettis, max_betti))
# index +=1
# end
#
# return plot_set
# end
#
# function make_hm_and_betti_plot(ordered_geom_gr, bettis, title_hmap, title_bettis, max_betti)
# # @debug "src" src
# # @debug "trgt" trgt
# hmap_plot = plot_square_heatmap(ordered_geom_gr, 10,size(ordered_geom_gr,1);plt_title = title_hmap)
# plot!(yflip = true,)
#
# bettis_plot_ref = plot(title=title_bettis);
# max_dim = size(bettis,2)
# for p = 1:max_dim
# x_vals = collect(1:size(bettis[:,1],1))./size(bettis[:,1])
#
# plot!(x_vals, bettis[:,p], label="\\beta_"*string(p));
# plot!(legend=true, )
# end
#
# plot!(ylim=(0,max_betti))
# plot!(xlim=(0,1))
# ylabel!("Number of cycles")
# xlabel!("Steps")
#
# final_plot = plot(hmap_plot, bettis_plot_ref, layout = 2,
# top_margin=2mm,
# left_margin=0mm,
# bottom_margin=2mm,
# size=(600,300))
# display(final_plot)
# return final_plot
# end
#
# # TODO BUG: substitution does not work- all the plots are the same
# function main_generation1()
# mat_size = 6
# dim = 80
# src_pts_number = 1
# trgt_pts_number = 2
# trgt_steps = 0
#
# src_points, trgt_points =
# get_replacing_points(mat_size, src_pts_number, trgt_pts_number)
#
# matrix_collection =
# get_matrix_collection(mat_size, dim, src_points, trgt_points; trgt_step=trgt_steps)
#
# ordered_matrices_collection = get_ord_mat_collection(matrix_collection)
#
# bettis_collection = get_bettis_collection(ordered_matrices_collection)
#
#
# plot_data = PlottingData(mat_size, dim, src_pts_number, trgt_pts_number, src_points, trgt_points, trgt_steps)
# # plot_data = PlottingData2(mat_size , dim, )
#
# plotting_data = print_hmap_with_bettis(ordered_matrices_collection,
# bettis_collection, plot_data)
# end
#
#
# function get_geom_matrix(mat_size, dim)
# # TODO change the matrix collection shape to be a matrix, not a vector
# point_cloud = generate_random_point_cloud(mat_size, dim)
# matrix_collection = generate_geometric_matrix(point_cloud)
# # matrix_collection = get_ordered_matrix(matrix_collection; assing_same_values=true)
#
# return matrix_collection
# end
#
# function get_rand_matrix(mat_size, dim)
# matrix_collection = generate_random_matrix(mat_size)
# matrix_collection = get_ordered_matrix(matrix_collection; assing_same_values=true)
#
# return matrix_collection
# end
#
# # TODO Analyse zero point behaviour
# function get_dist_mat_collection(dist_matrix, src_points, trgt_points, trgt_steps; do_ordering=false)
# dist_matrix_backup = copy(dist_matrix)
# mat_size = size(dist_matrix,1)
# src_points_num = size(src_points,1)
# trgt_points_num = size(trgt_points,1)
# # ordered_mat_coll = [zeros(Int, mat_size,mat_size) for k=1:(src_points_num*trgt_points_num)]
# ordered_mat_coll = Array[]
#
# swapping_iterator = 0
#
# for srcs = 1:src_points_num
# # replacement_row = get_row(dist_matrix, src_points[srcs])
#
# for target = 1:trgt_points_num
# @debug "src:" src_points[srcs]
# @debug "trgt:" trgt_points[target, srcs]
# replacement_row = get_row(dist_matrix_backup, src_points[srcs])
# # dist_matrix_backup .=
# set_row!(dist_matrix_backup, trgt_points[target, srcs], replacement_row)
# # ordered_mat_coll[srcs * target] = copy(dist_matrix_backup)
# if do_ordering
# swap_rows!(dist_matrix_backup, trgt_points[target, srcs], mat_size-swapping_iterator)
# swapping_iterator +=1
# end
# push!(ordered_mat_coll, copy(dist_matrix_backup))
# end
# end
#
# return ordered_mat_coll
# end
#
# function get_ordered_set(distance_matrices_collection)
# result = copy(distance_matrices_collection)
#
# for matrix = 1:size(distance_matrices_collection,1)
# result[matrix] = get_ordered_matrix(distance_matrices_collection[matrix];assing_same_values=true )
# end
# return result
# end
#
# function matrix_analysis(test_data::PlottingData;generation_function=get_geom_matrix)
# mat_size = test_data.mat_size
# dim = test_data.dim
# src_pts_number = test_data.src_pts_number
# trgt_pts_number = test_data.trgt_pts_number
# trgt_steps = 0
#
# src_points, trgt_points = get_replacing_points(mat_size, src_pts_number, trgt_pts_number)
# distance_matrix = generation_function(mat_size, dim)
#
# distance_matrices_collection = get_dist_mat_collection(distance_matrix, src_points, trgt_points, trgt_steps)
# ordered_matrices_collection = get_ordered_set(distance_matrices_collection)
# bettis_collection = get_bettis_collection(ordered_matrices_collection)
#
# plot_data = PlottingData(mat_size, dim, src_pts_number, trgt_pts_number, src_points, trgt_points, trgt_steps)
#
# plots_set = print_hmap_with_bettis(ordered_matrices_collection,
# bettis_collection, plot_data)
#
#
# return distance_matrices_collection, ordered_matrices_collection, bettis_collection, plot_data, plots_set
# end
#%%
# This does not belong here
function multiscale_matrix_testing(sample_space_dims = 3,
maxsim = 5,
min_B_dim = 1,
max_B_dim = 3,
size_start = 10,
size_step = 5,
size_stop = 50;
do_random = true,
control_saving = false,
perform_eavl = false)
"""
multiscale_matrix_testing(sample_space_dims = 3,
maxsim=5,
min_B_dim = 1,
max_B_dim = 3,
size_start = 10,
size_step = 5,
size_stop = 80; do_random=true)
Function for testing the average number of cycles from geometric and random
matrices.
It is possible to save intermidiate results- for that, @control_saving must be
set true.
Performance of computation of Betti curves can be monitored, if the
@perform_eavl is set too true. Bydefault, it is set to false.
"""
num_of_bettis = length(collect(min_B_dim:max_B_dim))
if length(sample_space_dims) > 1
@warn "Can not do random processing for multiple dimensions"
do_random = false
end
geom_mat_results = Any[]
if do_random
rand_mat_results = Any[]
result_list = [geom_mat_results, rand_mat_results]
else
result_list = [geom_mat_results]
end
for sample_space_dim in sample_space_dims
if !do_random
@info "Sampling space size: " sample_space_dim
end
repetitions = size_start:size_step:size_stop
for space_samples in repetitions
@info "Generating data for: " space_samples
# ==========================================
# ============= Generate data ==============
# ===
# Generate random matrix
if do_random
symm_mat_rand = [generate_random_matrix(space_samples) for i = 1:maxsim]
ordered_mat_rand = [
get_ordered_matrix(symm_mat_rand[i]; assing_same_values = false)
for i = 1:maxsim
]
end
# ===
# Generate geometric matrix
pts_rand = [
generate_random_point_cloud(sample_space_dim, space_samples)
for i = 1:maxsim
]
symm_mat_geom = [generate_geometric_matrix(pts_rand[i]') for i = 1:maxsim]
ordered_mat_geom = [
get_ordered_matrix(symm_mat_geom[i]; assign_same_values = false)
for i = 1:maxsim
]
# ======================================================================
# ========================= Do the Betti analysis ======================
if do_random
set = [ordered_mat_geom, ordered_mat_rand]
else
set = [ordered_mat_geom]
end
for matrix_set in set
@debug("Betti analysis!")
# ===
# Generate bettis
many_bettis = Array[]
if perform_eavl
many_timings = Float64[]
many_bytes = Float64[]
many_gctime = Float64[]
many_memallocs = Base.GC_Diff[]
end
for i = 1:maxsim
if i % 10 == 0
@info "Computing Bettis for: " i
end
if perform_eavl
results, timing, bytes, gctime, memallocs = @timed bettis_eirene(
matrix_set[i],
max_B_dim,
mindim = min_B_dim,
)
push!(many_bettis, results)
push!(many_timings, timing)
push!(many_bytes, bytes)
push!(many_gctime, gctime)
push!(many_memallocs, memallocs)
else
push!(
many_bettis,
bettis_eirene(matrix_set[i], max_B_dim, mindim = min_B_dim),
)
end
end
# ===
# Get maximal number of cycles from each Betti from simulations
max_cycles = zeros(maxsim, max_B_dim)
for i = 1:maxsim, betti_dim = 1:max_B_dim
@debug("\tFindmax in bettis")
max_cycles[i, betti_dim] = findmax(many_bettis[i][:, betti_dim])[1]
end
# ===
# Get the statistics
avg_cycles = zeros(1, length(min_B_dim:max_B_dim))
std_cycles = zeros(1, length(min_B_dim:max_B_dim))
k = 1
for betti_dim = min_B_dim:max_B_dim
avg_cycles[k] = mean(max_cycles[:, betti_dim])
std_cycles[k] = std(max_cycles[:, betti_dim])
k += 1
end
# ===
# Put results into dictionary
betti_statistics = Dict()
if matrix_set == ordered_mat_geom
@debug("Saving ordered")
betti_statistics["matrix_type"] = "ordered"
betti_statistics["space_dim"] = sample_space_dim
result_list = geom_mat_results
else
@debug("Saving radom")
betti_statistics["matrix_type"] = "random"
result_list = rand_mat_results
end
betti_statistics["space_samples"] = space_samples
betti_statistics["simualtions"] = maxsim
betti_statistics["min_betti_dim"] = min_B_dim
betti_statistics["max_betti_dim"] = max_B_dim
betti_statistics["avg_cycles"] = avg_cycles
betti_statistics["std_cycles"] = std_cycles
if perform_eavl
betti_statistics["many_timings"] = many_timings
betti_statistics["many_bytes"] = many_bytes
betti_statistics["many_gctime"] = many_gctime
betti_statistics["many_memallocs"] = many_memallocs
end
push!(result_list, betti_statistics)
end # matrix type loop
@debug("===============")
if control_saving
if do_random
save(
"multiscale_matrix_testing_$(space_samples)_$(sample_space_dim).jld",
"rand_mat_results",
rand_mat_results,
"geom_mat_results",
geom_mat_results,
)
else
save(
"multiscale_matrix_testing_dimension_$(space_samples)_$(sample_space_dim).jld",
"geom_mat_results",
geom_mat_results,
)
end
end
end # matrix_size_loop
end # sampled space dimension
if do_random
return geom_mat_results, rand_mat_results
else
return geom_mat_results
end
end
# function plot_betti_numbers(betti_numbers, edge_density, title="Geometric matrix"; stop=0.6)
# """
# Plots Betti curves. The betti numbers should be obtained with the clique-top
# library.
# """
# p1 = plot(edge_density, betti_numbers[:,1], label="beta_0", title=title, legend=:topleft) #, ylims = (0,maxy)
# plot!(edge_density, betti_numbers[:,2], label="beta_1")
# if size(betti_numbers,2)>2
# plot!(edge_density, betti_numbers[:,3], label="beta_2")
# end
#
# return p1
# end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 388 | """
check_existing_dir(dir_path::String)
Checks if the directory under `dir_path` exists. If not, throws IOError
"""
function check_existing_dir(dir_path::String)
if !isdir(dir_path)
@warn "Folder $(data_path) does not exists in current directory."
@warn "Terminating execution."
throw(ErrorException("Can nor find folder \""*dir_path*"\"."))
end
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 4819 | using LinearAlgebra
# integrate sinh^(n)(ax) from 0 to r
function integrate_sinh(n; r=1.0, a=1.0)
if n==0
return r
elseif n==1
return (cosh(a*r)-1)/a
else
return (sinh(a*r)^(n-1))*cosh(a*r)/(a*n) - (n-1)/n * integrate_sinh(n-2,r=r, a=a)
end
end
function hyp_radial_density(r, d; curvature=-1.0, radius=1.0)
# k = 1/(curvature^2)
k = 1.0
hyp_r = (sinh(r/k))^(d-1)/integrate_sinh(d-1,r=radius, a=radius/k)
return hyp_r
end
function euc_radial_density(r, d; radius=1.0)
return d*(r^(d-1))/radius^d
end
# rejection sampling n=numofpts points from density dens, where the argument lies between 0 and maxval
function rejection_sampling(dens::Function, maxval,numofpts=1)
max_val = dens(maxval)
iter = 1
rands = Array{Float64,1}(undef, numofpts)
while iter <= numofpts
x = rand()*maxval
val = dens(x)
u = rand()
if u*max_val < val
rands[iter] = x
iter+=1
end
end
return rands
end
function sample_hyp_rad(d, numofpts=1; curvature=-1.0, radius=1.0)
rands = rejection_sampling(x->hyp_radial_density(x,d,curvature=curvature, radius=radius), radius,numofpts)
# ...radius within the Poincare ball
euc_rands = map(x->tanh(x/2.0),rands)
return euc_rands
end
function sample_euc_rad(d, numofpts=1; radius=1.0)
rands = rejection_sampling(x->euc_radial_density(x,d,radius=radius), radius, numofpts)
return rands
end
function sample_sph(d, numofpts=1; curvature=1.0)
rands = []
i=0
while i<=numofpts
vec = randn(d+1)
if vec[d+1]>0
push!(rands, normalize(vec))
i+=1
end
end
return rands
end
function sample_sphere(d, numofpts=1)
vecs = randn(d, numofpts)
rands = []
for i=1:numofpts
push!(rands, normalize(vecs[:,i]))
end
return rands
end
function sample_hyp(d, numofpts=1; radius=1.0, curvature=-1)
sphere_pts = sample_sphere(d,numofpts)
radii = sample_hyp_rad(d, numofpts, radius=radius, curvature=curvature)
ball_pts = [radii[i]*sphere_pts[i] for i=1:numofpts]
return ball_pts
end
function sample_euc(d, numofpts=1; radius=1.0)
sphere_pts = sample_sphere(d,numofpts)
radii = sample_euc_rad(d, numofpts, radius=radius)
ball_pts = [radii[i]*sphere_pts[i] for i=1:numofpts]
return ball_pts
end
function sample_ball(d, numofpts=1; radius=1.0, curvature=0.0)
sphere_pts = sample_sphere(d,numofpts)
if curvature < 0
radii = sample_hyp_rad(d, numofpts, radius=radius, curvature=curvature)
ball_pts = [radii[i]*sphere_pts[i] for i=1:numofpts]
elseif curvature == 0.0
radii = sample_euc_rad(d, numofpts, radius=radius)
ball_pts = [radii[i]*sphere_pts[i] for i=1:numofpts]
elseif curvature > 0
ball_pts = sample_sph(d, numofpts, curvature=curvature)
end
end
function hyp_distance(pts; curvature=-1.0)
distances = zeros(length(pts), length(pts))
for i=1:length(pts)
for j=1:i-1
nx = 1-(norm(pts[i]))^2
ny = 1-(norm(pts[j]))^2
delta = 2 * norm(pts[i]-pts[j])^2/(nx*ny)
distances[i,j] = acosh(1+delta)
distances[j,i] = distances[i,j]
end
end
return distances
end
function euc_distance(pts)
distances = zeros(length(pts), length(pts))
for i=1:length(pts)
for j=1:i-1
distances[i,j] = norm(pts[i]-pts[j])
distances[j,i] = distances[i,j]
end
end
return distances
end
function sph_distance(pts; curvature=1.0)
distances = zeros(length(pts), length(pts))
for i=1:length(pts)
for j=1:i-1
distances[i,j] = acos(dot(pts[i],pts[j]))
distances[j,i] = distances[i,j]
end
end
return distances
end
function distance_matrix(pts; curvature=0.0)
if curvature < 0
return hyp_distance(pts, curvature=curvature)
elseif curvature == 0
return euc_distance(pts)
elseif curvature > 0
return sph_distance(pts, curvature=curvature)
end
end
function to_density(matr)
dens_matr = zeros(size(matr,1), size(matr,2))
n = size(matr)[1]
all_entries = sort(setdiff(unique(matr), 0.0))
total = binomial(n,2)
for i=1:n
for j=i+1:n
dens_matr[i,j] = (findfirst(x->x==matr[i,j], all_entries))/total
dens_matr[j,i] = dens_matr[i,j]
end
end
return dens_matr
end
function to_density!(matr)
matr = to_density(matr)
return matr
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 20266 | using Statistics
using Combinatorics
# using ImageFiltering
"""
rotate_img_around_center(img, angle = 5pi/6)
Function rotates a single image (or a frame) around the center of the image by
@angle radians.
"""
function rotate_img_around_center(img, angle = 5pi/6)
θ = angle
rot = recenter(RotMatrix(θ), [size(img)...] .÷ 2) # a rotation around the center
x_translation = 0
y_translation = 0
tform = rot ∘ Translation(y_translation, x_translation)
img2 = warp(img, rot, axes(img))
return img2
end
"""
get_local_gradients(video_array, centers, sub_img_size)
Computes the gradients in the subimage, takes the mean of sum of absolute
values of both hotizontal and vertical gradients as a representative of a
subimage.
"""
function get_local_gradients(video_array, centers, sub_img_size)
@debug "Entering get_local_gradients"
half_size = ceil(Int,(sub_img_size-1)/2)
half_range = half_size
h, w, len = get_video_dimension(video_array)
extracted_pixels = zeros(sub_img_size, sub_img_size, len)
@debug "starting frame procesing"
for frame = 1:len
img = video_array[frame]
img_grad = imgradients(img, KernelFactors.ando3, "replicate")
img_grad_abs = map(abs, img_grad[1]) + map(abs, img_grad[2])
for index_x = 1:size(centers,2)
c_x = centers[2, index_x]
for index_y = 1:size(centers,2)
c_y = centers[1, index_y]
sub_img = img_grad_abs[(c_x-half_size):(c_x+half_size),
(c_y-half_size):(c_y+half_size)]
extracted_pixels[index_x, index_y, frame] =mean(sub_img)
end
end
# @debug "Next frame" frame
end
return extracted_pixels
end
"""
get_img_gradient(img)
Computes the gradients in the `img`.
"""
function get_img_gradient(img)
@debug "Entering get_local_gradients"
img_grad = imgradients(img, KernelFactors.ando3, "replicate")
grad_1 = img_grad[1] .+ abs(findmin(img_grad[1])[1])
grad_1 ./= findmax(grad_1)[1]
grad_2 = img_grad[2] .+ abs(findmin(img_grad[2])[1])
grad_2 ./= findmax(grad_2)[1]
grad_sum = grad_1 + grad_2
grad_sum .+= abs(findmin(grad_sum)[1])
grad_sum ./= findmax(grad_sum)[1]
return grad_sum
end
"""
get_local_img_correlations(img, centers, sub_img_size, shift;
with_gradient=false)
Computes the correlation between the subimages and subimages shifted by values
from range -`shift`:`shift` and returns array of size
length(`centers`) x length(`centers`).
Each of the subimage is center around values stored in @centers
"""
function get_local_img_correlations(img, centers, sub_img_size::Int;
with_gradient=false)
# TODO BUG- function is not workig for even numbers
half_size = ceil(Int,(sub_img_size-1)/2)
half_range = half_size#
h, w = size(img)
extracted_pixels = zeros(sub_img_size, sub_img_size)
local_correlation = zeros(size(centers,1))
if with_gradient
img = get_img_gradient(img)
end
position = 1;
for index = centers
c_x = index[1]
c_y = index[2]
c_x_range = (c_x-half_range):(c_x+half_range)
c_y_range = (c_y-half_range):(c_y+half_range)
subimage = img[c_x_range,c_y_range]
center = img[c_x_range, c_y_range]
corelation = center .* subimage
corelation = sum(corelation)
local_correlation[position] += corelation
local_correlation[position] /= 256*(sub_img_size^2)^2
position += 1;
end
return local_correlation
end
"""
get_local_img_correlations(img,centers, masks)
Takes `img` and computes crosscorrelation with set of `masks` around the
`centers`. Crosscorrelation is computed as convolution of the mask and the area
around coordinates stored in `centres`.
"""
function get_local_img_correlations(img, centers, masks::Vector; with_gradient=false)
masks_num = length(masks)
sub_img_size = size(masks[1],1)
# half_size = ceil(Int,(sub_img_size-1)/2)
half_size = (sub_img_size)÷2
half_range = half_size
h, w = size(img)
local_correlation = zeros(masks_num, size(centers,1) )
index = centers[1]
masks_num = length(masks)
if with_gradient
img = get_img_gradient(img)
end
# position = 1;
# for index = centers
for pos = 1:length(centers)
# global position
index = centers[pos]
c_x = index[1]
c_y = index[2]
c_x_range = (c_x-half_range):(c_x+half_range)
c_y_range = (c_y-half_range):(c_y+half_range)
center = img[c_x_range, c_y_range]
# mask_pos = 1
# for mask in masks
for mask_pos = 1:length(masks)
mask = masks[mask_pos]
corelation = center .* mask
corelation = sum(corelation)
local_correlation[mask_pos, pos] += corelation
local_correlation[mask_pos, pos] /= (sub_img_size^2)
# local_correlation[position, mask_pos ] = sum(imfilter(center, mask))/(sub_img_size^2)
# mask_pos +=1
end
# position += 1;
end
return local_correlation
end
"""
extract_pixels_from_img(img, indicies_set, video_dim_tuple)
Takes every frame from @video_array and extracts pixels which indicies are in
@indicies_set, thus creating video with only chosen indicies.
"""
function extract_pixels_from_img(img, indicies_set, video_dim_tuple)
rows = size(indicies_set,2)
columns = size(indicies_set,2)
video_length = video_dim_tuple[3]
extracted_pixels = zeros(rows, columns, video_length)
extracted_pixels[:,:,] =
img[indicies_set[1,:],indicies_set[2,:]]
return extracted_pixels
end
"""
Returns evenly distributed centers of size `image_size`
"""
function get_local_img_centers(points_per_dim, img_size, shift=0, sub_img_size=0 )
# TODO Applied teproray solution here, so it works only for local gradients
# start = 0
# (points_per_dim>shift) ? start_ind = ceil(Int, points_per_dim/2)+ shift :
# start=shift
start_ind = ceil(Int, sub_img_size/2)
min_va, = findmin(img_size)
last_ind = min_va - start_ind
set = broadcast(floor, Int, range(start_ind, step=sub_img_size, stop=last_ind))
num_indexes = size(set,1)
centers = Any[]
for row = 1:num_indexes
for column = 1:num_indexes
push!(centers, CartesianIndex(set[row], set[column]))
end
end
return centers
end
"""
get_img_local_centers(img_size, sub_img_size=10)
Tiles the image of size @img_size into square subimages of size @sub_img_size
and returns vector with CartesianIndex coordinates of the subimages centre in
original image.
By default, takes smaller value from @img_size and then divides it by
@sub_img_size. Resulting value will be the number of returned subimages per
dimension. If @use_square is set to false, then evry dimension is treated
separately, resulting in rectangular grid.
It is possible to set overlap of the tiles with @overlap parameter. By default
it is set to zero, but can be any pixel value smaller that @sub_img_size. If
@overlap is set to value in range (0,1], a fraction of @sub_img_size is used.
"""
function get_img_local_centers(img_size, sub_img_size=10; use_square=true,
overlap=0)
@assert sub_img_size <= findmin(img_size)[1] "@sub_img_size is bigger than image!"
@assert sub_img_size > 0 "sub_img_size must be positive number"
@assert overlap<=sub_img_size "The overlap is biger than subimage size!"
@assert overlap >= 0 "overlap must be positive"
centers = CartesianIndex[]
start_ind = ceil(Int, sub_img_size/2)
if 2*start_ind == sub_img_size
start_ind +=1
end
if overlap>0 && overlap<1
overlap = floor(Int, sub_img_size*overlap)
end
if use_square
size_v = findmin(img_size)[1]
size_h = findmin(img_size)[1]
else
size_v = img_size[1]
size_h = img_size[2]
end
last_ind_v = size_v - start_ind # TODO check if it is starting at 1st row, not second
last_ind_h = size_h - start_ind
val_range_v = floor.(Int, range(start_ind, step=sub_img_size-overlap, stop=last_ind_v))
val_range_h = floor.(Int, range(start_ind, step=sub_img_size-overlap, stop=last_ind_h))
if isempty(val_range_v) && size_v <= sub_img_size
val_range_v = [start_ind]
end
if isempty(val_range_h) && size_h <= sub_img_size
val_range_h = [start_ind]
end
num_indexes_v = size(val_range_v,1)
num_indexes_h = size(val_range_h,1)
for row = 1:num_indexes_v, column = 1:num_indexes_h
push!(centers, CartesianIndex(val_range_v[row], val_range_h[column]))
end
return centers
end
"""
vectorize_img(video)
Rearrenges the video so that set of n frames (2D matrix varying in
time) the set of vectors is returned, in which each row is a pixel, and each
column is the value of the pixel in n-th frame.
"""
function vectorize_img(img)
rows, columns = size(img)
num_of_elements = rows*columns
vectorized_img = zeros(num_of_elements)
index = 1;
for row=1:rows
for column=1:columns
vectorized_img[index] = img[row, column];
index = index+1;
end
end
return vectorized_img
end
"""
get_video_mask(points_per_dim, video_dimensions; distribution="uniform", sorted=true, x=1, y=1)
Returns matrix of size @points_per_dim x 2 in which indicies of video frame are
stored. The indicies are chosen based one the @distribution argument. One option
is uniform distribution, the second is random distribution.
Uniform distribution: distance between the points in given dimension is the
even, but vertical distance may be different from horizontal distance between points. This depends on the size of a frame in a image.
Random distribution: the distance between the points is not constant, because
the points are chosen randomly in the ranges 1:horizontal size of frame,
1:vertical size of frame. The returned values may be sorted in ascending order,
if @sorted=true.
"""
function get_video_mask(points_per_dim, video_dimensions;
distribution="uniform", sorted=true, patch_params)
video_height, video_width, = video_dimensions
x=patch_params["x"]
y=patch_params["y"]
spread=patch_params["spread"]
if x == 1
x = floor(Int,video_width/2)
@warn "Given x is to close to the border. Seeting the value to " x
elseif x < ceil(Int,points_per_dim/2)
x = ceil(Int,points_per_dim/2)
@warn "Given x is to close to the border. Seeting the value to " x
elseif x > video_width-ceil(Int,points_per_dim/2)
x = video_width - ceil(Int,points_per_dim/2)
@warn "Given x is to close to the border. Seeting the value to " x
end
if y == 1
y = floor(Int,video_height/2)
@warn "Given y is to close to the border. Seeting the value to " y
elseif y < ceil(Int,points_per_dim/2)
y = ceil(Int,points_per_dim/2)
@warn "Given y is to close to the border. Seeting the value to " y
elseif y > video_height-ceil(Int,points_per_dim/2)
y = video_height - ceil(Int,points_per_dim/2)
@warn "Given y is to close to the border. Seeting the value to " y
end
if spread*points_per_dim+x > video_width || spread*points_per_dim+y > video_height
@warn "Given patch parameters might result in indicies exceeding frame size."
end
if distribution == "uniform"
columns = points_per_dim
rows = points_per_dim
# +1 is used so that the number of points returned is as requested
row_step = floor(Int,video_height/rows)
column_step = floor(Int,video_width/columns)
(video_height/row_step != points_per_dim) ? row_step+=1 : row_step
(video_width/column_step !=
points_per_dim) ? column_step+=1 : video_width
vertical_indicies = collect(1:row_step:video_height)
horizontal_indicies = collect(1:column_step:video_width)
vertical_indicies = reshape(vertical_indicies, (1,points_per_dim))
horizontal_indicies = reshape(horizontal_indicies, (1,points_per_dim))
indicies_set = [vertical_indicies; horizontal_indicies]
elseif distribution == "random"
vertical_indicies = rand(1:video_height,1, points_per_dim)
horizontal_indicies = rand(1:video_width,1, points_per_dim)
if sorted
vertical_indicies = sort(vertical_indicies[1,:])
horizontal_indicies = sort(horizontal_indicies[1,:])
vertical_indicies = reshape(vertical_indicies, (1,points_per_dim))
horizontal_indicies =
reshape(horizontal_indicies, (1,points_per_dim))
end
indicies_set = [vertical_indicies; horizontal_indicies]
elseif distribution == "patch"
indicies_set = [collect(1:spread:(spread*points_per_dim)).+x collect(1:spread:(spread*points_per_dim)).+y]'
end
return indicies_set
end
"""
get_gabor_mask_set(;filt_size=25, σ=[2], theta_rad=[0], λ=[15], γ=[0.2],
psi_rad=[0], re_part=true, im_part=false)
Returns set of gabor filters generated with given parameters. Parameters are described
below. Function uses Kernel.gabor() from ImageFiltering.
# Arguments
- `filt_size=30` : controls the patch in which filter is created, not wavelet itself
- `σ=2` : controls the width of the waves and thus number of cycles per unit
- `theta_rad=0` : is the rotation in radians,
- `λ=15` : controls the number of waves within the window- higher values- less waves
- `γ=0.2` : is the aspect ratio; small values give long filters
- `psi_rad=0` : phase in radians
- `re_part::Bool`: determines if real part of the Gabor filter is returned; real
part is normalized to be in range [-0.5,0.5]
- `im_part::Bool`: determines if imaginary part of the Gabor filter is returned
imaginary part is normalized to be in range [-0.5,0.5]
if both `re_part` and `im_part` are true, then absolute value of complex number
of form `re_part + im_part im` is returned (it is also normalized to range
[-0.5,0.5]).
"""
function get_gabor_mask_set(;filt_size=25, σ=[2], theta_rad=[0], λ=[15], γ=[0.2],
psi_rad=[0], re_part=true, im_part=false,
do_norm=true, do_negative=true)
kernels = Any[]
for sigma = σ
for angle1 = theta_rad
θ = angle1; #pi*(angle1/180)
for lambda in λ
for gamma in γ
for angle2 in psi_rad
ψ = angle2; #pi*(angle2/180)
kernel = Kernel.gabor(filt_size, filt_size,
sigma,
θ,
lambda,
gamma,
ψ)
if re_part && !im_part
# @debug "Doing real part"
if do_norm
kernel[1] .+= abs(findmin(kernel[1])[1])
kernel[1] ./= findmax(kernel[1])[1]
# @debug "Doing norm"
if do_negative
kernel[1] .-= 0.5
end
end
push!(kernels,Gray.((kernel[1])))
elseif im_part && !re_part
if do_norm
kernel[2] .+= abs(findmin(kernel[2])[1])
kernel[2] ./= findmax(kernel[2])[1]
if do_negative
kernel[2] .-= 0.5;
end
end
push!(kernels,Gray.((kernel[2])))
else
@debug "Using abs(re(A)+im(A))"
result = abs.(kernel[1] + kernel[2]im);
if do_norm
result .+= abs(findmin(result)[1])
result ./= findmax(result)[1]
end
push!(kernels,Gray.())
end
end # angle2
end # gamma
end # lambda
end # angle1
end # sigmas
return kernels
end
"""
rearrange_filters_arr(im_filter; showing_number=-1)
Creates image with elements stored in `im_filters`. `showing_number` determines
how many of the element from `im_fiter` are displayed.
`im_filters` is an array with elements of type Matrix{Gray}.
"""
function rearrange_filters_arr(im_filter; showing_number=-1, columns=-1)
mask_size = size(im_filter[1],1)
im_filter_num = length(im_filter)
if showing_number == -1 || showing_number > im_filter_num
max_indeks = im_filter_num
else
max_indeks = showing_number
end
if columns == -1
columns = Int(ceil(sqrt(im_filter_num)))
end
rows= Int(ceil(im_filter_num/columns))
all_filters = zeros(Gray, rows*mask_size, columns*mask_size)
mask_index = 1
for row in 1:rows
start_row = (row-1)*mask_size+1
row_range = start_row:(start_row+mask_size-1)
for col = 1:columns
start_col = (col-1)*mask_size+1
col_range = start_col:(start_col+mask_size-1)
if mask_index > max_indeks
break
else
all_filters[row_range, col_range] = im_filter[mask_index]
mask_index += 1
end
end
if mask_index > max_indeks
break
end
end
return all_filters
end
# TODO remove img size from arguments
function get_local_correlations(method::String, img, img_size, sub_img_size;
masks = 0,
points_per_dim=1,
shift=0,
with_grad = true,
overlap = 0,
use_square=true)
if method == "correlation"
@debug "local correlation"
centers = get_local_img_centers(points_per_dim, img_size, shift,
sub_img_size)
extracted_pixels_matrix = get_local_img_correlations(img, centers,
sub_img_size, shift)
elseif method == "gradient_gabor"
@info "local gradient gabor comparison"
centers = get_img_local_centers(img_size, sub_img_size)
local_correlations = get_local_img_correlations(img, centers, masks;
with_gradient = with_grad)
elseif method == "gabor"
@debug "local gabor comparison"
centers = get_img_local_centers(img_size, sub_img_size; overlap = overlap, use_square=use_square)
local_correlations = get_local_img_correlations(img, centers, masks )
elseif method == "gradient"
@debug "local gradient analysis"
centers = get_local_img_centers(points_per_dim, img_size, shift,
sub_img_size)
local_correlations = get_local_img_correlations(img, centers, sub_img_size;
with_gradient=with_grad)
else
indicies_set = get_video_mask(points_per_dim, img_size,
distribution="uniform", patch_params=patch_params)
local_correlations = extract_pixels_from_img(img, indicies_set,
img_size)
end
return local_correlations
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 3382 |
# ============================================================================
# exported from MatrixProcessing on 10.09.2020
"""
get_pairwise_correlation_matrix(vectorized_video, tau_max=25)
Computes pairwise correlation of the input signals accordingly to the formula
presented in paper "Clique topology reveals intrinsic geometric structure
in neural correlations" by Chad Giusti et al.
The Computations are done only for upper half of the matrix, the lower half is
a copy of upper half. Computation-wise the difference is at level of 1e-16, but
this causes that inverse is not the same as non-inverse matrix.
"""
function get_pairwise_correlation_matrix(vectorized_video, tau_max=25)
number_of_signals = size(vectorized_video,1)
T = size(vectorized_video,2)
C_ij = zeros(number_of_signals,number_of_signals);
# log_C_ij = zeros(number_of_signals,number_of_signals);
# this is given in frames
lags = -tau_max:1:tau_max
for row=1:number_of_signals
for column=row:number_of_signals
signal_ij = vectorized_video[row,:];
signal_ji = vectorized_video[column,:];
# cross_corelation
ccg_ij = crosscov(signal_ij, signal_ji, lags);
ccg_ij = ccg_ij ./ T;
A = sum(ccg_ij[tau_max+1:end]);
B = sum(ccg_ij[1:tau_max+1]);
r_i_r_j = 1;
C_ij[row, column] = max(A, B)/(tau_max*r_i_r_j);
C_ij[column, row] = C_ij[row, column]
# log_C_i_j[row, column] = log10(abs(C_ij[row, column]));
end
end
return C_ij
end
"""
get_subimg_correlations(video_array, centers, sub_img_size, shift)
Computes the correlation between the subimages and subimages shifted by values
from range -@shift:@shift and returns array with frames of size
length(@centers) x length(@centers) with the number of frames equal to the
number of rames in @video_array.
Each of the subimage is center around values stored in @centers
"""
# TODO Check if this is the same as some of the functions from the ImageProcessing
function get_subimg_correlations(video_array, centers, sub_img_size, shift)
half_size = ceil(Int,(sub_img_size-1)/2)
half_range = half_size + shift
h, w, len = get_video_dimension(video_array)
extracted_pixels = zeros(sub_img_size, sub_img_size, len)
for frame = 1:len
img = video_array[frame]
for index_x = 1:size(centers,2)
c_x = centers[2, index_x]
for index_y = 1:size(centers,2)
c_y = centers[1, index_y]
subimage = img[(c_x-half_range):(c_x+half_range),
(c_y-half_range):(c_y+half_range)]
center = img[(c_x-half_size):(c_x+half_size), (c_y-half_size):(c_y+half_size)]
for left_boundary = 1:(2*shift+1)
for lower_boundary = 1:(2*shift+1)
corelation = center .* subimage[left_boundary:left_boundary+sub_img_size-1, lower_boundary:lower_boundary+sub_img_size-1]
corelation = sum(corelation)
extracted_pixels[index_x, index_y, frame] += corelation
end
end
extracted_pixels[index_x, index_y, frame] /= 256*(sub_img_size^2)*(shift*2)^2
end
end
end
return extracted_pixels
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 959 | """
Save figures.
"""
function save_figure(plot_ref, results_path, plot_title; extension=".png" )
full_path = results_path*plot_title*extension
savefig(plot_ref, full_path)
@info "File saved under: " full_path
end
"""
Save betti curves.
"""
function save_betti(plot_ref, results_path, plot_title)
full_title = "betti_curves_"*plot_title;
save_figure(plot_ref, results_path, full_title)
end
"""
Save figures with set of parameters given as 'kwargs'.
"""
function save_figure_with_params(plot_reference, results_path; extension=".png", prefix="", kwargs... )
plot_title = ""
kwargs_kyes = keys(kwargs)
kwargs_vals = values(kwargs)
total_params = size(collect(kwargs_vals),1)
for param = 1:total_params
plot_title *= "$(kwargs_kyes[param])_$(kwargs_vals[param])_"
end
full_path = results_path*prefix*plot_title*extension
# savefig(plot_ref, full_path)
@info "File saved as: " full_path
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 6669 | using Eirene
using Plots
include("clique_top_Julia/CliqueTop.jl")
include("VideoProcessing.jl")
include("MatrixToolbox.jl")
include("Settings.jl")
function testing_pariwise_corr()
do_clique_top = test_params["do_clique_top"]
do_eirene = test_params["do_eirene"]
save_figures = test_params["save_figures"]
plot_betti_figrues = test_params["plot_betti_figrues"]
plot_vectorized_video = test_params["plot_vectorized_video"]
size_limiter = test_params["size_limiter"]
ind_distrib = test_params["ind_distrib"]
videos_set = test_params["videos_set"]
tau_max_set = test_params["tau_max_set"]
points_per_dim_set = test_params["points_per_dim_set"]
shifts_set = test_params["shifts_set"]
patch_params = test_params["patch_params"]
video_path = test_params["video_path"]
results_path = test_params["results_path"]
videos = test_params["videos_names"]
do_local_corr = false
do_local_grad = false
if ind_distrib == "local_corr"
shift_set = test_params["shift_set"]
sub_img_size_set = [9]
do_local_corr = true
do_local_grad = false
@debug "Doing local correlation" do_local_corr
elseif ind_distrib == "local_grad"
shift_set = [1]
sub_img_size_set = test_params["sub_img_size_set"]
do_local_corr = false
do_local_grad = true
@debug "Doing local gradient" do_local_grad
else
shift_set = [1]
sub_img_size_set = [9]
do_local_corr = false
do_local_grad = false
end
@info "Using following distribution: " test_params["ind_distrib"]
@debug "All videos are: " videos_names
@debug "Video set is : " videos_set
for video in videos_set
choice = videos_names[video]
@info "Selected video: " choice
@debug "Path and choice is:" video_path*choice
video_array = get_video_array_from_file(video_path*choice)
@info "Array extracted."
video_dimensions = get_video_dimension(video_array)
for points_per_dim in points_per_dim_set
for shift in shift_set, sub_img_size in sub_img_size_set
if do_local_corr
centers = get_local_centers(points_per_dim, video_dimensions, shift, sub_img_size)
extracted_pixels_matrix = get_subimg_correlations(video_array, centers, sub_img_size, shift)
elseif do_local_grad
centers = get_local_centers(points_per_dim, video_dimensions, shift, sub_img_size)
extracted_pixels_matrix = get_local_gradients(video_array, centers, sub_img_size)
else
indicies_set = get_video_mask(points_per_dim, video_dimensions, distribution=ind_distrib, patch_params)
extracted_pixels_matrix = extract_pixels_from_video(video_array, indicies_set, video_dimensions)
end
@info "Pixels extracted."
vectorized_video = vectorize_video(extracted_pixels_matrix)
@info "Video is vectorized, proceeding to Pairwise correlation."
for tau in tau_max_set
## Compute pairwise correlation
C_ij = get_pairwise_correlation_matrix(vectorized_video, tau)
# set the diagonal to zero
for diag_elem in 1:size(C_ij,1)
C_ij[diag_elem,diag_elem] = 0
end
@info "Pairwise correlation finished, proceeding to persistance homology."
# Compute persistance homology with CliqueTopJulia
size_limiter = test_params["size_limiter"]
@debug "using size limiter = " size_limiter
if size_limiter > size(C_ij,1)
@warn "Used size limiter is larger than matrix dimension: " size_limiter size(C_ij,1)
@warn "Using maximal size instead"
size_limiter = size(C_ij,1)
end
@debug "do_clique_top: " do_clique_top
@debug "test_params['do_clique_top']: " test_params["do_clique_top"]
if do_clique_top
@debug pwd()
@time c_ij_betti_num, edge_density, persistence_intervals, unbounded_intervals = compute_clique_topology(C_ij[1:size_limiter, 1:size_limiter], edgeDensity = 0.6)
end
@debug "do_eirene: " do_eirene
if do_eirene
C = eirene(C_ij[1:size_limiter, 1:size_limiter],maxdim=3,model="vr")
end
# ---------------------------------------------------------
# Plot results
@debug "Proceeding to plotting."
if plot_vectorized_video
vector_plot_ref = heatmap(vectorized_video, color=:grays)
if save_figures
name = split(choice, ".")[1]
name = "vec_" * name * "_sz$(size_limiter)_p$(points_per_dim)_tau$(tau).png"
savefig(vector_plot_ref, name)
end #save vec
end #plot vec
if plot_betti_figrues && do_clique_top
betti_numbers = c_ij_betti_num
title = "Betti curves for pairwise corr. matrix"
p1 = plot_betti_numbers(c_ij_betti_num, edge_density, title);
heat_map1 = heatmap(C_ij, color=:lightrainbow, title="Pariwise Correlation matrix, number of points: $(points_per_dim)");
betti_plot_clq_ref = plot(p1, heat_map1, layout = (2,1))
if save_figures
saving_figures(betti_plot_clq_ref, results_cliq_path, choice, points_per_dim, tau, size_limiter)
end#save fig
end #plot cliq
if plot_betti_figrues && do_eirene
p1, heat_map1 = plot_eirene_betti_curves(C, C_ij)
betti_plot_ei_ref = plot(p1, heat_map1, layout = (2,1))
if save_figures
saving_figures(betti_plot_ei_ref, results_cliq_path, choice, points_per_dim, tau, size_limiter)
end#save fig
end #plot eirene
end #for tau
end #for shift
end #for points_per_dim
end #for video set
@info "Finished testing"
end #func
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 9266 | #=
Creted: 2020-05-04
Author: Emil Dmitruk
Structures for storing matrices used at different stages of preprocessing for
topological analysis with Eirene library.
=#
# TODO draw a diagram of all structures
# ===
struct MethodsParams
plot_filters::Bool
plot_heatmaps::Bool
plot_betti_figrues::Bool
lower_dist_mat_resolution::Bool
lower_ord_mat_resolution::Bool
legend_on::Bool
do_dsiplay::Bool
save_gabor_params::Bool
save_subelements::Bool
save_figure::Bool
function MethodsParams(;plot_filters = false,
plot_heatmaps = true,
plot_betti_figrues = true,
lower_dist_mat_resolution = false,
lower_ord_mat_resolution = false,
legend_on = true,
do_dsiplay = false,
save_gabor_params = false,
save_subelements = false,
save_figure = false)
new(plot_filters, plot_heatmaps, plot_betti_figrues,
lower_dist_mat_resolution, lower_ord_mat_resolution,
legend_on, do_dsiplay, save_gabor_params, save_subelements,
save_figure)
end
end
struct ImageTopologyParams
total_bins::Int
max_size_limiter::Int
min_B_dim::Int
max_B_dim::Int
file_name::String
img_path::String
gruping::Bool
sub_img_size::Int
sub_sample_size::Int
pooling_method::String
gabor_set::Int
overlap::Number
gaussian_blurr::Number
function ImageTopologyParams(;total_bins = 5, max_size_limiter = 200,
min_B_dim = 1, max_B_dim = 4,
file_name = "", img_path="img/",
gruping = true, sub_img_size = 33, sub_sample_size=2,
pooling_method = "avg_pooling", gabor_set = 4, overlap = 0.0,
gaussian_blurr=0.25)
new(total_bins, max_size_limiter, min_B_dim, max_B_dim,
file_name, img_path, gruping, sub_img_size, sub_sample_size,
pooling_method, gabor_set, overlap, gaussian_blurr)
end
end
function get_params_description(par::ImageTopologyParams)
if par.pooling_method == "gauss_pooling"
met = "_$(par.pooling_method)$(ceil(Int, par.gaussian_blurr*100))"
else
met = "_$(par.pooling_method)"
end
return "file_$(split(par.file_name,'.')[1])"*
"_subimgsize$(par.sub_img_size)"*
"_maxB_$(par.max_B_dim)"*
"_minB_$(par.min_B_dim)"*
"_gaborset_$(par.gabor_set)"*
"_poolingsize_$(par.sub_sample_size)"*
"$(met)_"*
"_overlap_$(Int(par.overlap*10))_"
end
function get_ord_mat_from_img(par::ImageTopologyParams, met_par::MethodsParams; get_distances=false)
@info "Current img_size" par.sub_img_size
@info "Using file: " par.file_name
@debug "Used params: " par.total_bins, par.gabor_set
size_limiter = par.max_size_limiter
# =============================== Get image masks ======================
masks = get_filter_set(par.gabor_set, par.sub_img_size; plot_filters = false,
save_gabor_params = met_par.save_gabor_params)
# =============================== Get image ================================
file_n = split(par.file_name, ".")[1]
loaded_img = load(par.img_path*par.file_name)
img1_gray = Gray.(loaded_img)
img_size = size(img1_gray)
# ================================ Process Image =======================
# get the correlation matrix accordingly to choosen method
local_correlations = get_local_correlations("gabor", img1_gray,img_size,
par.sub_img_size; masks = masks,
overlap=par.overlap)
# ======================== Compute pairwise correlation ================
dist_mat = pairwise(Euclidean(), local_correlations, dims=2)
# =============================== Ordered matrix =======================
size_limiter = size(dist_mat,1)
if size_limiter > par.max_size_limiter
@warn "Restricting matrix size, because matrix is too big"
size_limiter = par.max_size_limiter
end
# ===
# Matrix gupping
met_par.lower_dist_mat_resolution && group_distances!(dist_mat, par.total_bins)
# ===
# Matrix ordering
ordered_matrix = get_ordered_matrix(dist_mat[1:size_limiter,1:size_limiter];
assign_same_values=par.gruping)
if met_par.lower_ord_mat_resolution
ordered_matrix = lower_ordmat_resolution(ordered_matrix, par.total_bins)
end
if get_distances
return dist_mat
else
return ordered_matrix
end
end
# ===
struct TopologyMatrixSet
file_name::String
# 2 below are not necessary, if params are includede in this structure
sub_sample_size::Int
pooling_method::String
# Matrices
ordered_matrix::Array
reordered_matrix
# reordered_map_ref
pooled_matrix
pooled_reord_matrix
renum_pooled_orig_matrix
renum_pooled_reord_matrix
reordered_renum_pooled_orig_matrix
matrix_collection
description_vector
ranks_collection
params::ImageTopologyParams
function TopologyMatrixSet(input_matrix::Array, params::ImageTopologyParams
; description_vector)
# TODO add parameter which describes which methods should be used
# @warn "Using constant in structure definition- TODO: change to variable"
# file_name = images_set[6]
# sub_sample_size = 2
# pooling_method = "avg_pooling"
# ===
file_name = params.file_name
reordered_matrix, reordered_map_ref =
order_max_vals_near_diagonal2(input_matrix; do_final_plot=false, do_all_plots = false);
pooled_matrix = reorganize_matrix(input_matrix; subsamp_size=params.sub_sample_size, method=params.pooling_method, gauss_sigma=params.gaussian_blurr)
pooled_reord_matrix = reorganize_matrix(reordered_matrix; subsamp_size=params.sub_sample_size, method=params.pooling_method, gauss_sigma=params.gaussian_blurr)
# =
# gaussian_blurr = g_blurr
# used_kernel = Kernel.gaussian(gaussian_blurr)
# pooled_matrix = ceil.(Int,imfilter(input_matrix, used_kernel))
# pooled_reord_matrix = ceil.(Int,imfilter(reordered_matrix, used_kernel))
# =
renum_pooled_orig_matrix = get_ordered_matrix(pooled_matrix; assign_same_values=true)
renum_pooled_reord_matrix = get_ordered_matrix(pooled_reord_matrix; assign_same_values=true)
reordered_renum_pooled_orig_matrix, reordered_renum_pooled_orig_matrix_ref =
order_max_vals_near_diagonal2(renum_pooled_orig_matrix; do_final_plot=false, do_all_plots = false);
matrix_collection = Array[]
push!(matrix_collection, input_matrix)
push!(matrix_collection, reordered_matrix)
push!(matrix_collection, pooled_matrix)
push!(matrix_collection, pooled_reord_matrix)
push!(matrix_collection, renum_pooled_orig_matrix)
push!(matrix_collection, renum_pooled_reord_matrix)
push!(matrix_collection, reordered_renum_pooled_orig_matrix)
ranks_collection = zeros(Int,size(matrix_collection)[1])
for mat = 1: size(matrix_collection)[1]
ranks_collection[mat] = rank(matrix_collection[mat])
end
new(file_name, params.sub_sample_size, params.pooling_method, input_matrix,
reordered_matrix,
# reordered_map_ref,
pooled_matrix, pooled_reord_matrix,
renum_pooled_orig_matrix, renum_pooled_reord_matrix,
reordered_renum_pooled_orig_matrix,
matrix_collection, description_vector, ranks_collection, params)
end
end
# ===
struct TopologyMatrixBettisSet
min_B_dim::Int
max_B_dim::Int
bettis_collection
function TopologyMatrixBettisSet(top_mat_set::TopologyMatrixSet;min_B_dim=1, max_B_dim=3)
bettis_collection = Any[]
for matrix = top_mat_set.matrix_collection
# ===
# Persistent homology
eirene_geom = eirene(matrix,maxdim=top_mat_set.params.max_B_dim,model="vr")
bett_geom = get_bettis(eirene_geom, top_mat_set.params.max_B_dim, min_dim = top_mat_set.params.min_B_dim)
push!(bettis_collection,bett_geom)
end
new(min_B_dim, max_B_dim, bettis_collection)
end
end
# ===
struct MatrixHeatmap
heat_map
matrix_property::String
function MatrixHeatmap(in_array, description)
hmap_len = size(in_array)[1]
ordered_map1 = plot_square_heatmap(in_array, 5, hmap_len; plt_title=description,)
new(ordered_map1, description)
end
end
# ===
struct TopologyMatrixHeatmapsSet
heatmaps::Array{MatrixHeatmap}
heatmap_plots_set
function TopologyMatrixHeatmapsSet(topology_matrix::TopologyMatrixSet)
heatmaps = [MatrixHeatmap(topology_matrix.ordered_matrix,"original"),
MatrixHeatmap(topology_matrix.reordered_matrix,"reordered"),
MatrixHeatmap(topology_matrix.pooled_matrix,"pooled_origi"),
MatrixHeatmap(topology_matrix.pooled_reord_matrix,"pooled_reordered"),
MatrixHeatmap(topology_matrix.renum_pooled_orig_matrix,"renum_pooled_orig"),
MatrixHeatmap(topology_matrix.renum_pooled_reord_matrix,"renum_pooled_reord"),
MatrixHeatmap(topology_matrix.reordered_renum_pooled_orig_matrix,"reordered_renum_pooled_original"),
]
heatmap_plots_set = Any[]
for hmap in heatmaps
push!(heatmap_plots_set,hmap.heat_map)
end
new(heatmaps,heatmap_plots_set)
end
end
# ===
struct BettiPlot
betti_plot
function BettiPlot(in_array; min_B_dim=1)
betti_plot = plot_bettis2(in_array, "", legend_on=false, min_dim=min_B_dim);
xlabel!("Steps");
new(betti_plot)
end
end
# ===
struct TopologyMatrixBettisPlots
betti_plots_set
function TopologyMatrixBettisPlots(bettis_collection::TopologyMatrixBettisSet)
total_bettis = size(bettis_collection.bettis_collection)[1]
betti_plots_set = Any[]
for bett = 1:total_bettis
betti_plot = BettiPlot(bettis_collection.bettis_collection[bett])
push!(betti_plots_set, betti_plot.betti_plot)
end
new(betti_plots_set)
end
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 18524 | # import Makie
import VideoIO
using StatsBase
using Images
using ImageFeatures
# using TestImages
# using ImageDraw
using CoordinateTransformations
# using Makie
# using Logging
export get_video_array_from_file,
get_video_dimension,
get_video_mask,
extract_pixels_from_video,
vectorize_video,
get_pairwise_correlation_matrix,
get_average_from_tiles,
rotate_img_around_center,
rotate_vid_around_center,
export_images_to_vid,
rotate_and_save_video,
get_local_correlations,
get_local_centers,
get_local_gradients,
normalize_to_01,
shift_to_non_negative,
plotimg;
"""
get_video_array_from_file(video_name)
Returns array to which video frames are copied. Frames are in grayscale.
Function opens stream, then loads the file and gets all the frames from a
video.
"""
function get_video_array_from_file(video_name)
video_streamer = VideoIO.open(video_name) # candle
video_array = Vector{Array{UInt8}}(undef,0);
video_file = VideoIO.openvideo(video_streamer, target_format=VideoIO.AV_PIX_FMT_GRAY8)
while !eof(video_file)
push!(video_array,reinterpret(UInt8, read(video_file)))
end
close(video_file)
return video_array
end
"""
get_video_dimension(video_array)
Returns the tuple which contains width, height and the number of the frames of
array in whcih video was loaded.
"""
function get_video_dimension(video_array)
v_hei = size(video_array[1],1)
v_wid = size(video_array[1],2)
v_len = size(video_array,1)
video_dim_tuple = (video_height=v_hei, video_width=v_wid, video_length=v_len)
return video_dim_tuple
end
"""
get_video_mask(points_per_dim, video_dimensions; distribution="uniform", sorted=true, x=1, y=1)
Returns matrix of size @points_per_dim x 2 in which indicies of video frame are
stored. The indicies are chosen based one the @distribution argument. One option
is uniform distribution, the second is random distribution.
Uniform distribution: distance between the points in given dimension is the
even, but vertical distance may be different from horizontal distance between points. This depends on the size of a frame in a image.
Random distribution: the distance between the points is not constant, because
the points are chosen randomly in the ranges 1:horizontal size of frame,
1:vertical size of frame. The returned values may be sorted in ascending order,
if @sorted=true.
"""
function get_video_mask(points_per_dim, video_dimensions;
distribution="uniform", sorted=true, patch_params)
video_height, video_width, = video_dimensions
y=patch_params["y"]
spread=patch_params["spread"]
if x == 1
x = Int64(floor(video_width/2))
@warn "Given x is to close to the border. Seeting the value to " x
elseif x < Int64(ceil(points_per_dim/2))
x = Int64(ceil(points_per_dim/2))
@warn "Given x is to close to the border. Seeting the value to " x
elseif x > video_width-Int64(ceil(points_per_dim/2))
x = video_width - Int64(ceil(points_per_dim/2))
@warn "Given x is to close to the border. Seeting the value to " x
end
if y == 1
y = Int64(floor(video_height/2))
@warn "Given y is to close to the border. Seeting the value to " y
elseif y < Int64(ceil(points_per_dim/2))
y = Int64(ceil(points_per_dim/2))
@warn "Given y is to close to the border. Seeting the value to " y
elseif y > video_height-Int64(ceil(points_per_dim/2))
y = video_height - Int64(ceil(points_per_dim/2))
@warn "Given y is to close to the border. Seeting the value to " y
end
if spread*points_per_dim+x > video_width || spread*points_per_dim+y > video_height
@warn "Given patch parameters might result in indicies exceeding frame size."
end
if distribution == "uniform"
columns = points_per_dim
rows = points_per_dim
# +1 is used so that the number of points returned is as requested
row_step = Int64(floor(video_height/rows))
column_step = Int64(floor(video_width/columns))
(video_height/row_step != points_per_dim) ? row_step+=1 : row_step
(video_width/column_step !=
points_per_dim) ? column_step+=1 : video_width
vertical_indicies = collect(1:row_step:video_height)
horizontal_indicies = collect(1:column_step:video_width)
vertical_indicies = reshape(vertical_indicies, (1,points_per_dim))
horizontal_indicies = reshape(horizontal_indicies, (1,points_per_dim))
indicies_set = [vertical_indicies; horizontal_indicies]
elseif distribution == "random"
vertical_indicies = rand(1:video_height,1, points_per_dim)
horizontal_indicies = rand(1:video_width,1, points_per_dim)
if sorted
vertical_indicies = sort(vertical_indicies[1,:])
horizontal_indicies = sort(horizontal_indicies[1,:])
vertical_indicies = reshape(vertical_indicies, (1,points_per_dim))
horizontal_indicies =
reshape(horizontal_indicies, (1,points_per_dim))
end
indicies_set = [vertical_indicies; horizontal_indicies]
elseif distribution == "patch"
indicies_set = [collect(1:spread:(spread*points_per_dim)).+x collect(1:spread:(spread*points_per_dim)).+y]'
end
return indicies_set
end
"""
extract_pixels_from_video(video_array, indicies_set, video_dim_tuple)
Takes every frame from @video_array and extracts pixels which indicies are in
@indicies_set, thus creating video with only chosen indicies.
"""
function extract_pixels_from_video(video_array, indicies_set, video_dim_tuple)
rows = size(indicies_set,2)
columns = size(indicies_set,2)
video_length = video_dim_tuple[3]
extracted_pixels = zeros(rows, columns, video_length)
for frame_number in 1:video_length
extracted_pixels[:,:,frame_number] =
video_array[frame_number][indicies_set[1,:],indicies_set[2,:]]
end
return extracted_pixels
end
"""
vectorize_video(video)
Rearrenges the video so that set of n frames (2D matrix varying in
time) the set of vectors is returned, in which each row is a pixel, and each
column is the value of the pixel in n-th frame.
"""
function vectorize_video(video)
video_length = size(video, 3)
rows = size(video,1)
columns = size(video,2)
number_of_vectors = rows*columns
vectorized_video = zeros(number_of_vectors, video_length)
index = 1;
for row=1:rows
for column=1:columns
vectorized_video[index,:] = video[row, column,:];
index = index+1;
end
end
return vectorized_video
end
"""
get_pairwise_correlation_matrix(vectorized_video, tau_max=25)
Computes pairwise correlation of the input signals accordingly to the formula
presented in paper "Clique topology reveals intrinsic geometric structure
in neural correlations" by Chad Giusti et al.
The Computations are done only for upper half of the matrix, the lower half is
a copy of upper half. Computation-wise the difference is at level of 1e-16, but
this causes that inverse is not the same as non-inverse matrix.
"""
function get_pairwise_correlation_matrix(vectorized_video, tau_max=25)
number_of_signals = size(vectorized_video,1)
T = size(vectorized_video,2)
C_ij = zeros(number_of_signals,number_of_signals);
# log_C_ij = zeros(number_of_signals,number_of_signals);
# this is given in frames
lags = -tau_max:1:tau_max
for row=1:number_of_signals
for column=row:number_of_signals
signal_ij = vectorized_video[row,:];
signal_ji = vectorized_video[column,:];
# cross_corelation
ccg_ij = crosscov(signal_ij, signal_ji, lags);
ccg_ij = ccg_ij ./ T;
A = sum(ccg_ij[tau_max+1:end]);
B = sum(ccg_ij[1:tau_max+1]);
r_i_r_j = 1;
C_ij[row, column] = max(A, B)/(tau_max*r_i_r_j);
C_ij[column, row] = C_ij[row, column]
# log_C_i_j[row, column] = log10(abs(C_ij[row, column]));
end
end
return C_ij
end
"""
get_average_from_tiles(extracted_pixels_matrix, N)
Fnction takes a 3D array in which video is stored and splits every frame into
non overlaping tiles of size NxN. If size of @extracted_pixels_matrix is not
square of N, then only N^2 x N^2 matrix will be used for averaging.
"""
function get_average_from_tiles(extracted_pixels_matrix, N)
# N = size(extracted_pixels,1)
num_frames = size(extracted_pixels_matrix,3)
mask_matrix = ones(N, N)
result_matrix = zeros(N, N, num_frames)
col_index = 1
row_index = 1
for frame = 1:num_frames
for col = 1:N:N^2
for row = 1:N:N^2
result_matrix[mod(col,N), mod(row,N), frame] =
dot(extracted_pixels_matrix[col:(col+N-1),
row:(row+N-1), frame], mask_matrix) ./N^2
row_index += 1
end
col_index += 1
end
end
return result_matrix
end
"""
rotate_img_around_center(img, angle = 5pi/6)
Function rotates a single image (or a frame) around the center of the image by
@angle radians.
"""
function rotate_img_around_center(img, angle = 5pi/6)
θ = angle
rot = recenter(RotMatrix(θ), [size(img)...] .÷ 2) # a rotation around the center
x_translation = 0
y_translation = 0
tform = rot ∘ Translation(y_translation, x_translation)
img2 = warp(img, rot, axes(img))
return img2
end
"""
rotate_vid_around_center(img, rotation = 5pi/6)
Function rotates a video around the center of the image by @rotation radians and
the outout into matrix.
"""
function rotate_vid_around_center(src_vid_path,src_vid_name; rotation = 5pi/6)
video_array = []
video_src_strm = VideoIO.open(src_vid_path*src_vid_name)
video_src = VideoIO.openvideo(video_src_strm,
target_format=VideoIO.AV_PIX_FMT_GRAY8)
while !eof(video_src)
img = read(video_src)
img = rotate_img_around_center(img, rotation)
push!(video_array,img)
end
close(video_src)
return video_array
end
"""
export_images_to_vid(video_array, dest_file)
Exports set of images stored in @video_array to the dest_file.
"""
function export_images_to_vid(video_array, dest_file)
@debug "Exporting set of images to file"
fname = dest_file
video_dimensions = get_video_dimension(video_array)
h = video_dimensions.video_height
w = video_dimensions.video_width
nframes = video_dimensions.video_length
overwrite=true
fps=30
options = ``
ow = overwrite ? `-y` : `-n`
open(`ffmpeg
-loglevel warning
$ow
-f rawvideo
-pix_fmt rgb24
-s:v $(h)x$(w)
-r $fps
-i pipe:0
$options
-vf "transpose=0"
-pix_fmt yuv420p
$fname`, "w") do out
for i = 1:nframes
write(out, convert.(RGB{N0f8}, clamp01.(video_array[i])))
end
end
@debug "Video was saved"
end
"""
rotate_and_save_video(src_vid_path, src_vid_name, dest_vid_name;
rotation=5pi/6)
Fuction opens the @src_vid_name file, collects all the frames and then rotates
the frame aroung the center and saves new video as @dest_vid_name at
@src_vid_path.
Function was tested for following extensions;
.mov
A solution for writing to a video file was taken from:
https://discourse.julialang.org/t/creating-a-video-from-a-stack-of-images/646/7
"""
function rotate_and_save_video(src_vid_path, src_vid_name, dest_vid_name, rotation=5pi/6)
@debug src_vid_path src_vid_name dest_vid_name
if !isfile(src_vid_path*src_vid_name)
@warn "Source file at given path does not exist. Please give another name."
return
elseif isfile(src_vid_path*dest_vid_name)
@warn "File with destination video name at src_video_path already exists. Please give another name."
return
end
video_array = rotate_vid_around_ceter(src_vid_path, src_vid_name)
@debug "Video was rotated"
export_images_to_exist_vid(video_array, src_vid_path*dest_vid_name)
@info "The file was created:\n $fname"
end
"""
get_local_correlations(video_array, centers, sub_img_size, shift)
Computes the correlation between the subimages and subimages shifted by values
from range -@shift:@shift and returns array with frames of size
length(@centers) x length(@centers) with the number of frames equal to the
number of rames in @video_array.
Each of the subimage is center around values stored in @centers
"""
function get_local_correlations(video_array, centers, sub_img_size, shift)
half_size = ceil(Int,(sub_img_size-1)/2)
half_range = half_size + shift
h, w, len = get_video_dimension(video_array)
extracted_pixels = zeros(sub_img_size, sub_img_size, len)
for frame = 1:len
img = video_array[frame]
for index_x = 1:size(centers,2)
c_x = centers[2, index_x]
for index_y = 1:size(centers,2)
c_y = centers[1, index_y]
subimage = img[(c_x-half_range):(c_x+half_range),
(c_y-half_range):(c_y+half_range)]
center = img[(c_x-half_size):(c_x+half_size), (c_y-half_size):(c_y+half_size)]
for left_boundary = 1:(2*shift+1)
for lower_boundary = 1:(2*shift+1)
corelation = center .* subimage[left_boundary:left_boundary+sub_img_size-1, lower_boundary:lower_boundary+sub_img_size-1]
corelation = sum(corelation)
extracted_pixels[index_x, index_y, frame] += corelation
end
end
extracted_pixels[index_x, index_y, frame] /= 256*(sub_img_size^2)*(shift*2)^2
end
end
end
return extracted_pixels
end
function get_local_centers(points_per_dim, video_dimensions, shift=0, sub_img_size=0 )
/# TODO Applied teproray solution here, so it works only for local gradients
# start = 0
# (points_per_dim>shift) ? start_ind = ceil(Int, points_per_dim/2)+ shift :
# start=shift
start_ind = ceil(Int, sub_img_size/2)
min_va, = findmin(video_dimensions)
last_ind = min_va - start_ind
set = broadcast(floor, Int, range(start_ind, stop=last_ind, length=points_per_dim))
centers = [set set]'
return centers
end
"""
get_local_gradients(video_array, centers, sub_img_size)
Computes the gradients in the subimage, takes the mean of sum of absolute
values of both hotizontal and vertical gradients as a representative of a
subimage.
"""
function get_local_gradients(video_array, centers, sub_img_size)
@debug "Entering get_local_gradients"
half_size = ceil(Int,(sub_img_size-1)/2)
half_range = half_size
h, w, len = get_video_dimension(video_array)
extracted_pixels = zeros(sub_img_size, sub_img_size, len)
@debug "starting frame procesing"
for frame = 1:len
img = video_array[frame]
img_grad = imgradients(img, KernelFactors.ando3, "replicate")
img_grad_abs = map(abs, img_grad[1]) + map(abs, img_grad[2])
for index_x = 1:size(centers,2)
c_x = centers[2, index_x]
for index_y = 1:size(centers,2)
c_y = centers[1, index_y]
sub_img = img_grad_abs[(c_x-half_size):(c_x+half_size),
(c_y-half_size):(c_y+half_size)]
extracted_pixels[index_x, index_y, frame] =mean(sub_img)
end
end
# @debug "Next frame" frame
end
return extracted_pixels
end
"""
normalize_to_01(matrix, norm_factor=256)
Returns a matrix which values are in range [0, 1]. If the values in the input
matrix are below 0, then they are shifted so that only positive numbers are in
the matrix. If the values in the matrix of shifted matrix excced value of the
@norm_factor parameter, then the matrix is normalized to the maximal value from
the matrix.
"""
function normalize_to_01(matrix, norm_factor=256)
normalized_matrix = copy(matrix)
min_val = findmin(normalized_matrix)[1]
max_val = findmax(normalized_matrix)[1]
if min_val < 0
normalized_matrix .-= min_val
end
if max_val > norm_factor
@warn "Values normalized to maximal value, not notmalization factor."
normalized_matrix = normalized_matrix./max_val
else
normalized_matrix = normalized_matrix./norm_factor
end
return normalized_matrix
end
"""
shift_to_non_negative(matrix)
Returns a matrix in which values are non-negative. This is done by finding the
minimal value in the input matrix and adding its absolute value to the matix
elements.
"""
function shift_to_non_negative(matrix)
min_val = findmin(matrix)[1]
if min_val < 0
return matrix .-= min_val
else
return matrix
end
end
"""
plotimg(matrix_to_plot)
Display an image as a plot. The values from the input matrix are adjusted to the
value range of [0, 1].
If @cut_off is true then the matrix values above 256 are set to 256 and then all
values are normalized to the value 256. If @cut_off is false, then values are
normalized to maximal value.
"""
function plotimg(matrix_to_plot, cut_off=false)
matrix_type = typeof(matrix_to_plot)
min_val = findmin(matrix_to_plot)[1]
int_types_arr = [Matrix{UInt8}; Matrix{UInt16}; Matrix{UInt32};
Matrix{UInt64}; Matrix{UInt128}; Matrix{Int8};
Matrix{Int16}; Matrix{Int32}; Matrix{Int64};
Matrix{Int128}]
float_types_arr = [Matrix{Float16} Matrix{Float32} Matrix{Float64}]
if min_val<0
matrix_to_plot = shift_to_non_negative(matrix_to_plot)
end
max_val = findmax(matrix_to_plot)[1]
if max_val > 256 && cut_off
matrix_to_plot[findall(x -> x>256, matrix_to_plot)] = 256
end
if in(matrix_type, int_types_arr)
matrix_to_plot = normalize_to_01(matrix_to_plot)
elseif in(matrix_type, float_types_arr)
matrix_to_plot = normalize_to_01(matrix_to_plot, max_val)
end
return colorview(Gray, matrix_to_plot)
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 12072 | using TopologyPreprocessing
using Test
using Eirene
#%%
@testset "BettiCurves.jl" begin
sample_distance_matrix1 = [0 1 25 4 5 9 13 17;
1 0 2 26 6 10 14 18;
25 2 0 3 7 11 15 19;
4 26 3 0 8 12 16 20;
5 6 7 8 0 21 27 24;
9 10 11 12 21 0 22 28;
13 14 15 16 27 22 0 23;
17 18 19 20 24 28 23 0 ]
sample_distance_matrix2 = [1 1 41 4 5 9 13 17 25 33;
1 1 2 42 6 10 14 18 26 34;
41 2 1 3 7 11 15 19 27 35;
4 42 3 1 8 12 16 20 28 36;
5 6 7 8 1 21 43 24 29 37;
9 10 11 12 21 1 22 44 30 38;
13 14 15 16 43 22 1 23 31 39;
17 18 19 20 24 44 23 1 32 40;
25 26 27 28 29 30 31 32 1 45;
33 34 35 36 37 38 39 40 45 1;]
# get_bettis
for matrix = [sample_distance_matrix1, sample_distance_matrix2]
for max_B_dim = 1:4
eirene_results = eirene(matrix, model="vr", maxdim = max_B_dim)
all_bettis = get_bettis(eirene_results, max_B_dim)
@test length(all_bettis) == max_B_dim
@test all_bettis isa Vector{Array{Float64,2}}
end
for max_B_dim = 1:4, min_B_dim = 1:3
if min_B_dim > max_B_dim
@debug "Continue at " min_B_dim, max_B_dim
continue
end
eirene_results = eirene(matrix, model="vr", maxdim = max_B_dim)
all_bettis = get_bettis(eirene_results, max_B_dim, min_dim=min_B_dim)
@test length(all_bettis) == max_B_dim - (min_B_dim-1)
@test all_bettis isa Vector{Array{Float64,2}}
end
end
# normalise_bettis
# as betticurve results
for matrix = [sample_distance_matrix1, sample_distance_matrix2]
for max_B_dim = 1:4, min_B_dim = 1:3
if min_B_dim > max_B_dim
@debug "Continue at " min_B_dim, max_B_dim
continue
end
eirene_results = eirene(matrix, model="vr", maxdim = max_B_dim)
betti_result = betticurve(eirene_results, dim=max_B_dim)
normed_all_bettis = normalise_bettis(betti_result)
@test typeof(normed_all_bettis) == typeof(betti_result)
@test length(normed_all_bettis) != max_B_dim
@test size(normed_all_bettis) == size(betti_result)
@test normed_all_bettis isa Array{Float64,2}
# Betti values are unchanged:
@test normed_all_bettis[:,2] == betti_result[:,2]
# Max val is 1
@test findmax(normed_all_bettis[:,1])[1] == 1.
end
end
# as get_bettis results
for matrix = [sample_distance_matrix1, sample_distance_matrix2]
for max_B_dim = 1:4, min_B_dim = 1:3
if min_B_dim > max_B_dim
@debug "Continue at " min_B_dim, max_B_dim
continue
end
eirene_results = eirene(matrix, model="vr", maxdim = max_B_dim)
all_bettis = get_bettis(eirene_results, max_B_dim)
normed_all_bettis = normalise_bettis(all_bettis)
@test typeof(normed_all_bettis) == typeof(all_bettis)
@test length(normed_all_bettis) == max_B_dim
@test normed_all_bettis isa Vector{Array{Float64,2}}
# Betti values are unchanged:
@test normed_all_bettis[max_B_dim][:,2] == all_bettis[max_B_dim][:,2]
# Max val is 1
@test findmax(normed_all_bettis[max_B_dim][:,1])[1] == 1.
end
end
# get_vectorized_bettis
let max_B_dim = 5,
min_B_dim = 1,
eirene_results = eirene(sample_distance_matrix1, model="vr", maxdim = max_B_dim)
let eirene_bettis = get_bettis(eirene_results, max_B_dim, min_dim=min_B_dim),
vectorized_bettis = get_vectorized_bettis(eirene_results, max_B_dim, min_dim=min_B_dim)
@test size(vectorized_bettis)[2] == max_B_dim - (min_B_dim-1)
for d in min_B_dim:max_B_dim
@test vectorized_bettis[:,d] == eirene_bettis[d][:,2]
end
end
end
end
@testset "BettiCurves.jl -> plot bettis" begin
# TODO remove tests which test Plots.plot function and not plot_bettis functionality
sample_distance_matrix1 = [0 1 25 4 5 9 13 17;
1 0 2 26 6 10 14 18;
25 2 0 3 7 11 15 19;
4 26 3 0 8 12 16 20;
5 6 7 8 0 21 27 24;
9 10 11 12 21 0 22 28;
13 14 15 16 27 22 0 23;
17 18 19 20 24 28 23 0 ]
sample_distance_matrix2 = [1 1 41 4 5 9 13 17 25 33;
1 1 2 42 6 10 14 18 26 34;
41 2 1 3 7 11 15 19 27 35;
4 42 3 1 8 12 16 20 28 36;
5 6 7 8 1 21 43 24 29 37;
9 10 11 12 21 1 22 44 30 38;
13 14 15 16 43 22 1 23 31 39;
17 18 19 20 24 44 23 1 32 40;
25 26 27 28 29 30 31 32 1 45;
33 34 35 36 37 38 39 40 45 1;]
# plot_bettis tests for get_bettis:
let max_B_dim = 5,
min_B_dim = 1,
eirene_results = eirene(sample_distance_matrix1, model="vr", maxdim = max_B_dim)
all_bettis = get_bettis(eirene_results, max_B_dim)
p = plot_bettis(all_bettis);
@test length(p.series_list) == max_B_dim-(min_B_dim-1)
@test p.attr[:plot_title] == ""
@test_throws DomainError plot_bettis(all_bettis, min_dim=max_B_dim+1)
for (dim_index, dim)= enumerate(min_B_dim:max_B_dim)
@test p.series_list[dim_index][:label] == "β$(dim)"
if !isnan(all_bettis[dim_index][:,1][1])
@test p.series_list[dim_index][:x] == all_bettis[dim_index][:,1]
@test p.series_list[dim_index][:y] == all_bettis[dim_index][:,2]
end
end
# for dim = min_B_dim:max_B_dim
# p = plot_bettis(all_bettis, min_dim = dim);
# @test length(p.series_list) == max_B_dim-(dim-1)
# end
let p1 = plot_bettis(all_bettis, betti_labels=false)
for (dim_index, dim)= enumerate(min_B_dim:max_B_dim)
@test p1.series_list[dim][:label] == "y$(dim)"
if !isnan(all_bettis[dim_index][:,1][1])
@test p1.series_list[dim][:x] == all_bettis[dim][:,1]
@test p1.series_list[dim][:y] == all_bettis[dim][:,2]
end
end
end
let lw=4,
p1 = plot_bettis(all_bettis, betti_labels=true, lw=lw)
for (dim_index, dim)= enumerate(min_B_dim:max_B_dim)
@test p1.series_list[dim_index][:label] == "β$(dim)"
@test p1.series_list[dim_index][:linewidth] == lw
end
end
let plt_title = "test_title",
p1 = plot_bettis(all_bettis, title=plt_title, lw=9, xlabel="2")
@test_skip p1.attr[:plot_title] == plt_title # why plot-title is not returning the title?
for dim = min_B_dim:max_B_dim
@test p1.series_list[dim][:label] == "β$(dim)"
end
end
let plt_title = "test_title",
lw = 9,
p1 = plot_bettis(all_bettis, title=plt_title, lw=lw, xlabel="2", default_labels=false)
@test_skip p1.attr[:plot_title] == plt_title # why plot-title is not returning the title?
for dim = min_B_dim:max_B_dim
@test p1.series_list[dim][:label] == "β$(dim)"
@test p1.series_list[dim][:linewidth] == lw
# @test for xlabel
# @test for no label
end
end
end
# plot_bettis tests for get_vectorized_bettis:
let max_B_dim = 5,
min_B_dim = 1,
eirene_results = eirene(sample_distance_matrix1, model="vr", maxdim = max_B_dim)
all_bettis = get_vectorized_bettis(eirene_results, max_B_dim)
end
end
@testset "BettiCurves.jl -> area under betti curves" begin
let sample_distance_matrix1 = [0 1 25 4 5 9 13 17;
1 0 2 26 6 10 14 18;
25 2 0 3 7 11 15 19;
4 26 3 0 8 12 16 20;
5 6 7 8 0 21 27 24;
9 10 11 12 21 0 22 28;
13 14 15 16 27 22 0 23;
17 18 19 20 24 28 23 0 ],
sample_distance_matrix2 = [1 1 41 4 5 9 13 17 25 33;
1 1 2 42 6 10 14 18 26 34;
41 2 1 3 7 11 15 19 27 35;
4 42 3 1 8 12 16 20 28 36;
5 6 7 8 1 21 43 24 29 37;
9 10 11 12 21 1 22 44 30 38;
13 14 15 16 43 22 1 23 31 39;
17 18 19 20 24 44 23 1 32 40;
25 26 27 28 29 30 31 32 1 45;
33 34 35 36 37 38 39 40 45 1;],
max_B_dim = 5,
min_B_dim = 1
#==
checks if the size is anyhow changed during proces;
checks is the values Array{Matrix} and reshaped matrix are the same
==#
for min_B_dim in [1, 2, 3, 4, 5]
eirene_results1 =
eirene(sample_distance_matrix1, model = "vr", maxdim = max_B_dim)
eirene_results2 =
eirene(sample_distance_matrix2, model = "vr", maxdim = max_B_dim)
bettis_collection = [
get_bettis(eirene_results1, max_B_dim),
get_bettis(eirene_results2, max_B_dim),
]
for bettis_col in bettis_collection
total_vecs = length(bettis_col)
vec_len, vec_width = size(bettis_col[1])
reshaped_betti = TopologyPreprocessing.vectorize_bettis(bettis_col)
@test vec_len .== size(reshaped_betti, 1)
@test total_vecs .== size(reshaped_betti, 2)
for k = 1:total_vecs
@test reshaped_betti[:, k] == bettis_col[k][:, 2]
end
end
end
#==
checks if get vectorized bettis has same values as get_bettis
==#
for min_B_dim in [1, 2, 3, 4, 5]
eirene_results1 =
eirene(sample_distance_matrix1, model = "vr", maxdim = max_B_dim)
eirene_results2 =
eirene(sample_distance_matrix2, model = "vr", maxdim = max_B_dim)
bettis_collection = [
get_bettis(eirene_results1, max_B_dim),
get_bettis(eirene_results2, max_B_dim),
]
vec_bett_collection = [
get_vectorized_bettis(eirene_results1, max_B_dim),
get_vectorized_bettis(eirene_results2, max_B_dim),
]
for index = 1:length(bettis_collection)
bettis_col = bettis_collection[index]
vec_bettis_col = vec_bett_collection[index]
total_vecs = length(bettis_col)
for k = 1:total_vecs
@test vec_bettis_col[:, k] == bettis_col[k][:, 2]
end
end
end
end
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 9341 | using TopologyPreprocessing
using Test
using Random
Random.seed!(1234)
# using MatrixOrganization
@testset "MatrixOrganization.jl -> matrix pooling" begin
in_vector = [1 2 3 4 3 2 1]
in_matrix = [1 2 3; 5 6 7; 8 9 0]
in_matrix2 = [1 2 3; 5 6 7; 8 9 0]
sqr_matrix0 = [1 2 3; 2 6 7; 3 7 0]
resulting_matrix0_2 = [1 2 3; 2 6 7; 3 7 0]
sqr_matrix1 = [ 0 1 13 4 5 9;
1 0 2 14 6 10;
13 2 0 3 7 11;
4 14 3 0 8 12;
5 6 7 8 0 15;
9 10 11 12 15 0]
resulting_matrix1_2 = [0 1 14 14 10 10;
1 0 14 14 10 10;
14 14 0 3 12 12;
14 14 3 0 12 12;
10 10 12 12 0 15;
10 10 12 12 15 0]
sqr_matrix2 = [ 0 1 113 4 5 9 13 17 81 82 83 84;
1 0 2 114 6 10 14 18 85 86 87 88;
113 2 0 3 7 11 15 19 89 90 91 92;
4 114 3 0 8 12 16 20 93 94 95 96;
5 6 7 8 0 21 115 24 29 30 31 32;
9 10 11 12 21 0 22 116 33 34 35 36;
13 14 15 16 115 22 0 23 37 38 39 40;
17 18 19 20 24 116 23 0 41 42 43 44;
81 85 89 93 29 33 37 41 0 25 117 28;
82 86 90 94 30 34 38 42 25 0 26 118;
83 87 91 95 31 35 39 43 117 26 0 27;
84 88 92 96 32 36 40 44 28 118 27 0]
result_matrix2_2 = [ 0 1 114 114 10 10 18 18 86 86 88 88;
1 0 114 114 10 10 18 18 86 86 88 88;
114 114 0 3 12 12 20 20 94 94 96 96;
114 114 3 0 12 12 20 20 94 94 96 96;
10 10 12 12 0 21 116 116 34 34 36 36;
10 10 12 12 21 0 116 116 34 34 36 36;
18 18 20 20 116 116 0 23 42 42 44 44;
18 18 20 20 116 116 23 0 42 42 44 44;
86 86 94 94 34 34 42 42 0 25 118 118;
86 86 94 94 34 34 42 42 25 0 118 118;
88 88 96 96 36 36 44 44 118 118 0 27;
88 88 96 96 36 36 44 44 118 118 27 0]
result_matrix2_3 = [ 0 1 113 114 114 114 89 89 89 92 92 92;
1 0 2 114 114 114 89 89 89 92 92 92;
113 2 0 114 114 114 89 89 89 92 92 92;
114 114 114 0 8 12 116 116 116 96 96 96;
114 114 114 8 0 21 116 116 116 96 96 96;
114 114 114 12 21 0 116 116 116 96 96 96;
89 89 89 116 116 116 0 23 37 117 117 117;
89 89 89 116 116 116 23 0 41 117 117 117;
89 89 89 116 116 116 37 41 0 117 117 117;
92 92 92 96 96 96 117 117 117 0 26 118;
92 92 92 96 96 96 117 117 117 26 0 27;
92 92 92 96 96 96 117 117 117 118 27 0]
result_matrix2_4 = [ 0 1 114 114 20 20 20 20 96 96 96 96;
1 0 114 114 20 20 20 20 96 96 96 96;
114 114 0 3 20 20 20 20 96 96 96 96;
114 114 3 0 20 20 20 20 96 96 96 96;
20 20 20 20 0 21 116 116 44 44 44 44;
20 20 20 20 21 0 116 116 44 44 44 44;
20 20 20 20 116 116 0 23 44 44 44 44;
20 20 20 20 116 116 23 0 44 44 44 44;
96 96 96 96 44 44 44 44 0 25 118 118;
96 96 96 96 44 44 44 44 25 0 118 118;
96 96 96 96 44 44 44 44 118 118 0 27;
96 96 96 96 44 44 44 44 118 118 27 0]
@test matrix_poling(in_vector) !== in_vector
@test matrix_poling(in_vector, method="max_pooling") == [4 4 4 4 4 4 4]
# @test matrix_poling!(in_vector) === in_vector
# @test matrix_poling!(in_vector) == [4 4 4 4 4 4 4]
@test matrix_poling(in_matrix) !== in_matrix
@test matrix_poling(in_matrix, method="max_pooling") == 9 .* ones(size(in_matrix))
# @test matrix_poling!(in_matrix) === in_matrix
# @test matrix_poling!(in_matrix) == 9 .* ones(size(in_matrix))
@test matrix_poling(in_matrix2[1:2,1:2], method="max_pooling") == 6 .* ones(size(in_matrix2[1:2,1:2]))
@test matrix_poling(in_matrix2[1:2,1:2], method="max_pooling") != in_matrix2[1:2,1:2]
# ====
# Subsampling matrix
# Function is supposed to work only on upper half, and here the upper half is too small, so there are no operations
@test subsample_matrix(sqr_matrix0, subsamp_size=2, method="max_pooling") == resulting_matrix0_2
@test subsample_matrix(sqr_matrix1, subsamp_size=2, method="max_pooling") == resulting_matrix1_2
@test subsample_matrix(sqr_matrix2, subsamp_size=2, method="max_pooling") == result_matrix2_2
@test subsample_matrix(sqr_matrix2, subsamp_size=3, method="max_pooling") == result_matrix2_3
@test subsample_matrix(sqr_matrix2, subsamp_size=4, method="max_pooling") == result_matrix2_4
end
@testset "MatrixOrganization.jl -> add_random_patch" begin
# TODO set seed for add_random_path
# TODO Seed has to be set for this test
in_vector = [1, 2, 3, 4, 3, 2, 1]
sqr_matrix0 = [ 1 2 3;
2 6 7;
3 7 0]
sqr_matrix1 = [1 2 3 4 5;
2 1 6 7 8;
3 6 1 9 10;
4 7 9 1 11;
5 8 10 11 1]
sqr_matrix2 = [ 0 1 13 4 5 9;
1 0 2 14 6 10;
13 2 0 3 7 11;
4 14 3 0 8 12;
5 6 7 8 0 15;
9 10 11 12 15 0]
sqr_matrix3 = [ 0 1 113 4 5 9 13 17 81 82 83 84;
1 0 2 114 6 10 14 18 85 86 87 88;
113 2 0 3 7 11 15 19 89 90 91 92;
4 114 3 0 8 12 16 20 93 94 95 96;
5 6 7 8 0 21 115 24 29 30 31 32;
9 10 11 12 21 0 22 116 33 34 35 36;
13 14 15 16 115 22 0 23 37 38 39 40;
17 18 19 20 24 116 23 0 41 42 43 44;
81 85 89 93 29 33 37 41 0 25 117 28;
82 86 90 94 30 34 38 42 25 0 26 118;
83 87 91 95 31 35 39 43 117 26 0 27;
84 88 92 96 32 36 40 44 28 118 27 0]
function get_unchanged_indices(input_matrix,ind)
indices = CartesianIndices(size(input_matrix))
indices = findall(x->x!=ind,indices)
for i = ind
indices = indices[findall(x->x!=i,indices)]
end
return indices
end
out_m, ind = add_random_patch(sqr_matrix0)
indices = get_unchanged_indices(sqr_matrix0,ind)
@test size(ind) == (1,2)
@test sqr_matrix0[indices] == out_m[indices]
big_sqr_matrix0 = sqr_matrix0 .*100
out_m, ind = add_random_patch(big_sqr_matrix0, patch_size=1,total_patches=2)
indices = get_unchanged_indices(big_sqr_matrix0,ind)
@test size(ind) == (2,2)
@test big_sqr_matrix0[indices] == out_m[indices]
@test sum(big_sqr_matrix0[ind] .!= out_m[ind]) == 4
@test sum(big_sqr_matrix0[ind] .== out_m[ind]) == 0
out_m, ind = add_random_patch(sqr_matrix1, patch_size=1,total_patches=2)
indices = get_unchanged_indices(sqr_matrix1,ind)
@test size(ind) == (2,2)
@test sqr_matrix1[indices] == out_m[indices]
# TODO those 2 tests fails when random value is the equal to one that is replaced
# @test sum(sqr_matrix1[ind] .!= out_m[ind]) == 4
# @test sum(sqr_matrix1[ind] .== out_m[ind]) == 0
# ===
input_matrix = sqr_matrix1
function test_adding_rand_patch(input_matrix, t_patches,p_size)
out_m, ind = add_random_patch(input_matrix, patch_size=p_size, total_patches=t_patches)
indices = get_unchanged_indices(input_matrix,ind)
@test size(ind) == (t_patches*p_size^2,2)
@test input_matrix[indices] == out_m[indices]
# For values from range, tests below does not make sense:
# @test sum(input_matrix[ind] .!= out_m[ind]) == length(ind)
# @test sum(input_matrix[ind] .== out_m[ind]) == 0
end
t_patches = 1
p_size = 2
test_adding_rand_patch(sqr_matrix0, t_patches,p_size)
test_adding_rand_patch(sqr_matrix1, t_patches,p_size)
test_adding_rand_patch(sqr_matrix2, t_patches,p_size)
test_adding_rand_patch(sqr_matrix3, t_patches,p_size)
t_patches = 1
p_size = 3
test_adding_rand_patch(sqr_matrix0, t_patches,p_size)
test_adding_rand_patch(sqr_matrix1, t_patches,p_size)
test_adding_rand_patch(sqr_matrix2, t_patches,p_size)
test_adding_rand_patch(sqr_matrix3, t_patches,p_size)
t_patches = 1
p_size = 4
correct_error = 3
# TODO change this into test_throws
try
add_random_patch(sqr_matrix0, patch_size=p_size, total_patches=t_patches)
catch err
my_error = 0
if isa(err, DomainError)
println("DomainError")
my_error = 1
elseif isa(err, DimensionMismatch)
println("DimensionMismatch")
my_error = 2
else
println("Unknow error")
my_error = 3
end
@test my_error == correct_error
end
test_adding_rand_patch(sqr_matrix1, t_patches, p_size)
test_adding_rand_patch(sqr_matrix2, t_patches, p_size)
test_adding_rand_patch(sqr_matrix3, t_patches, p_size)
t_patches = 3
p_size = 5
test_adding_rand_patch(sqr_matrix2, t_patches,p_size)
test_adding_rand_patch(sqr_matrix3, t_patches,p_size)
# ===
# locations
locations1 = [CartesianIndex(1,2), CartesianIndex(2,3)]
locations2 = [CartesianIndex(1,2), CartesianIndex(99,3)]
t_patches = 1
p_size = 1
out_m, ind = add_random_patch(sqr_matrix1, patch_size=p_size, total_patches=t_patches,locations=locations1)
indices = get_unchanged_indices(sqr_matrix1,ind)
@test ind[:,1] == locations1
@test size(ind) == (size(locations1)[1]*p_size^2,2)
@test sqr_matrix1[indices] == out_m[indices]
# The number of
@test sum(sqr_matrix1[locations1] .!= out_m[locations1]) == length(locations1)
@test sum(sqr_matrix1[locations1] .== out_m[locations1]) == 0
correct_error = 0
try
out_m, ind = add_random_patch(sqr_matrix1, patch_size=p_size, total_patches=t_patches,locations=locations2)
catch err
# global correct_error
if isa(err, DomainError)
correct_error = 1
else
correct_error = 2
end
finally
# global correct_error
@test correct_error == 2
end
# TODO test for index below diagonal
# TODO too many indices
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 28975 | using TopologyPreprocessing
using Test
using LinearAlgebra
# using MatrixOrganization
@testset "MatrixProcessing.jl -> basics" begin
# Ints
positive_matrix = [1 2 3;
4 5 6]
negative_matrix = [1 2 -3;
-4 5 6]
@test shift_to_non_negative(positive_matrix) == positive_matrix
@test isempty(findall(x->x<0, shift_to_non_negative(negative_matrix)))
# Floats
positive_matrix2 = [1. 2 3; 4 5 6]
negative_matrix2 = [1 2 -3; -4 5 6]
@test shift_to_non_negative(positive_matrix2) == positive_matrix2
@test isempty(findall(x->x<0, shift_to_non_negative(negative_matrix2)))
positive_3d_matrix = rand(2,3,4)
negative_3d_matrix = rand(2,3,4).*2 .-1
@test shift_to_non_negative(positive_3d_matrix) == positive_3d_matrix
@test isempty(findall(x->x<0, shift_to_non_negative(negative_3d_matrix)))
# =====================
@test findmin(normalize_to_01(negative_matrix))[1] == 0
@test findmax(normalize_to_01(negative_matrix))[1] == 1
powers_of_2 = [0 ; [2^k for k in 0:2:8]]
powers_of_3 = [0 ; [3^k for k in 0:2:8]]
@test findmin(normalize_to_01(powers_of_2, use_factor=true))[1] == 0
@test findmax(normalize_to_01(powers_of_2, use_factor=true))[1] == 1
@test findmin(normalize_to_01(powers_of_3, use_factor=true))[1] == 0
@test findmax(normalize_to_01(powers_of_3, use_factor=true))[1] > 1
@test findmin(normalize_to_01(powers_of_3, use_factor=true, norm_factor=3^8))[1] == 0
@test findmax(normalize_to_01(powers_of_3, use_factor=true, norm_factor=3^8))[1] == 1
# =====================
square_matrix = [1 2 3;
4 5 6;
7 8 9]
@test issymmetric(diagonal_symmetrize(square_matrix))
@test LinearAlgebra.checksquare(diagonal_symmetrize(square_matrix)) == 3
@test issymmetric(diagonal_symmetrize(positive_matrix))
@test LinearAlgebra.checksquare(diagonal_symmetrize(positive_matrix)) == 2
@test diagonal_symmetrize(square_matrix)[end,1] == square_matrix[1,end]
@test diagonal_symmetrize(square_matrix)[2,1] == square_matrix[1,2]
@test diagonal_symmetrize(square_matrix, below_over_upper=true)[1,end] == square_matrix[end,1]
@test diagonal_symmetrize(square_matrix, below_over_upper=true)[1,2] == square_matrix[2,1]
end
@testset "MatrixProcessing.jl -> distances and indices" begin
# Ints
positive_matrix = [1 2 3;4 5 6]
positive_matrix2 = [1. 2 3; 4 5 6]
positive_3d_matrix = rand(2,3,4)
negative_matrix = [1 2 -3;-4 5 6]
negative_matrix2 = [1 2 -3; -4 5 6]
negative_3d_matrix = rand(2,3,4).*2 .-1
negative_6d_matrix = rand(2,3,4,5,6,7).*2 .-1
powers_of_2 = [0 ; [2^k for k in 0:2:8]]
powers_of_3 = [0 ; [3^k for k in 0:2:8]]
square_matrix = [1 2 3;
4 5 6;
7 8 9]
# ========================================================
@test length(unique(group_distances(square_matrix,1))) == 1
@test length(unique(group_distances(square_matrix,2))) == 2
@test length(unique(group_distances(square_matrix,9))) == 9
@test_throws DomainError group_distances(square_matrix,20)
@test length(unique(group_distances(positive_matrix,1))) == 1
@test length(unique(group_distances(positive_matrix,2))) == 2
@test length(unique(group_distances(positive_matrix,6))) == 6
@test_throws DomainError group_distances(positive_matrix,20)
@test length(unique(group_distances(positive_3d_matrix,2))) == 2
@test length(unique(group_distances(negative_3d_matrix,2))) == 2
@test length(unique(group_distances(negative_6d_matrix,2))) == 2
group_distances(square_matrix,2)
# ========================================================
@test length(generate_indices(3))==3^2
@test length(generate_indices(223))==223^2
n=3
@test length(generate_indices(n, symmetry_order=true, include_diagonal=false)) == ((n^2 - n) ÷ 2)
n=9
@test length(generate_indices(n, symmetry_order=true, include_diagonal=false)) == ((n^2 - n) ÷ 2)
index_matrix1 = generate_indices(n, symmetry_order=true, include_diagonal=false)
@test length(findall(x-> x == CartesianIndex(n,n),index_matrix1)) == 0
@test length(findall(x-> x == CartesianIndex(n-2,n-1),index_matrix1)) == 1
@test length(findall(x-> x == CartesianIndex(n-1,n-2),index_matrix1)) == 0
n=223
@test length(generate_indices(n, symmetry_order=true, include_diagonal=false)) == ((n^2 - n) ÷ 2)
@test length(generate_indices(n, symmetry_order=true, include_diagonal=true)) == ((n^2 - n) ÷ 2 + n)
n=3
index_matrix2 = generate_indices(n, symmetry_order=true, include_diagonal=true)
@test length(index_matrix2) == (((n-1)*n)÷2 +n)
@test findmax(index_matrix2)[1] == CartesianIndex(n,n)
@test length(findall(x-> x == CartesianIndex(1,n),index_matrix2)) == 1
@test length(findall(x-> x == CartesianIndex(1,1),index_matrix2)) == 1
@test length(findall(x-> x == CartesianIndex(n,n),index_matrix2)) == 1
n=9
@test length(generate_indices(n, symmetry_order=true, include_diagonal=true)) == (((n-1)*n)÷2 +n)
n=223
@test length(generate_indices(n, symmetry_order=true, include_diagonal=true)) == (((n-1)*n)÷2 +n)
n=9
@test length(generate_indices((n,n), symmetry_order=true, include_diagonal=true)) == (((n-1)*n)÷2 +n)
n=223
@test length(generate_indices((n,n), symmetry_order=true, include_diagonal=true)) == (((n-1)*n)÷2 +n)
end
# @testset "MatrixProcessing.jl -> arrays of arrays" begin
# # Need to be taken from Bettis
# # arr_of_arrs
#
# # reduce_arrs_to_min_len(arr_of_arrs)
#
# end
@testset "MatrixProcessing.jl -> matrix ordering helping functions" begin
let testing_matrix0 = Array{Float64,2}(undef, 2, 3),
testing_matrix1 = [1 2 3; 4 5 6; 7 8 9],
testing_matrix2 = ones((2,3,4))
testing_matrix3 = [1 2 3; 4 5 6]
testing_matrix4 = [1, 4, 7, 2, 5, 8, 3, 6, 9]
@test arr_to_vec(testing_matrix0) isa Vector
@test length(testing_matrix0) == length(arr_to_vec(testing_matrix0))
@test arr_to_vec(testing_matrix1) isa Vector
@test length(testing_matrix1) == length(arr_to_vec(testing_matrix1))
@test arr_to_vec(testing_matrix1) == [1, 4, 7, 2, 5, 8, 3, 6, 9]
@test arr_to_vec(testing_matrix2) isa Vector
@test length(testing_matrix2) == length(arr_to_vec(testing_matrix2))
@test arr_to_vec(testing_matrix3) isa Vector
@test length(testing_matrix3) == length(arr_to_vec(testing_matrix3))
@test arr_to_vec(testing_matrix3) == [1, 4, 2, 5, 3, 6]
@test arr_to_vec(testing_matrix4) isa Vector
@test length(testing_matrix4) == length(arr_to_vec(testing_matrix4))
@test arr_to_vec(testing_matrix4) == [1, 4, 7, 2, 5, 8, 3, 6, 9]
end
let testing_matrix1 = CartesianIndices((2,2)),
testing_matrix2 = CartesianIndices((9,1))
@test cartesianInd_to_vec(testing_matrix1) isa Vector
@test length(cartesianInd_to_vec(testing_matrix1)) == length(testing_matrix1)
@test cartesianInd_to_vec(testing_matrix2) isa Vector
@test length(cartesianInd_to_vec(testing_matrix2)) == length(testing_matrix2)
end
let vals_matrix1a = [1 2 3; 4 5 6],
vals_matrix1b = [6 5 4; 3 2 1],
vals_matrix1c = [4 5 6; 1 2 3]
let index_matrix1a = [CartesianIndex(1,1), CartesianIndex(1,2), CartesianIndex(1,3), CartesianIndex(2,1), CartesianIndex(2,2), CartesianIndex(2,3)]
@test length(sort_indices_by_values(vals_matrix1a, index_matrix1a)) == length(vals_matrix1a)
@test sort_indices_by_values(vals_matrix1a, index_matrix1a) == collect(1:6)
@test length(sort_indices_by_values(vals_matrix1b, index_matrix1a)) == length(vals_matrix1b)
@test sort_indices_by_values(vals_matrix1b, index_matrix1a) == collect(6:-1:1)
@test length(sort_indices_by_values(vals_matrix1c, index_matrix1a)) == length(vals_matrix1c)
@test sort_indices_by_values(vals_matrix1c, index_matrix1a) == [4, 5, 6, 1, 2, 3]
end
let index_matrix1b = CartesianIndices((2,3))
@test_throws TypeError sort_indices_by_values(vals_matrix1a, index_matrix1b)
end
end
let vals_matrix2 = [1 2 3; 4 5 6; 7 8 9],
index_matrix2a = CartesianIndices((3,3)),
index_matrix2b = [CartesianIndex(1,1) CartesianIndex(1,2) CartesianIndex(1,3);
CartesianIndex(2,1) CartesianIndex(2,2) CartesianIndex(2,3);
CartesianIndex(3,1) CartesianIndex(3,2) CartesianIndex(3,3)],
index_matrix2c = [CartesianIndex(1,1), CartesianIndex(1,2), CartesianIndex(1,3),
CartesianIndex(2,1), CartesianIndex(2,2), CartesianIndex(2,3),
CartesianIndex(3,1), CartesianIndex(3,2), CartesianIndex(3,3)]
@test_throws TypeError sort_indices_by_values(vals_matrix2, index_matrix2a)
@test_throws TypeError sort_indices_by_values(vals_matrix2, index_matrix2b)
@test sort_indices_by_values(vals_matrix2, index_matrix2c) isa Vector
@test sort_indices_by_values(vals_matrix2, index_matrix2c) == 1:9
@test length(sort_indices_by_values(vals_matrix2, index_matrix2c)) == length(vals_matrix2)
end
let vals_matrix3 = [1, 4, 7, 2, 5, 8, 3, 6, 9],
index_matrix3a = CartesianIndices((9,1)),
index_matrix3b = CartesianIndices((9,)),
index_matrix3c = [1, 4, 7, 2, 5, 8, 3, 6, 9]
@test_throws TypeError sort_indices_by_values(vals_matrix3, index_matrix3a)
@test_throws TypeError sort_indices_by_values(vals_matrix3, index_matrix3b)
@test sort_indices_by_values(vals_matrix3, index_matrix3c) isa Vector
@test sort_indices_by_values(vals_matrix3, index_matrix3c) == 1:9
@test length(sort_indices_by_values(vals_matrix3, index_matrix3c)) == length(vals_matrix3)
end
let target_coords1 = CartesianIndex(2,3),
target_value = -20
let some_matrix = [1 2 3; 4 5 6; 7 8 9]
set_values!(some_matrix, target_coords1, target_value; do_symmetry=false)
@test some_matrix[target_coords1] == target_value
end
let some_matrix = [1 2 3; 4 5 6; 7 8 9]
another_matrix = set_values!(some_matrix, target_coords1, target_value; do_symmetry=false)
@test some_matrix[target_coords1] == target_value
@test another_matrix[target_coords1] == target_value
@test another_matrix === some_matrix
end
let some_matrix = [1 2 3; 4 5 6; 7 8 9]
another_matrix = set_values!(some_matrix, target_coords1, target_value; do_symmetry=true)
@test some_matrix[target_coords1] == target_value
@test some_matrix[target_coords1[1],target_coords1[2]] == target_value
@test some_matrix[target_coords1[2],target_coords1[1]] == target_value
@test another_matrix === some_matrix
end
let some_matrix = [1 2 3; 4 5 6; 7 8 9],
some_matrix2 = [1 2 3; 4 5 6; 7 8 9],
target_coords2 = CartesianIndex(8,9)
@test_throws BoundsError set_values!(some_matrix, target_coords2, target_value; do_symmetry=false)
@test some_matrix == some_matrix2
end
let some_matrix = [1 2 3; 4 5 6; 7 8 9],
target_coords3 = CartesianIndices((2,2))
@test_throws MethodError set_values!(some_matrix, target_coords3, target_value; do_symmetry=false)
end
end
end
@testset "MatrixProcessing.jl -> matrix ordering" begin
"""
check_for_min_val_position(input_matrix::Matrix; force_symmetry=false, assign_same_values=false)
Takes an 'input_matrix' and checks if its ordered form has the minimum value
in the same position as the minimum value of 'input_matrix'.
"""
function check_for_min_val_position(input_matrix::Matrix; force_symmetry=false, assign_same_values=false)
# check if min val in uniquely value matrix is in the same position
ordered_matrix = get_ordered_matrix(input_matrix, force_symmetry=force_symmetry, assign_same_values=assign_same_values)
if issymmetric(input_matrix) || force_symmetry
off_diag_ind = generate_indices(size(input_matrix),include_diagonal=false)
else
off_diag_ind = generate_indices(size(input_matrix),include_diagonal=true)
end
min_val = findmin(input_matrix[off_diag_ind])[1]
# min_orig = findmin(input_matrix[off_diag_ind])[2]
all_min_input = findall(x->x==min_val,input_matrix[off_diag_ind])
all_min_ordered = findall(x->x==1,ordered_matrix[off_diag_ind])
return off_diag_ind[all_min_input] == off_diag_ind[all_min_ordered]
end
# ==
let power_matrix = zeros(Int, (2,3,4))
for k = 1:4
power_matrix[:,:,k] = reshape([(k+1)^n for n =1:6], (2,3))
end
ordered_power_matrix = copy(power_matrix)
ordered_power_matrix[:, :, 1] =[1 6 12;
3 8 13]
ordered_power_matrix[:, :, 2] =[2 11 17;
7 15 20]
ordered_power_matrix[:, :, 3] = [4 14 21;
9 18 23]
ordered_power_matrix[:, :, 4] =[5 16 22;
10 19 24]
@test !isempty(get_ordered_matrix(power_matrix) == ordered_power_matrix)
@test get_ordered_matrix(power_matrix) == ordered_power_matrix
# @test_throws MethodError get_ordered_matrix(power_matrix)
end
# ==
let square_matrix1 = [1 2 3; 4 5 6; 7 8 9],
square_matrix2 = [1 4 7; 2 5 8; 3 6 9]
@test get_ordered_matrix(10 .*square_matrix1) == (square_matrix1 )
@test get_ordered_matrix(10 .*square_matrix2) == (square_matrix2 )
@test sum(get_ordered_matrix(square_matrix1) .== square_matrix1 ) == 9
@test sum(get_ordered_matrix(square_matrix2) .== square_matrix2 ) == 9
@test get_ordered_matrix(10 .*square_matrix1, force_symmetry=true) == get_ordered_matrix(square_matrix1, force_symmetry=true)
@test get_ordered_matrix(10 .*square_matrix2, force_symmetry=true) == get_ordered_matrix(square_matrix2, force_symmetry=true)
# check if min val in uniquely value matrix is in the same position
let input_matrix = 10square_matrix1
@test check_for_min_val_position(input_matrix)
end
end
# ==
let square_matrix_same_vals1 = [1 2 3; 3 4 5; 6 7 8],
square_matrix_same_vals2 = [1 3 6; 2 4 7; 3 5 8]
@test get_ordered_matrix(10 .*square_matrix_same_vals1, assign_same_values=true) == (square_matrix_same_vals1 )
@test get_ordered_matrix(10 .*square_matrix_same_vals2, assign_same_values=true) == (square_matrix_same_vals2 )
# forcing symmetry test
some_ord_mat = get_ordered_matrix(10 .*square_matrix_same_vals1, force_symmetry=true, assign_same_values=false)
# remove 1, because 0 is not off diagonal
@test length(unique(some_ord_mat))-1 == (size(square_matrix_same_vals1,1)*(size(square_matrix_same_vals1,1)-1))/2
end
# ==
let square_matrix_same_vals3 = [1 2 3; 3 4 3; 5 6 7],
square_matrix_same_vals4 = [1 3 3; 2 4 6; 3 3 7]
@test get_ordered_matrix(10 .*square_matrix_same_vals3, force_symmetry=true, assign_same_values=true) ==
get_ordered_matrix(square_matrix_same_vals3, force_symmetry=true, assign_same_values=true)
@test get_ordered_matrix(10 .*square_matrix_same_vals4, force_symmetry=true, assign_same_values=true) ==
get_ordered_matrix(square_matrix_same_vals4, force_symmetry=true, assign_same_values=true)
end
# ==================
# Tests on symmetric matrices
let test_mat_b1 = [ 1 1 1 4 5 9 ;
1 1 2 3 6 10;
1 2 1 3 7 11;
4 3 3 1 8 12;
5 6 7 8 1 13;
9 10 11 12 13 1 ;]
# no same values
let test_mat_b1_ord1 = [ 0 1 2 6 7 11;
1 0 3 4 8 12;
2 3 0 5 9 13;
6 4 5 0 10 14;
7 8 9 10 0 15;
11 12 13 14 15 0],
test_mat_b1_indices = generate_indices(size(test_mat_b1))
ord_mat_b1_1 = get_ordered_matrix(10 .*test_mat_b1, force_symmetry=false, assign_same_values=false)
@test issymmetric(ord_mat_b1_1)
@test ord_mat_b1_1[test_mat_b1_indices] == test_mat_b1_ord1[test_mat_b1_indices]
ord_mat_b1_2 = get_ordered_matrix(10 .*test_mat_b1, force_symmetry=true, assign_same_values=false)
@test issymmetric(ord_mat_b1_2)
@test ord_mat_b1_2[test_mat_b1_indices] == test_mat_b1_ord1[test_mat_b1_indices]
end
# assign same values
let test_mat_b1_ord2 = [ 0 1 1 4 5 9 ;
1 0 2 3 6 10;
1 2 0 3 7 11;
4 3 3 0 8 12;
5 6 7 8 0 13;
9 10 11 12 13 0 ;]
let ord_mat_b1_3 = get_ordered_matrix(10 .*test_mat_b1, force_symmetry=false, assign_same_values=true)
@test issymmetric(ord_mat_b1_3)
# Removed, because ordered diagonal is all 1: @test ord_mat_b1_3 == (test_mat_b1 )
@test ord_mat_b1_3 == test_mat_b1_ord2
end
let ord_mat_b1_4 = get_ordered_matrix(10 .*test_mat_b1, force_symmetry=true, assign_same_values=true)
@test issymmetric(ord_mat_b1_4)
# Removed, because ordered diagonal is all 1: @test ord_mat_b1_4 == (test_mat_b1 )
@test ord_mat_b1_4 == test_mat_b1_ord2
end
end
let input_matrix = 10 .*test_mat_b1
@test check_for_min_val_position(input_matrix; force_symmetry=false, assign_same_values=true)
end
end
# ==
# Non-symmetric matrix test
let test_mat_b2 = [ 1 1 3 4 5 9 ;
1 1 2 3 6 10;
14 2 1 3 7 11;
4 15 3 1 8 12;
5 5 7 8 1 13;
9 10 11 12 13 1 ;]
let ord_mat_b2_1 = get_ordered_matrix(10 .*test_mat_b2, force_symmetry=false, assign_same_values=false)
@test !issymmetric(ord_mat_b2_1)
@test findall(x->x<=8,ord_mat_b2_1) == findall(x->x==1,test_mat_b2) # all values with one are first 8 values used for ordering
@test length(unique(ord_mat_b2_1)) == length(test_mat_b2) #check if all values are unique
end
let test_mat_b2_ord1 = [0 1 6 4 7 11;
1 0 2 5 8 12;
6 2 0 3 9 13;
4 5 3 0 10 14;
7 8 9 10 0 15;
11 12 13 14 15 0 ;]
test_mat_b2_indices = generate_indices(size(test_mat_b2))
filter!(x->x!=CartesianIndex(1,3), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(1,4), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(2,4), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(3,4), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(3,1), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(4,1), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(4,2), test_mat_b2_indices)
filter!(x->x!=CartesianIndex(4,3), test_mat_b2_indices)
ord_mat_b2_2 = get_ordered_matrix(10 .*test_mat_b2, force_symmetry=true, assign_same_values=false)
@test issymmetric(ord_mat_b2_2)
@test ord_mat_b2_2[test_mat_b2_indices] == test_mat_b2_ord1[test_mat_b2_indices]
# forcing symmetry test:
@test length(unique(ord_mat_b2_2))-1 == (size(test_mat_b2,1)*(size(test_mat_b2,1)-1))/2
end
# TODO to fix tests, add 1 to all values off diagonal for the input matrix
let test_mat_b2_ord2 = [0 0 2 3 4 8;
0 0 1 2 5 9;
13 1 0 2 6 10;
3 14 2 0 7 11;
4 4 6 7 0 12;
8 9 10 11 12 0 ;]
ord_mat_b2_3 = get_ordered_matrix(10 .*test_mat_b2, force_symmetry=false, assign_same_values=true)
@test_skip !issymmetric(ord_mat_b2_3)
@test_skip ord_mat_b2_3 == test_mat_b2_ord2
end
# TODO to fix tests, add 1 to all values off diagonal for the input matrix
let test_mat_b2_ord3 = [0 0 2 3 4 8
0 0 1 2 5 9
2 1 0 2 6 10
3 2 2 0 7 11
4 5 6 7 0 12
8 9 10 11 12 0 ;]
ord_mat_b2_4 = get_ordered_matrix(10 .*test_mat_b2, force_symmetry=true, assign_same_values=true)
@test_skip issymmetric(ord_mat_b2_4)
@test_skip ord_mat_b2_4 == test_mat_b2_ord3
end
end
# ==
let test_mat_b3 = [ 1 1 3 4 5 9 ;
1 1 2 3 6 10;
3 2 1 3 7 11;
4 3 3 1 8 12;
5 6 7 8 1 13;
9 10 11 12 13 1 ;]
let test_mat_b3_ord1 = [ 0 0 5 3 6 10;
0 0 1 4 7 11;
5 1 0 2 8 12;
3 4 2 0 9 13;
6 7 8 9 0 14;
10 11 12 13 14 0 ;],
test_mat_b3_indices = generate_indices(size(test_mat_b3))
filter!(x->x!=CartesianIndex(1,3), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(1,4), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(2,4), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(3,4), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(3,1), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(4,1), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(4,2), test_mat_b3_indices)
filter!(x->x!=CartesianIndex(4,3), test_mat_b3_indices)
ord_mat_b3_1 = get_ordered_matrix(10 .*test_mat_b3, force_symmetry=false, assign_same_values=false)
@test issymmetric(ord_mat_b3_1)
@test_skip ord_mat_b3_1[test_mat_b3_indices] == test_mat_b3_ord1[test_mat_b3_indices]
ord_mat_b3_2 = get_ordered_matrix(10 .*test_mat_b3, force_symmetry=true, assign_same_values=false)
@test issymmetric(ord_mat_b3_2)
@test_skip ord_mat_b3_2[test_mat_b3_indices] == test_mat_b3_ord1[test_mat_b3_indices]
end
# TODO remove tests that do not add anything new for testing and are just another similar case
let test_mat_b3_ord2 = [ 0 0 2 3 4 8 ;
0 0 1 2 5 9 ;
2 1 0 2 6 10;
3 2 2 0 7 11;
4 5 6 7 0 12;
8 9 10 11 12 0 ;]
ord_mat_b3_3 = get_ordered_matrix(10 .*test_mat_b3, force_symmetry=false, assign_same_values=true)
@test issymmetric(ord_mat_b3_3)
@test_skip ord_mat_b3_3 == (test_mat_b3 .-1)
@test_skip ord_mat_b3_3 == test_mat_b3_ord2
ord_mat_b3_4 = get_ordered_matrix(10 .*test_mat_b3, force_symmetry=true, assign_same_values=true)
@test issymmetric(ord_mat_b3_4)
@test_skip ord_mat_b3_4 == (test_mat_b3 .-1)
@test_skip ord_mat_b3_4 == test_mat_b3_ord2
end
let input_matrix = 10 .*test_mat_b3
@test check_for_min_val_position(input_matrix; force_symmetry=false, assign_same_values=true)
end
end
# ==
let test_mat_b4 = [ 1 1 41 4 5 9 13 17 25 33;
1 1 2 42 6 10 14 18 26 34;
41 2 1 3 7 11 15 19 27 35;
4 42 3 1 8 12 16 20 28 36;
5 6 7 8 1 21 43 24 29 37;
9 10 11 12 21 1 22 44 30 38;
13 14 15 16 43 22 1 23 31 39;
17 18 19 20 24 44 23 1 32 40;
25 26 27 28 29 30 31 32 1 45;
33 34 35 36 37 38 39 40 45 1;]
@test_skip get_ordered_matrix(10 .*test_mat_b4, force_symmetry=false, assign_same_values=false) == (test_mat_b4 .-1)
@test_skip get_ordered_matrix(10 .*test_mat_b4, force_symmetry=false, assign_same_values=true) == (test_mat_b4 .-1)
let ord_mat = get_ordered_matrix(10 .*test_mat_b4, force_symmetry=true, assign_same_values=false)
@test issymmetric(ord_mat)
@test_skip ord_mat == (test_mat_b4 .-1)
end
let ord_mat = get_ordered_matrix(10 .*test_mat_b4, force_symmetry=true, assign_same_values=true)
@test issymmetric(ord_mat)
@test_skip ord_mat == (test_mat_b4 .-1)
end
let input_matrix = 10test_mat_b4
@test check_for_min_val_position(input_matrix)
end
end
# ==
let test_mat_b5 = -[1 1 3 4 5 9 ;
1 1 2 3 6 10;
14 2 1 3 7 11;
4 15 3 1 8 12;
5 5 7 8 1 13;
9 10 11 12 13 1 ;]
let ord_mat_b5_1 = get_ordered_matrix(10 .*test_mat_b5, force_symmetry=false, assign_same_values=false)
@test !issymmetric(ord_mat_b5_1)
@test_skip findall(x->x>=28,ord_mat_b5_1) == findall(x->x==-1,test_mat_b5) # all values with one are first 8 values used for ordering
@test length(unique(ord_mat_b5_1)) == length(test_mat_b5) #check if all values are unique
end
let test_mat_b5_ord1 = [ 0 14 9 11 8 4
14 0 13 10 7 3
9 13 0 12 6 2
11 10 12 0 5 1
8 7 6 5 0 0
4 3 2 1 0 0 ;]
test_mat_b5_indices = generate_indices(size(test_mat_b5))
filter!(x->x!=CartesianIndex(1,3), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(1,4), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(2,4), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(3,4), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(3,1), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(4,1), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(4,2), test_mat_b5_indices)
filter!(x->x!=CartesianIndex(4,3), test_mat_b5_indices)
ord_mat_b5_2 = get_ordered_matrix(10 .*test_mat_b5, force_symmetry=true, assign_same_values=false)
@test issymmetric(ord_mat_b5_2)
@test_skip ord_mat_b5_2[test_mat_b5_indices] == test_mat_b5_ord1[test_mat_b5_indices]
# forcing symmetry test:
@test length(unique(ord_mat_b5_2))-1 == (size(test_mat_b5,1)*(size(test_mat_b5,1)-1))/2
end
let test_mat_b5_ord2 = [14 14 12 11 10 6;
14 14 13 12 9 5;
1 13 14 12 8 4;
11 0 12 14 7 3;
10 10 8 7 14 2;
6 5 4 3 2 14],
ord_mat_b5_3 = get_ordered_matrix(10 .*test_mat_b5, force_symmetry=false, assign_same_values=true)
@test !issymmetric(ord_mat_b5_3)
@test_skip ord_mat_b5_3 == test_mat_b5_ord2
end
let test_mat_b5_ord3 = [0 12 10 9 8 4;
12 0 11 10 7 3;
10 11 0 10 6 2;
9 10 10 0 5 1;
8 7 6 5 0 0;
4 3 2 1 0 0]
ord_mat_b5_4 = get_ordered_matrix(10 .*test_mat_b5, force_symmetry=true, assign_same_values=true)
@test issymmetric(ord_mat_b5_4)
@test_skip ord_mat_b5_4 == test_mat_b5_ord3
end
end
# ==================
end
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | code | 464 | using SafeTestsets
# TODO add description to the tests
## ===-===-===-===-===-===-===-===-
@safetestset "MatrixProcessing tests" begin
include("matrixProcessing_tests.jl")
end
## ===-===-===-===-===-===-===-===-
@safetestset "MatrixOrganization tests" begin
include("matrixOrganisation_tests.jl")
end
## ===-===-===-===-===-===-===-===-
@safetestset "BettiCurves tests" begin
include("bettiCurves_tests.jl")
end
## ===-===-===-===-===-===-===-===-
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | docs | 352 | # TopologyPreprocessing

## Installation
This package registeration is being processed now. After being registered, to install it, run the following.
```julia
julia> using Pkg
julia> Pkg.add("TopologyPreprocessing")
```
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | 523f376b635406653aedc47262f302626d592d57 | docs | 114 | # Example.jl Documentation
```@contents
```
## Functions
```@docs
get_barcodes(x)
```
## Index
```@index
```
| TopologyPreprocessing | https://github.com/edd26/TopologyPreprocessing.git |
|
[
"MIT"
] | 0.1.6 | ac6f8ac979a738a894d1a0a5777d6b8e43f0e94e | code | 9780 | module OpenIDConnect
using HTTP
using JSON
using MbedTLS
using Base64
using Random
using JWTs
const DEFAULT_SCOPES = ["openid", "profile", "email"]
const DEFAULT_STATE_TIMEOUT_SECS = 60
const DEFAULT_SKEW_SECS = 2*60
const STATE_PURGE_TRIGGER = 1024
const DEFAULT_KEY_REFRESH_SECS = 60*60
export OIDCCtx, flow_request_authorization_code, flow_get_authorization_code, flow_get_token, flow_validate_id_token, flow_refresh_token
"""
Holds an OpenID Connect context that can be used in subsequent OpenID request flows.
The context holds request states, and configuration options.
"""
struct OIDCCtx
states::Dict{String,Float64}
state_timeout_secs::Int
allowed_skew_secs::Int
openid_config::Dict{String,Any}
http_tls_opts::Dict{Symbol,Any}
validator::JWKSet
key_refresh_secs::Int
last_key_refresh::Float64
client_id::String
client_secret::String
scopes::Vector{String}
redirect_uri::String
random_device::RandomDevice
function OIDCCtx(issuer::String, redirect_uri::String, client_id::String, client_secret::String, scopes::Vector{String}=DEFAULT_SCOPES;
verify::Union{Nothing,Bool}=nothing, cacrt::Union{Nothing,String,MbedTLS.CRT}=nothing,
state_timeout_secs::Int=DEFAULT_STATE_TIMEOUT_SECS, allowed_skew_secs::Int=DEFAULT_SKEW_SECS, key_refresh_secs::Int=DEFAULT_KEY_REFRESH_SECS,
random_device::RandomDevice=RandomDevice())
endswith(issuer, "/") || (issuer = issuer * "/")
openid_config_url = issuer * ".well-known/openid-configuration"
http_tls_opts = Dict{Symbol,Any}()
http_tls_opts[:socket_type_tls] = MbedTLS.SSLContext
if verify !== nothing
http_tls_opts[:require_ssl_verification] = verify
end
if cacrt !== nothing
if isa(cacrt, String)
cacrt = isfile(cacrt) ? MbedTLS.crt_parse_file(cacrt) : MbedTLS.crt_parse(cacrt)
end
conf = MbedTLS.SSLConfig(verify === nothing || verify)
MbedTLS.ca_chain!(conf, cacrt)
http_tls_opts[:sslconfig] = conf
end
# fetch and store the openid config, along with the additional args for SSL
openid_config = JSON.parse(String(HTTP.request("GET", openid_config_url; status_exception=true, http_tls_opts...).body))
validator = JWKSet(openid_config["jwks_uri"])
new(Dict{String,Float64}(), state_timeout_secs, allowed_skew_secs, openid_config, http_tls_opts, validator, key_refresh_secs, 0.0, client_id, client_secret, scopes, redirect_uri, random_device)
end
end
authorization_endpoint(ctx::OIDCCtx) = ctx.openid_config["authorization_endpoint"]
token_endpoint(ctx::OIDCCtx) = ctx.openid_config["token_endpoint"]
function remember_state(ctx::OIDCCtx, state::String)
ctx.states[state] = time()
nothing
end
function validate_state(ctx::OIDCCtx, state::String)
statestore = ctx.states
if state in keys(statestore)
t = statestore[state]
delete!(statestore, state)
if (time() - t) <= ctx.state_timeout_secs
return true
end
end
@info("encountered an unknown or expired state")
if length(statestore) > STATE_PURGE_TRIGGER
purge_states!(ctx)
end
false
end
function purge_states(ctx::OIDCCtx)
tnow = time()
tmout = ctx.state_timeout_secs
filter!(nv->(tnow-nv[2])>tmout, ctx.states)
nothing
end
"""
API calling error detected by this library
"""
struct APIError
error::String
end
"""
Error returned from OpenID server
See section 3.1.2.6 of https://openid.net/specs/openid-connect-core-1_0.html
"""
struct AuthServerError
error::String
error_description::Union{Nothing,String}
error_uri::Union{Nothing,String}
end
"""
Authentication request. Uses the authorization code flow.
Acceptable optional args as listed in section 3.1.2.1 of specifications (https://openid.net/specs/openid-connect-core-1_0.html)
Returns a String with the redirect URL.
Caller must perform the redirection.
"""
function flow_request_authorization_code(ctx::OIDCCtx; nonce=nothing, display=nothing, prompt=nothing, max_age=nothing, ui_locales=nothing, id_token_hint=nothing, login_hint=nothing, acr_values=nothing)
@debug("oidc negotiation: initiating...")
scopes = join(ctx.scopes, ' ')
state = randstring(ctx.random_device, 10)
remember_state(ctx, state)
query = Dict("response_type"=>"code", "client_id"=>ctx.client_id, "redirect_uri"=>ctx.redirect_uri, "scope"=>scopes, "state"=>state)
(nonce === nothing) || (query["nonce"] = String(nonce))
(display === nothing) || (query["display"] = String(display))
(prompt === nothing) || (query["prompt"] = String(prompt))
(max_age === nothing) || (query["max_age"] = String(max_age))
(ui_locales === nothing) || (query["ui_locales"] = String(ui_locales))
(id_token_hint === nothing) || (query["id_token_hint"] = String(id_token_hint))
(login_hint === nothing) || (query["login_hint"] = String(login_hint))
(acr_values === nothing) || (query["acr_values"] = String(acr_values))
uri = HTTP.URIs.URI(HTTP.URIs.URI(authorization_endpoint(ctx)); query=query)
return string(uri)
end
"""
Given the params from the redirected response from the authentication request, extract the authorization code.
See sections 3.1.2.5 and 3.1.2.6 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns the authorization code on success.
Returns one of APIError or AuthServerError on failure.
"""
function flow_get_authorization_code(ctx::OIDCCtx, @nospecialize(query))
state = get(query, "state", get(query, :state, nothing))
if state === nothing
return APIError("invalid request, no state found")
end
if validate_state(ctx, String(state)) === nothing
return APIError("invalid or expired state")
end
code = get(query, "code", get(query, :code, nothing))
if code !== nothing
return String(code)
end
errcode = get(query, "error", nothing)
if errcode !== nothing
return AuthServerError(errcode, get(query, "error_description", nothing), get(query, "error_uri", nothing))
end
return APIError("invalid request, no code or error found")
end
function parse_token_response(tok_res)
@info("oidc: success response from token endpoint")
resp_str = String(tok_res.body)
if tok_res.status == 200
return JSON.parse(resp_str)
end
try
err_resp = JSON.parse(resp_str)
errcode = get(err_resp, "error", nothing)
if errcode !== nothing
return AuthServerError(errcode, get(err_resp, "error_description", nothing), get(err_resp, "error_uri", nothing))
end
catch
return APIError("unknown response from server: " * resp_str)
end
end
"""
Token Request. Given the authorization code obtained, invoke the token end point and obtain an id_token, access_token, refresh_token.
See section 3.1.3.1 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns a JSON object containing tokens on success.
Returns a AuthServerError or APIError object on failure.
"""
function flow_get_token(ctx::OIDCCtx, code)
data = Dict("grant_type"=>"authorization_code",
"code"=>String(code),
"redirect_uri"=>ctx.redirect_uri,
"client_id"=>ctx.client_id,
"client_secret"=>ctx.client_secret)
headers = Dict("Content-Type"=>"application/x-www-form-urlencoded")
tok_res = HTTP.request("POST", token_endpoint(ctx), headers, HTTP.URIs.escapeuri(data); status_exception=false, ctx.http_tls_opts...)
return parse_token_response(tok_res)
end
"""
Token Refresh. Given the refresh code obtained, invoke the token end point and obtain new tokens.
See section 12 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns a JSON object containing tokens on success.
Returns a AuthServerError or APIError object on failure.
"""
function flow_refresh_token(ctx::OIDCCtx, refresh_token)
data = Dict("grant_type"=>"refresh_token",
"refresh_token"=>String(refresh_token),
"client_id"=>ctx.client_id,
"client_secret"=>ctx.client_secret)
headers = Dict("Content-Type"=>"application/x-www-form-urlencoded")
tok_res = HTTP.request("POST", token_endpoint(ctx), headers, HTTP.URIs.escapeuri(data); status_exception=false, ctx.http_tls_opts...)
return parse_token_response(tok_res)
end
"""
Validate an OIDC token.
Validates both the structure and signature.
See section 3.1.3.7 of https://openid.net/specs/openid-connect-core-1_0.html
"""
flow_validate_id_token(ctx::OIDCCtx, id_token) = flow_validate_id_token(ctx, JWT(;jwt=String(id_token)))
function flow_validate_id_token(ctx::OIDCCtx, jwt::JWT)
isvalid = false
if issigned(jwt)
try
tokclaims = claims(jwt)
issue_time = tokclaims["iat"] - ctx.allowed_skew_secs
expiry_time = tokclaims["exp"] + ctx.allowed_skew_secs
isvalid = issue_time <= round(Int, time()) <= expiry_time
catch ex
@info("invalid token format ($ex)")
end
if isvalid
validator = ctx.validator
if (time() - ctx.last_key_refresh) >= ctx.key_refresh_secs
jstr = String(HTTP.get(ctx.validator.url; ctx.http_tls_opts...).body)
keys = JSON.parse(jstr)["keys"]
keysetdict = Dict{String,JWK}()
refresh!(keys, keysetdict)
validator.keys = keysetdict
end
isvalid = validate!(jwt, validator)
end
end
return isvalid
end
end # module
| OpenIDConnect | https://github.com/tanmaykm/OpenIDConnect.jl.git |
|
[
"MIT"
] | 0.1.6 | ac6f8ac979a738a894d1a0a5777d6b8e43f0e94e | code | 3577 | using OpenIDConnect
using Test
using Random
using HTTP
function test_state_store()
@testset "State store" begin
ctx = OIDCCtx("https://accounts.google.com", "http://127.0.0.1:8888/auth/login", "test_client_id", "test_client_secret"; state_timeout_secs=5)
state = randstring(10)
OpenIDConnect.remember_state(ctx, state)
@test length(ctx.states) == 1
@test OpenIDConnect.validate_state(ctx, state)
sleep(10)
@info("expecting an invalid state")
@test !OpenIDConnect.validate_state(ctx, state)
@test length(ctx.states) == 0
nothing
end
end
function test_oidc_flow()
@testset "OIDC flow" begin
ctx = OIDCCtx("https://accounts.google.com", "http://127.0.0.1:8888/auth/login", "test_client_id", "test_client_secret"; state_timeout_secs=5)
@test OpenIDConnect.authorization_endpoint(ctx) == "https://accounts.google.com/o/oauth2/v2/auth"
@test OpenIDConnect.token_endpoint(ctx) == "https://oauth2.googleapis.com/token"
# flow request authorization code
uri_string = flow_request_authorization_code(ctx)
uri = HTTP.URIs.URI(uri_string)
@test uri.host == "accounts.google.com"
query = HTTP.URIs.queryparams(uri)
@test get(query, "client_id", "") == "test_client_id"
@test get(query, "redirect_uri", "") == "http://127.0.0.1:8888/auth/login"
@test get(query, "scope", "") == "openid profile email"
@test get(query, "response_type", "") == "code"
@test !isempty(get(query, "state", ""))
uri_string = flow_request_authorization_code(ctx; nonce="test_nonce", display="test_display", prompt="test_prompt", max_age="12345", ui_locales="en", id_token_hint="test_id_tok_hint", login_hint="test_login_hint", acr_values="test_acr")
uri = HTTP.URIs.URI(uri_string)
@test uri.host == "accounts.google.com"
query = HTTP.URIs.queryparams(uri)
@test get(query, "client_id", "") == "test_client_id"
@test get(query, "redirect_uri", "") == "http://127.0.0.1:8888/auth/login"
@test get(query, "scope", "") == "openid profile email"
@test get(query, "response_type", "") == "code"
@test !isempty(get(query, "state", ""))
@test get(query, "nonce", "") == "test_nonce"
@test get(query, "display", "") == "test_display"
@test get(query, "prompt", "") == "test_prompt"
@test get(query, "max_age", "") == "12345"
@test get(query, "ui_locales", "") == "en"
@test get(query, "id_token_hint", "") == "test_id_tok_hint"
@test get(query, "login_hint", "") == "test_login_hint"
@test get(query, "acr_values", "") == "test_acr"
# flow get authorization code
@test isa(flow_get_authorization_code(ctx, Dict()), OpenIDConnect.APIError)
@info("expecting an invalid state")
@test isa(flow_get_authorization_code(ctx, Dict("state"=>"teststate")), OpenIDConnect.APIError)
OpenIDConnect.remember_state(ctx, "teststate")
@test isa(flow_get_authorization_code(ctx, Dict("state"=>"teststate")), OpenIDConnect.APIError)
@info("expecting an invalid state")
@test isa(flow_get_authorization_code(ctx, Dict("state"=>"teststate", "error"=>"testerror")), OpenIDConnect.AuthServerError)
@info("expecting an invalid state")
@test "testcode" == flow_get_authorization_code(ctx, Dict("state"=>"teststate", "code"=>"testcode"))
end
end
@testset "OpenIDConnect" begin
test_state_store()
test_oidc_flow()
end
| OpenIDConnect | https://github.com/tanmaykm/OpenIDConnect.jl.git |
|
[
"MIT"
] | 0.1.6 | ac6f8ac979a738a894d1a0a5777d6b8e43f0e94e | code | 3736 | using Mux
using HTTP
using JSON
using OpenIDConnect
using JWTs
headers(req) = req[:headers]
query(req) = parse_query(req[:query])
function parse_query(qstr)
res = Dict{String,String}()
for qsub in split(qstr, '&')
nv = split(qsub, '=')
res[nv[1]] = length(nv) > 1 ? nv[2] : ""
end
res
end
function pretty(j)
iob = IOBuffer()
JSON.print(iob, j, 4)
String(take!(iob))
end
function login(oidcctx::OIDCCtx)
openid_config = oidcctx.openid_config
issuer = openid_config["issuer"]
openid_config_url = issuer * ".well-known/openid-configuration"
"""
<html><head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/oidc-client/1.5.1/oidc-client.js"></script>
<script>
var settings = {
issuer: '$issuer',
authority: '$openid_config_url',
metadata: {
issuer: '$issuer',
authorization_endpoint: '$(openid_config["authorization_endpoint"])',
userinfo_endpoint: '$(openid_config["token_endpoint"])',
jwks_uri: '$(openid_config["jwks_uri"])',
},
client_id: '$(oidcctx.client_id)',
redirect_uri: 'http://127.0.0.1:8888/auth/login',
response_type: 'code',
scope: 'openid email profile offline_access'
};
var mgr = new Oidc.UserManager(settings);
var user = mgr.signinRedirect();
</script>
</head><body></body></html>
"""
end
function show_token(oidcctx::OIDCCtx, authresp, authenticated)
id_token = authresp["id_token"]
jwt = JWT(;jwt=id_token)
isvalid = flow_validate_id_token(oidcctx, string(jwt))
token_claims = claims(jwt)
jbox_auth = Dict(
"Authorization" => ("Bearer " * id_token)
)
authenticated[] = true
can_refresh = "refresh_token" in keys(authresp)
refresh_link = can_refresh ? """<hr/><a href="/auth/refresh?refresh_token=$(authresp["refresh_token"])">Refresh</a>""" : ""
"""<html><body>
OpenID Authentication:
<pre>$(pretty(authresp))</pre><hr/>
JWT Token:
<pre>$(pretty(token_claims))</pre><hr/>
Authentication Bearer Token:
<pre>$(pretty(jbox_auth))</pre><hr/>
Validation success: $isvalid
$(refresh_link)
</body></html>"""
end
function token(oidcctx::OIDCCtx, req, authenticated)
resp = query(req)
code = resp["code"]
authresp = flow_get_token(oidcctx, code)
show_token(oidcctx, authresp, authenticated)
end
function refresh(oidcctx::OIDCCtx, req, authenticated)
resp = query(req)
refresh_token = resp["refresh_token"]
authresp = flow_refresh_token(oidcctx, refresh_token)
show_token(oidcctx, authresp, authenticated)
end
function main()
if length(ARGS) != 1
println("Usage: julia oidc_standalone.jl <configuration_file>")
exit(1)
end
config = open(ARGS[1]) do f
JSON.parse(f)
end
oidcctx = OIDCCtx(String(config["issuer"]), "http://127.0.0.1:8888/auth/login", String(config["client_id"]), String(config["client_secret"]), ["openid", "email", "profile", "offline_access"])
authenticated = Ref(false)
@app test = (
Mux.defaults,
page("/", req->login(oidcctx)),
page("/auth/login", req->token(oidcctx, req, authenticated)),
page("/auth/refresh", req->refresh(oidcctx, req, authenticated)),
Mux.notfound())
@info("Standalone OIDC test server starting on port 8888")
serve(test, 8888)
while config["do_refresh"] || !(authenticated[])
sleep(10)
end
sleep(10)
end
main()
| OpenIDConnect | https://github.com/tanmaykm/OpenIDConnect.jl.git |
|
[
"MIT"
] | 0.1.6 | ac6f8ac979a738a894d1a0a5777d6b8e43f0e94e | docs | 5233 | # OpenIDConnect
[](https://github.com/tanmaykm/OpenIDConnect.jl/actions?query=workflow%3ACI+branch%3Amaster)
[](http://codecov.io/github/tanmaykm/OpenIDConnect.jl?branch=master)
[OpenID Connect](https://openid.net/specs/openid-connect-core-1_0.html) is a simple identity layer on top of the OAuth 2.0 protocol. It enables Clients to verify the identity of the End-User based on the authentication performed by an Authorization Server, as well as to obtain basic profile information about the End-User in an interoperable and REST-like manner.
This is an implementation of OpenID Connect in Julia, with methods implementing the authorization code flow.
# OpenID Connect Context (OIDCCtx)
The OpenID Connect context holds all states for a single OpenID Connect client configuration.
```julia
function OIDCCtx(
issuer::String,
redirect_uri::String,
client_id::String,
client_secret::String,
scopes::Vector{String}=DEFAULT_SCOPES;
verify::Union{Nothing,Bool}=nothing,
cacrt::Union{Nothing,String,MbedTLS.CRT}=nothing,
state_timeout_secs::Int=DEFAULT_STATE_TIMEOUT_SECS,
allowed_skew_secs::Int=DEFAULT_SKEW_SECS,
key_refresh_secs::Int=DEFAULT_KEY_REFRESH_SECS),
random_device::RandomDevice=RandomDevice()
)
```
Parameters:
- `issuer`: Issuer URL, pointing to the OpenID server
- `redirect_uri`: The app URI to which OpenID server must redirect after authorization
- `client_id`, and `client_secret`: Client ID and secret that this context represents
- `scopes`: The scopes to request during authorization (default: openid, profile, email)
Keyword Parameters:
- `verify`: whether to validate the server certificate
- `cacrt`: the CA certificate to use to check the server certificate
- `state_timeout_secs`: seconds for which to keep the state associated with an authorization request (default: 60 seconds), server responses beyond this are rejected as stale
- `allowed_skew_secs`: while validating tokens, seconds to allow to account for time skew between machines (default: 120 seconds)
- `key_refresh_secs`: time interval in which to refresh the JWT signing keys (default: 1hr)
# Error Structures
- `OpenIDConnect.APIError`: Error detected at the client side. Members:
- `error`: error code or message (String)
- `OpenIDConnect.AuthServerError`: Error returned from the OpenID server (see section 3.1.2.6 of https://openid.net/specs/openid-connect-core-1_0.html)
- `error`: error code (String)
- `error_description`: optional error description (String)
- `error_uri`: optional error URI (String)
# Authorization Code Flow
## Authentication request.
### `flow_request_authorization_code`
Returns a String with the redirect URL. Caller must perform the redirection.
Acceptable optional args as listed in section 3.1.2.1 of specifications (https://openid.net/specs/openid-connect-core-1_0.html)
```julia
function flow_request_authorization_code(
ctx::OIDCCtx;
nonce=nothing,
display=nothing,
prompt=nothing,
max_age=nothing,
ui_locales=nothing,
id_token_hint=nothing,
login_hint=nothing,
acr_values=nothing
)
```
### `flow_get_authorization_code`
Given the params from the redirected response from the authentication request, extract the authorization code.
See sections 3.1.2.5 and 3.1.2.6 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns the authorization code on success.
Returns one of APIError or AuthServerError on failure.
```julia
function flow_get_authorization_code(
ctx::OIDCCtx,
query # name-value pair Dict with query parameters are received from the OpenID server redirect
)
```
## Token Requests
### `flow_get_token`
Token Request. Given the authorization code obtained, invoke the token end point and obtain an id_token, access_token, refresh_token.
See section 3.1.3.1 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns a JSON object containing tokens on success.
Returns a AuthServerError or APIError object on failure.
```julia
function flow_get_token(
ctx::OIDCCtx,
code
)
```
### `flow_refresh_token`
Token Refresh. Given the refresh code obtained, invoke the token end point and obtain new tokens.
See section 12 of https://openid.net/specs/openid-connect-core-1_0.html.
Returns a JSON object containing tokens on success.
Returns a AuthServerError or APIError object on failure.
```julia
function flow_refresh_token(
ctx::OIDCCtx,
refresh_token
)
```
## Token Validation
### `flow_validate_id_token`
Validate an OIDC token.
Validates both the structure and signature.
See section 3.1.3.7 of https://openid.net/specs/openid-connect-core-1_0.html
```
function flow_validate_id_token(
ctx::OIDCCtx,
id_token::Union{JWTs.JWT, String}
)
```
# Examples
An example application built using OpenIDClient with Mux and HTTP is available as a [tool](tools/oidc_standalone.jl). Populate a configuration file following this [template](tools/settings.template) and start the standalone application. Point your browser to it to experience the complete flow.
| OpenIDConnect | https://github.com/tanmaykm/OpenIDConnect.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 8979 | using BERT
using Knet
import Base: length, iterate
using Random
using CSV
using PyCall
using Dates
VOCABFILE = "bert-base-uncased-vocab.txt"
NUM_CLASSES = 2
LEARNING_RATE = 2e-5
NUM_OF_EPOCHS = 30
TRAIN = true
token2int = Dict()
f = open(VOCABFILE) do file
lines = readlines(file)
for (i,line) in enumerate(lines)
token2int[line] = i
end
end
int2token = Dict(value => key for (key, value) in token2int)
VOCABSIZE = length(token2int)
# include("preprocess.jl")
# include("optimizer.jl")
function convert_to_int_array(text, dict; lower_case=true)
tokens = bert_tokenize(text, dict, lower_case=lower_case)
out = Int[]
for token in tokens
if token in keys(dict)
push!(out, dict[token])
else
push!(out, dict["[UNK]"])
end
end
return out
end
function read_and_process(filename, dict; lower_case=true)
data = CSV.File(filename, delim="\t")
x = Array{Int,1}[]
y = Int8[]
for i in data
push!(x, convert_to_int_array(i.sentence, dict, lower_case=lower_case))
push!(y, Int8(i.label + 1)) # negative 1, positive 2
end
# Padding to maximum
# max_seq = findmax(length.(x))[1]
# for i in 1:length(x)
# append!(x[i], fill(1, max_seq - length(x[i]))) # 1 is for "[PAD]"
# end
return (x, y)
end
mutable struct ClassificationData
input_ids
input_mask
segment_ids
labels
batchsize
ninstances
shuffled
end
function ClassificationData(input_file, token2int; batchsize=8, shuffled=true, seq_len=64)
input_ids = []
input_mask = []
segment_ids = []
labels = []
(x, labels) = read_and_process(input_file, token2int)
for i in 1:length(x)
if length(x[i]) >= seq_len
x[i] = x[i][1:seq_len]
mask = Array{Int64}(ones(seq_len))
else
mask = Array{Int64}(ones(length(x[i])))
append!(x[i], fill(1, seq_len - length(x[i]))) # 1 is for "[PAD]"
append!(mask, fill(0, seq_len - length(mask))) # 0's vanish with masking operation
end
push!(input_ids, x[i])
push!(input_mask, mask)
push!(segment_ids, Array{Int64}(ones(seq_len)))
end
ninstances = length(input_ids)
return ClassificationData(input_ids, input_mask, segment_ids, labels, batchsize, ninstances, shuffled)
end
function length(d::ClassificationData)
d, r = divrem(d.ninstances, d.batchsize)
return r == 0 ? d : d+1
end
function iterate(d::ClassificationData, state=ifelse(d.shuffled, randperm(d.ninstances), 1:d.ninstances))
state === nothing && return nothing
if length(state) > d.batchsize
new_state = state[d.batchsize+1:end]
input_ids = hcat(d.input_ids[state[1:d.batchsize]]...)
input_mask = hcat(d.input_mask[state[1:d.batchsize]]...)
segment_ids = hcat(d.segment_ids[state[1:d.batchsize]]...)
labels = hcat(d.labels[state[1:d.batchsize]]...)
else
new_state = nothing
input_ids = hcat(d.input_ids[state]...)
input_mask = hcat(d.input_mask[state]...)
segment_ids = hcat(d.segment_ids[state]...)
labels = hcat(d.labels[state]...)
end
return ((input_ids, input_mask, segment_ids, labels), new_state)
end
# mutable struct ClassificationData2
# input_ids
# input_mask
# segment_ids
# labels
# batchsize
# ninstances
# shuffled
# end
# function ClassificationData2(input_file; batchsize=8, shuffled=true, seq_len=64)
# input_ids = []
# input_mask = []
# segment_ids = []
# labels = []
# f = open(input_file)
# tmp = split.(readlines(f), "\t")
# for i in 1:length(tmp)
# instance = eval.(Meta.parse.(tmp[i]))
# push!(input_ids, (instance[1] .+ 1)[1:seq_len])
# push!(input_mask, instance[2][1:seq_len])
# push!(segment_ids, (instance[3] .+ 1)[1:seq_len])
# push!(labels, (instance[4] + 1))
# end
# ninstances = length(input_ids)
# return ClassificationData2(input_ids, input_mask, segment_ids, labels, batchsize, ninstances, shuffled)
# end
# function length(d::ClassificationData2)
# d, r = divrem(d.ninstances, d.batchsize)
# return r == 0 ? d : d+1
# end
# function iterate(d::ClassificationData2, state=ifelse(d.shuffled, randperm(d.ninstances), 1:d.ninstances))
# state === nothing && return nothing
# if length(state) > d.batchsize
# new_state = state[d.batchsize+1:end]
# input_ids = hcat(d.input_ids[state[1:d.batchsize]]...)
# input_mask = hcat(d.input_mask[state[1:d.batchsize]]...)
# segment_ids = hcat(d.segment_ids[state[1:d.batchsize]]...)
# labels = hcat(d.labels[state[1:d.batchsize]]...)
# else
# new_state = nothing
# input_ids = hcat(d.input_ids[state]...)
# input_mask = hcat(d.input_mask[state]...)
# segment_ids = hcat(d.segment_ids[state]...)
# labels = hcat(d.labels[state]...)
# end
# return ((input_ids, input_mask, segment_ids, labels), new_state)
# end
# include("model.jl")
# Embedding Size, Vocab Size, Intermediate Hidden Size, Max Sequence Length, Sequence Length, Num of Segments, Num of Heads in Attention, Num of Encoders in Stack, Batch Size, Matrix Type, General Dropout Rate, Attention Dropout Rate, Activation Function
config = BertConfig(768, 30522, 3072, 512, 64, 2, 12, 12, 8, KnetArray{Float32}, 0.1, 0.1, "gelu")
if TRAIN
dtrn = ClassificationData("../project/mytrain.tsv", token2int, batchsize=config.batchsize, seq_len=config.seq_len)
ddev = ClassificationData("../project/dev.tsv", token2int, batchsize=config.batchsize, seq_len=config.seq_len)
else
dtst = ClassificationData("../project/mytest.tsv", token2int, batchsize=config.batchsize, seq_len=config.seq_len)
end
if TRAIN
model = BertClassification(config, NUM_CLASSES)
@pyimport torch
torch_model = torch.load("../project/pytorch_model.bin")
model = load_from_torch_base(model, config.num_encoder, config.atype, torch_model)
end
function accuracy2(model, dtst)
true_count = 0
all_count = 0
for (x, attention_mask, segment_ids, y) in dtst
probs = model(x, segment_ids, attention_mask=attention_mask)
preds = map(x -> x[1], argmax(Array{Float32}(probs),dims=1))
true_count += sum(y .== preds)
all_count += length(y)
end
return true_count/all_count
end
function initopt!(model, t_total; lr=0.001, warmup=0.1)
for par in params(model)
if length(size(value(par))) === 1
par.opt = BertAdam(lr=lr, warmup=warmup, t_total=t_total, w_decay_rate=0.01)
else
par.opt = BertAdam(lr=lr, warmup=warmup, t_total=t_total)
end
end
end
function mytrain!(model, dtrn, ddev, best_acc)
losses = []
accs = []
for (k, (x, attention_mask, segment_ids, labels)) in enumerate(dtrn)
J = @diff model(x, segment_ids, labels, attention_mask=attention_mask)
for par in params(model)
g = grad(J, par)
update!(value(par), g, par.opt)
end
push!(losses, value(J))
if k % 500 == 0
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Training loss up to $k iteration is : ", Knet.mean(losses))
flush(stdout)
acc = accuracy2(model, ddev)
push!(accs, acc)
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Accuracy at $k iteration : ", acc)
flush(stdout)
if acc > best_acc
best_acc = acc
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Saving...")
Knet.save("model_bert.jld2", "model", model)
flush(stdout)
end
end
end
return (best_acc, Knet.mean(losses), accs)
end
if TRAIN
t_total = length(dtrn) * NUM_OF_EPOCHS
initopt!(model, t_total, lr=LEARNING_RATE)
dev_accs = [0.0]
best_acc = 0.0
for epoch in 1:NUM_OF_EPOCHS
global best_acc
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Epoch : ", epoch)
flush(stdout)
(best_acc, lss, acc) = mytrain!(model, dtrn, ddev, best_acc)
append!(dev_accs, acc)
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Training loss for $epoch epoch is : $lss")
println(dev_accs)
flush(stdout)
#=
acc = accuracy2(model, ddev)
println("Accuracy : ", acc)
if acc > best_acc
best_acc = acc
println("Saving...")
Knet.save("model_bert.jld2", "model", model)
end
=#
end
Knet.save("accuracies.jld2", "dev_accs", dev_accs)
else
model = Knet.load("model_bert.jld2", "model")
result = accuracy2(model, dtst)
print(Dates.format(now(), "HH:MM:SS"), " -> ")
println("Test accuracy is : $result")
end
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 3218 | using BERT
using Knet
import Base: length, iterate
using Random
using CSV
using PyCall
VOCABFILE = "bert-base-uncased-vocab.txt"
NUM_CLASSES = 2
token2int = Dict()
f = open(VOCABFILE) do file
lines = readlines(file)
for (i,line) in enumerate(lines)
token2int[line] = i
end
end
int2token = Dict(value => key for (key, value) in token2int)
VOCABSIZE = length(token2int)
mutable struct ClassificationData2
input_ids
input_mask
segment_ids
labels
batchsize
ninstances
shuffled
end
function ClassificationData2(input_file; batchsize=8, shuffled=true, seq_len=64)
input_ids = []
input_mask = []
segment_ids = []
labels = []
f = open(input_file)
tmp = split.(readlines(f), "\t")
for i in 1:length(tmp)
instance = eval.(Meta.parse.(tmp[i]))
push!(input_ids, (instance[1] .+ 1)[1:seq_len])
push!(input_mask, instance[2][1:seq_len])
push!(segment_ids, (instance[3] .+ 1)[1:seq_len])
push!(labels, (instance[4] + 1))
end
ninstances = length(input_ids)
return ClassificationData2(input_ids, input_mask, segment_ids, labels, batchsize, ninstances, shuffled)
end
function length(d::ClassificationData2)
d, r = divrem(d.ninstances, d.batchsize)
return r == 0 ? d : d+1
end
function iterate(d::ClassificationData2, state=ifelse(d.shuffled, randperm(d.ninstances), 1:d.ninstances))
state === nothing && return nothing
if length(state) > d.batchsize
new_state = state[d.batchsize+1:end]
input_ids = hcat(d.input_ids[state[1:d.batchsize]]...)
input_mask = hcat(d.input_mask[state[1:d.batchsize]]...)
segment_ids = hcat(d.segment_ids[state[1:d.batchsize]]...)
labels = hcat(d.labels[state[1:d.batchsize]]...)
else
new_state = nothing
input_ids = hcat(d.input_ids[state]...)
input_mask = hcat(d.input_mask[state]...)
segment_ids = hcat(d.segment_ids[state]...)
labels = hcat(d.labels[state]...)
end
return ((input_ids, input_mask, segment_ids, labels), new_state)
end
# Embedding Size, Vocab Size, Intermediate Hidden Size, Max Sequence Length, Sequence Length, Num of Segments, Num of Heads in Attention, Num of Encoders in Stack, Batch Size, Matrix Type, General Dropout Rate, Attention Dropout Rate, Activation Function
config = BertConfig(768, 30522, 3072, 512, 64, 2, 12, 12, 8, KnetArray{Float32}, 0.1, 0.1, "gelu")
dtst = ClassificationData2("../project/sst-test.tsv", batchsize=config.batchsize, seq_len=config.seq_len)
model = BertClassification(config, NUM_CLASSES)
@pyimport torch
torch_model = torch.load("../project/model-64-32.pt")
model = load_from_torch_classification(model, config.num_encoder, config.atype, torch_model)
function accuracy2(model, dtst)
true_count = 0
all_count = 0
for (x, attention_mask, segment_ids, y) in dtst
probs = model(x, segment_ids, attention_mask=attention_mask)
preds = map(x -> x[1], argmax(Array{Float32}(probs),dims=1))
true_count += sum(y .== preds)
all_count += length(y)
end
return true_count/all_count
end
result = accuracy2(model, dtst)
println("Test accuracy is : $result")
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 398 | using BERT
config = BertConfig(128, 30022, 256, 512, 4, 2, 8, 2, 3, Array{Float32}, 0.1, 0.1, "relu")
model = BertPreTraining(config)
x = [213 234 7789; 712 9182 8912; 7812 12 432; 12389 1823 8483] # 4x3
segment_ids = [1 1 1;1 2 1;1 2 1;1 1 1]
mlm_labels = [-1 234 -1; -1 -1 8912; -1 -1 -1; 12389 -1 -1]
nsp_labels = [1, 2, 1]
loss = model(x, segment_ids, mlm_labels, nsp_labels)
println(loss)
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 337 | module BERT
export
BertPreTraining,
BertClassification,
BertConfig,
load_from_torch_base,
load_from_torch_pretraining,
load_from_torch_classification,
BertAdam,
bert_tokenize
using Knet, SpecialFunctions, LinearAlgebra
include("model.jl")
include("optimizer.jl")
include("preprocess.jl")
end # module
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 19559 | # import Base: *
# import Knet: getindex, setindex!
# Matmuls 2d and 3d arrays
# function *(a::AbstractArray{T,2}, b::AbstractArray{T,3}) where T<:Real
# b_sizes = size(b)
# a = a * reshape(b, b_sizes[1], :)
# return reshape(a, :, b_sizes[2:end]...)
# end
# Matmuls 2d and 3d arrays for KnetArrays
# function *(a::KnetArray{T,2}, b::KnetArray{T,3}) where T<:Real
# b_sizes = size(b)
# a = a * reshape(b, b_sizes[1], :)
# return reshape(a, :, b_sizes[2:end]...)
# end
# TODO :
# Since backprop doesn't work with this new import, we define it as a complex function consisting primitives that Autograd can take derivatives of. A primitive derivative of this function would speed things up.
# function matmul23(a::KnetArray{T,2}, b::KnetArray{T,3}) where T<:Real
# b_sizes = size(b)
# a = a * reshape(b, b_sizes[1], :)
# return reshape(a, :, b_sizes[2:end]...)
# end
gelu(x) = x .* 0.5 .* (1.0 .+ erf.(x ./ sqrt(2.0)))
function matmul23(a, b)
b_sizes = size(b)
a = a * reshape(b, b_sizes[1], :)
return reshape(a, :, b_sizes[2:end]...)
end
# Wrote these first, then realized we don't need them. Might come in handy later.
# function matmul23(a::AbstractArray{T,2}, b::AbstractArray{T,3}) where T<:Real
# b_sizes = size(b)
# a = a * reshape(b, b_sizes[1], :)
# return reshape(a, :, b_sizes[2:end]...)
# end
# function matmul23(a::Param{KnetArray{T,2}}, b::KnetArray{T,3}) where T<:Real
# matmul23(value(a), b)
# end
# function matmul23(a::Param{AbstractArray{T,2}}, b::AbstractArray{T,3}) where T<:Real
# matmul23(value(a), b)
# end
# function matmul23(a::Param{KnetArray{T,2}}, b::AutoGrad.Result{KnetArray{T,3}}) where T<:Real
# matmul23(value(a), value(b))
# end
# function matmul23(a::Param{AbstractArray{T,2}}, b::AutoGrad.Result{AbstractArray{T,3}}) where T<:Real
# matmul23(value(a), value(b))
# end
# @primitive *(x1::KnetArray{T,2},x2::KnetArray{T,3}),dy
# Not using this anymore
# function getindex(A::KnetArray{Float32,3}, ::Colon, I::Real, ::Colon)
# sizes = size(A)
# A = reshape(A, :, sizes[3])
# return A[(I-1)*sizes[1]+1:I*sizes[1],:]
# # reshape(A, :, size(A,3))[(I-1)*size(A,1)+1:I*size(A,1),:]
# end
# Does not work
# function setindex!(A::KnetArray{Float32,3}, v, ::Colon, I::Real, ::Colon)
# A = reshape(A, :, size(A,3))
# # setindex!(A, v, (I-1)*size(A,1)+1:I*size(A,1), ::Colon)
# A[(I-1)*size(A,1)+1:I*size(A,1),:] = v
# end
# std doesn't work!
std2(a, μ, ϵ) = sqrt.(Knet.mean(abs2.(a .- μ), dims=1) .+ ϵ)
# Legend
# V -> Vocab size, E -> Embedding size, S -> Sequence length, B -> Batch size
# H -> head_size, N -> num_heads
abstract type Layer end
# MAYBE TODO : sin-cos positionwise embeddings. This will reduce model size by max_seq_len * E
mutable struct Embedding <: Layer
w
end
Embedding(vocabsize::Int,embed::Int; atype=Array{Float32}) = Embedding(param(embed,vocabsize, atype=atype))
function (e::Embedding)(x)
e.w[:,x]
end
# If we need 0's as pads
#=
struct SegmentEmbedding <: Layer
w
atype
end
SegmentEmbedding(vocabsize::Int,embed::Int; atype=Array{Float32}) = SegmentEmbedding(param(embed,vocabsize, atype=atype), atype)
function (e::SegmentEmbedding)(x)
x != 0 ? e.w[:,x] : e.atype(zeros(size(e.w,1)))
end
=#
mutable struct Linear <: Layer
w
b
end
Linear(input_size::Int, output_size::Int; atype=Array{Float32}) = Linear(param(output_size, input_size, atype=atype), param0(output_size, atype=atype))
function (l::Linear)(x)
return l.w * x .+ l.b
end
mutable struct Linear3D <: Layer
w
b
end
Linear3D(input_size::Int, output_size::Int; atype=Array{Float32}) = Linear3D(param(output_size, input_size, atype=atype), param0(output_size, atype=atype))
function (l::Linear3D)(x)
return matmul23(l.w, x) .+ l.b
end
# Absolutely no difference between Dense and Linear! Except one has dropout and activation function.
mutable struct Dense <: Layer
linear
pdrop
func
end
function Dense(input_size::Int, output_size::Int; pdrop=0.0, func=identity, atype=Array{Float32}, threeD=false)
if threeD
return Dense(Linear3D(input_size, output_size, atype=atype), pdrop, func)
else
return Dense(Linear(input_size, output_size, atype=atype), pdrop, func)
end
end
function (a::Dense)(x)
return a.func.(dropout(a.linear(x), a.pdrop))
end
mutable struct LayerNormalization <: Layer
γ
β
ϵ
end
LayerNormalization(hidden_size::Int; epsilon=1e-12, atype=Array{Float32}) = LayerNormalization(Param(atype(ones(hidden_size))), param0(hidden_size, atype=atype), epsilon)
function (n::LayerNormalization)(x)
μ = Knet.mean(x, dims=1)
x = (x .- μ) ./ std2(x, μ, n.ϵ) # corrected=false for n
return n.γ .* x .+ n.β
end
mutable struct EmbedLayer <: Layer
wordpiece::Embedding
positional::Embedding
# segment::SegmentEmbedding
segment::Embedding
layer_norm::LayerNormalization
seq_len::Int
pdrop
end
function EmbedLayer(config)
wordpiece = Embedding(config.vocab_size, config.embed_size, atype=config.atype)
positional = Embedding(config.max_seq_len, config.embed_size, atype=config.atype)
#segment = SegmentEmbedding(config.num_segment, config.embed_size, atype=config.atype)
segment = Embedding(config.num_segment, config.embed_size, atype=config.atype)
layer_norm = LayerNormalization(config.embed_size, atype=config.atype)
return EmbedLayer(wordpiece, positional, segment, layer_norm, config.seq_len, config.pdrop)
end
function (e::EmbedLayer)(x, segment_ids) # segment_ids are SxB, containing 1 or 2, or 0 in case of pads.
x = e.wordpiece(x)
positions = zeros(Int64, e.seq_len, size(x,3)) .+ collect(1:e.seq_len) # size(x,3) is batchsize. Resulting matrix is SxB
x = x .+ e.positional(positions)
#x .+= reshape(hcat(e.segment.(segment_ids)...), (:, size(segment_ids,1),size(segment_ids,2)))
x = x .+ e.segment(segment_ids)
x = e.layer_norm(x)
return dropout(x, e.pdrop)
end
function divide_to_heads(x, num_heads, head_size, seq_len)
x = reshape(x, (head_size, num_heads, seq_len, :))
x = permutedims(x, (1,3,2,4))
return reshape(x, (head_size, seq_len, :)) # Reshape to 3D so bmm can handle it.
end
mutable struct SelfAttention <: Layer
query::Linear3D # N*H x E
key::Linear3D
value::Linear3D
linear::Linear3D
num_heads::Int
seq_len::Int
embed_size::Int
head_size::Int
head_size_sqrt::Int
attention_pdrop
pdrop
end
function SelfAttention(config)
config.embed_size % config.num_heads != 0 && throw("Embed size should be divisible by number of heads!")
head_size = Int(config.embed_size / config.num_heads)
head_size_sqrt = Int(sqrt(head_size))
head_size_sqrt * head_size_sqrt != head_size && throw("Square root of head size should be an integer!")
query = Linear3D(config.embed_size, head_size*config.num_heads, atype=config.atype) # H*N is always equal to E
key = Linear3D(config.embed_size, head_size*config.num_heads, atype=config.atype)
value = Linear3D(config.embed_size, head_size*config.num_heads, atype=config.atype)
linear = Linear3D(config.embed_size, config.embed_size, atype=config.atype)
return SelfAttention(query, key, value, linear, config.num_heads, config.seq_len, config.embed_size, head_size, head_size_sqrt, config.attention_pdrop, config.pdrop)
end
function (s::SelfAttention)(x, attention_mask)
# We make all the batchsize ones colon, in case of batches smaller than batchsize.
# x is ExSxB
query = divide_to_heads(s.query(x), s.num_heads, s.head_size, s.seq_len) # H x S x N*B
key = divide_to_heads(s.key(x), s.num_heads, s.head_size, s.seq_len)
value = divide_to_heads(s.value(x), s.num_heads, s.head_size, s.seq_len)
# Scaled Dot Product Attention
query = bmm(permutedims(key, (2,1,3)), query)
query = query ./ s.head_size_sqrt # Scale down. I init this value to avoid taking sqrt every forward operation.
# Masking. First reshape to 4d, then add mask, then reshape back to 3d.
query = reshape(reshape(query, (s.seq_len, s.seq_len, s.num_heads, :)) .+ attention_mask, (s.seq_len, s.seq_len, :))
query = Knet.softmax(query, dims=1)
query = dropout(query, s.attention_pdrop)
query = bmm(value, query)
query = permutedims(reshape(query, (s.head_size, s.seq_len, s.num_heads, :)), (1,3,2,4))
query = reshape(query, (s.embed_size, s.seq_len, :)) # Concat
return dropout(s.linear(query), s.pdrop) # Linear transformation at the end
# In pytorch version dropout is after layer_norm!
end
mutable struct FeedForward <: Layer
dense::Dense
linear::Linear3D
pdrop
end
function FeedForward(config)
dense = Dense(config.embed_size, config.ff_hidden_size, func=eval(Meta.parse(config.func)), atype=config.atype, threeD=true)
linear = Linear3D(config.ff_hidden_size, config.embed_size, atype=config.atype)
return FeedForward(dense, linear, config.pdrop)
end
function (f::FeedForward)(x)
x = f.dense(x)
return dropout(f.linear(x), f.pdrop)
end
mutable struct Encoder <: Layer
self_attention::SelfAttention
layer_norm1::LayerNormalization
feed_forward::FeedForward
layer_norm2::LayerNormalization
end
function Encoder(config)
return Encoder(SelfAttention(config), LayerNormalization(config.embed_size, atype=config.atype), FeedForward(config), LayerNormalization(config.embed_size, atype=config.atype))
end
function (e::Encoder)(x, attention_mask)
x = e.layer_norm1(x .+ e.self_attention(x, attention_mask))
return e.layer_norm2(x .+ e.feed_forward(x))
end
mutable struct Bert <: Layer
embed_layer::EmbedLayer
encoder_stack
atype
end
function Bert(config)
embed_layer = EmbedLayer(config)
encoder_stack = Encoder[]
for _ in 1:config.num_encoder
push!(encoder_stack, Encoder(config))
end
return Bert(embed_layer, encoder_stack, config.atype)
end
# x and segment_ids are SxB integers
function (b::Bert)(x, segment_ids; attention_mask=nothing)
# Init attention_mask if it's not given
attention_mask = attention_mask == nothing ? ones(size(x)) : attention_mask
attention_mask = reshape(attention_mask, (size(attention_mask,1), 1, 1, size(attention_mask,2))) # Make it 4d
attention_mask = (1 .- attention_mask) .* -10000.0 # If integer was 0, now it is masking. ones(size(attention_mask))
attention_mask = b.atype(attention_mask)
x = b.embed_layer(x, segment_ids)
for encoder in b.encoder_stack
x = encoder(x, attention_mask)
end
return x
end
mutable struct Pooler <: Layer
linear::Linear
end
Pooler(embed_size::Int; atype=Array{Float32}) = Pooler(Linear(embed_size, embed_size, atype=atype))
function (p::Pooler)(x)
# TODO :
# Gave up on getindex function for 3D matrices because I could not figure out how to write setindex! for backprop
# x = reshape(x, :, size(x,3))
# return tanh.(p.linear(x[:,1,:])) # Use only CLS token. Returns ExB
return tanh.(p.linear(reshape(x, :, size(x,3))[1:size(x,1),:]))
end
mutable struct NSPHead <: Layer
linear::Linear
end
NSPHead(embed_size::Int; atype=Array{Float32}) = NSPHead(Linear(embed_size, 2, atype=atype))
(n::NSPHead)(x) = n.linear(x)
mutable struct MLMHead <: Layer
dense::Dense
layer_norm::LayerNormalization
linear::Linear3D
end
function MLMHead(config, embedding_matrix)
dense = Dense(config.embed_size, config.embed_size, func=eval(Meta.parse(config.func)), pdrop=0.0, atype=config.atype, threeD=true)
layer_norm = LayerNormalization(config.embed_size, atype=config.atype)
linear = Linear3D(config.embed_size, config.vocab_size, atype=config.atype)
# TODO : Do this a shared weight
#linear.w = permutedims(embedding_matrix, (2,1))
return MLMHead(dense, layer_norm, linear)
end
function (m::MLMHead)(x)
x = m.dense(x)
x = m.layer_norm(x)
return m.linear(x)
end
mutable struct BertPreTraining <: Layer
bert::Bert
pooler::Pooler
nsp::NSPHead
mlm::MLMHead
end
function BertPreTraining(config)
bert = Bert(config)
pooler = Pooler(config.embed_size, atype=config.atype)
nsp = NSPHead(config.embed_size, atype=config.atype)
mlm = MLMHead(config, bert.embed_layer.wordpiece.w) # TODO : Dont forget about embedding matrix
return BertPreTraining(bert, pooler, nsp, mlm)
end
# We do not need a predictor, since this is only for pretraining
function (b::BertPreTraining)(x, segment_ids, mlm_labels, nsp_labels; attention_mask=nothing) # mlm_labels are SxB, so we just flatten them.
x = b.bert(x, segment_ids, attention_mask=attention_mask)
nsp_preds = b.nsp(b.pooler(x)) # 2xB
mlm_preds = b.mlm(x) # VxSxB
mlm_preds = reshape(mlm_preds, size(mlm_preds, 1), :) # VxS*B
nsp_loss = nll(nsp_preds, nsp_labels)
mlm_labels = reshape(mlm_labels, :) # S*B
mlm_loss = nll(mlm_preds[:,mlm_labels.!=-1], mlm_labels[mlm_labels.!=-1])
return mlm_loss + nsp_loss
end
function (b::BertPreTraining)(dtrn)
lvals = []
for (x, attention_mask, segment_ids, mlm_labels, nsp_labels) in dtrn
push!(lvals, b(x, segment_ids, mlm_labels, nsp_labels, attention_mask=attention_mask))
end
return Knet.mean(lvals)
end
mutable struct BertClassification <: Layer
bert::Bert
pooler::Pooler
linear::Linear
pdrop
end
function BertClassification(config, num_of_classes)
bert = Bert(config)
pooler = Pooler(config.embed_size, atype=config.atype)
linear = Linear(config.embed_size, num_of_classes, atype=config.atype)
return BertClassification(bert, pooler, linear, config.pdrop)
end
function (b::BertClassification)(x, segment_ids; attention_mask=nothing)
x = b.bert(x, segment_ids, attention_mask=attention_mask)
x = dropout(b.pooler(x), b.pdrop) # 2xB
return b.linear(x)
end
function (b::BertClassification)(x, segment_ids, y; attention_mask=nothing)
return nll(b(x, segment_ids, attention_mask=attention_mask), y)
end
function (b::BertClassification)(dtrn)
lvals = []
for (x, attention_mask, segment_ids, y) in dtrn
push!(lvals, b(x, segment_ids, y, attention_mask=attention_mask))
end
return Knet.mean(lvals)
end
mutable struct BertConfig
embed_size::Int
vocab_size::Int
ff_hidden_size::Int
max_seq_len::Int
seq_len::Int
num_segment::Int
num_heads::Int
num_encoder::Int
batchsize::Int
atype
pdrop
attention_pdrop
func
end
function load_from_torch_base(model, num_encoder, atype, torch_model)
# Embed Layer
model.bert.embed_layer.wordpiece.w = Param(atype(permutedims(torch_model["bert.embeddings.word_embeddings.weight"][:cpu]()[:numpy](), (2,1))))
model.bert.embed_layer.positional.w = Param(atype(permutedims(torch_model["bert.embeddings.position_embeddings.weight"][:cpu]()[:numpy](), (2,1))))
model.bert.embed_layer.segment.w = Param(atype(permutedims(torch_model["bert.embeddings.token_type_embeddings.weight"][:cpu]()[:numpy](), (2,1))))
model.bert.embed_layer.layer_norm.γ = Param(atype(torch_model["bert.embeddings.LayerNorm.gamma"][:cpu]()[:numpy]()))
model.bert.embed_layer.layer_norm.β = Param(atype(torch_model["bert.embeddings.LayerNorm.beta"][:cpu]()[:numpy]()))
# Encoder Stack
for i in 1:num_encoder
model.bert.encoder_stack[i].self_attention.query.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.query.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.query.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.query.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.key.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.key.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.key.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.key.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.value.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.value.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.value.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.self.value.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.linear.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.output.dense.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].self_attention.linear.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.output.dense.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].layer_norm1.γ = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.output.LayerNorm.gamma"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].layer_norm1.β = Param(atype(torch_model["bert.encoder.layer.$(i-1).attention.output.LayerNorm.beta"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].feed_forward.dense.linear.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).intermediate.dense.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].feed_forward.dense.linear.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).intermediate.dense.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].feed_forward.linear.w = Param(atype(torch_model["bert.encoder.layer.$(i-1).output.dense.weight"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].feed_forward.linear.b = Param(atype(torch_model["bert.encoder.layer.$(i-1).output.dense.bias"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].layer_norm2.γ = Param(atype(torch_model["bert.encoder.layer.$(i-1).output.LayerNorm.gamma"][:cpu]()[:numpy]()))
model.bert.encoder_stack[i].layer_norm2.β = Param(atype(torch_model["bert.encoder.layer.$(i-1).output.LayerNorm.beta"][:cpu]()[:numpy]()))
end
# Pooler
model.pooler.linear.w = Param(atype(torch_model["bert.pooler.dense.weight"][:cpu]()[:numpy]()))
model.pooler.linear.b = Param(atype(torch_model["bert.pooler.dense.bias"][:cpu]()[:numpy]()))
return model
end
function load_from_torch_pretraining(model, num_encoder, atype, torch_model)
model = load_from_torch_base(model, num_encoder, atype, torch_model)
# NSP Head
model.nsp.linear.w = Param(atype(torch_model["cls.seq_relationship.weight"][:cpu]()[:numpy]()))
model.nsp.linear.b = Param(atype(torch_model["cls.seq_relationship.bias"][:cpu]()[:numpy]()))
# MLM Head.
model.mlm.dense.linear.w = Param(atype(torch_model["cls.predictions.transform.dense.weight"][:cpu]()[:numpy]()))
model.mlm.dense.linear.b = Param(atype(torch_model["cls.predictions.transform.dense.bias"][:cpu]()[:numpy]()))
model.mlm.layer_norm.γ = Param(atype(torch_model["cls.predictions.transform.LayerNorm.gamma"][:cpu]()[:numpy]()))
model.mlm.layer_norm.β = Param(atype(torch_model["cls.predictions.transform.LayerNorm.beta"][:cpu]()[:numpy]()))
model.mlm.linear.w = Param(atype(torch_model["cls.predictions.decoder.weight"][:cpu]()[:numpy]()))
model.mlm.linear.b = Param(atype(torch_model["cls.predictions.bias"][:cpu]()[:numpy]()))
return model
end
function load_from_torch_classification(model, num_encoder, atype, torch_model)
model = load_from_torch_base(model, num_encoder, atype, torch_model)
model.linear.w = Param(atype(torch_model["classifier.weight"][:cpu]()[:numpy]()))
model.linear.b = Param(atype(torch_model["classifier.bias"][:cpu]()[:numpy]()))
return model
end
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 1751 | import Knet: update!
warmup_cosine(x, warmup=0.002) = x < warmup ? x/warmup : 0.5 * (1.0 + cos(π * x))
warmup_constant(x, warmup=0.002) = x < warmup ? x/warmup : 1.0
warmup_linear(x, warmup=0.002) = x < warmup ? x/warmup : 1.0 - x
mutable struct BertAdam
lr::AbstractFloat
beta1::AbstractFloat
beta2::AbstractFloat
eps::AbstractFloat
t::Int
gclip::AbstractFloat
fstm
scndm
w_decay_rate::AbstractFloat
schedule
warmup
t_total
end
BertAdam(; lr=0.001, gclip=1.0, beta1=0.9, beta2=0.999, eps=1e-6, w_decay_rate=0.0, schedule="warmup_linear", warmup=-1, t_total=-1)=BertAdam(lr, beta1, beta2, eps, 0, gclip, nothing, nothing, w_decay_rate, schedule, warmup, t_total)
for T in (Array{Float32},Array{Float64},KnetArray{Float32},KnetArray{Float64}); @eval begin
function update!(w::$T, g::$T, p::BertAdam)
Knet.gclip!(g, p.gclip)
if p.fstm===nothing; p.fstm=zero(w); p.scndm=zero(w); end
lmul!(p.beta1, p.fstm)
axpy!(1-p.beta1, g, p.fstm)
lmul!(p.beta2, p.scndm)
axpy!(1-p.beta2, g .* g, p.scndm)
# They don't do bias correction for some reason
#fstm_corrected = p.fstm / (1 - p.beta1 ^ p.t)
#scndm_corrected = p.scndm / (1 - p.beta2 ^ p.t)
if p.t_total !== -1
schedule_func = eval(Meta.parse(p.schedule))
lr_scheduled = p.lr * schedule_func(p.t/p.t_total, p.warmup)
else
lr_scheduled = p.lr
end
if p.w_decay_rate > 0.0
axpy!(-lr_scheduled, (p.fstm ./ (sqrt.(p.scndm) .+ p.eps)) .+ (p.w_decay_rate * w), w)
else
axpy!(-lr_scheduled, (p.fstm ./ (sqrt.(p.scndm) .+ p.eps)), w)
end
p.t += 1
end
end;end
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | code | 1689 | function wordpiece_tokenize(token, dict)
# This is a longest-match-first algorithm.
out_tokens = []
start = 1
while start <= length(token)
finish = length(token)
final_token = ""
for i in finish:-1:start
# String Indexing Error for an unknown reason. Might be because of unicode chars.
tkn = try
start == 1 ? token[start:i] : string("##", token[start:i])
catch
""
end
if tkn in keys(dict)
final_token = tkn
finish = i
break
end
end
if final_token == "" # if there is no match at all, assign unk token
return ["[UNK]"]
end
push!(out_tokens, final_token)
start = finish + 1
end
return out_tokens
end
function process_punc(tokens)
out_tokens = []
for token in tokens
out = []
str = ""
for (i, char) in enumerate(token)
if ispunct(char)
str != "" && push!(out, str)
str = ""
push!(out, string(char))
else
str = string(str, char)
end
end
str != "" && push!(out, str)
append!(out_tokens, out)
end
return out_tokens
end
function bert_tokenize(text, dict; lower_case=true)
text = strip(text)
text == "" && return []
if lower_case
text = lowercase(text)
end
tokens = split(text)
tokens = process_punc(tokens)
out_tokens = []
for token in tokens
append!(out_tokens, wordpiece_tokenize(token, dict))
end
return out_tokens
end
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"Apache-2.0"
] | 0.1.0 | 5dd50891df13013c7551fd92b1f37f4cdac9976b | docs | 197 | # BERT-in-Knet
This repo is for the final project for Comp541 Deep Learning class in Koc University.
This is a replication attempt of the original https://github.com/google-research/bert in Knet.
| BERT | https://github.com/OsmanMutlu/BERT.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 649 |
if Base.active_project() != joinpath(@__DIR__, "Project.toml")
import Pkg
Pkg.activate(@__DIR__)
Pkg.develop(Pkg.PackageSpec(; path=(@__DIR__) * "/../"))
Pkg.resolve()
Pkg.instantiate()
end
using Documenter
using ManifoldGroupTesting
makedocs(
sitename = "ManifoldGroupTesting",
format = Documenter.HTML(),
modules = [ManifoldGroupTesting]
)
# Documenter can also automatically deploy documentation to gh-pages.
# See "Hosting Documentation" and deploydocs() in the Documenter manual
# for more information.
deploydocs(
repo="github.com/olivierverdier/ManifoldGroupTesting.jl.git",
push_preview=true,
)
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 2026 |
_compose_dir(G, χ1, χ2, ::LeftAction) = compose(G, χ1, χ2)
_compose_dir(G, χ1, χ2, ::RightAction) = compose(G, χ2, χ1)
"""
check_action_morphism(α::GroupAction, χ1, χ2, p)
For a group action `α`,
```math
α(χ_1, α(χ_2, p)) = α(m(χ_1, χ_2), p)
```
where for a *left* action
```math
m(χ_1, χ_2) = χ_1 χ_2
```
and for a *right* action
```math
m(χ_1, χ_2) = χ_2 χ_1
```
"""
check_action_morphism(A::AbstractGroupAction{TAD}, χ1, χ2, p) where {TAD} = begin
G = base_group(A)
M = group_manifold(A)
prod_first = apply(A, _compose_dir(G, χ1, χ2, TAD()), p)
act_first = apply(A, χ1, apply(A, χ2, p))
return isapprox(M, prod_first, act_first)
end
"""
check_apply_morphism_Identity(α::AbstractGroupAction, p)
For any action ``α`` and ``p`` in the manifold, one has
```math
α(1, p) = p
```
where ``1`` denotes ``Identity(G)`` where ``G`` is the
action group.
"""
check_apply_morphism_Identity(A::AbstractGroupAction, p) = begin
G = base_group(A)
p_ = apply(A, Identity(G), p)
return isapprox(group_manifold(A), p, p_)
end
"""
check_trivial_infinitesimal_action(A::GroupAction, p)
For an action ``α`` and a point ``p∈ M``,
consider the function ``f : G → M`` defined as
``f(χ) := α(χ, p)``.
The tangent map at identity ``D := T f(1)`` is a linear map
and sends zero to zero:
```math
D 0 = 0
```
"""
check_trivial_infinitesimal_action(A::AbstractGroupAction, p, id=Identity) = begin
G = base_group(A)
ξ = zero_vector(G, id(G))
computed = apply_diff_group(A, Identity(G), ξ, p)
M = group_manifold(A)
expected = zero_vector(M, p)
TM = TangentSpace(group_manifold(A), p)
return isapprox(TM, computed, expected)
end
"""
check_switch_action_direction(α::GroupAction, χ, p)
For any group action ``α`` and its dual ``α'`` obtained
by `switch_direction` one has
```math
α(χ,p) = α'(χ^{-1}, p)
```
"""
check_switch_action_direction(A::AbstractGroupAction, χ, p) = isapprox(group_manifold(A), apply(switch_direction(A), χ, p), apply(A, inv(base_group(A), χ), p))
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 1978 |
#--------------------------------
_get_side_from_action_dir(::LeftAction) = RightSide()
_get_side_from_action_dir(::RightAction) = LeftSide()
_transporter(G, χ, ξ, dir) = translate_from_id(G, χ, ξ, _get_side_from_action_dir(dir))
"""
check_apply_diff_group(
A::AbstractGroupAction{TAD}, # group action G ⊂ Diff(M)
χ, # element of G
ξ, # element of Alg(G)
p, # element of M
id_func, # `identity_element` or `Identity`
)
This should hold for *any* group action ``A`` on any manifold.
If you define ``π_p(χ) := A(χ, p)`` for ``χ ∈ G`` and ``p ∈ M``,
and define, for ``ξ ∈ Alg(G)``,
``T_R(χ, ξ) := ξχ`` (the right translation),
and ``T_L(χ, ξ) := χξ`` (the left translation), then we have the identity:
```math
⟨Dπ_{p}(χ), T(χ, ξ)⟩ = ⟨Dπ_{A(χ,p)}(1), ξ⟩
```
where, for a *left* action, ``T`` is the *right* translation,
and for a *right* action, ``T`` is the *left* translation.
"""
check_apply_diff_group(A::AbstractGroupAction{TAD}, χ, ξ, p, id_func=Identity) where {TAD} = begin
G = base_group(A)
p_ = apply(A, χ, p)
v1 = apply_diff_group(A, χ, _transporter(G, χ, ξ, TAD()), p)
v2 = apply_diff_group(A, id_func(G), ξ, p_)
return isapprox(TangentSpace(G, p_), v1, v2)
end
#--------------------------------
"""
check_inv_diff(
G, # Group
χ, # group element
ξ, # Lie algebra element
side::Manifolds.GroupActionSide,
)
Test the differential of the inverse on a Lie group `G`.
Denote this inverse by ``I(χ) := χ^{-1}``.
If the left and right transports are ``T_L(χ,ξ) := χξ``
and ``T_R(χ,ξ) := ξχ`` respectively, then
```math
⟨DI(χ), T_L(χ,ξ)⟩ = -T_R(χ^{-1}, ξ)
```
and
``` math
⟨DI(χ), T_R(χ,ξ)⟩ = -T_L(χ^{-1}, ξ)
```
"""
check_inv_diff(G, χ, ξ, side) = begin
χ_ = inv(G, χ)
computed = inv_diff(G, χ, translate_from_id(G, χ, ξ, side))
expected = -translate_from_id(G, χ_, ξ, switch_side(side))
return isapprox(TangentSpace(G, χ_), computed, expected)
end
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 5341 | using ManifoldGroupUtils
import ManifoldGroupUtils: matrix_from_lin_endomorphism
"""
A collection of general purpose methods
for testing Lie groups.
"""
"""
``Ad`` and ``exp`` commute:
```math
Ad_{exp(ξ_1)}ξ_2 = exp(ad_{ξ_1}) ξ_2
```
"""
function check_exp_ad(G, vel, tvel)
χ = exp_lie(G, vel)
Ad_exp = adjoint_action(G, χ, tvel)
lie_bracket(G, vel, tvel)
B = DefaultOrthogonalBasis()
der = matrix_from_lin_endomorphism(G, ξ -> lie_bracket(G, vel, ξ), B)
mor = exp(der)
tvel_coord = get_coordinates_lie(G, tvel, B)
exp_ad = get_vector_lie(G, mor * tvel_coord, B)
return isapprox(algebra(G), Ad_exp, exp_ad)
end
"""
check_adjoint_action(G, grp_rep, alg_rep, χ, ξ)
The group representation ``ρ`` and algebra representation ``ρ``
commute with the adjoint action:
```math
ρ(χ) ρ(ξ) ρ(χ)^{-1} = ρ(Ad_χ (ξ))
```
"""
check_adjoint_action(G, grp_rep, alg_rep, χ, ξ) = begin
mat = grp_rep(G, χ)
matinv = inv(mat)
expected = mat * alg_rep(G, ξ) * matinv
computed = alg_rep(G, adjoint_action(G, χ, ξ))
return isapprox(computed, expected)
end
"""
check_inv_rep(G, grp_rep, χ)
The group representation ``ρ`` commutes with the inverse.
```math
ρ(χ)^{-1} = ρ(χ^{-1})
```
"""
check_inv_rep(G, grp_rep, χ) = begin
computed = grp_rep(G, inv(G, χ))
expected = inv(grp_rep(G, χ))
return isapprox(computed, expected)
end
_switch_sign(ξ, ::LeftSide) = ξ
_switch_sign(ξ, ::RightSide) = -ξ
"""
check_apply_diff_group_at_id(G, side::GroupActionSide)
The left group operation action on itself ``α(χ_1)χ_2``
is either (left side)
```math
α(χ_1)χ_2 = χ_1 χ_2
```
or (right side)
```math
α(χ_1)χ_2 = χ_2 χ_1^{-1}
```
Now fix ``χ_2 = 1`` (1 is the identity of ``G``) and define ``f : G → G`` by ``f(χ) := α(χ) 1``. Since ``f(1) = 1``,
its differential at identity is a map ``Alg(G) → Alg(G)``.
This map is either
- ``Id`` (left side)
- ``-Id`` (right side)
"""
check_apply_diff_group_at_id(G, ξ, side::Manifolds.GroupActionSide, id_func=Identity) = begin
id = id_func(G)
ξ_ = apply_diff_group(GroupOperationAction(G, (LeftAction(), side)), id, ξ, id)
return isapprox(algebra(G), ξ, _switch_sign(ξ_, side))
end
"""
check_exp_lie_point(G, ξ)
The Lie group exponential sends the vector ξ
to an element in the group.
"""
check_exp_lie_point(G, ξ) = is_point(G, exp_lie(G, ξ))
"""
check_adjoint_action_in_alg(G, χ, ξ)
The adjoint action of χ on ξ is an element of Alg(G):
```math
Ad_{χ}ξ ∈ Alg(G)
```
"""
check_adjoint_action_in_alg(G, χ, ξ) = is_vector(G, identity_element(G), adjoint_action(G, χ, ξ))
"""
check_grp_rep_Identity(G)
The representation works at the Identity(G) point.
"""
check_grp_rep_Identity(G, grp_rep) = begin
expected = grp_rep(G, identity_element(G))
computed = grp_rep(G, Identity(G))
return isapprox(expected, computed)
end
"""
check_grp_rep_compose(G, ρ, χ1, χ2)
The group representation ``ρ`` commutes with composition.
```math
ρ(χ_1 χ_2) = ρ(χ_1) ρ(χ_2)
```
where the latter is a matrix multiplication.
"""
check_grp_rep_compose(G, grp_rep, χ1, χ2) = begin
m1, m2 = [grp_rep(G, p) for p in [χ1, χ2]]
expected = m1 * m2
computed = grp_rep(G, compose(G, χ1, χ2))
return isapprox(expected, computed)
end
"""
check_alg_rep(G, alg_rep, ξ1, ξ2)
The algebra representation ``ρ`` is an algebra morphism.
```math
ρ([ξ_1, ξ_2]) = [ρ(ξ_1), ρ(ξ_2)]
```
where the latter is a matrix commutator.
"""
check_alg_rep(G, alg_rep, ξ1, ξ2) = begin
m1, m2 = [alg_rep(G, v) for v in [ξ1,ξ2]]
expected = m1*m2 - m2*m1
computed = alg_rep(G, lie_bracket(G, ξ1, ξ2))
return isapprox(expected, computed)
end
check_zero_Identity(G) = isapprox(algebra(G),
zero_vector(G, Identity(G)),
zero_vector(G, identity_element(G)))
"""
check_exp_invariant(G, exp, χ, v, χ_)
The invariant exponential of a Lie group fulfils
```math
χ' \exp_{χ}(v) = \exp_{χ'χ}(χ' v)
```
There is a right version which is not implemented. It would check that
```math
\exp_χ(v) χ' = \exp_{χχ'}(v χ')
```
"""
check_exp_invariant(G, exp, χ, v, χ_) = begin
χ1 = translate(G, χ_, exp(G, χ, v), (LeftAction(), LeftSide()))
v_ = translate_diff(G, χ_, χ, v, (LeftAction(), LeftSide()))
χ_χ = translate(G, χ_, χ, (LeftAction(), LeftSide()))
χ2 = exp(G, χ_χ, v_)
return isapprox(G, χ1, χ2)
end
"""
check_exp_exp_(G, exp, exp!, χ_, χ, v)
Compare `exp` and `exp!`.
"""
check_exp_exp_(G, exp, exp!, χ_, χ, v) = begin
χ1 = exp!(G, χ_, χ, v)
χ2 = exp(G, χ, v)
return χ1 === χ_ && isapprox(G, χ1, χ2)
end
"""
check_log_log_(G, log, log!, v_, χ1, χ2)
Compare `log` and `log!`.
"""
check_log_log_(G, log, log!, v_, χ1, χ2) = begin
v__ = log!(G, v_, χ1, χ2)
v = log(G, χ1, χ2)
return v__ === v_ && isapprox(TangentSpace(G, χ1), v, v__)
end
"""
check_exp_log(G, exp, log, χ1, χ2)
Check the identity
```math
exp_{χ_1}(log_{χ_1}(χ_2)) = χ_2
```
"""
check_exp_log(G, exp, log, χ1, χ2) = begin
v = log(G, χ1, χ2)
χ_ = exp(G, χ1, v)
return isapprox(G, χ2, χ_)
end
"""
check_log_exp(G, log, exp, χ, v)
Check the identity
```math
log_{χ}(exp_{χ}(v)) = v
```
"""
check_log_exp(G, log, exp, χ, v) = begin
χ_ = exp(G, χ, v)
v_ = log(G, χ, χ_)
return isapprox(TangentSpace(G, χ), v, v_)
end
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 111 | module ManifoldGroupTesting
using Manifolds
include("Diff.jl")
include("Group.jl")
include("Action.jl")
end
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 210 | import ManifoldGroupTesting as GT
using Test
using Manifolds
import ManifoldGroupUtils: rand_lie, translate_from_id
import Random
rng = Random.default_rng()
include("test_group.jl")
include("test_action.jl")
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 909 |
@testset "Action $name" for (name, A) in [
"Rot plane" => RotationAction(Euclidean(3), SpecialOrthogonal(3)),
"SO L" => GroupOperationAction(SpecialOrthogonal(3), Manifolds.LeftForwardAction()),
"SO R*" => GroupOperationAction(SpecialOrthogonal(3), Manifolds.RightForwardAction()),
"SO L*" => GroupOperationAction(SpecialOrthogonal(3), Manifolds.LeftBackwardAction()),
]
G = base_group(A)
χ1, χ2 = [rand(rng, G) for i in 1:2]
ξ1, ξ2 = [rand_lie(rng, G) for i in 1:2]
p = rand(rng, group_manifold(A))
@test GT.check_action_morphism(A, χ1, χ2, p)
@test GT.check_apply_morphism_Identity(A, p)
@test GT.check_trivial_infinitesimal_action(A, p, identity_element)
@test GT.check_switch_action_direction(A, χ1, p)
@testset for id_func in [Identity, identity_element]
@test GT.check_apply_diff_group(A, χ1, ξ1, p) broken = A isa RotationAction
end
end
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | code | 1324 |
grp_rep(::Any, x) = x
grp_rep(G, ::Identity) = grp_rep(G, identity_element(G))
alg_rep(::Any, x) = x
@testset "Group Testing.jl" for G in [SpecialOrthogonal(3)]
χ1, χ2 = [rand(rng, G) for i in 1:2]
ξ1, ξ2 = [rand_lie(rng, G) for i in 1:2]
v1 = translate_from_id(G, χ1, ξ1, LeftSide())
@test GT.check_exp_lie_point(G, ξ1)
@test GT.check_adjoint_action_in_alg(G, χ1, ξ1)
@test GT.check_grp_rep_Identity(G, grp_rep)
@test GT.check_grp_rep_compose(G, grp_rep, χ1, χ2)
@test GT.check_alg_rep(G, alg_rep, ξ1, ξ2)
@test GT.check_zero_Identity(G) broken=true # fails for SpecialOrthogonal
@test GT.check_exp_ad(G, ξ1, ξ2)
@test GT.check_adjoint_action(G, grp_rep, alg_rep, χ1, ξ1)
@test GT.check_inv_rep(G, grp_rep, χ1)
@testset "$side" for side in [LeftSide(), RightSide()]
@test GT.check_apply_diff_group_at_id(G, ξ1, side, Identity)
@test GT.check_apply_diff_group_at_id(G, ξ1, side, identity_element)
@test GT.check_inv_diff(G, χ1, ξ1, side)
end
@test GT.check_exp_invariant(G, exp, χ1, v1, χ2)
@test GT.check_exp_log(G, exp, log, χ1, χ2)
@test GT.check_log_exp(G, log, exp, χ1, v1)
v = similar(v1)
@test GT.check_log_log_(G, log, log!, v, χ1, χ2)
χ = similar(χ1)
@test GT.check_exp_exp_(G, exp, exp!, χ, χ1, v1)
end
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | docs | 463 | # Changelog
All notable changes to this project will be documented in this file.
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.1.0/),
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html).
## [0.2.0] - 2024-08-26
### Added
- exponential invariance tests
- compatibility exponential/logarithm
- tests for the mutable versions
### Removed
- `translation_from_id` moved to `ManifoldGroupUtils.jl`
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | docs | 666 | # ManifoldGroupTesting
[](https://github.com/olivierverdier/ManifoldGroupTesting.jl/actions/workflows/CI.yml?query=branch%3Amain)
[](https://codecov.io/gh/olivierverdier/ManifoldGroupTesting.jl)
[](https://olivierverdier.github.io/ManifoldGroupTesting.jl/dev/)
Utilities to help testing new groups and actions created with [Manifolds.jl](https://github.com/JuliaManifolds/Manifolds.jl).
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.2.2 | 2eb42778b27590c7e5f002623748e1c2a2c328d3 | docs | 201 | # ManifoldGroupTesting.jl
`ManifoldGroupTesting.jl` contains several useful functions to test new group implementations.
```@autodocs
Modules = [ManifoldGroupTesting]
Order = [:type, :function]
```
| ManifoldGroupTesting | https://github.com/olivierverdier/ManifoldGroupTesting.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 514 | using SciMLBenchmarks
target = ARGS[1]
if isdir(target)
if !isfile(joinpath(target, "Project.toml"))
error("Cannot benchmark folder $(target) without Project.toml!")
end
println("Benchmarking the $(target) folder")
SciMLBenchmarks.weave_folder(target)
elseif isfile(target)
folder = dirname(target)
file = basename(target)
println("Benchmarking $(folder)/$(file)")
SciMLBenchmarks.weave_file(folder, file)
else
error("Unable to find benchmarking target $(target)!")
end
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 2208 | using Pkg
Pkg.add(["Git", "GitHub", "Dates"])
using Git, GitHub, Dates
gh_token = ARGS[1]
myauth = GitHub.authenticate(gh_token)
(@isdefined myauth) ? @info("Authentication token is found...") : @info("Coudn't find the authentication token")
const git = Git.git()
date = Dates.format(now(), "yyyy-mm-dd")
benchpath = joinpath(@__DIR__, "..", "..", "benchmarks")
# Get all the open PRs and their number
gh_prs = GitHub.pull_requests("SciML/SciMLBenchmarks.jl"; auth=myauth)
prs = Dict{String, Int64}()
for i in 1:length(gh_prs[1])
prs[gh_prs[1][i].head.ref] = gh_prs[1][i].number
end
# Get all the branches from the repo
gh_branches = GitHub.branches("SciML/SciMLBenchmarks.jl"; auth=myauth)
branches = [gh_branches[1][i].name for i in 1:length(gh_branches[1])]
@info("PRs and branches", prs, branches)
for dir in readdir(benchpath)
model_dir = joinpath(benchpath, dir)
isdir(model_dir) || continue
println("--- Inspecting $dir ---")
cd(model_dir)
Pkg.activate(".") do
Pkg.update()
end
manpath = (joinpath(benchpath, "Manifest.toml"))
if length(readlines(`git status . --porcelain`)) > 1
if dir ∉ branches
run(`git checkout -b $(dir) master`)
run(`$git add -A . :!$(manpath)`)
run(`$git commit -m "Updated $(dir) on $(date)"`)
run(`$git push --set-upstream origin $(dir)`)
else
run(`$git fetch origin`)
run(`$git checkout $(dir)`)
run(`$git pull -Xours`)
run(`$git add -A . :!$(manpath)`)
run(`$git commit -m "Updated $(dir) on $(date)"`)
run(`$git push`)
end
if dir ∉ keys(prs)
params = Dict(
"title" => "Updated $(dir) for benchmarks",
"head" => "$(dir)",
"base" => "master"
)
@info("Creating a pull request from head: ", dir)
GitHub.create_pull_request("SciML/SciMLBenchmarks.jl"; params=params, auth=myauth)
else
@info("Updating the pull request numbered: ", prs[dir])
GitHub.update_pull_request("SciML/SciMLBenchmarks.jl", prs[dir]; auth=myauth)
end
end
end
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 8742 | """
---
title: Burgers FDM Work-Precision Diagrams with Various MethodOfLines Methods
author: Alex Jones
---
This benchmark is for the MethodOfLines.jl package, which is an automatic PDE discretization package.
It is concerned with comparing the performance of various discretization methods for the Burgers equation.
"""
using MethodOfLines, DomainSets, OrdinaryDiffEq, ModelingToolkit, DiffEqDevTools, LinearAlgebra,
LinearSolve, Plots
using PDESystemLibrary
"""
Next we define some functions to generate approproiate discretizations for the PDESystemLibrary systems.
"""
function center_uniform_grid(ex, ivs, N)
map(ivs) do x
xdomain = ex.domain[findfirst(d -> isequal(x, d.variables), ex.domain)]
x => (supremum(xdomain.domain) - infimum(xdomain.domain)) /
(floor(N^(1 / length(ivs))) - 1)
end
end
function edge_uniform_grid(ex, ivs, N)
map(ivs) do x
xdomain = ex.domain[findfirst(d -> isequal(x, d.variables), ex.domain)]
x => (supremum(xdomain.domain) - infimum(xdomain.domain)) /
(floor(N^(1 / length(ivs))))
end
end
function center_chebygrid(ex, ivs, N)
map(ivs) do x
xdomain = ex.domain[findfirst(d -> isequal(x, d.variables), ex.domain)]
x => chebyspace(xdomain, trunc(Int, N^(1 / length(ivs))))
end
end
function edge_chebygrid(ex, ivs, N)
map(ivs) do x
xdomain = ex.domain[findfirst(d -> isequal(x, d.variables), ex.domain)]
x => chebyspace(xdomain, trunc(Int, N^(1 / length(ivs))) - 1)
end
end
function uniformupwind1(ex, ivs, t, N)
dxs = center_uniform_grid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=UpwindScheme())
end
function uniformupwind2(ex, ivs, t, N)
dxs = edge_uniform_grid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=UpwindScheme(), grid_align=edge_align)
end
function chebyupwind1(ex, ivs, t, N)
dxs = center_chebygrid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=UpwindScheme())
end
function chebyupwind2(ex, ivs, t, N)
dxs = edge_chebygrid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=UpwindScheme(), grid_align=edge_align)
end
function discweno1(ex, ivs, t, N)
dxs = center_uniform_grid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=WENOScheme())
end
function discweno2(ex, ivs, t, N)
dxs = edge_uniform_grid(ex, ivs, N)
MOLFiniteDifference(dxs, t, advection_scheme=WENOScheme(), grid_align=edge_align)
end
"""
This script tests all systems in PDESystemLibrary against different MethodOfLines.jl discretizations.
"""
N = 100
for ex in PSL.all_systems
try
if ex.analytic_func === nothing
continue
end
ivs = filter(x -> !isequal(Symbol(x), :t), ex.ivs)
if length(ivs) == 0
continue
elseif length(ivs) == length(ex.ivs)
# Skip nonlinear systems until I know the syntax for that
continue
# advection = false
# discuu1 = uniformupwind1(ex, ivs, nothing, N)
# discuu2 = uniformupwind2(ex, ivs, nothing, N)
# discnu1 = chebyupwind1(ex, ivs, nothing, N)
# discnu2 = chebyupwind2(ex, ivs, nothing, N)
# discs = [discuu1, discuu2, discnu1, discnu2]
# if "Advection" in ex.metadata
# advection = true
# discw1 = discweno1(ex, ivs, nothing, N)
# discw2 = discweno2(ex, ivs, nothing, N)
# push!(discs, discw1, discw2)
# end
# probs = map(discs) do disc
# discretize(ex, disc, analytic = ex.analytic_func)
# end
# title = "Work Precision Diagram for $(ex.name), Tags: $(ex.metadata)"
# println("Running $title")
# if advection
# dummy_appxsol = [nothing for i in 1:length(probs1)]
# abstols = 1.0 ./ 10.0 .^ (5:8)
# reltols = 1.0 ./ 10.0 .^ (1:4);
# setups = [Dict(:alg => solver, :prob_choice => 1),
# Dict(:alg => solver, :prob_choice => 2),
# Dict(:alg => solver, :prob_choice => 3),
# Dict(:alg => solver, :prob_choice => 4),
# Dict(:alg => solver, :prob_choice => 5),
# Dict(:alg => solver, :prob_choice => 6),]
# names = ["Uniform Upwind, center_align", "Uniform Upwind, edge_align",
# "Chebyshev Upwind, center_align", "Chebyshev Upwind, edge_align",
# "Uniform WENO, center_align", "Uniform WENO, edge_align"];
# wp = WorkPrecisionSet(probs, abstols, reltols, setups; names=names,
# save_everystep=false, appxsol = dummy_appxsol, maxiters=Int(1e5),
# numruns=10, wrap=Val(false))
# plot(wp, title=title)
# else
# dummy_appxsol = [nothing for i in 1:length(probs)]
# abstols = 1.0 ./ 10.0 .^ (5:8)
# reltols = 1.0 ./ 10.0 .^ (1:4);
# setups = [Dict(:alg => solver, :prob_choice => 1),
# Dict(:alg => solver, :prob_choice => 2),
# Dict(:alg => solver, :prob_choice => 3),
# Dict(:alg => solver, :prob_choice => 4),]
# names = ["Uniform Upwind, center_align", "Uniform Upwind, edge_align",
# "Chebyshev Upwind, center_align", "Chebyshev Upwind, edge_align"];
# wp = WorkPrecisionSet(probs1, abstols, reltols, setups; names=names,
# save_everystep=false, appxsol = dummy_appxsol, maxiters=Int(1e5),
# numruns=10, wrap=Val(false))
# plot(wp, title=title)
# end
else
@parameters t
# Create discretizations
advection = false
discuu1 = uniformupwind1(ex, ivs, t, N)
discuu2 = uniformupwind2(ex, ivs, t, N)
discnu1 = chebyupwind1(ex, ivs, t, N)
discnu2 = chebyupwind2(ex, ivs, t, N)
discs = [discuu1, discuu2, discnu1, discnu2]
if "Advection" in ex.metadata
advection = true
discw1 = discweno1(ex, ivs, t, N)
discw2 = discweno2(ex, ivs, t, N)
push!(discs, discw1, discw2)
end
# Create problems
probs = map(discs) do disc
discretize(ex, disc, analytic = ex.analytic_func)
end
title = "Work Precision Diagram for $(ex.name), Tags: $(ex.metadata)"
println("Running $title")
if advection
dummy_appxsol = [nothing for i in 1:length(probs1)]
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:alg => solver, :prob_choice => 1),
Dict(:alg => solver, :prob_choice => 2),
Dict(:alg => solver, :prob_choice => 3),
Dict(:alg => solver, :prob_choice => 4),
Dict(:alg => solver, :prob_choice => 5),
Dict(:alg => solver, :prob_choice => 6),]
names = ["Uniform Upwind, center_align", "Uniform Upwind, edge_align",
"Chebyshev Upwind, center_align", "Chebyshev Upwind, edge_align",
"Uniform WENO, center_align", "Uniform WENO, edge_align"];
wp = WorkPrecisionSet(probs, abstols, reltols, setups; names=names,
save_everystep=false, appxsol = dummy_appxsol, maxiters=Int(1e5),
numruns=10, wrap=Val(false))
plot(wp, title=title)
else
dummy_appxsol = [nothing for i in 1:length(probs)]
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:alg => solver, :prob_choice => 1),
Dict(:alg => solver, :prob_choice => 2),
Dict(:alg => solver, :prob_choice => 3),
Dict(:alg => solver, :prob_choice => 4),]
names = ["Uniform, center_align", "Uniform, edge_align",
"Chebyshev, center_align", "Chebyshev, edge_align"];
wp = WorkPrecisionSet(probs1, abstols, reltols, setups; names=names,
save_everystep=false, appxsol = dummy_appxsol, maxiters=Int(1e5),
numruns=10, wrap=Val(false))
plot(wp, title=title)
end
end
catch e
println("Failed on $(ex.name):")
println(e)
end
end
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 544 | using Documenter, SciMLBenchmarksOutput
dir = @__DIR__() * "/.."
@show dir
@show readdir(dir)
include("pages.jl")
makedocs(
sitename="The SciML Benchmarks",
authors="Chris Rackauckas",
modules=[SciMLBenchmarksOutput],
clean=true, doctest=false,
format=Documenter.HTML(#analytics = "UA-90474609-3",
assets=["assets/favicon.ico"],
canonical="https://benchmarks.sciml.ai/stable/"),
pages=pages
)
deploydocs(;
repo="github.com/SciML/SciMLBenchmarksOutput",
devbranch="main",
branch="main"
)
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 2937 | # This file assumes `dir` is the directory for the package! dir = @__DIR__() * "/.."
dir = @__DIR__() * "/.."
cp(joinpath(dir, "markdown"), joinpath(dir, "docs", "src"), force=true)
cp(joinpath(dir, "docs", "extrasrc", "assets"), joinpath(dir, "docs", "src", "assets"), force=true)
cp(joinpath(dir, "README.md"), joinpath(dir, "docs", "src", "index.md"), force=true)
benchmarksdir = joinpath(dir, "docs", "src")
@show readdir(benchmarksdir)
pages = Any["SciMLBenchmarks.jl: Benchmarks for Scientific Machine Learning (SciML), Equation Solvers, and AI for Science"=>"index.md"]
for folder in readdir(benchmarksdir)
newpages = Any[]
if folder[end-2:end] != ".md" && folder != "Testing" && folder != "figures" && folder != "assets"
for file in filter(x -> x[end-2:end] == ".md", readdir(
joinpath(benchmarksdir, folder)))
try
filecontents = readlines(joinpath(benchmarksdir, folder, file))
title = filecontents[3][9:end-1]
# Cut out the first 5 lines from the file to remove the Weave header stuff
open(joinpath(benchmarksdir, folder, file), "w") do output
println(output, "# $title")
for line in Iterators.drop(filecontents, 4)
println(output, line)
end
end
push!(newpages, title => joinpath(folder, file))
catch e
@show folder, file, e
end
end
push!(pages, folder => newpages)
end
end
# The result is in alphabetical order, change to the wanted order
permute!(pages,
[1, 10, 13, 19, 4, 5, 9, 6, 11, 14, 20, 12, 18, 7, 17, 3, 8, 15, 16, 2]
)
names = [
"SciMLBenchmarks.jl: Benchmarks for Scientific Machine Learning (SciML) and Equation Solvers",
"Multi-Language Wrapper Benchmarks",
"Non-Stiff Ordinary Differential Equations",
"Stiff Ordinary Differential Equations",
"Biological Differential Equations",
"Differential-Algebraic Equations (DAEs)",
"Method of Lines Partial Differential Equations (PDEs)",
"Dynamical ODEs (Hamiltonian and Second Order)",
"N-Body Problem Benchmarks",
"Non-Stiff Stochastic Differential Equations",
"Stiff Stochastic Differential Equations",
"Non-Stiff Delay Differential Equations",
"Stiff Delay Differential equations",
"Jump Process Equations (Gillespie Benchmarks)",
"Parameter Estimation and Inverse Problem Benchmarks",
"Bayesian Inference and Probabilistic Inverse Problem Benchmarks",
"MethodOfLines.jl Partial Differential Equation (PDE) Formulations",
"Physics-Informed Neural Network (Neural Network PDE Solver) Cost Function Benchmarks",
"Physics-Informed Neural Network (Neural Network PDE Solver) Optimizer Benchmarks",
"SDE Adaptivity Benchmarks"]
for i in 1:length(pages)
pages[i] = names[i] => pages[i][2]
end
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 3604 | module SciMLBenchmarks
using Weave, Pkg, IJulia, InteractiveUtils, Markdown
repo_directory = joinpath(@__DIR__,"..")
function weave_file(folder,file,build_list=(:script,:github))
target = joinpath(folder, file)
@info("Weaving $(target)")
if isfile(joinpath(folder, "Project.toml")) && build_list != (:notebook,)
@info("Instantiating", folder)
Pkg.activate(folder)
Pkg.instantiate()
Pkg.build()
end
args = Dict{Symbol,String}(:folder=>folder,:file=>file)
if :script ∈ build_list
println("Building Script")
dir = joinpath(repo_directory,"script",basename(folder))
mkpath(dir)
tangle(target; out_path=dir)
end
if :html ∈ build_list
println("Building HTML")
dir = joinpath(repo_directory,"html",basename(folder))
mkpath(dir)
weave(target,doctype = "md2html",out_path=dir,args=args,fig_ext=".svg")
end
if :pdf ∈ build_list
println("Building PDF")
dir = joinpath(repo_directory,"pdf",basename(folder))
mkpath(dir)
try
weave(target,doctype="md2pdf",out_path=dir,args=args)
catch ex
@warn "PDF generation failed" exception=(ex, catch_backtrace())
end
end
if :github ∈ build_list
println("Building Github Markdown")
dir = joinpath(repo_directory,"markdown",basename(folder))
mkpath(dir)
weave(target,doctype = "github",out_path=dir,args=args)
end
if :notebook ∈ build_list
println("Building Notebook")
dir = joinpath(repo_directory,"notebook",basename(folder))
mkpath(dir)
Weave.convert_doc(target,joinpath(dir,file[1:end-4]*".ipynb"))
end
end
function weave_all(build_list=(:script,:github))
for folder in readdir(joinpath(repo_directory,"benchmarks"))
folder == "test.jmd" && continue
weave_folder(joinpath(repo_directory,"benchmarks",folder),build_list)
end
end
function weave_folder(folder, build_list=(:script,:github))
for file in readdir(folder)
# Skip non-`.jmd` files
if !endswith(file, ".jmd")
continue
end
try
weave_file(folder, file, build_list)
catch e
@show folder, file
@error(e)
end
end
end
function bench_footer(folder=nothing, file=nothing)
display(md"""
## Appendix
These benchmarks are a part of the SciMLBenchmarks.jl repository, found at: <https://github.com/SciML/SciMLBenchmarks.jl>.
For more information on high-performance scientific machine learning, check out the SciML Open Source Software Organization <https://sciml.ai>.
""")
if folder !== nothing && file !== nothing
display(Markdown.parse("""
To locally run this benchmark, do the following commands:
```
using SciMLBenchmarks
SciMLBenchmarks.weave_file("$folder","$file")
```
"""))
end
display(md"Computer Information:")
vinfo = sprint(InteractiveUtils.versioninfo)
display(Markdown.parse("""
```
$(vinfo)
```
"""))
display(md"""
Package Information:
""")
proj = sprint(io -> Pkg.status(io=io))
mani = sprint(io -> Pkg.status(io=io, mode = Pkg.PKGMODE_MANIFEST))
md = """
```
$(chomp(proj))
```
And the full manifest:
```
$(chomp(mani))
```
"""
display(Markdown.parse(md))
end
function open_notebooks()
Base.eval(Main, Meta.parse("import IJulia"))
weave_all((:notebook,))
path = joinpath(repo_directory,"notebook")
IJulia.notebook(;dir=path)
newpath = joinpath(pwd(),"generated_notebooks")
mv(path, newpath)
IJulia.notebook(;dir=newpath)
end
end # module SciMLBenchmarks
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | code | 443 | using SciMLBenchmarks, Test
@testset "weave_file" begin
benchmarks_dir = joinpath(dirname(@__DIR__), "benchmarks")
SciMLBenchmarks.weave_file(joinpath(benchmarks_dir, "Testing"), "test.jmd")
#@test isfile(joinpath(dirname(@__DIR__), "script", "Testing", "test.jl"))
#@test isfile(joinpath(dirname(@__DIR__), "html", "Testing", "test.html"))
@test isfile(joinpath(dirname(@__DIR__), "markdown", "Testing", "test.md"))
end
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 11742 | # SciMLBenchmarks.jl: Benchmarks for Scientific Machine Learning (SciML) and Equation Solvers
[](https://julialang.zulipchat.com/#narrow/stream/279055-sciml-bridged)
[](https://docs.sciml.ai/SciMLBenchmarksOutput/stable/)
[](https://buildkite.com/julialang/scimlbenchmarks-dot-jl)
[](https://github.com/SciML/ColPrac)
[](https://github.com/SciML/SciMLStyle)
SciMLBenchmarks.jl holds webpages, pdfs, and notebooks showing the benchmarks
for the SciML Scientific Machine Learning Software ecosystem, including:
- Benchmarks of equation solver implementations
- Speed and robustness comparisons of methods for parameter estimation / inverse problems
- Training universal differential equations (and subsets like neural ODEs)
- Training of physics-informed neural networks (PINNs)
- Surrogate comparisons, including radial basis functions, neural operators (DeepONets, Fourier Neural Operators), and more
The SciML Bench suite is made to be a comprehensive open source benchmark from the ground up, covering the methods of
computational science and scientific computing all the way to AI for science.
## Rules: Optimal, Fair, and Reproducible
These benchmarks are meant to represent good optimized coding style. Benchmarks are preferred to be run on the provided open
benchmarking hardware for full reproducibility (though in some cases, such as with language barriers, this can be difficult).
Each benchmark is documented with the compute devices used along with package versions for necessary reproduction. These
benchmarks attempt to measure in terms of work-precision efficiency, either timing with an approximately matching the error
or building work-precision diagrams for direct comparison of speed at given error tolerances.
**If any of the code from any of the languages can be improved, please open a pull request**.
## Results
To view the results of the SciML Benchmarks, go to [benchmarks.sciml.ai](https://benchmarks.sciml.ai/stable/). By default, this
will lead to the latest tagged version of the benchmarks. To see the in-development version of the benchmarks, go to
[https://benchmarks.sciml.ai/dev/](https://benchmarks.sciml.ai/dev/).
Static outputs in pdf, markdown, and html reside in [SciMLBenchmarksOutput](https://github.com/SciML/SciMLBenchmarksOutput).
## Citing
To cite the SciML Benchmarks, please cite the following:
```bib
@article{rackauckas2019confederated,
title={Confederated modular differential equation APIs for accelerated algorithm development and benchmarking},
author={Rackauckas, Christopher and Nie, Qing},
journal={Advances in Engineering Software},
volume={132},
pages={1--6},
year={2019},
publisher={Elsevier}
}
@article{DifferentialEquations.jl-2017,
author = {Rackauckas, Christopher and Nie, Qing},
doi = {10.5334/jors.151},
journal = {The Journal of Open Research Software},
keywords = {Applied Mathematics},
note = {Exported from https://app.dimensions.ai on 2019/05/05},
number = {1},
pages = {},
title = {DifferentialEquations.jl – A Performant and Feature-Rich Ecosystem for Solving Differential Equations in Julia},
url = {https://app.dimensions.ai/details/publication/pub.1085583166 and http://openresearchsoftware.metajnl.com/articles/10.5334/jors.151/galley/245/download/},
volume = {5},
year = {2017}
}
```
## Current Summary
The following is a quick summary of the benchmarks. These paint broad strokes
over the set of tested equations and some specific examples may differ.
### Non-Stiff ODEs
- OrdinaryDiffEq.jl's methods are the most efficient by a good amount
- The `Vern` methods tend to do the best in every benchmark of this category
- At lower tolerances, `Tsit5` does well consistently.
- ARKODE and Hairer's `dopri5`/`dop853` perform very similarly, but are both
far less efficient than the `Vern` methods.
- The multistep methods, `CVODE_Adams` and `lsoda`, tend to not do very well.
- The ODEInterface multistep method `ddeabm` does not do as well as the other
multistep methods.
- ODE.jl's methods are not able to consistently solve the problems.
- Fixed time step methods are less efficient than the adaptive methods.
### Stiff ODEs
- In this category, the best methods are much more problem dependent.
- For smaller problems:
- `Rosenbrock23`, `lsoda`, and `TRBDF2` tend to be the most efficient at high
tolerances.
- `Rodas4` and `Rodas5` tend to be the most efficient at low tolerances.
- For larger problems (Filament PDE):
- `QNDF` and `FBDF` does the best at all normal tolerances.
- The ESDIRK methods like `TRBDF2` and `KenCarp4` can come close.
- `radau` is always the most efficient when tolerances go to the low extreme
(`1e-13`)
- Fixed time step methods tend to diverge on every tested problem because the
high stiffness results in divergence of the Newton solvers.
- ARKODE is very inconsistent and requires a lot of tweaking in order to not
diverge on many of the tested problems. When it doesn't diverge, the similar
algorithms in OrdinaryDiffEq.jl (`KenCarp4`) are much more efficient in most
cases.
- ODE.jl and GeometricIntegrators.jl fail to converge on any of the tested
problems.
### Dynamical ODEs
- Higher order (generally order >=6) symplectic integrators are much more
efficient than the lower order counterparts.
- For high accuracy, using a symplectic integrator is not preferred. Their extra
cost is not necessary since the other integrators are able to not drift simply
due to having low enough error.
- In this class, the `DPRKN` methods are by far the most efficient. The `Vern`
methods do well for not being specific to the domain.
### Non-Stiff SDEs
- For simple 1-dimensional SDEs at low accuracy, the `EM` and `RKMil` methods
can do well. Beyond that, they are simply outclassed.
- The `SRA` and `SRI` methods both are very similar within-class on the simple
SDEs.
- `SRA3` is the most efficient when applicable and the tolerances are low.
- Generally, only low accuracy is necessary to get to sampling error of the mean.
- The adaptive method is very conservative with error estimates.
### Stiff SDEs
- The high order adaptive methods (`SRIW1`) generally do well on stiff problems.
- The "standard" low-order implicit methods, `ImplicitEM` and `ImplicitRK`, do
not do well on all stiff problems. Some exceptions apply to well-behaved
problems like the Stochastic Heat Equation.
### Non-Stiff DDEs
- The efficiency ranking tends to match the ODE Tests, but the cutoff from
low to high tolerance is lower.
- `Tsit5` does well in a large class of problems here.
- The `Vern` methods do well in low tolerance cases.
### Stiff DDEs
- The Rosenbrock methods, specifically `Rodas5`, perform well.
### Parameter Estimation
- Broadly two different approaches have been used, Bayesian Inference and Optimisation
algorithms.
- In general it seems that the optimisation algorithms perform more accurately but that can be
attributed to the larger number of data points being used in the optimisation cases, Bayesian
approach tends to be slower of the two and hence lesser data points are used, accuracy can
increase if proper data is used.
- Within the different available optimisation algorithms, BBO from the BlackBoxOptim package and GN_CRS2_LM
for the global case while LD_SLSQP,LN_BOBYQA and LN_NELDERMEAD for the local case from the NLopt package
perform the best.
- Another algorithm being used is the [QuadDIRECT](https://github.com/timholy/QuadDIRECT.jl) algorithm, it gives very good results in the shorter problem case
but doesn't do very well in the case of the longer problems.
- The choice of global versus local optimization make a huge difference in the timings. BBO tends to find
the correct solution for a global optimization setup. For local optimization, most methods in NLopt,
like :LN_BOBYQA, solve the problem very fast but require a good initial condition.
- The different backends options available for Bayesian method offer some tradeoffs beteween
time, accuracy and control. It is observed that sufficiently high accuracy can be observed with
any of the backends with the fine tuning of stepsize, constraints on the parameters, tightness of the
priors and number of iterations being passed.
## Interactive Notebooks
To generate the interactive notebooks, first install the SciMLBenchmarks, instantiate the
environment, and then run `SciMLBenchmarks.open_notebooks()`. This looks as follows:
```julia
]add SciMLBenchmarks#master
]activate SciMLBenchmarks
]instantiate
using SciMLBenchmarks
SciMLBenchmarks.open_notebooks()
```
The benchmarks will be generated at your `pwd()` in a folder called `generated_notebooks`.
Note that when running the benchmarks, the packages are not automatically added. Thus you
will need to add the packages manually or use the internal Project/Manifest tomls to
instantiate the correct packages. This can be done by activating the folder of the benchmarks.
For example,
```julia
using Pkg
Pkg.activate(joinpath(pkgdir(SciMLBenchmarks),"benchmarks","NonStiffODE"))
Pkg.instantiate()
```
will add all of the packages required to run any benchmark in the `NonStiffODE` folder.
## Contributing
All of the files are generated from the Weave.jl files in the `benchmarks` folder. The generation process runs automatically,
and thus one does not necessarily need to test the Weave process locally. Instead, simply open a PR that adds/updates a
file in the "benchmarks" folder and the PR will generate the benchmark on demand. Its artifacts can then be inspected in the
Buildkite as described below before merging. Note that it will use the Project.toml and Manifest.toml of the subfolder, so
any changes to dependencies requires that those are updated.
### Reporting Bugs and Issues
Report any bugs or issues at [the SciMLBenchmarks repository](https://github.com/SciML/SciMLBenchmarks.jl).
### Inspecting Benchmark Results
To see benchmark results before merging, click into the BuildKite, click onto
Artifacts, and then investigate the trained results.
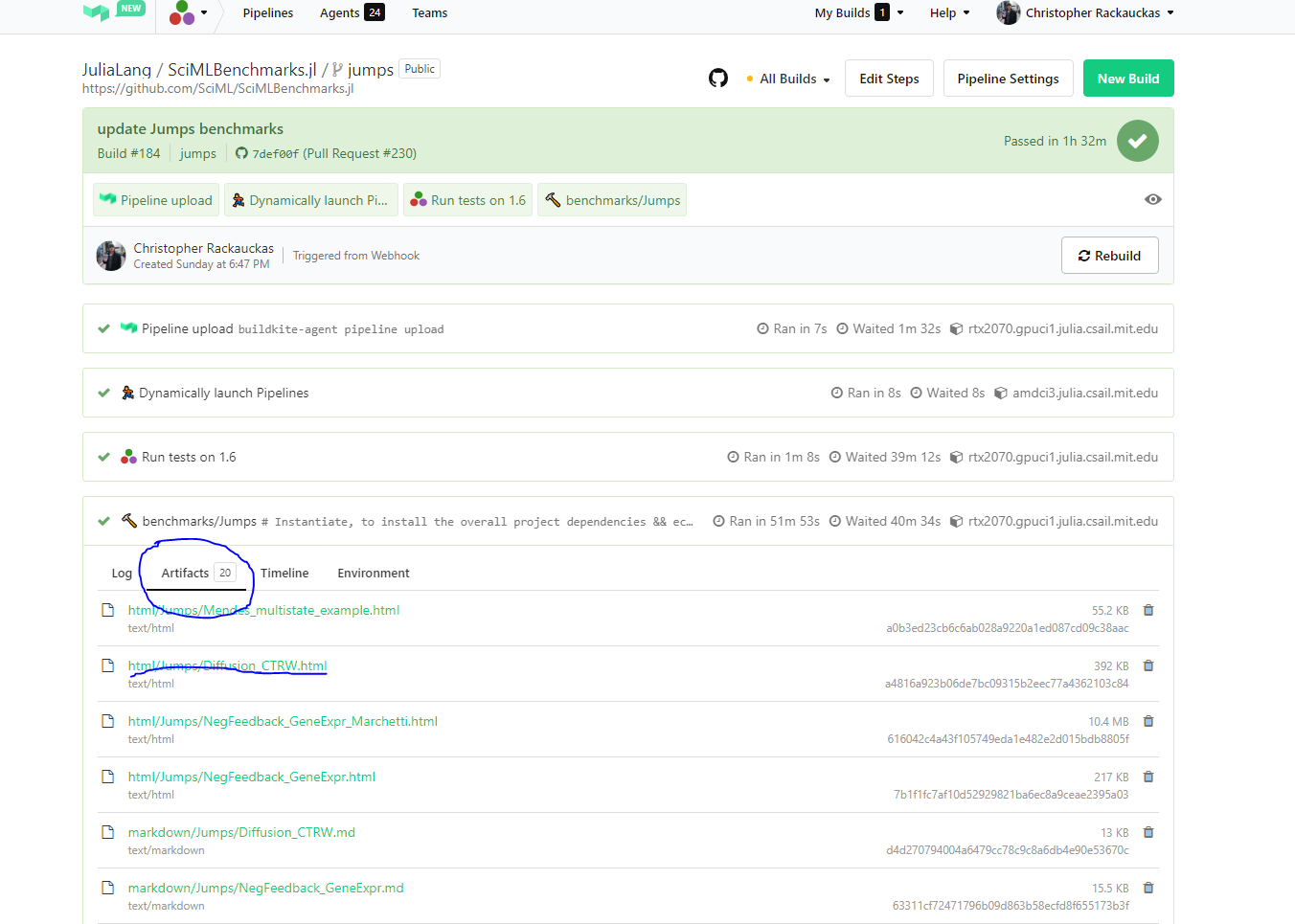
### Manually Generating Files
All of the files are generated from the Weave.jl files in the `benchmarks` folder. To run the generation process, do for example:
```julia
]activate SciMLBenchmarks # Get all of the packages
using SciMLBenchmarks
SciMLBenchmarks.weave_file(joinpath(pkgdir(SciMLBenchmarks),"benchmarks","NonStiffODE"),"linear_wpd.jmd")
```
To generate all of the files in a folder, for example, run:
```julia
SciMLBenchmarks.weave_folder(joinpath(pkgdir(SciMLBenchmarks),"benchmarks","NonStiffODE"))
```
To generate all of the notebooks, do:
```julia
SciMLBenchmarks.weave_all()
```
Each of the benchmarks displays the computer characteristics at the bottom of
the benchmark. Since performance-necessary computations are normally performed on
compute clusters, the official benchmarks use a workstation with an
AMD EPYC 7502 32-Core Processor @ 2.50GHz to match the performance characteristics of
a standard node in a high performance computing (HPC) cluster or cloud computing
setup.
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 6311 | ---
title: Adaptive Efficiency Tests
author: Chris Rackauckas
---
```julia
using Distributed
addprocs(2)
p1 = Vector{Any}(undef,3)
p2 = Vector{Any}(undef,3)
p3 = Vector{Any}(undef,3)
@everywhere begin
using StochasticDiffEq, SDEProblemLibrary, DiffEqNoiseProcess, Plots, ParallelDataTransfer
import SDEProblemLibrary: prob_sde_additive,
prob_sde_linear, prob_sde_wave
end
using StochasticDiffEq, SDEProblemLibrary, DiffEqNoiseProcess, Plots, ParallelDataTransfer
import SDEProblemLibrary: prob_sde_additive,
prob_sde_linear, prob_sde_wave
probs = Matrix{SDEProblem}(undef,3,3)
## Problem 1
prob = prob_sde_linear
probs[1,1] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM1)))
probs[1,2] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM2)))
probs[1,3] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM3)))
## Problem 2
prob = prob_sde_wave
probs[2,1] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM1)))
probs[2,2] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM2)))
probs[2,3] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM3)))
## Problem 3
prob = prob_sde_additive
probs[3,1] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM1)))
probs[3,2] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM2)))
probs[3,3] = SDEProblem(prob.f,prob.g,prob.u0,prob.tspan,prob.p,noise=WienerProcess(0.0,0.0,0.0,rswm=RSWM(adaptivealg=:RSwM3)))
fullMeans = Vector{Array}(undef,3)
fullMedians = Vector{Array}(undef,3)
fullElapsed = Vector{Array}(undef,3)
fullTols = Vector{Array}(undef,3)
offset = 0
Ns = [17,23,
17]
```
Timings are only valid if no workers die. Workers die if you run out of memory.
```julia
for k in 1:size(probs,1)
global probs, Ns, fullMeans, fullMedians, fullElapsed, fullTols
println("Problem $k")
## Setup
N = Ns[k]
msims = Vector{Any}(undef,N)
elapsed = Array{Float64}(undef,N,3)
medians = Array{Float64}(undef,N,3)
means = Array{Float64}(undef,N,3)
tols = Array{Float64}(undef,N,3)
#Compile
prob = probs[k,1]
ParallelDataTransfer.sendto(workers(), prob=prob)
monte_prob = EnsembleProblem(prob)
solve(monte_prob,SRIW1(),dt=1/2^(4),adaptive=true,trajectories=1000,abstol=2.0^(-1),reltol=0)
println("RSwM1")
for i=1+offset:N+offset
tols[i-offset,1] = 2.0^(-i-1)
msims[i-offset] = DiffEqBase.calculate_monte_errors(solve(monte_prob,SRIW1(),
trajectories=1000,abstol=2.0^(-i-1),
reltol=0,force_dtmin=true))
elapsed[i-offset,1] = msims[i-offset].elapsedTime
medians[i-offset,1] = msims[i-offset].error_medians[:final]
means[i-offset,1] = msims[i-offset].error_means[:final]
end
println("RSwM2")
prob = probs[k,2]
ParallelDataTransfer.sendto(workers(), prob=prob)
monte_prob = EnsembleProblem(prob)
solve(monte_prob,SRIW1(),dt=1/2^(4),adaptive=true,trajectories=1000,abstol=2.0^(-1),reltol=0)
for i=1+offset:N+offset
tols[i-offset,2] = 2.0^(-i-1)
msims[i-offset] = DiffEqBase.calculate_monte_errors(solve(monte_prob,SRIW1(),
trajectories=1000,abstol=2.0^(-i-1),
reltol=0,force_dtmin=true))
elapsed[i-offset,2] = msims[i-offset].elapsedTime
medians[i-offset,2] = msims[i-offset].error_medians[:final]
means[i-offset,2] = msims[i-offset].error_means[:final]
end
println("RSwM3")
prob = probs[k,3]
ParallelDataTransfer.sendto(workers(), prob=prob)
monte_prob = EnsembleProblem(prob)
solve(monte_prob,SRIW1(),dt=1/2^(4),adaptive=true,trajectories=1000,abstol=2.0^(-1),reltol=0)
for i=1+offset:N+offset
tols[i-offset,3] = 2.0^(-i-1)
msims[i-offset] = DiffEqBase.calculate_monte_errors(solve(monte_prob,SRIW1(),
adaptive=true,trajectories=1000,abstol=2.0^(-i-1),
reltol=0,force_dtmin=true))
elapsed[i-offset,3] = msims[i-offset].elapsedTime
medians[i-offset,3] = msims[i-offset].error_medians[:final]
means[i-offset,3] = msims[i-offset].error_means[:final]
end
fullMeans[k] = means
fullMedians[k] =medians
fullElapsed[k] = elapsed
fullTols[k] = tols
end
```
```julia
gr(fmt=:svg)
lw=3
leg=String["RSwM1","RSwM2","RSwM3"]
titleFontSize = 16
guideFontSize = 14
legendFontSize= 14
tickFontSize = 12
for k in 1:size(probs,1)
global probs, Ns, fullMeans, fullMedians, fullElapsed, fullTols
p1[k] = Plots.plot(fullTols[k],fullMeans[k],xscale=:log10,yscale=:log10, xguide="Absolute Tolerance",yguide="Mean Final Error",title="Example $k" ,linewidth=lw,grid=false,lab=leg,titlefont=font(titleFontSize),legendfont=font(legendFontSize),tickfont=font(tickFontSize),guidefont=font(guideFontSize))
p2[k] = Plots.plot(fullTols[k],fullMedians[k],xscale=:log10,yscale=:log10,xguide="Absolute Tolerance",yguide="Median Final Error",title="Example $k",linewidth=lw,grid=false,lab=leg,titlefont=font(titleFontSize),legendfont=font(legendFontSize),tickfont=font(tickFontSize),guidefont=font(guideFontSize))
p3[k] = Plots.plot(fullTols[k],fullElapsed[k],xscale=:log10,yscale=:log10,xguide="Absolute Tolerance",yguide="Elapsed Time",title="Example $k" ,linewidth=lw,grid=false,lab=leg,titlefont=font(titleFontSize),legendfont=font(legendFontSize),tickfont=font(tickFontSize),guidefont=font(guideFontSize))
end
Plots.plot!(p1[1])
Plots.plot(p1[1],p1[2],p1[3],layout=(3,1),size=(1000,800))
```
```julia
#savefig("meanvstol.png")
#savefig("meanvstol.pdf")
```
```julia
plot(p3[1],p3[2],p3[3],layout=(3,1),size=(1000,800))
#savefig("timevstol.png")
#savefig("timevstol.pdf")
```
```julia
plot(p1[1],p3[1],p1[2],p3[2],p1[3],p3[3],layout=(3,2),size=(1000,800))
```
```julia
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 2397 | ---
title: qmax Determination
author: Chris Rackauckas
---
```julia
qs = 1.0 .+ 2.0.^(-5:2)
times = Array{Float64}(undef,length(qs),4)
means = Array{Float64}(undef,length(qs),4)
using StochasticDiffEq, SDEProblemLibrary, Random,
Plots, ParallelDataTransfer, DiffEqMonteCarlo, Distributed
Random.seed!(99)
full_prob = SDEProblemLibrary.oval2ModelExample(largeFluctuations=true,useBigs=false)
import SDEProblemLibrary: prob_sde_additivesystem,
prob_sde_additive, prob_sde_2Dlinear, prob_sde_linear, prob_sde_wave
prob = remake(full_prob,tspan=(0.0,1.0))
println("Solve once to compile.")
sol = solve(prob,EM(),dt=1/2^(18))
Int(sol.u[end][1]!=NaN)
println("Compilation complete.")
num_runs = 10000
probs = Vector{SDEProblem}(undef,3)
p1 = Vector{Any}(undef,3)
p2 = Vector{Any}(undef,3)
p3 = Vector{Any}(undef,3)
## Problem 1
probs[1] = prob_sde_linear
## Problem 2
probs[2] = prob_sde_wave
## Problem 3
probs[3] = prob_sde_additive
println("Setup Complete")
## Timing Runs
function runAdaptive(i,k)
sol = solve(prob,SRIW1(),dt=1/2^(8),abstol=2.0^(-15),reltol=2.0^(-10),
verbose=false,maxIters=Int(1e12),qmax=qs[k])
Int(any(isnan,sol[end]) || sol.t[end] != 1)
end
#Compile
monte_prob = EnsembleProblem(probs[1])
test_mc = solve(monte_prob,SRIW1(),dt=1/2^(4),adaptive=true,trajectories=1000,abstol=2.0^(-1),reltol=0)
DiffEqBase.calculate_monte_errors(test_mc);
```
## qmax test on Oval2 Model
```julia
for k in eachindex(qs)
global times
Random.seed!(99)
adaptiveTime = @elapsed numFails = sum(map((i)->runAdaptive(i,k),1:num_runs))
println("k was $k. The number of Adaptive Fails is $numFails. Elapsed time was $adaptiveTime")
times[k,4] = adaptiveTime
end
```
## qmax test on other problems
```julia
for k in eachindex(probs)
global probs, times, means, qs
println("Problem $k")
## Setup
prob = probs[k]
for i in eachindex(qs)
msim = solve(monte_prob,dt=1/2^(4),SRIW1(),adaptive=true,trajectories=num_runs,abstol=2.0^(-13),reltol=0,qmax=qs[i])
test_msim = DiffEqBase.calculate_monte_errors(msim)
times[i,k] = test_msim.elapsedTime
means[i,k] = test_msim.error_means[:final]
println("for k=$k and i=$i, we get that the error was $(means[i,k]) and it took $(times[i,k]) seconds")
end
end
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 3211 | ---
title: Fitzhugh-Nagumo Bayesian Parameter Estimation Benchmarks
author: Vaibhav Dixit, Chris Rackauckas
---
```julia
using DiffEqBayes, BenchmarkTools
```
```julia
using OrdinaryDiffEq, RecursiveArrayTools, Distributions, ParameterizedFunctions, StanSample, DynamicHMC
using Plots, StaticArrays, Turing, LinearAlgebra
```
```julia
gr(fmt=:png)
```
### Defining the problem.
The [FitzHugh-Nagumo model](https://en.wikipedia.org/wiki/FitzHugh%E2%80%93Nagumo_model) is a simplified version of [Hodgkin-Huxley model](https://en.wikipedia.org/wiki/Hodgkin%E2%80%93Huxley_model) and is used to describe an excitable system (e.g. neuron).
```julia
fitz = @ode_def FitzhughNagumo begin
dv = v - 0.33*v^3 -w + l
dw = τinv*(v + a - b*w)
end a b τinv l
```
```julia
prob_ode_fitzhughnagumo = ODEProblem(fitz, [1.0,1.0], (0.0,10.0), [0.7,0.8,1/12.5,0.5])
sol = solve(prob_ode_fitzhughnagumo, Tsit5())
```
```julia
sprob_ode_fitzhughnagumo = ODEProblem{false,SciMLBase.FullSpecialize}(fitz, SA[1.0,1.0], (0.0,10.0), SA[0.7,0.8,1/12.5,0.5])
sol = solve(sprob_ode_fitzhughnagumo, Tsit5())
```
Data is genereated by adding noise to the solution obtained above.
```julia
t = collect(range(1,stop=10,length=10))
sig = 0.20
data = convert(Array, VectorOfArray([(sol(t[i]) + sig*randn(2)) for i in 1:length(t)]))
```
### Plot of the data and the solution.
```julia
scatter(t, data[1,:])
scatter!(t, data[2,:])
plot!(sol)
```
### Priors for the parameters which will be passed for the Bayesian Inference
```julia
priors = [truncated(Normal(1.0,0.5),0,1.5), truncated(Normal(1.0,0.5),0,1.5), truncated(Normal(0.0,0.5),0.0,0.5), truncated(Normal(0.5,0.5),0,1)]
```
### Benchmarks
#### Stan.jl backend
```julia
@time bayesian_result_stan = stan_inference(prob_ode_fitzhughnagumo,t,data,priors; delta = 0.65, num_samples = 10_000, print_summary=false, vars=(DiffEqBayes.StanODEData(), InverseGamma(2, 3)))
```
### Direct Turing.jl
```julia
@model function fitlv(data, prob)
# Prior distributions.
σ ~ InverseGamma(2, 3)
a ~ truncated(Normal(1.0,0.5),0,1.5)
b ~ truncated(Normal(1.0,0.5),0,1.5)
τinv ~ truncated(Normal(0.0,0.5),0.0,0.5)
l ~ truncated(Normal(0.5,0.5),0,1)
# Simulate Lotka-Volterra model.
p = SA[a,b,τinv,l]
_prob = remake(prob, p = p)
predicted = solve(_prob, Tsit5(); saveat=t)
# Observations.
for i in 1:length(predicted)
data[:, i] ~ MvNormal(predicted[i], σ^2 * I)
end
return nothing
end
model = fitlv(data, sprob_ode_fitzhughnagumo)
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
```julia
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
#### Turing.jl backend
```julia
@time bayesian_result_turing = turing_inference(prob_ode_fitzhughnagumo,Tsit5(),t,data,priors;num_samples = 10_000)
```
# Conclusion
FitzHugh-Ngumo is a standard problem for parameter estimation studies. In the FitzHugh-Nagumo model the parameters to be estimated were `[0.7,0.8,0.08,0.5]`.
`dynamichmc_inference` has issues with the model and hence was excluded from this benchmark.
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 4408 | ---
title: Lorenz Bayesian Parameter Estimation Benchmarks
author: Vaibhav Dixit, Chris Rackauckas
---
## Parameter estimation of Lorenz Equation using DiffEqBayes.jl
```julia
using DiffEqBayes
using DiffEqCallbacks, StaticArrays
using Distributions, StanSample, DynamicHMC, Turing
using OrdinaryDiffEq, RecursiveArrayTools, ParameterizedFunctions, DiffEqCallbacks
using Plots, LinearAlgebra
```
```julia
gr(fmt=:png)
```
#### Initializing the problem
```julia
g1 = @ode_def LorenzExample begin
dx = σ*(y-x)
dy = x*(ρ-z) - y
dz = x*y - β*z
end σ ρ β
```
```julia
r0 = [1.0; 0.0; 0.0]
tspan = (0.0, 30.0)
p = [10.0,28.0,2.66]
```
```julia
prob = ODEProblem(g1, r0, tspan, p)
sol = solve(prob,Tsit5())
```
```julia
sr0 = SA[1.0; 0.0; 0.0]
tspan = (0.0, 30.0)
sp = SA[10.0,28.0,2.66]
sprob = ODEProblem{false,SciMLBase.FullSpecialize}(g1, sr0, tspan, sp)
sol = solve(sprob,Tsit5())
```
#### Generating data for bayesian estimation of parameters from the obtained solutions using the `Tsit5` algorithm by adding random noise to it.
```julia
t = collect(range(1, stop=30, length=30))
sig = 0.49
data = convert(Array, VectorOfArray([(sol(t[i]) + sig*randn(3)) for i in 1:length(t)]))
```
#### Plots of the generated data and the actual data.
```julia
Plots.scatter(t, data[1,:],markersize=4,color=:purple)
Plots.scatter!(t, data[2,:],markersize=4,color=:yellow)
Plots.scatter!(t, data[3,:],markersize=4,color=:black)
plot!(sol)
```
#### Uncertainity Quantification plot is used to decide the tolerance for the differential equation.
```julia
cb = AdaptiveProbIntsUncertainty(5)
monte_prob = EnsembleProblem(prob)
sim = solve(monte_prob,Tsit5(),trajectories=100,callback=cb,reltol=1e-5,abstol=1e-5)
plot(sim,vars=(0,1),linealpha=0.4)
```
```julia
cb = AdaptiveProbIntsUncertainty(5)
monte_prob = EnsembleProblem(prob)
sim = solve(monte_prob,Tsit5(),trajectories=100,callback=cb,reltol=1e-6,abstol=1e-6)
plot(sim,vars=(0,1),linealpha=0.4)
```
```julia
cb = AdaptiveProbIntsUncertainty(5)
monte_prob = EnsembleProblem(prob)
sim = solve(monte_prob,Tsit5(),trajectories=100,callback=cb,reltol=1e-8,abstol=1e-8)
plot(sim,vars=(0,1),linealpha=0.4)
```
```julia
priors = [truncated(Normal(10,2),1,15),truncated(Normal(30,5),1,45),truncated(Normal(2.5,0.5),1,4)]
```
## Using Stan.jl backend
Lorenz equation is a chaotic system hence requires very low tolerance to be estimated in a reasonable way, we use 1e-8 obtained from the uncertainity plots. Use of truncated priors is necessary to prevent Stan from stepping into negative and other improbable areas.
```julia
@time bayesian_result_stan = stan_inference(prob,t,data,priors; delta = 0.65, reltol=1e-8,abstol=1e-8, vars=(DiffEqBayes.StanODEData(), InverseGamma(2, 3)))
```
### Direct Turing.jl
```julia
@model function fitlv(data, prob)
# Prior distributions.
α ~ InverseGamma(2, 3)
σ ~ truncated(Normal(10, 2), 1, 15)
ρ ~ truncated(Normal(30, 5), 1, 45)
β ~ truncated(Normal(2.5, 0.5), 1, 4)
# Simulate Lotka-Volterra model.
p = SA[σ, ρ, β]
_prob = remake(prob, p = p)
predicted = solve(_prob, Vern9(); saveat=t)
# Observations.
for i in 1:length(predicted)
data[:, i] ~ MvNormal(predicted[i], α^2 * I)
end
return nothing
end
model = fitlv(data, sprob)
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
```julia
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
### Using Turing.jl backend
```julia
@time bayesian_result_turing = turing_inference(prob, Vern9(), t, data, priors; reltol=1e-8, abstol=1e-8, likelihood=(u, p, t, σ) -> MvNormal(u, Diagonal((σ) .^ 2 .* ones(length(u)))), likelihood_dist_priors=[InverseGamma(2, 3), InverseGamma(2, 3), InverseGamma(2, 3)])
```
### Using DynamicHMC.jl backend
```julia
@time bayesian_result_dynamichmc = dynamichmc_inference(prob,Tsit5(),t,data,priors;solve_kwargs = (reltol=1e-8,abstol=1e-8,))
```
## Conclusion
Due to the chaotic nature of Lorenz Equation, it is a very hard problem to estimate as it has the property of exponentially increasing errors.
Its uncertainity plot points to its chaotic behaviour and goes awry for different values of tolerance, we use 1e-8 as the tolerance as it makes its uncertainity small enough to be trusted in `(0,30)` time span.
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 3435 | ---
title: Lotka-Volterra Bayesian Parameter Estimation Benchmarks
author: Vaibhav Dixit, Chris Rackauckas
---
## Parameter Estimation of Lotka-Volterra Equation using DiffEqBayes.jl
```julia
using DiffEqBayes, StanSample, DynamicHMC, Turing
```
```julia
using Distributions, BenchmarkTools, StaticArrays
using OrdinaryDiffEq, RecursiveArrayTools, ParameterizedFunctions
using Plots, LinearAlgebra
```
```julia
gr(fmt=:png)
```
#### Initializing the problem
```julia
f = @ode_def LotkaVolterraTest begin
dx = a*x - b*x*y
dy = -c*y + d*x*y
end a b c d
```
```julia
u0 = [1.0,1.0]
tspan = (0.0,10.0)
p = [1.5,1.0,3.0,1,0]
```
```julia
prob = ODEProblem(f, u0, tspan, p)
sol = solve(prob,Tsit5())
```
```julia
su0 = SA[1.0,1.0]
sp = SA[1.5,1.0,3.0,1,0]
sprob = ODEProblem{false,SciMLBase.FullSpecialize}(f, su0, tspan, sp)
sol = solve(sprob,Tsit5())
```
#### We take the solution data obtained and add noise to it to obtain data for using in the Bayesian Inference of the parameters
```julia
t = collect(range(1,stop=10,length=10))
sig = 0.49
data = convert(Array, VectorOfArray([(sol(t[i]) + sig*randn(2)) for i in 1:length(t)]))
```
#### Plots of the actual data and generated data
```julia
scatter(t, data[1,:], lab="#prey (data)")
scatter!(t, data[2,:], lab="#predator (data)")
plot!(sol)
```
```julia
priors = [truncated(Normal(1.5,0.5),0.5,2.5),truncated(Normal(1.2,0.5),0,2),truncated(Normal(3.0,0.5),1,4),truncated(Normal(1.0,0.5),0,2)]
```
### Stan.jl backend
The solution converges for tolerance values lower than 1e-3, lower tolerance leads to better accuracy in result but is accompanied by longer warmup and sampling time, truncated normal priors are used for preventing Stan from stepping into negative values.
```julia
@btime bayesian_result_stan = stan_inference(prob,t,data,priors,num_samples=10_000,print_summary=false,delta = 0.65, vars = (DiffEqBayes.StanODEData(), InverseGamma(2, 3)))
```
### Direct Turing.jl
```julia
@model function fitlv(data, prob)
# Prior distributions.
σ ~ InverseGamma(2, 3)
α ~ truncated(Normal(1.5, 0.5), 0.5, 2.5)
β ~ truncated(Normal(1.2, 0.5), 0, 2)
γ ~ truncated(Normal(3.0, 0.5), 1, 4)
δ ~ truncated(Normal(1.0, 0.5), 0, 2)
# Simulate Lotka-Volterra model.
p = SA[α, β, γ, δ]
_prob = remake(prob, p = p)
predicted = solve(_prob, Tsit5(); saveat=t)
# Observations.
for i in 1:length(predicted)
data[:, i] ~ MvNormal(predicted[i], σ^2 * I)
end
return nothing
end
model = fitlv(data, sprob)
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
```julia
@time chain = sample(model, NUTS(0.65), 10000; progress=false)
```
### Turing.jl backend
```julia
@btime bayesian_result_turing = turing_inference(prob, Tsit5(), t, data, priors, num_samples=10_000)
```
### DynamicHMC.jl backend
```julia
@btime bayesian_result_dynamichmc = dynamichmc_inference(prob,Tsit5(),t,data,priors,num_samples=10_000)
```
## Conclusion
Lotka-Volterra Equation is a "predator-prey" model, it models population of two species in which one is the predator (wolf) and the other is the prey (rabbit).
It depicts a cyclic behaviour, which is also seen in its Uncertainity Quantification Plots. This behaviour makes it easy to estimate even at very high tolerance values (1e-3).
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 11413 | ---
title: BCR Work-Precision Diagrams
author: Samuel Isaacson and Chris Rackauckas
---
The following benchmark is of 1122 ODEs with 24388 terms that describe a stiff
chemical reaction network modeling the BCR signaling network from [Barua et
al.](https://doi.org/10.4049/jimmunol.1102003). We use
[`ReactionNetworkImporters`](https://github.com/isaacsas/ReactionNetworkImporters.jl)
to load the BioNetGen model files as a
[Catalyst](https://github.com/SciML/Catalyst.jl) model, and then use
[ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl) to convert the
Catalyst network model to ODEs.
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools,
LinearSolve
gr()
datadir = joinpath(dirname(pathof(ReactionNetworkImporters)),"../data/bcr")
const to = TimerOutput()
tf = 100000.0
# generate ModelingToolkit ODEs
@timeit to "Parse Network" prnbng = loadrxnetwork(BNGNetwork(), joinpath(datadir, "bcr.net"))
show(to)
rn = prnbng.rn
obs = [eq.lhs for eq in observed(rn)]
@timeit to "Create ODESys" osys = convert(ODESystem, rn)
show(to)
tspan = (0.,tf)
@timeit to "ODEProb No Jac" oprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[])
show(to)
oprob_sparse = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[]; sparse=true);
```
```julia
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
```
```julia
@show numspecies(rn) # Number of ODEs
@show numreactions(rn) # Apprx. number of terms in the ODE
@show length(parameters(rn)); # Number of Parameters
```
## Time ODE derivative function compilation
As compiling the ODE derivative functions has in the past taken longer than
running a simulation, we first force compilation by evaluating these functions
one time.
```julia
u = ModelingToolkit.varmap_to_vars(nothing, species(rn); defaults=ModelingToolkit.defaults(rn))
du = copy(u)
p = ModelingToolkit.varmap_to_vars(nothing, parameters(rn); defaults=ModelingToolkit.defaults(rn))
@timeit to "ODE rhs Eval1" oprob.f(du,u,p,0.)
@timeit to "ODE rhs Eval2" oprob.f(du,u,p,0.)
sparsejacprob.f(du,u,p,0.)
```
We also time the ODE rhs function with BenchmarkTools as it is more accurate
given how fast evaluating `f` is:
```julia
@btime oprob.f($du,$u,$p,0.)
```
```julia
Js = similar(sparsejacprob.f.jac_prototype)
@timeit to "SparseJac Eval1" sparsejacprob.f.jac(Js,u,p,0.)
@timeit to "SparseJac Eval2" sparsejacprob.f.jac(Js,u,p,0.)
show(to)
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF(), saveat=tf/1000., reltol=1e-5, abstol=1e-5)
plot(sol; idxs=obs, legend=false, fmt=:png)
```
## Generate Test Solution
```julia
@time sol = solve(oprob, CVODE_BDF(), abstol=1/10^12, reltol=1/10^12)
test_sol = TestSolution(sol);
```
## Setups
#### Sets plotting defaults
```julia
default(legendfontsize=7,framestyle=:box,gridalpha=0.3,gridlinewidth=2.5)
```
#### Declares a plotting helper function
```julia
function plot_settings(wp)
times = vcat(map(wp -> wp.times, wp.wps)...)
errors = vcat(map(wp -> wp.errors, wp.wps)...)
xlimit = 10 .^ (floor(log10(minimum(errors))), ceil(log10(maximum(errors))))
ylimit = 10 .^ (floor(log10(minimum(times))), ceil(log10(maximum(times))))
return xlimit,ylimit
end
```
#### Declare pre-conditioners
```julia
using IncompleteLU, LinearAlgebra
const τ = 1e2
const τ2 = 1e2
jaccache = sparsejacprob.f.jac(oprob.u0,oprob.p,0.0)
W = I - 1.0*jaccache
prectmp = ilu(W, τ = τ)
preccache = Ref(prectmp)
function psetupilu(p, t, u, du, jok, jcurPtr, gamma)
if !jok
sparsejacprob.f.jac(jaccache,u,p,t)
jcurPtr[] = true
# W = I - gamma*J
@. W = -gamma*jaccache
idxs = diagind(W)
@. @view(W[idxs]) = @view(W[idxs]) + 1
# Build preconditioner on W
preccache[] = ilu(W, τ = τ)
end
end
function precilu(z,r,p,t,y,fy,gamma,delta,lr)
ldiv!(z,preccache[],r)
end
function incompletelu(W,du,u,p,t,newW,Plprev,Prprev,solverdata)
if newW === nothing || newW
Pl = ilu(convert(AbstractMatrix,W), τ = τ2)
else
Pl = Plprev
end
Pl,nothing
end;
```
#### Sets tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (5:8);
```
## Failures
Before proceding to the results, we note the notable omissions. CVODE with KLU diverges in the solution, and
thus it is omitted from the results:
```julia
solve(sparsejacprob,CVODE_BDF(linear_solver=:KLU), abstol=1e-8, reltol=1e-8);
```
## Work-Precision Diagrams (CVODE and lsoda solvers)
#### Declare solvers.
```julia
setups = [
Dict(:alg=>lsoda(), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:LapackDense), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES,prec=precilu,psetup=psetupilu,prec_side=1), :prob_choice => 2),
];
```
#### Plot Work-Precision Diagram.
```julia
wp = WorkPrecisionSet([oprob,oprob_sparse,sparsejacprob],abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=[test_sol,test_sol,test_sol],maxiters=Int(1e6),numruns=1)
names = ["lsoda" "CVODE_BDF" "CVODE_BDF (LapackDense)" "CVODE_BDF (GMRES)" "CVODE_BDF (GMRES, iLU)" "CVODE_BDF (KLU, sparse jac)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Work-Precision Diagrams (various Julia solvers)
#### Declare solvers (using default linear solver).
```julia
setups = [
Dict(:alg=>TRBDF2(autodiff=false)),
Dict(:alg=>QNDF(autodiff=false)),
Dict(:alg=>FBDF(autodiff=false)),
Dict(:alg=>KenCarp4(autodiff=false))
];
```
#### Plot Work-Precision Diagram (using default linear solver).
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e6),numruns=1)
names = ["TRBDF2" "QNDF" "FBDF" "KenCarp4"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using GMRES linear solver).
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>KenCarp4(linsolve=KrylovJL_GMRES(),autodiff=false))
];
```
#### Plot Work-Precision Diagram (using GMRES linear solver).
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e6),numruns=1)
names = ["TRBDF2 (GMRES)" "QNDF (GMRES)" "FBDF (GMRES)" "KenCarp4 (GMRES)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using GMRES linear solver, with pre-conditioner).
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>KenCarp4(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true))
];
```
#### Plot Work-Precision Diagram (using GMRES linear solver, with pre-conditioner).
```julia
wp = WorkPrecisionSet(sparsejacprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e6),numruns=1)
names = ["TRBDF2 (GMRES, iLU)" "QNDF (GMRES, iLU)" "FBDF (GMRES, iLU)" "KenCarp4 (GMRES, iLU)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using sparse jacobian)
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>QNDF(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>FBDF(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>KenCarp4(linsolve=KLUFactorization(),autodiff=false))
];
```
#### Plot Work-Precision Diagram (using sparse jacobian)
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(sparsejacprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e6),numruns=1)
names = ["TRBDF2 (KLU, sparse jac)" "QNDF (KLU, sparse jac)" "FBDF (KLU, sparse jac)" "KenCarp4 (KLU, sparse jac)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Summary of results
Finally, we compute a single diagram comparing the various solvers used.
#### Declare solvers
We designate the solvers we wish to compare.
```julia
setups = [
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES,prec=precilu,psetup=psetupilu,prec_side=1), :prob_choice => 2),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true), :prob_choice => 3),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true), :prob_choice => 3),
Dict(:alg=>QNDF(linsolve=KLUFactorization(),autodiff=false), :prob_choice => 3),
Dict(:alg=>FBDF(linsolve=KLUFactorization(),autodiff=false), :prob_choice => 3),
Dict(:alg=>KenCarp4(linsolve=KLUFactorization(),autodiff=false), :prob_choice => 3)
];
```
#### Plot Work-Precision Diagram
For these, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet([oprob,oprob_sparse,sparsejacprob],abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=[test_sol,test_sol,test_sol],maxiters=Int(1e9),numruns=200)
names = ["CVODE_BDF (GMRES, iLU)" "QNDF (GMRES, iLU)" "FBDF (GMRES, iLU)" "QNDF (KLU, sparse jac)" "FBDF (KLU, sparse jac)" "KenCarp4 (KLU, sparse jac)"]
colors = [:green :deepskyblue1 :dodgerblue2 :royalblue2 :slateblue3 :lightskyblue]
markershapes = [:octagon :hexagon :rtriangle :pentagon :ltriangle :star5]
xlimit,ylimit = plot_settings(wp)
xlimit = xlimit .* [0.95,1/0.95]; ylimit = ylimit .* [0.95,1/0.95];
plot(wp;label=names,left_margin=10Plots.mm,right_margin=10Plots.mm,xlimit=xlimit,ylimit=ylimit,xticks=[1e-3,1e-2,1e-1,1e0,1e1,1e2,1e3],yticks=[1e0,1e1,1e2,1e3],color=colors,markershape=markershapes,legendfontsize=15,tickfontsize=15,guidefontsize=15, legend=:topright, lw=20, la=0.8, markersize=20,markerstrokealpha=1.0, markerstrokewidth=1.5, gridalpha=0.3, gridlinewidth=7.5,size=(1100,1000))
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 76290 | ---
title: Bidkhori2012 Work-Precision Diagrams
author: Utkarsh
---
The following benchmark is of 109 ODEs and 188 parameters that describe a stiff
SBML model, modelling the EGFR signalling between normal and NSCLC cells [Bidkhori et
al.](https://doi.org/10.1371/journal.pone.0048004). It is referenced from [Biomodels Database](https://www.ebi.ac.uk/biomodels/curation/index),
and the model was parsed to Julia are finally to ODEs using [ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl).
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools, JLD2
gr()
par = load(joinpath(@__DIR__, "params_Bidkhori2012.jld2"))
```
# Defining the reaction system
```julia
function sbml_model!(du, u, p, t)
# assignmentRule: variable = mwa6994523_5d45_4000_af0c_3e94073bf183
u88 = u[80] + u[79]
reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12 = p["reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12_mw575f7f49_3663_47f1_b492_5b92c1c4345d"] * u[1] * u[2] - p["reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12_mw53c64fd3_9a1c_4947_a734_74a73554964c"] * u[3]
reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d = p["reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d_mw8cfaf07f_dabe_45de_93cc_ef2c7fd31104"] * u[3] * u[3] - p["reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d_mwab52aceb_4b19_4317_b2da_97ccbb973dab"] * u[4]
reaction_mw413c6d45_ab23_4d3e_87b3_a8ed4629b923 = p["reaction_mw413c6d45_ab23_4d3e_87b3_a8ed4629b923_mw6b97a1ec_2cba_4bce_96f7_ec1d0fa2d16c"] * u[4]
reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04 = p["reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04_mwf1697f55_a3f4_4fb6_ae1d_f96f09ad1daa"] * u[5] * u[7] - p["reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04_mw880a5942_7549_4466_bd19_0e1768a3a533"] * u[8]
reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644 = p["reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644_mw7e889122_d26c_4d09_bae4_d313b992dc8e"] * u[5] * u[9] - p["reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644_mwff6f49f7_268a_4f08_8d36_3ad8449d7472"] * u[10]
reaction_mw974c39f5_b82e_44b3_abec_7a724f46c526 = p["reaction_mw974c39f5_b82e_44b3_abec_7a724f46c526_mwe645e76e_bb00_4c22_b25e_a2e77a6aada2"] * u[8]
reaction_mw9544e67b_b6d0_4941_b7e0_ecd4f400a335 = p["reaction_mw9544e67b_b6d0_4941_b7e0_ecd4f400a335_mwb0744746_88a2_488e_a483_266747a044c6"] * u[10]
reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe = p["reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe_mw9e24066c_51a5_4c7a_af7c_4656155a4eb0"] * u[11] - p["reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe_mwab1ef4d4_2acc_4fa2_b07c_fac51fb7bfaf"] * u[5] * u[12]
reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db = p["reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db_mwc4824ff0_2b51_4d66_ad48_1145f670a6e1"] * u[12] * u[9] - p["reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db_mw0f1d282f_1c6b_455c_8254_3760632c6ecc"] * u[13]
reaction_mweda6a945_fb5d_4d99_9958_11b2b2840308 = p["reaction_mweda6a945_fb5d_4d99_9958_11b2b2840308_mw0aa92e25_f9aa_461e_92b8_23b1b5b3ab92"] * u[13]
reaction_mwd4bf58ea_70c9_43ea_a831_1fcde130ba28 = p["reaction_mwd4bf58ea_70c9_43ea_a831_1fcde130ba28_mw2a4ed8a2_fce4_44a4_adb9_edc24a06b4e1"] * u[12]
reaction_mw4817365e_a33b_451f_bee1_de748377ede2 = p["reaction_mw4817365e_a33b_451f_bee1_de748377ede2_mwe879a9ac_4b8d_4c9a_a157_a3751761cf63"] * u[11] * u[14] - p["reaction_mw4817365e_a33b_451f_bee1_de748377ede2_mwa18578d7_236f_4939_baca_52259e38fe15"] * u[15]
reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2 = p["reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2_mw289fed85_e6ee_43e6_a69f_77b5f487a452"] * u[15] * u[9] - p["reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2_mw8768b5c7_b227_4825_aa55_a525b0d915c2"] * u[16]
reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6 = p["reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6_mwd12a67b3_6d98_40e9_a54b_282a577498eb"] * u[16]
reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3 = p["reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3_mw6ac313e2_e8a9_42a9_b13a_27e55c1012a2"] * u[15] * u[17] - p["reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3_mw93f832d7_eefb_43dd_853c_a0d7a76023cf"] * u[18]
reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a = p["reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a_mwbb727dc5_30e8_45f4_9d15_3b34be5c1e93"] * u[14] * u[17] - p["reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a_mw7ae1ee96_563e_4684_bc9a_8f4ef373620e"] * u[20]
reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc = p["reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc_mwbc5340b6_06b7_4081_bd0c_e7a397f06a92"] * u[11] * u[20] - p["reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc_mw0df80c0e_c32b_4f90_99bd_e8f90e4c8109"] * u[18]
reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61 = p["reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61_mwc585e0e4_b7e7_4290_8a6d_10fcd9759a2d"] * u[5] * u[14] - p["reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61_mwf44d37d0_fe7f_4e47_bf10_1e734fbc3391"] * u[21]
reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb = p["reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb_mw3d564c3c_aa54_4c16_90be_662cfcbf8bc8"] * u[21] * u[9] - p["reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb_mw371642bb_3836_4ded_93a5_68fa9b464896"] * u[22]
reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93 = p["reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93_mw736e4a7b_4a25_4d32_b96b_b088e3bd41e7"] * u[22]
reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2 = p["reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2_mw084cd67b_f328_48a7_8e16_1d6256c8c137"] * u[21] * u[17] - p["reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2_mw43f177dc_f522_4dd1_b8e5_21b2b8fdfdba"] * u[23]
reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd = p["reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd_mwfa6a58ab_0ca5_4c05_92b0_870593ac135d"] * u[5] * u[20] - p["reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd_mwb9547c37_09b7_4258_95ab_8039d4088298"] * u[23]
reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e = p["reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e_mw7e09242b_bd80_4af0_90c8_e0cddace89fe"] * u[18] * u[25] - p["reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e_mw2dfc8a19_1792_4e12_af38_8bfbda31a577"] * u[26]
reaction_mw921ee820_1dbb_4b5f_866c_87da620d8f89 = p["reaction_mw921ee820_1dbb_4b5f_866c_87da620d8f89_mw553c0b3c_af7f_4309_8c61_0f1e2c32347c"] * u[27]
reaction_mw0bcfad86_59b9_42ff_bcb7_fbb44845049d = p["reaction_mw0bcfad86_59b9_42ff_bcb7_fbb44845049d_mwfc146e94_8070_4727_8416_fb55829068cb"] * u[26]
reaction_mwe9b50ac7_dac3_4eba_b1db_b3fd392d8fb7 = p["reaction_mwe9b50ac7_dac3_4eba_b1db_b3fd392d8fb7_mw26688d02_8ab9_4123_89c4_022b981cb72c"] * u[28]
reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f = p["reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f_mw5639395a_a5cd_46dd_81b8_30fe72400a2e"] * u[23] * u[25] - p["reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f_mw9cc637fe_d9ca_47d2_a4dc_66009d458094"] * u[28]
reaction_mw3c617363_649b_4460_a694_36f7a3127a62 = p["reaction_mw3c617363_649b_4460_a694_36f7a3127a62_mw19173345_925d_427b_8658_add0978e5931"] * u[27] * u[29] - p["reaction_mw3c617363_649b_4460_a694_36f7a3127a62_mw9f6790d7_19ce_41d9_b4de_a1658c047501"] * u[30]
reaction_mwf31259aa_32b7_4104_be70_045297b9a512 = p["reaction_mwf31259aa_32b7_4104_be70_045297b9a512_mw23e16d40_acbb_4658_a336_be5d0b0dd86a"] * u[30]
reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c = p["reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c_mw10c97b8e_72aa_4f56_b3b9_c94baad7e213"] * u[5] * u[29] - p["reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c_mw0b6eb5f7_b133_4b3d_bf15_9fd6c2e9332d"] * u[31]
reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53 = p["reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53_mwe483687f_b591_4c42_9abc_7ea9f47470bf"] * u[31] * u[27] - p["reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53_mwcf964aba_9db6_46c5_b687_beafc5d89169"] * u[32]
reaction_mw652570eb_c9d3_499b_b877_61d360b10980 = p["reaction_mw652570eb_c9d3_499b_b877_61d360b10980_mwb881f20a_cf8a_493a_aa84_59ee90f26dd9"] * u[32]
reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19 = p["reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19_mwb4c6ed27_c7ec_438f_bafd_4a09a9f356f1"] * u[31] * u[9] - p["reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19_mwba77a9ba_078d_4ec6_a8b8_d7042a2cefe7"] * u[33]
reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a = p["reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a_mwe1743f7b_ca2c_47d4_91d7_aed2748d98c5"] * u[33]
reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3 = p["reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3_mw9f1dbbe6_8aa3_4180_bcea_04343649d7ba"] * u[34] * u[27] - p["reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3_mwdf20ff60_f0b7_4c2a_b393_586ec1337e67"] * u[35]
reaction_mw42c97708_4f85_45a8_9141_d0ae529409ca = p["reaction_mw42c97708_4f85_45a8_9141_d0ae529409ca_mw91f2ca92_9556_4fb8_ae12_0b72f3e3f261"] * u[35]
reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33 = p["reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33_mw77c60377_28ae_4aad_b911_5768fc8b824f"] * u[36] * u[37] - p["reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33_mw2eed2db0_ba78_435b_b2c8_ee91efdba1b4"] * u[38]
reaction_mw1bd186cf_4762_480a_b70d_d7a775462398 = p["reaction_mw1bd186cf_4762_480a_b70d_d7a775462398_mw7e974605_8d9c_4250_8f69_072aab1f24f7"] * u[38]
reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2 = p["reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2_mw11cdaca9_941c_4a59_ba2a_3bfeafb65aeb"] * u[36] * u[39] - p["reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2_mw58c37b3e_91e7_445e_846e_77cd0b2320af"] * u[40]
reaction_mwb49058ff_2997_4187_abe7_4dce4ccf6ff4 = p["reaction_mwb49058ff_2997_4187_abe7_4dce4ccf6ff4_mw432640ec_11b9_484d_ba26_415538ab9a10"] * u[40]
reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe = p["reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe_mw11bb74b8_d908_46f0_ac4d_06e8dd1aa5ae"] * u[41] * u[42] - p["reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe_mwb44117f5_20b2_495e_adf3_3467cd119fd6"] * u[43]
reaction_mwf95f743d_6108_49fe_8ffd_bdcc1a9f9a8d = p["reaction_mwf95f743d_6108_49fe_8ffd_bdcc1a9f9a8d_mwa4c71b8d_fb74_465b_b76e_cec4e4c95484"] * u[43]
reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9 = p["reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9_mwc40b3165_cc16_4f78_86b5_e34f2731dcbb"] * u[41] * u[44] - p["reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9_mw8bff2fe0_b582_4020_8f05_83f14451b1c0"] * u[45]
reaction_mw6fd24d16_f57d_46c6_82f5_3f00759fa16b = p["reaction_mw6fd24d16_f57d_46c6_82f5_3f00759fa16b_mw3d07dc22_f821_49a5_9712_820ba9592353"] * u[45]
reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d = p["reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d_mwa8f70790_9f44_4548_988e_49d13016d2f1"] * u[36] * u[47] - p["reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d_mwaad540b6_783e_4576_8862_ad522fd897db"] * u[48]
reaction_mw87711dc1_43d7_40fc_b9e9_a24e2f92419d = p["reaction_mw87711dc1_43d7_40fc_b9e9_a24e2f92419d_mwfbc395b5_05b8_4e27_9696_c3ba52edaf74"] * u[48]
reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b = p["reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b_mwc489f472_68ce_44e7_aad1_f8d2f6dda4ff"] * u[41] * u[49] - p["reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b_mw56f1bdc0_66fd_47c0_806a_beeaf123e2f2"] * u[50]
reaction_mw40950d59_1012_4361_8418_73e25758e367 = p["reaction_mw40950d59_1012_4361_8418_73e25758e367_mwa17c895f_29d8_4977_a99f_cf9bf6216785"] * u[50]
reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419 = p["reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419_mwafd23622_952d_44b3_a437_4aa12422add7"] * u[39] * u[49] - p["reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419_mw9d9a7d08_b19a_44f1_a806_151597049345"] * u[51]
reaction_mwa4b69c77_6226_46da_b78c_3e6027d0be41 = p["reaction_mwa4b69c77_6226_46da_b78c_3e6027d0be41_mwac85fd83_4e73_43f1_9c42_01773349d50f"] * u[51]
reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48 = p["reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48_mwd23d026b_c5b7_4742_aab9_b9beb18ec9bc"] * u[46] * u[52] - p["reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48_mwf4c4d7a7_1498_4f6c_9d72_cd5cb012146c"] * u[54]
reaction_mw61305f93_7b2d_4a2d_8d16_f7be026d8671 = p["reaction_mw61305f93_7b2d_4a2d_8d16_f7be026d8671_mwe3e5abe4_9f92_43eb_92e4_cea771f5bf14"] * u[54]
reaction_mwcc31b497_6c50_446c_bbc2_6c5739507252 = p["reaction_mwcc31b497_6c50_446c_bbc2_6c5739507252_mwa617804d_95cc_4197_a39b_264a2c66b5a3"] * u[53]
reaction_mw1d8c2435_bb85_4352_a25f_82033250579e = p["reaction_mw1d8c2435_bb85_4352_a25f_82033250579e_mw254868f8_c9fb_493c_bc1d_807cc83c18e6"] * u[44] * u[52] - p["reaction_mw1d8c2435_bb85_4352_a25f_82033250579e_mw78a41659_4abc_4614_9e83_38cbfe1c5262"] * u[53]
reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c = p["reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c_mwbc2119ce_ade3_4e2a_a3bc_a29cd77adf72"] * u[46] * u[18] - p["reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c_mw54b0e5e9_710f_438e_a8d3_749c594667bc"] * u[55]
reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730 = p["reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730_mw1ddaf9f4_dcab_4dc2_a6fa_5ce85b9d7a3a"] * u[55]
reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30 = p["reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30_mw60892818_7ef4_4f65_8003_9700a708c66c"] * u[46] * u[23] - p["reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30_mw6843d346_6e9f_43d5_97f6_1059f164aa16"] * u[57]
reaction_mw4685274a_2b55_429f_927f_3fd863592af6 = p["reaction_mw4685274a_2b55_429f_927f_3fd863592af6_mwdaa378da_64fe_4ea4_b79d_c25733837b9f"] * u[57]
reaction_mw8e331e43_16b4_478d_880b_d5a3244540e4 = p["reaction_mw8e331e43_16b4_478d_880b_d5a3244540e4_mw3f5e2165_9bb6_4ac3_992e_50943dd2ea05"] * u[56]
reaction_mw47dee769_daa0_4af4_978a_5ab17e504c2f = p["reaction_mw47dee769_daa0_4af4_978a_5ab17e504c2f_mwe49ede89_014e_40f2_acfd_0d1a0cd11fe7"] * u[58]
reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b = p["reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b_mw90873203_7a5d_4fca_a789_5e989ff0c999"] * u[18] * u[6] - p["reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b_mw92d81b3b_fa59_4637_8540_8cb8482490d9"] * u[19]
reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630 = p["reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630_mwcc2a950d_261b_4fd7_9c08_9f3c194ba09d"] * u[19] * u[60] - p["reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630_mw1351daea_68be_404a_b7b0_105920ff3371"] * u[59]
reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657 = p["reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657_mwc6b3c76f_af7b_488c_8751_28f1d9ab90a1"] * u[59]
reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4 = p["reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4_mwf9c81339_e73a_45b5_a714_0854b718d44f"] * u[23] * u[6] - p["reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4_mw587125c7_6092_4627_9cdd_2415b77a8307"] * u[24]
reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014 = p["reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014_mwa575cf96_3d57_4222_ac71_bd17006ef035"] * u[24] * u[60] - p["reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014_mwf7658bc6_acb6_411e_ae2c_9d8de7738d5f"] * u[61]
reaction_mweb93165f_cf03_48f1_b035_59d79e324314 = p["reaction_mweb93165f_cf03_48f1_b035_59d79e324314_mwa137184a_0eb0_4bcb_971c_8e19231b2c07"] * u[61]
reaction_mw85e457d1_73f8_4236_bb61_a128d300003f = p["reaction_mw85e457d1_73f8_4236_bb61_a128d300003f_mwfa680314_051c_4b10_afc9_7e7fbee49e3f"] * u[5] * u[6] - p["reaction_mw85e457d1_73f8_4236_bb61_a128d300003f_mw97b9ab43_02ae_4e42_a524_6b781633a255"] * u[62]
reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6 = p["reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6_mwcc0d3fcd_9b9e_4390_b588_e57b57d89d22"] * u[62] * u[60] - p["reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6_mw56f1be7e_e303_4a72_be17_5bd08e3eb1f2"] * u[63]
reaction_mwc9b3b248_3290_452a_9b7c_8fdada3e6687 = p["reaction_mwc9b3b248_3290_452a_9b7c_8fdada3e6687_mw1decb177_5075_41f3_a348_ca13b8f4497e"] * u[63]
reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d = p["reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d_mw001b8124_b461_482a_8c8e_30bffc6718f7"] * u[5] * u[64] - p["reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d_mw40eca7d6_80b2_4926_9c2f_330422db0814"] * u[65]
reaction_mw2c5858f3_0988_49b0_a94a_057853b84e91 = p["reaction_mw2c5858f3_0988_49b0_a94a_057853b84e91_mwf3d00ca5_89dc_4693_92ec_a47db8150144"] * u[65] - p["reaction_mw2c5858f3_0988_49b0_a94a_057853b84e91_mw91a84697_3231_4fa6_b6ff_d69ee86056dc"] * u[66]
reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8 = p["reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8_mw901b5284_bdae_4040_b77d_10f1ec267f06"] * u[65] - p["reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8_mw94cadd24_0432_4f89_a6fc_96cb0475c44e"] * u[5] * u[67]
reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31 = p["reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31_mw688106ee_719d_4995_b1a0_faeefdb0af5a"] * u[68] * u[67] - p["reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31_mw85c8ff7d_8d7c_4403_8a58_4996a3e6ac28"] * u[69]
reaction_mw837b5ad7_4a8c_4c55_94ff_0fdd63048044 = p["reaction_mw837b5ad7_4a8c_4c55_94ff_0fdd63048044_mw4f6f44d9_408e_49b2_bedf_d34b2448725e"] * u[69]
reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621 = p["reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621_mwd3e2533f_8d57_407c_834d_e0dde30b7f4a"] * u[70] - p["reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621_mwbd416b7b_f9b6_4464_b9e8_be4ac001d13d"] * u[68] * u[64]
reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0 = p["reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0_mw64664eb9_353a_4f1d_a8dc_e22bcb06e2c2"] * u[67] * u[71] - p["reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0_mw0573df9d_f365_40b7_83d4_3846a05aefdc"] * u[72]
reaction_mw5dcc8719_3180_4bd0_8797_08e256131961 = p["reaction_mw5dcc8719_3180_4bd0_8797_08e256131961_mw134431c3_e8e5_4375_89a0_2c51a03d65dd"] * u[72]
reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b = p["reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b_mw22510791_ef7e_4373_907c_9eecbc8adda7"] * u[74] * u[73] - p["reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b_mwf59d397b_cfee_4a84_9279_134cc951db8c"] * u[75]
reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef = p["reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef_mwe2aded94_f2b5_4513_8670_71a86abf7968"] * u[75] * u[76] - p["reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef_mw8d6eacb6_7184_4564_8cde_53e93add2146"] * u[77]
reaction_mw98da32e0_b061_40c5_9d32_40744134f3fa = p["reaction_mw98da32e0_b061_40c5_9d32_40744134f3fa_mw3c3648cb_6d56_4d9d_be47_129483778fd6"] * u[77]
reaction_mw31369230_1f14_45bd_be02_a44a275c6e31 = p["reaction_mw31369230_1f14_45bd_be02_a44a275c6e31_mw98405e53_330b_4a64_a700_a62bb3f21426"] * u[78] - p["reaction_mw31369230_1f14_45bd_be02_a44a275c6e31_mw11f8de84_6639_486d_bf17_8f7021f54b66"] * u[79] * u[76]
reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6 = p["reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6_mw65e1222f_39ad_4a29_ae76_04b7d591af38"] * u[79] - p["reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6_mw11e520e6_b1f1_4802_af71_92a2bd9cb644"] * u[80] * u[73]
reaction_mwf3d393e9_ae09_4eab_a39a_ed0eef0f54bc = p["reaction_mwf3d393e9_ae09_4eab_a39a_ed0eef0f54bc_mw6a4e035b_11a7_4155_9a78_cfba13631cb1"] * u[81]
reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92 = p["reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92_mw6eebbe41_cf28_46e8_930c_26f50e08d602"] * u[82] - p["reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92_mw751c2663_d807_482f_991b_c8032cb6d996"] * u[74] * u[83]
reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9 = p["reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9_mwd2d0b340_bbdb_40bd_9eac_992a2a402b94"] * u[80] * u[83] - p["reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9_mwb1b46773_a218_4f99_a000_a98fbc1275d7"] * u[81]
reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371 = p["reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371_mw193f2553_1ab3_4b07_9b4b_201ee9e08c96"] * u[79] * u[83] - p["reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371_mwb7292ff5_dd13_41aa_b9b8_2c0c75d35fb1"] * u[84]
reaction_mw94b3bae0_4da9_4358_a5ac_a46a5cbf621b = p["reaction_mw94b3bae0_4da9_4358_a5ac_a46a5cbf621b_mwf4069175_b898_4633_ac1e_20f44431c36a"] * u[84]
reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e = p["reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e_mw6d852e8c_c64a_4926_80c4_781a9c04b20e"] * u[85] - p["reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e_mw4d614bfc_3e20_450e_8890_6326afd0a0d7"] * u[75] * u[83]
reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5 = p["reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5_mw3676a900_b098_4a74_a511_e15984ca0cd2"] * u[78] * u[83] - p["reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5_mwf68a0726_94b5_4be1_933f_1ac48053601d"] * u[86]
reaction_mw75acd2d1_3fdf_4c3f_8d99_6d62f825d5e2 = p["reaction_mw75acd2d1_3fdf_4c3f_8d99_6d62f825d5e2_mwb4f0353c_d140_44cc_ab75_566fcc2909c5"] * u[86]
reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174 = p["reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174_mw6165953d_ce44_4b21_a18a_c401c04993f1"] * u[87] - p["reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174_mw99a30aef_212a_4577_bcfd_8c5764057cca"] * u[77] * u[83]
reaction_mw950485f2_4463_4309_a4e4_cc81d16ffb7f = p["reaction_mw950485f2_4463_4309_a4e4_cc81d16ffb7f_mw94b0216f_3353_4b36_b9b7_fd34a0510b08"] * u88 * u[36] / (p["reaction_mw950485f2_4463_4309_a4e4_cc81d16ffb7f_mw2034bbe7_27cc_410c_9870_1f8a5986dfa5"] + u[36])
reaction_mw62f71309_e066_47d2_9b99_01f78a51c218 = p["reaction_mw62f71309_e066_47d2_9b99_01f78a51c218_mw0cea56f3_1cdb_410e_a5a4_f3635ba5c94b"] * u[89]
reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01 = p["reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01_mw50a0e884_a88c_46a7_b985_788868bc1029"] * u[5] * u[90] - p["reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01_mw2c88e0e2_e9c3_4e4c_bb2e_b0cd1f6420f4"] * u[91]
reaction_mw65b9e026_bc6c_4c94_8b37_8b9acdf50c8a = p["reaction_mw65b9e026_bc6c_4c94_8b37_8b9acdf50c8a_mw95e2190d_8e39_419b_ad26_7cc141f7b87b"] * u[91]
reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3 = p["reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3_mw76d68ace_272d_4178_bba2_74dfdf260c70"] * u[5] * u[92] - p["reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3_mwe37b936f_7781_4a01_b59b_96bd7db0c49e"] * u[93]
reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618 = p["reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618_mwb6701ead_d3f2_4eb3_8b08_341cea49a4b2"] * u[92] * u[94] - p["reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618_mwa5016035_3f9f_44fc_9f69_1d7a0155eb36"] * u[95]
reaction_mwe9988e4a_083c_4f8e_b154_3e599c9307b0 = p["reaction_mwe9988e4a_083c_4f8e_b154_3e599c9307b0_mw26164d03_adda_4a21_b5ac_59e1d5a8d8ab"] * u[95]
reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735 = p["reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735_mw9fe16c2b_7271_4e4f_b6de_c149721a3198"] * u[92] * u[92] - p["reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735_mw74ea5b55_ead0_4b6f_8da0_fd1dcf7e231d"] * u[97]
reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2 = p["reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2_mw8cbe6595_6f16_4704_afe2_0dd043a175fa"] * u[97] * u[94] - p["reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2_mw21d22acd_ddd4_4794_9700_52201984f75b"] * u[96]
reaction_mw75c6078f_fb76_4ca9_9fdd_e221e3ba57ad = p["reaction_mw75c6078f_fb76_4ca9_9fdd_e221e3ba57ad_mw81384973_14a0_4498_ab21_f70666d46d7f"] * u[96]
reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2 = p["reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2_mw9f1a7f64_0b37_42df_9dd5_e1a44efdcbba"] * u[90] * u[92] - p["reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2_mw366e6f17_4081_4cdc_9fa5_0aeb354d692c"] * u[98]
reaction_mwec4127b5_6bcf_4128_aff4_a6b3c470f690 = p["reaction_mwec4127b5_6bcf_4128_aff4_a6b3c470f690_mw1df2caba_8e41_4fe5_a1b5_7777eb98ed1c"] * u[97]
reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a = p["reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a_mw5a798f7a_b4eb_4a27_b413_4ff3956b90e9"] * u[100] * u[100] - p["reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a_mw54178365_18c1_47e0_94ee_6b96582c52ef"] * u[99]
reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7 = p["reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7_mw1ff4e75e_fce5_4a7a_907b_05df4981f80b"] * u[99] * u[101] - p["reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7_mw8b269d52_eda9_4dd1_8616_ebcf29c971fa"] * u[102]
reaction_mw3b0c171c_6d60_41ca_8193_83cd5e6c188c = p["reaction_mw3b0c171c_6d60_41ca_8193_83cd5e6c188c_mw90b25c4b_ad1a_4ee5_ae20_c60451484516"] * u[102]
reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7 = p["reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7_mwa0806e7a_a90d_4187_9c37_6d9ea569a447"] * u[104] * u[100] - p["reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7_mw95cb9071_56e2_447d_b7c7_59ac96baa623"] * u[103]
reaction_mw45d92b79_0656_4795_87d0_7a465949ca43 = p["reaction_mw45d92b79_0656_4795_87d0_7a465949ca43_mwba545ecf_c7d4_4a6c_8c47_9e91f052d5a9"] * u[100] * u[101] - p["reaction_mw45d92b79_0656_4795_87d0_7a465949ca43_mw01c5ceef_57a1_4baa_b2cd_fd39e9588a10"] * u[105]
reaction_mwb71945c2_03a8_4fad_a995_e1caeee98525 = p["reaction_mwb71945c2_03a8_4fad_a995_e1caeee98525_mw7aba6db3_c7ec_4192_bb5e_0ac4b466c1a5"] * u[105]
reaction_mwd189238c_e8f9_40be_b4ea_18a42bba1b4f = p["reaction_mwd189238c_e8f9_40be_b4ea_18a42bba1b4f_mw31eb851a_c381_419d_b694_f158b7f5cfb6"] * u[104]
reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df = p["reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df_mwe09b67b9_0d2a_4b82_91ef_5284216beb94"] * u[91] * u[6] - p["reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df_mw77a6c207_ff8c_463c_9b4e_8a7d96652b79"] * u[106]
reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e = p["reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e_mw1df53838_48e5_4331_9084_3790409ad5ff"] * u[106] * u[60] - p["reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e_mwe4573b2c_5f99_40d0_9f9e_c238caa5ccbe"] * u[107]
reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19 = p["reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19_mw8ed5885f_774e_48a0_9338_fe8cdd512023"] * u[107]
reaction_mwb205f533_4013_406b_8a4b_691ec3949555 = p["reaction_mwb205f533_4013_406b_8a4b_691ec3949555_mwa6ef5f75_f152_414d_811c_dd037d4b3ca1"] * u[65] * u[6] - p["reaction_mwb205f533_4013_406b_8a4b_691ec3949555_mwee51df1b_3f69_43f8_a1d5_5a8c5d0215f2"] * u[108]
reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d = p["reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d_mw2e0b4751_7227_4815_bf6f_fa5e2370b1d3"] * u[108] * u[60] - p["reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d_mwa8eec8e9_74b9_4afc_b6db_1116fe48e858"] * u[109]
reaction_mwfab3a9ec_b094_44f0_bd59_12ac56ca1c99 = p["reaction_mwfab3a9ec_b094_44f0_bd59_12ac56ca1c99_mwc3426c7e_3452_4507_9189_4b83ab147bdd"] * u[109]
reaction_mw4fceada8_6eb0_4230_a083_b2ab094d2961 = p["reaction_mw4fceada8_6eb0_4230_a083_b2ab094d2961_mw9cafad09_6002_46e1_8336_bb91c3716d70"] * u[73]
# Species: id = mwe2fff28d_182c_4a1c_9882_f17774c0958a; name = EGF; affected by kineticLaw
du[1] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12))
# Species: id = mw93907b2d_53db_4080_9e3f_3eb304441ab9; name = EGFR; affected by kineticLaw
du[2] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12) + (1.0 * reaction_mw47dee769_daa0_4af4_978a_5ab17e504c2f))
# Species: id = mw7eacabf9_d68c_491a_aba2_ec0809a8ecc8; name = EGF-EGFR; affected by kineticLaw
du[3] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwa67e40c1_693d_4214_adc8_b2f2b71cef12) + (-1.0 * reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d) + (-1.0 * reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d))
# Species: id = mwa8f2e7b2_0927_4ab4_a817_dddc43bb4fa3; name = EGF-EGFR2; affected by kineticLaw
du[4] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw877cd1e3_b48b_42e8_ab23_682dd893fd9d) + (-1.0 * reaction_mw413c6d45_ab23_4d3e_87b3_a8ed4629b923) + (1.0 * reaction_mw9544e67b_b6d0_4941_b7e0_ecd4f400a335) + (1.0 * reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6) + (1.0 * reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93) + (1.0 * reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a))
# Species: id = mwbfcf6773_1915_432c_b1d2_1f246094cc74; name = pEGF-EGFR2; affected by kineticLaw
du[5] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw413c6d45_ab23_4d3e_87b3_a8ed4629b923) + (-1.0 * reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04) + (-1.0 * reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644) + (1.0 * reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe) + (-1.0 * reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61) + (-1.0 * reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd) + (-1.0 * reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c) + (1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730) + (1.0 * reaction_mw4685274a_2b55_429f_927f_3fd863592af6) + (-1.0 * reaction_mw85e457d1_73f8_4236_bb61_a128d300003f) + (-1.0 * reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d) + (1.0 * reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8) + (-1.0 * reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01) + (1.0 * reaction_mw65b9e026_bc6c_4c94_8b37_8b9acdf50c8a) + (-1.0 * reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3))
# Species: id = mw19122f7d_f92e_4dc0_922f_6b681db65b0b; name = cbl; affected by kineticLaw
du[6] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b) + (1.0 * reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657) + (-1.0 * reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4) + (1.0 * reaction_mweb93165f_cf03_48f1_b035_59d79e324314) + (-1.0 * reaction_mw85e457d1_73f8_4236_bb61_a128d300003f) + (1.0 * reaction_mwc9b3b248_3290_452a_9b7c_8fdada3e6687) + (-1.0 * reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df) + (1.0 * reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19) + (-1.0 * reaction_mwb205f533_4013_406b_8a4b_691ec3949555))
# Species: id = mw3c2e1b43_29ca_491a_93e9_c723a993d6fb; name = Shc; affected by kineticLaw
du[7] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04) + (1.0 * reaction_mweda6a945_fb5d_4d99_9958_11b2b2840308) + (1.0 * reaction_mwd4bf58ea_70c9_43ea_a831_1fcde130ba28))
# Species: id = mw5198d3c2_879c_4f0d_b4f8_cd40efe0b1cf; name = pEGF-EGFR2-Shc; affected by kineticLaw
du[8] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf61e086d_0345_4d4c_b91d_0b105e543d04) + (-1.0 * reaction_mw974c39f5_b82e_44b3_abec_7a724f46c526))
# Species: id = mwe57c3282_5935_405c_8c0b_7fadb7a5de17; name = SHP; affected by kineticLaw
du[9] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644) + (1.0 * reaction_mw9544e67b_b6d0_4941_b7e0_ecd4f400a335) + (-1.0 * reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db) + (1.0 * reaction_mweda6a945_fb5d_4d99_9958_11b2b2840308) + (-1.0 * reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2) + (1.0 * reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6) + (-1.0 * reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb) + (1.0 * reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93) + (-1.0 * reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19) + (1.0 * reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a))
# Species: id = mw954e8fcb_ac0a_459d_8878_f19080208a17; name = pEGF-EGFR2-SHP2; affected by kineticLaw
du[10] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw91f49311_efdc_47c6_b8b8_a619e042d644) + (-1.0 * reaction_mw9544e67b_b6d0_4941_b7e0_ecd4f400a335))
# Species: id = mwa98802cb_c977_4fe0_9e67_5000904c2c36; name = pEGF-EGFR2-pShc; affected by kineticLaw
du[11] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw974c39f5_b82e_44b3_abec_7a724f46c526) + (-1.0 * reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe) + (-1.0 * reaction_mw4817365e_a33b_451f_bee1_de748377ede2) + (-1.0 * reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc))
# Species: id = mwa0349407_8187_48fc_9e94_5698ccc4e06d; name = pShc; affected by kineticLaw
du[12] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw486c5261_3d03_4589_a1e9_978b62ad2dfe) + (-1.0 * reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db) + (-1.0 * reaction_mwd4bf58ea_70c9_43ea_a831_1fcde130ba28) + (1.0 * reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6) + (1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730) + (1.0 * reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657))
# Species: id = mwf9999977_6f0e_4e35_9b73_75587f3448e9; name = pShc-SHP2; affected by kineticLaw
du[13] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw2cf8a809_63d8_4717_91fc_070516e6f3db) + (-1.0 * reaction_mweda6a945_fb5d_4d99_9958_11b2b2840308))
# Species: id = mwf430a579_ecbf_48ba_80c2_06e455808f2a; name = Grb2; affected by kineticLaw
du[14] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw4817365e_a33b_451f_bee1_de748377ede2) + (1.0 * reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6) + (-1.0 * reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a) + (-1.0 * reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61) + (1.0 * reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93) + (1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730) + (1.0 * reaction_mw4685274a_2b55_429f_927f_3fd863592af6))
# Species: id = mw504578d8_96c3_471f_8a7e_8c14e7535d3d; name = pEGF-EGFR2-pShc-Grb2; affected by kineticLaw
du[15] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw4817365e_a33b_451f_bee1_de748377ede2) + (-1.0 * reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2) + (-1.0 * reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3))
# Species: id = mw45ab688a_6467_4a3e_a779_2118fa84d69e; name = pEGF-EGFR2-pShc-Grb2-SHP2; affected by kineticLaw
du[16] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw03998474_934b_4e4a_8c0c_ca359e402ac2) + (-1.0 * reaction_mw7bb43f0a_c87e_41ff_8a43_cdf45c8f05e6))
# Species: id = mw9dcaa655_a755_426e_a3fa_1ad7c3c45575; name = SOS; affected by kineticLaw
du[17] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3) + (-1.0 * reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a) + (-1.0 * reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2) + (1.0 * reaction_mw8e331e43_16b4_478d_880b_d5a3244540e4))
# Species: id = mwfbda4e09_0cbb_49bc_ae69_f88b7a79ed21; name = pEGF-EGFR2-pShc-Grb2-SOS; affected by kineticLaw
du[18] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwd9262331_e35a_4614_943a_89bcf8a492e3) + (1.0 * reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc) + (-1.0 * reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e) + (1.0 * reaction_mw0bcfad86_59b9_42ff_bcb7_fbb44845049d) + (-1.0 * reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c) + (-1.0 * reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b))
# Species: id = mwb1bc2058_e6d8_4680_9e6c_d27bb366cde0; name = pEGF-EGFR2-pShc-Grb2-SOS-cbl; affected by kineticLaw
du[19] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwbd8a133e_1b70_44e8_bef8_78b14141166b) + (-1.0 * reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630))
# Species: id = mw1093b3af_1864_4ba3_a541_6009a9921282; name = Grb2-SOS; affected by kineticLaw
du[20] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwc5f121dc_d27d_4c3d_90f2_67d0adaf144a) + (-1.0 * reaction_mw23a29b42_9813_4e46_b8ae_966e3215e6dc) + (-1.0 * reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd) + (1.0 * reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657) + (1.0 * reaction_mweb93165f_cf03_48f1_b035_59d79e324314))
# Species: id = mwd9462e5b_a272_4b66_ab66_fde9266b1a43; name = pEGF-EGFR2-Grb2; affected by kineticLaw
du[21] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw0e459167_515b_4c4d_8b67_bf0a5b3e9d61) + (-1.0 * reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb) + (-1.0 * reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2))
# Species: id = mw925b938a_fe73_4664_ba6f_e72e57780891; name = pEGF-EGFR2-Grb2-SHP2; affected by kineticLaw
du[22] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwc52e0f9b_1e0c_46ca_8d18_f05ef4a080cb) + (-1.0 * reaction_mw4f89bf6c_8691_41a6_a1ac_13e6aa8c4b93))
# Species: id = mwf8cc7834_bf4f_4ccd_8235_d0890badf0f6; name = pEGF-EGFR2-Grb2-SOS; affected by kineticLaw
du[23] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw35f71989_f89b_4440_b1a4_ebc7b4cc18b2) + (1.0 * reaction_mwd0d92dd4_81b7_4385_bfd7_5de82e193ecd) + (1.0 * reaction_mwe9b50ac7_dac3_4eba_b1db_b3fd392d8fb7) + (-1.0 * reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f) + (-1.0 * reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30) + (-1.0 * reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4))
# Species: id = mw481cd12b_61ba_44e5_93bf_8b88c6c4a4e7; name = pEGF-EGFR2-Grb2-SOS-cbl; affected by kineticLaw
du[24] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw6bee0112_92dc_4169_9109_2633772b3aa4) + (-1.0 * reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014))
# Species: id = mw8f5a7b5c_ca4c_4a4c_85b1_e5d640c426bf; name = Ras-GDP; affected by kineticLaw
du[25] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e) + (1.0 * reaction_mw921ee820_1dbb_4b5f_866c_87da620d8f89) + (-1.0 * reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f) + (1.0 * reaction_mwf31259aa_32b7_4104_be70_045297b9a512) + (1.0 * reaction_mw652570eb_c9d3_499b_b877_61d360b10980))
# Species: id = mwf40d6176_abfc_4a30_886f_83a19fcffc48; name = pEGF-EGFR2-pShc-Grb2-SOS-Ras-GDP; affected by kineticLaw
du[26] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwbb77e3d6_6065_4344_9361_e30c03514f4e) + (-1.0 * reaction_mw0bcfad86_59b9_42ff_bcb7_fbb44845049d))
# Species: id = mwa54a9c38_c98b_45e5_8432_4119fb777e44; name = Ras-GTP; affected by kineticLaw
du[27] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw921ee820_1dbb_4b5f_866c_87da620d8f89) + (1.0 * reaction_mw0bcfad86_59b9_42ff_bcb7_fbb44845049d) + (1.0 * reaction_mwe9b50ac7_dac3_4eba_b1db_b3fd392d8fb7) + (-1.0 * reaction_mw3c617363_649b_4460_a694_36f7a3127a62) + (-1.0 * reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53) + (-1.0 * reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3) + (1.0 * reaction_mw42c97708_4f85_45a8_9141_d0ae529409ca))
# Species: id = mw28464aad_8013_4a23_ae09_a406954859a6; name = pEGF-EGFR2-Grb2-SOS-Ras-GDP; affected by kineticLaw
du[28] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwe9b50ac7_dac3_4eba_b1db_b3fd392d8fb7) + (1.0 * reaction_mw934c3638_603e_4ff0_a763_68f9405fa01f))
# Species: id = mw7cff9a0e_094d_498e_bf7f_7b162c61d63a; name = Ras-GAP; affected by kineticLaw
du[29] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw3c617363_649b_4460_a694_36f7a3127a62) + (1.0 * reaction_mwf31259aa_32b7_4104_be70_045297b9a512) + (-1.0 * reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c) + (1.0 * reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a))
# Species: id = mwdf82303e_323f_4c51_a858_56a59233cd98; name = Ras-GTP-Ras-GAP; affected by kineticLaw
du[30] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw3c617363_649b_4460_a694_36f7a3127a62) + (-1.0 * reaction_mwf31259aa_32b7_4104_be70_045297b9a512))
# Species: id = mwd39388fd_4f85_4d1c_b2a3_37857c595a2d; name = pEGF-EGFR2-Ras-GAP; affected by kineticLaw
du[31] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw0a51fbf0_409b_4b45_b4ac_0220af4c4e3c) + (-1.0 * reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53) + (1.0 * reaction_mw652570eb_c9d3_499b_b877_61d360b10980) + (-1.0 * reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19))
# Species: id = mwd7bf31ba_b05c_4c45_bb2f_6a2468a2a507; name = pEGF-EGFR2-Ras-GAP-Ras-GTP; affected by kineticLaw
du[32] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw33baddbd_a23f_45bb_b126_0ba60bbf6c53) + (-1.0 * reaction_mw652570eb_c9d3_499b_b877_61d360b10980))
# Species: id = mwbf5cb039_b830_4282_aa22_a3dda6272ec1; name = pEGF-EGFR2-Ras-GAP-SHP2; affected by kineticLaw
du[33] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwc5aae1f8_52e4_4bcd_b044_3768f90b7b19) + (-1.0 * reaction_mw642ac312_2ee7_4e66_8f3e_e2da2bb6412a))
# Species: id = mw66ac98c4_7e7b_4071_954d_43eb17584220; name = Raf1; affected by kineticLaw
du[34] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3) + (1.0 * reaction_mw87711dc1_43d7_40fc_b9e9_a24e2f92419d))
# Species: id = mw83de7813_4941_45a6_a320_a551165bf22a; name = Raf1-Ras-GTP; affected by kineticLaw
du[35] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw584a64d0_560a_4297_9882_80cb4eff73f3) + (-1.0 * reaction_mw42c97708_4f85_45a8_9141_d0ae529409ca))
# Species: id = mwaff92910_ed3d_40b9_a29c_e4866167e828; name = Raf1active; affected by kineticLaw
du[36] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw42c97708_4f85_45a8_9141_d0ae529409ca) + (-1.0 * reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33) + (1.0 * reaction_mw1bd186cf_4762_480a_b70d_d7a775462398) + (-1.0 * reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2) + (1.0 * reaction_mwb49058ff_2997_4187_abe7_4dce4ccf6ff4) + (-1.0 * reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d) + (-1.0 * reaction_mw950485f2_4463_4309_a4e4_cc81d16ffb7f) + (1.0 * reaction_mw62f71309_e066_47d2_9b99_01f78a51c218))
# Species: id = mw0834731b_0477_4217_a53b_30cef851191b; name = MEK; affected by kineticLaw
du[37] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33) + (1.0 * reaction_mwa4b69c77_6226_46da_b78c_3e6027d0be41))
# Species: id = mw4628f984_eb87_4922_9760_4975095ce6eb; name = Raf1active-MEK; affected by kineticLaw
du[38] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwaa65a34e_fabf_4d6d_ae0b_f1d08b068f33) + (-1.0 * reaction_mw1bd186cf_4762_480a_b70d_d7a775462398))
# Species: id = mw9b25f809_18a1_4c14_8f4b_cf18e6d93c28; name = pMEK; affected by kineticLaw
du[39] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw1bd186cf_4762_480a_b70d_d7a775462398) + (-1.0 * reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2) + (1.0 * reaction_mw40950d59_1012_4361_8418_73e25758e367) + (-1.0 * reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419))
# Species: id = mw12ba4000_d452_420c_be63_96d2848aca32; name = Raf1active-pMEK; affected by kineticLaw
du[40] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf5573ddf_ad7f_478a_a784_557a9cddaaf2) + (-1.0 * reaction_mwb49058ff_2997_4187_abe7_4dce4ccf6ff4))
# Species: id = mwf816df4c_4593_4d23_990f_0d7c15ddde5d; name = ppMEK; affected by kineticLaw
du[41] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwb49058ff_2997_4187_abe7_4dce4ccf6ff4) + (-1.0 * reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe) + (1.0 * reaction_mwf95f743d_6108_49fe_8ffd_bdcc1a9f9a8d) + (-1.0 * reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9) + (1.0 * reaction_mw6fd24d16_f57d_46c6_82f5_3f00759fa16b) + (-1.0 * reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b))
# Species: id = mw7e23b961_186b_47a0_a8b5_5e9957766792; name = ERK; affected by kineticLaw
du[42] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe) + (1.0 * reaction_mwcc31b497_6c50_446c_bbc2_6c5739507252))
# Species: id = mwcedf8ecd_67bd_4b91_aa04_d58782dec2a4; name = ppMEK-ERK; affected by kineticLaw
du[43] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw8301b154_9463_4516_b4c5_c8f8b68691fe) + (-1.0 * reaction_mwf95f743d_6108_49fe_8ffd_bdcc1a9f9a8d))
# Species: id = mwcc894c94_0ddf_42cc_913e_cdcc4d471d94; name = pERK; affected by kineticLaw
du[44] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf95f743d_6108_49fe_8ffd_bdcc1a9f9a8d) + (-1.0 * reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9) + (1.0 * reaction_mw61305f93_7b2d_4a2d_8d16_f7be026d8671) + (-1.0 * reaction_mw1d8c2435_bb85_4352_a25f_82033250579e))
# Species: id = mw6cb74b27_ffef_49bb_8ffb_622d552caa9e; name = ppMEK-pERK; affected by kineticLaw
du[45] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw51d9d6b8_f0c0_4763_9d11_9be61b5cf5c9) + (-1.0 * reaction_mw6fd24d16_f57d_46c6_82f5_3f00759fa16b))
# Species: id = mwd784228d_0cb5_468a_ac70_02d8f04b3d9c; name = ppERK; affected by kineticLaw
du[46] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw6fd24d16_f57d_46c6_82f5_3f00759fa16b) + (-1.0 * reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48) + (-1.0 * reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c) + (1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730) + (-1.0 * reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30) + (1.0 * reaction_mw4685274a_2b55_429f_927f_3fd863592af6))
# Species: id = mwbaaeb210_4806_4076_9d60_219f4ed945b6; name = Pase; affected by kineticLaw
du[47] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d) + (1.0 * reaction_mw87711dc1_43d7_40fc_b9e9_a24e2f92419d))
# Species: id = mw19a33ad5_5ba4_46c7_84eb_c1287f02bcd5; name = Raf1active-Pase; affected by kineticLaw
du[48] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw9c208e18_c70d_4231_af0b_ad17cd0bba2d) + (-1.0 * reaction_mw87711dc1_43d7_40fc_b9e9_a24e2f92419d))
# Species: id = mwf9e2a044_7774_400b_a74e_a111b4a21f30; name = Pase2; affected by kineticLaw
du[49] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b) + (1.0 * reaction_mw40950d59_1012_4361_8418_73e25758e367) + (-1.0 * reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419) + (1.0 * reaction_mwa4b69c77_6226_46da_b78c_3e6027d0be41))
# Species: id = mwcb572fe2_c3ac_40e7_8141_da7d55fce18a; name = ppMEK-Pase2; affected by kineticLaw
du[50] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw4b445876_bdce_42d0_867b_fd3c74128a6b) + (-1.0 * reaction_mw40950d59_1012_4361_8418_73e25758e367))
# Species: id = mwa0acc0ac_5fac_4a42_a3be_e36db44994b0; name = pMEK-Pase2; affected by kineticLaw
du[51] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwbfa79c95_487d_4c6f_b437_9e579451a419) + (-1.0 * reaction_mwa4b69c77_6226_46da_b78c_3e6027d0be41))
# Species: id = mwd087f76b_65dc_47f1_ba21_c43774457686; name = Pase3; affected by kineticLaw
du[52] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48) + (1.0 * reaction_mw61305f93_7b2d_4a2d_8d16_f7be026d8671) + (1.0 * reaction_mwcc31b497_6c50_446c_bbc2_6c5739507252) + (-1.0 * reaction_mw1d8c2435_bb85_4352_a25f_82033250579e))
# Species: id = mw35f5adaa_d1c0_433c_817d_76e317f4cb15; name = pERK-Pase3; affected by kineticLaw
du[53] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwcc31b497_6c50_446c_bbc2_6c5739507252) + (1.0 * reaction_mw1d8c2435_bb85_4352_a25f_82033250579e))
# Species: id = mwa7e3103a_6394_472c_b0f4_8ed527f68604; name = ppERK-Pase3; affected by kineticLaw
du[54] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf8bb22e2_5aa3_4c25_a022_a266b1856a48) + (-1.0 * reaction_mw61305f93_7b2d_4a2d_8d16_f7be026d8671))
# Species: id = mw5babe3d5_a9af_4dfd_ac01_35474ef64af2; name = ppERK-pEGF-EGFR2-pShc-Grb2-SOS; affected by kineticLaw
du[55] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw8dec1159_1925_45d9_af25_3cb709a5017c) + (-1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730))
# Species: id = mw31ac308f_da36_4f73_830f_67f3e5b945d9; name = pSOS; affected by kineticLaw
du[56] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwcf9f1b1d_e19a_4fa8_85ba_8f17e2cec730) + (1.0 * reaction_mw4685274a_2b55_429f_927f_3fd863592af6) + (-1.0 * reaction_mw8e331e43_16b4_478d_880b_d5a3244540e4))
# Species: id = mw31261227_9cd6_4059_a0bb_04dbf4888080; name = ppERK-pEGF-EGFR2-Grb2-SOS; affected by kineticLaw
du[57] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwa5c135b4_77e2_4411_98e1_2000c39d4b30) + (-1.0 * reaction_mw4685274a_2b55_429f_927f_3fd863592af6))
# Species: id = mw0a0ca6ba_cb28_44c7_a0c0_1593cb720966; name = ProEGFR; affected by kineticLaw
du[58] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw47dee769_daa0_4af4_978a_5ab17e504c2f))
# Species: id = mw06b8aada_c92a_48eb_8ee7_af3778cfe62f; name = pEGF-EGFR2-pShc-Grb2-SOS-cbl-EPn; affected by kineticLaw
du[59] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630) + (-1.0 * reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657))
# Species: id = mwb2366216_0b3c_4f28_8303_fec92c68dd57; name = EPn; affected by kineticLaw
du[60] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw3a87ca5a_845d_4ac4_8806_e343cbbfc630) + (1.0 * reaction_mw363a5271_1f51_4d5e_87a7_42ea25cb5657) + (-1.0 * reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014) + (1.0 * reaction_mweb93165f_cf03_48f1_b035_59d79e324314) + (-1.0 * reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6) + (1.0 * reaction_mwc9b3b248_3290_452a_9b7c_8fdada3e6687) + (-1.0 * reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e) + (1.0 * reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19) + (-1.0 * reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d) + (1.0 * reaction_mwfab3a9ec_b094_44f0_bd59_12ac56ca1c99))
# Species: id = mw1d5948e7_5504_4224_9d71_227911b4f1ee; name = pEGF-EGFR2-Grb2-SOS-cbl-EPn; affected by kineticLaw
du[61] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwbac9e6ff_2df1_45eb_b3f4_4cae74c64014) + (-1.0 * reaction_mweb93165f_cf03_48f1_b035_59d79e324314))
# Species: id = mwec1b368b_8f73_47eb_9636_9956389836eb; name = pEGF-EGFR2-cbl; affected by kineticLaw
du[62] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw85e457d1_73f8_4236_bb61_a128d300003f) + (-1.0 * reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6))
# Species: id = mwa455ec7e_1a12_4659_95a2_a5695d09ca60; name = pEGF-EGFR2-cbl-EPn; affected by kineticLaw
du[63] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw6b159c8f_eee0_4337_b711_2e230c9e2cf6) + (-1.0 * reaction_mwc9b3b248_3290_452a_9b7c_8fdada3e6687))
# Species: id = mw2ba1db9a_4483_44fa_a3a2_b4a5ea66898c; name = PI3K; affected by kineticLaw
du[64] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d) + (1.0 * reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621) + (1.0 * reaction_mwfab3a9ec_b094_44f0_bd59_12ac56ca1c99))
# Species: id = mw0dc4e5eb_4366_4799_bebc_cfcffe5c06f5; name = pEGF-EGFR2-PI3K; affected by kineticLaw
du[65] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw77484632_4e33_468a_9937_24e9bfd0e17d) + (-1.0 * reaction_mw2c5858f3_0988_49b0_a94a_057853b84e91) + (-1.0 * reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8) + (-1.0 * reaction_mwb205f533_4013_406b_8a4b_691ec3949555))
# Species: id = mw1e591998_65c0_484e_8a3b_537a38d94de1; name = pEGF-EGFR2-pPI3K; affected by kineticLaw
du[66] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw2c5858f3_0988_49b0_a94a_057853b84e91))
# Species: id = mw78e207c4_4faf_4b48_8e22_1ee666e9cc4c; name = pPI3K; affected by kineticLaw
du[67] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwd3a36af9_3ccc_4bb1_9867_3b9823ba4ac8) + (-1.0 * reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31) + (-1.0 * reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0) + (1.0 * reaction_mw5dcc8719_3180_4bd0_8797_08e256131961))
# Species: id = mwfc4a9c3d_3ebb_4033_8b7d_f4d7613d2078; name = TP4; affected by kineticLaw
du[68] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31) + (1.0 * reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621))
# Species: id = mwbd6bb050_89bd_41df_8cea_d2e1fb77bafe; name = TP4-pPI3K; affected by kineticLaw
du[69] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw9f000f29_2512_4d4a_9dd9_e59aaf296d31) + (-1.0 * reaction_mw837b5ad7_4a8c_4c55_94ff_0fdd63048044))
# Species: id = mw7033dfd6_53c5_433b_a132_f8cb34dea20f; name = TP4-PI3K; affected by kineticLaw
du[70] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw837b5ad7_4a8c_4c55_94ff_0fdd63048044) + (-1.0 * reaction_mwd15926b3_069a_4b16_a6fc_c0c15083d621))
# Species: id = mwb561d9f3_a9ed_4bdb_8d40_87be5cc3237a; name = PIP2; affected by kineticLaw
du[71] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0) + (1.0 * reaction_mw4fceada8_6eb0_4230_a083_b2ab094d2961))
# Species: id = mw014cc419_b720_4b90_9192_2ec6e706c87d; name = pPI3K-PIP2; affected by kineticLaw
du[72] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw3a5e0932_d50f_4fe6_b8cb_0ad649f305b0) + (-1.0 * reaction_mw5dcc8719_3180_4bd0_8797_08e256131961))
# Species: id = mwd7f41594_8377_4e2e_9528_45d5a82ffdb4; name = PIP3; affected by kineticLaw
du[73] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw5dcc8719_3180_4bd0_8797_08e256131961) + (-1.0 * reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b) + (1.0 * reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6) + (-1.0 * reaction_mw4fceada8_6eb0_4230_a083_b2ab094d2961))
# Species: id = mwcef73e0e_d195_4077_ae71_723664ee1602; name = Akt; affected by kineticLaw
du[74] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b) + (1.0 * reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92))
# Species: id = mw62bf5275_ce02_4e86_b3b6_3f87a335e1de; name = Aktm; affected by kineticLaw
du[75] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw376b0685_ef73_4fcc_94af_2ada24cf8a8b) + (-1.0 * reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef) + (1.0 * reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e))
# Species: id = mw6e01967b_3e2a_433d_bec6_9f9cf3ba243c; name = PDK1; affected by kineticLaw
du[76] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef) + (1.0 * reaction_mw31369230_1f14_45bd_be02_a44a275c6e31))
# Species: id = mw6353aa36_d4a4_4254_8a1f_1f7f571d4233; name = Aktm-PDK1; affected by kineticLaw
du[77] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwcc7cfa9c_4945_403a_938e_b237c371a5ef) + (-1.0 * reaction_mw98da32e0_b061_40c5_9d32_40744134f3fa) + (1.0 * reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174))
# Species: id = mwc1935afc_56b1_4a87_923c_ae6d82455d80; name = pAktm-PDK1; affected by kineticLaw
du[78] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw98da32e0_b061_40c5_9d32_40744134f3fa) + (-1.0 * reaction_mw31369230_1f14_45bd_be02_a44a275c6e31) + (-1.0 * reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5))
# Species: id = mw3d81860d_d786_4fcc_b8bb_64f1a2d7739d; name = pAktm; affected by kineticLaw
du[79] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw31369230_1f14_45bd_be02_a44a275c6e31) + (-1.0 * reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6) + (-1.0 * reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371))
# Species: id = mw16796ffe_4764_4a9f_942e_149f42c1cd28; name = pAkt; affected by kineticLaw
du[80] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw12311a84_3f8d_40c6_8b14_961a8a58d1b6) + (-1.0 * reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9))
# Species: id = mwa6e82fc9_a0ce_461c_93c8_17f3c807c1a1; name = pAkt-Takt; affected by kineticLaw
du[81] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwf3d393e9_ae09_4eab_a39a_ed0eef0f54bc) + (1.0 * reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9))
# Species: id = mw236a3250_4c96_4f6e_b94c_ab3d12852801; name = Akt-Takt; affected by kineticLaw
du[82] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf3d393e9_ae09_4eab_a39a_ed0eef0f54bc) + (-1.0 * reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92))
# Species: id = mw11a8b702_b8ac_4513_b4aa_063e51089812; name = Takt; affected by kineticLaw
du[83] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw2698f402_d00b_451e_8b22_93a322fe9a92) + (-1.0 * reaction_mw028e8b3e_b531_4466_9c3a_e3fcf7fc9be9) + (-1.0 * reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371) + (1.0 * reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e) + (-1.0 * reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5) + (1.0 * reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174))
# Species: id = mw1a0cb97a_b657_430b_963c_92217f643081; name = pAktm-Takt; affected by kineticLaw
du[84] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwc5e0c166_6a3a_4913_9ed1_dafe97bdb371) + (-1.0 * reaction_mw94b3bae0_4da9_4358_a5ac_a46a5cbf621b))
# Species: id = mw9b937ca3_0d82_46d5_8f5a_0f9701002797; name = Aktm-Takt; affected by kineticLaw
du[85] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw94b3bae0_4da9_4358_a5ac_a46a5cbf621b) + (-1.0 * reaction_mw362ca1b3_224a_42fb_a14b_6ff467748a5e))
# Species: id = mw57a44eb0_ace7_4294_905a_219e87d3c281; name = pAktm-PDK1-Takt; affected by kineticLaw
du[86] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw3994e898_7232_4b70_9c58_b3476e8655f5) + (-1.0 * reaction_mw75acd2d1_3fdf_4c3f_8d99_6d62f825d5e2))
# Species: id = mwd746a5d5_5e65_4a4c_9f84_0e4a3cb7d2fc; name = Aktm-PDK1-Takt; affected by kineticLaw
du[87] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw75acd2d1_3fdf_4c3f_8d99_6d62f825d5e2) + (-1.0 * reaction_mw4a334f7d_9bce_4690_b623_a427ed66a174))
# Species: id = mwa6994523_5d45_4000_af0c_3e94073bf183, name = pAkt_total, defined in a rule du[88] = u[88]
# Species: id = mwdf92bdc0_f426_45b0_9ad0_876521f41312; name = pRaf1active; affected by kineticLaw
du[89] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw950485f2_4463_4309_a4e4_cc81d16ffb7f) + (-1.0 * reaction_mw62f71309_e066_47d2_9b99_01f78a51c218))
# Species: id = mw13abe2a6_9905_40e5_8c23_3fc8834b572a; name = STAT3c; affected by kineticLaw
du[90] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01) + (1.0 * reaction_mwe9988e4a_083c_4f8e_b154_3e599c9307b0) + (-1.0 * reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2) + (1.0 * reaction_mwd189238c_e8f9_40be_b4ea_18a42bba1b4f) + (1.0 * reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19))
# Species: id = mw2fd710a6_7fe2_4484_bca6_59c187bade8b; name = pEGF-EGFR2-STAT3c; affected by kineticLaw
du[91] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwe8647e48_f4a9_40f4_9b32_f89ded572e01) + (-1.0 * reaction_mw65b9e026_bc6c_4c94_8b37_8b9acdf50c8a) + (-1.0 * reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df))
# Species: id = mwb6a9aa2c_62e7_410f_9c33_dbe36dfcc4af; name = pSTAT3c; affected by kineticLaw
du[92] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw65b9e026_bc6c_4c94_8b37_8b9acdf50c8a) + (-1.0 * reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3) + (-1.0 * reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618) + (-1.0 * reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735) + (-1.0 * reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735) + (-1.0 * reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2))
# Species: id = mw341082a0_8017_4cc7_9d00_b1211a196072; name = pEGF-EGFR2-pSTAT3c; affected by kineticLaw
du[93] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw1c9d29fa_bff4_4d2f_9d5f_f1791e4882a3))
# Species: id = mwcea1f1c1_2f85_4af1_98ea_ef14cf580c09; name = PP1; affected by kineticLaw
du[94] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618) + (1.0 * reaction_mwe9988e4a_083c_4f8e_b154_3e599c9307b0) + (-1.0 * reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2) + (1.0 * reaction_mw75c6078f_fb76_4ca9_9fdd_e221e3ba57ad))
# Species: id = mwdc34472c_a6f9_4002_951d_e0e8da64eb42; name = pSTAT3c-PP1; affected by kineticLaw
du[95] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwad97bd5a_3dae_49d9_990b_2e6574740618) + (-1.0 * reaction_mwe9988e4a_083c_4f8e_b154_3e599c9307b0))
# Species: id = mw472d5cb9_120e_4f60_bbae_1ae2552837dd; name = pSTAT3c-pSTAT3c-PP1; affected by kineticLaw
du[96] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2) + (-1.0 * reaction_mw75c6078f_fb76_4ca9_9fdd_e221e3ba57ad))
# Species: id = mw4f575c55_7dff_45d7_94ad_cda9621d5b63; name = pSTAT3c-pSTAT3c; affected by kineticLaw
du[97] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwf8bacf1a_6c1a_49b6_b344_2d3bd404a735) + (-1.0 * reaction_mwc9b945cf_3a14_4bd9_b253_7064498c75e2) + (-1.0 * reaction_mwec4127b5_6bcf_4128_aff4_a6b3c470f690))
# Species: id = mwd2c465fb_eea7_499a_8ea4_f318a64cb9ee; name = STAT3c-pSTAT3c; affected by kineticLaw
du[98] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw75c6078f_fb76_4ca9_9fdd_e221e3ba57ad) + (1.0 * reaction_mw177fa7b0_f0be_4c3e_8b47_2ac4e13159a2))
# Species: id = mw4110f531_7513_4786_8896_7c9d969ff558; name = pSTAT3n-pSTAT3n; affected by kineticLaw
du[99] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwec4127b5_6bcf_4128_aff4_a6b3c470f690) + (1.0 * reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a) + (-1.0 * reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7))
# Species: id = mwe3fd7f65_b0d1_44d9_b6f3_d2f7d332f664; name = pSTAT3n; affected by kineticLaw
du[100] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a) + (-1.0 * reaction_mw5c806b00_59a1_491e_99a1_2c932b2d5d7a) + (-1.0 * reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7) + (-1.0 * reaction_mw45d92b79_0656_4795_87d0_7a465949ca43))
# Species: id = mw0e1be972_fded_4bff_a93d_091ec942485f; name = PP2; affected by kineticLaw
du[101] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7) + (1.0 * reaction_mw3b0c171c_6d60_41ca_8193_83cd5e6c188c) + (-1.0 * reaction_mw45d92b79_0656_4795_87d0_7a465949ca43) + (1.0 * reaction_mwb71945c2_03a8_4fad_a995_e1caeee98525))
# Species: id = mw0facb8f2_95cf_4ddf_a959_b24ba64f320b; name = pSTAT3n-pSTAT3n-PP2; affected by kineticLaw
du[102] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw26fdabae_323b_4a78_b134_4c2eb70ea6a7) + (-1.0 * reaction_mw3b0c171c_6d60_41ca_8193_83cd5e6c188c))
# Species: id = mw9686f53e_d343_45fd_b441_9c992219546a; name = STAT3n-pSTAT3n; affected by kineticLaw
du[103] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw3b0c171c_6d60_41ca_8193_83cd5e6c188c) + (1.0 * reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7))
# Species: id = mw960bddeb_e567_46dd_b2f3_ed5e6a5c7972; name = STAT3n; affected by kineticLaw
du[104] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((-1.0 * reaction_mwc38a99c8_74cf_49f2_a16b_f6610ca1a0a7) + (1.0 * reaction_mwb71945c2_03a8_4fad_a995_e1caeee98525) + (-1.0 * reaction_mwd189238c_e8f9_40be_b4ea_18a42bba1b4f))
# Species: id = mw8c85ff7f_6368_4b11_a2ed_ce83481b55e6; name = pSTAT3n-PP2; affected by kineticLaw
du[105] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw45d92b79_0656_4795_87d0_7a465949ca43) + (-1.0 * reaction_mwb71945c2_03a8_4fad_a995_e1caeee98525))
# Species: id = mw548c81c2_c626_4df8_9177_a1a6fc3d4ce8; name = pEGF-EGFR2-STAT3c-cbl; affected by kineticLaw
du[106] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwcb637bf1_7618_4d8a_ab5c_399145ecf1df) + (-1.0 * reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e))
# Species: id = mw142e6dc4_ec15_459d_a184_6b20be04f08d; name = pEGF-EGFR2-STAT3c-cbl-EPn; affected by kineticLaw
du[107] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw401dde7e_c0a1_4780_b6cc_8f98681c862e) + (-1.0 * reaction_mw0dd5a91d_d76c_494e_9dd6_57f2836aaa19))
# Species: id = mw2c47ae3f_06d9_40ec_a252_535db0ae5caa; name = pEGF-EGFR2-PI3K-cbl; affected by kineticLaw
du[108] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mwb205f533_4013_406b_8a4b_691ec3949555) + (-1.0 * reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d))
# Species: id = mwd32d108b_49c2_4df2_9b67_d6c6b84f54b9; name = pEGF-EGFR2-PI3K-cbl-EPn; affected by kineticLaw
du[109] = (1 / (p["compartment_mw1637dd35_5f09_4a8d_bb7f_58717cdf1612"])) * ((1.0 * reaction_mw602726ea_89ee_41b8_bda6_e2811bb42c1d) + (-1.0 * reaction_mwfab3a9ec_b094_44f0_bd59_12ac56ca1c99))
end
u0=zeros(109)
u0[1] = 0.0081967
u0[2] = 0.3
u0[3] = 0.0
u0[4] = 0.0
u0[5] = 0.0
u0[6] = 0.8
u0[7] = 1.0
u0[8] = 0.0
u0[9] = 0.1
u0[10] = 0.0
u0[11] = 0.0
u0[12] = 0.0
u0[13] = 0.0
u0[14] = 1.0
u0[15] = 0.0
u0[16] = 0.0
u0[17] = 0.3
u0[18] = 0.0
u0[19] = 0.0
u0[20] = 0.0
u0[21] = 0.0
u0[22] = 0.0
u0[23] = 0.0
u0[24] = 0.0
u0[25] = 0.15
u0[26] = 0.0
u0[27] = 0.0
u0[28] = 0.0
u0[29] = 0.1
u0[30] = 0.0
u0[31] = 0.0
u0[32] = 0.0
u0[33] = 0.0
u0[34] = 0.5
u0[35] = 0.0
u0[36] = 0.0
u0[37] = 0.68
u0[38] = 0.0
u0[39] = 0.0
u0[40] = 0.0
u0[41] = 0.0
u0[42] = 0.4
u0[43] = 0.0
u0[44] = 0.0
u0[45] = 0.0
u0[46] = 0.0
u0[47] = 0.5
u0[48] = 0.0
u0[49] = 0.02
u0[50] = 0.0
u0[51] = 0.0
u0[52] = 0.002
u0[53] = 0.0
u0[54] = 0.0
u0[55] = 0.0
u0[56] = 0.0
u0[57] = 0.0
u0[58] = 1.0
u0[59] = 0.0
u0[60] = 0.5
u0[61] = 0.0
u0[62] = 0.0
u0[63] = 0.0
u0[64] = 0.2
u0[65] = 0.0
u0[66] = 0.0
u0[67] = 0.0
u0[68] = 0.2
u0[69] = 0.0
u0[70] = 0.0
u0[71] = 0.5
u0[72] = 0.0
u0[73] = 0.0
u0[74] = 0.1
u0[75] = 0.0
u0[76] = 0.1
u0[77] = 0.0
u0[78] = 0.0
u0[79] = 0.0
u0[80] = 0.0
u0[81] = 0.0
u0[82] = 0.0
u0[83] = 0.1
u0[84] = 0.0
u0[85] = 0.0
u0[86] = 0.0
u0[87] = 0.0
u0[88] = 0.0
u0[89] = 0.0
u0[90] = 1.0
u0[91] = 0.0
u0[92] = 0.0
u0[93] = 0.0
u0[94] = 0.5
u0[95] = 0.0
u0[96] = 0.0
u0[97] = 0.0
u0[98] = 0.0
u0[99] = 0.0
u0[100] = 0.0
u0[101] = 0.6
u0[102] = 0.0
u0[103] = 0.0
u0[104] = 0.0
u0[105] = 0.0
u0[106] = 0.0
u0[107] = 0.0
u0[108] = 0.0
u0[109] = 0.0
tspan = (0.0,100.0)
prob = ODEProblem{true,SciMLBase.FullSpecialize}(sbml_model!, u0, tspan, par)
sys = modelingtoolkitize(prob)
sys = structural_simplify(sys)
const to = TimerOutput()
@timeit to "ODEProb No Jac" oprob = ODEProblem{true,SciMLBase.FullSpecialize}(sys, Float64[], tspan, Float64[])
@timeit to "ODEProb DenseJac" densejacprob = ODEProblem{true,SciMLBase.FullSpecialize}(sys, Float64[], tspan, Float64[], jac=true)
```
```julia
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true,SciMLBase.FullSpecialize}(sys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
```
```julia
@show numspecies(sys) # Number of ODEs
@show numreactions(sys) # Apprx. number of terms in the ODE
@show length(parameters(sys)) # Number of Parameters
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF())
plot(sol, legend=false, fmt=:png)
```
For these benchmarks we will be using the time-series error with these saving
points since the final time point is not well-indicative of the solution
behavior (capturing the oscillation is the key!).
## Generate Test Solution
```julia
@time sol = solve(oprob, CVODE_BDF(), abstol=1/10^14, reltol=1/10^14)
test_sol = TestSolution(sol)
```
## Setups
```julia
abstols = 1.0 ./ 10.0 .^ (9:13)
reltols = 1.0 ./ 10.0 .^ (6:10)
setups = [
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rodas4()),
Dict(:alg=>QNDF()),
Dict(:alg=>lsoda()),
Dict(:alg=>radau()),
Dict(:alg=>seulex()),
Dict(:alg=>ImplicitEulerExtrapolation(min_order = 8, init_order = 9,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitEulerExtrapolation(min_order = 8, init_order = 9,threading = false)),
Dict(:alg=>ImplicitEulerBarycentricExtrapolation(min_order = 7, init_order = 8,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitEulerBarycentricExtrapolation(min_order = 7, init_order = 8,threading = false)),
Dict(:alg=>ImplicitHairerWannerExtrapolation(init_order = 5,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitHairerWannerExtrapolation(init_order = 5, threading = false)),
]
solnames = ["CVODE_BDF","KenCarp4","Rodas4","QNDF","lsoda","radau","seulex","ImplEulerExtpl (threaded)", "ImplEulerExtpl (non-threaded)",
"ImplEulerBaryExtpl (threaded)","ImplEulerBaryExtpl (non-threaded)","ImplHWExtpl (threaded)","ImplHWExtpl (non-threaded)"]
```
## Automatic Jacobian Solves
First we test using auto-generated Jacobians (finite difference)
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;
names = solnames,appxsol=test_sol,save_everystep=false,maxiters=Int(1e5),numruns=10)
plot(wp, title = "Implicit Methods: SBML Model",legend=:outertopleft,size = (1000,500),
xticks = 10.0 .^ (-15:1:1),
yticks = 10.0 .^ (-6:0.3:5),
bottom_margin= 5Plots.mm)
```
## Analytical Jacobian
Now we test using the generated analytic Jacobian function.
```julia
setups = [
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rodas4()),
Dict(:alg=>QNDF()),
#Dict(:alg=>lsoda()),
#Dict(:alg=>radau()),
#Dict(:alg=>seulex()),
Dict(:alg=>ImplicitEulerExtrapolation(min_order = 8, init_order = 9,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitEulerExtrapolation(min_order = 8, init_order = 9,threading = false)),
Dict(:alg=>ImplicitEulerBarycentricExtrapolation(min_order = 7, init_order = 8,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitEulerBarycentricExtrapolation(min_order = 7, init_order = 8,threading = false)),
Dict(:alg=>ImplicitHairerWannerExtrapolation(init_order = 5,threading = OrdinaryDiffEq.PolyesterThreads())),
Dict(:alg=>ImplicitHairerWannerExtrapolation(init_order = 5, threading = false)),
]
solnames = ["CVODE_BDF","KenCarp4","Rodas4","QNDF","ImplEulerExtpl (threaded)", "ImplEulerExtpl (non-threaded)",
"ImplEulerBaryExtpl (threaded)","ImplEulerBaryExtpl (non-threaded)","ImplHWExtpl (threaded)","ImplHWExtpl (non-threaded)"]
wp = WorkPrecisionSet(densejacprob,abstols,reltols,setups;
names = solnames,appxsol=test_sol,save_everystep=false,maxiters=Int(1e5),numruns=10)
plot(wp, title = "Implicit Methods: SBML Model",legend=:outertopleft,size = (1000,500),
xticks = 10.0 .^ (-15:1:1),
yticks = 10.0 .^ (-6:0.3:5),
bottom_margin= 5Plots.mm)
```
## Sparse Jacobian
Finally we test using the generated sparse analytic Jacobian function.
Note that the extrapolation methods currently do not support sparse Jacobians.
```julia
setups = [
#Dict(:alg=>CVODE_BDF()), #Fails!
Dict(:alg=>KenCarp4(autodiff = false)),
Dict(:alg=>Rodas4(autodiff = false)),
Dict(:alg=>QNDF(autodiff = false)),
#Dict(:alg=>lsoda()),
#Dict(:alg=>radau()),
#Dict(:alg=>seulex()),
#Dict(:alg=>ImplicitEulerExtrapolation(autodiff = false,min_order = 8, init_order = 9,threading = OrdinaryDiffEq.PolyesterThreads())),
#Dict(:alg=>ImplicitEulerExtrapolation(autodiff = false,min_order = 8, init_order = 9,threading = false)),
#Dict(:alg=>ImplicitEulerBarycentricExtrapolation(autodiff = false,min_order = 7, init_order = 8,threading = OrdinaryDiffEq.PolyesterThreads())),
#Dict(:alg=>ImplicitEulerBarycentricExtrapolation(autodiff = false,min_order = 7, init_order = 8,threading = false)),
#Dict(:alg=>ImplicitHairerWannerExtrapolation(autodiff = false,init_order = 5,threading = OrdinaryDiffEq.PolyesterThreads())),
#Dict(:alg=>ImplicitHairerWannerExtrapolation(autodiff = false,init_order = 5, threading = false)),
]
solnames = ["KenCarp4","Rodas4","QNDF",#"ImplEulerExtpl (threaded)", "ImplEulerExtpl (non-threaded)",
#"ImplEulerBaryExtpl (threaded)","ImplEulerBaryExtpl (non-threaded)","ImplHWExtpl (threaded)","ImplHWExtpl (non-threaded)"
]
wp = WorkPrecisionSet(sparsejacprob ,abstols,reltols,setups;
names = solnames,appxsol=test_sol,save_everystep=false,maxiters=Int(1e5),numruns=10)
plot(wp, title = "Implicit Methods: SBML Model",legend=:outertopleft,size = (1000,500),
xticks = 10.0 .^ (-15:1:1),
yticks = 10.0 .^ (-6:0.3:5),
bottom_margin= 5Plots.mm)
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 10474 | ---
title: Egfr_net Work-Precision Diagrams
author: Torkel Loman
---
The following benchmark is of 356 ODEs with 3749 terms that describe a
chemical reaction network. This egfr_net model was used as a benchmark model in [Gupta et
al.](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC6013266/). It describes the epidermal growth factor receptor signalling system [Blinov et
al.](https://pubmed.ncbi.nlm.nih.gov/16233948/). We use
[`ReactionNetworkImporters`](https://github.com/isaacsas/ReactionNetworkImporters.jl)
to load the BioNetGen model files as a
[Catalyst](https://github.com/SciML/Catalyst.jl) model, and then use
[ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl) to convert the
Catalyst network model to ODEs.
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools,
LinearSolve
gr()
const to = TimerOutput()
tf = 10.0
# generate ModelingToolkit ODEs
@timeit to "Parse Network" prnbng = loadrxnetwork(BNGNetwork(), joinpath(@__DIR__, "Models/egfr_net.net"))
show(to)
rn = prnbng.rn
obs = [eq.lhs for eq in observed(rn)]
@timeit to "Create ODESys" osys = convert(ODESystem, rn)
show(to)
tspan = (0.,tf)
@timeit to "ODEProb No Jac" oprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[])
show(to);
```
```julia
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
```
```julia
@show numspecies(rn) # Number of ODEs
@show numreactions(rn) # Apprx. number of terms in the ODE
@show length(parameters(rn)); # Number of Parameters
```
## Time ODE derivative function compilation
As compiling the ODE derivative functions has in the past taken longer than
running a simulation, we first force compilation by evaluating these functions
one time.
```julia
u = ModelingToolkit.varmap_to_vars(nothing, species(rn); defaults=ModelingToolkit.defaults(rn))
du = copy(u)
p = ModelingToolkit.varmap_to_vars(nothing, parameters(rn); defaults=ModelingToolkit.defaults(rn))
@timeit to "ODE rhs Eval1" oprob.f(du,u,p,0.)
@timeit to "ODE rhs Eval2" oprob.f(du,u,p,0.)
sparsejacprob.f(du,u,p,0.)
```
We also time the ODE rhs function with BenchmarkTools as it is more accurate
given how fast evaluating `f` is:
```julia
@btime oprob.f($du,$u,$p,0.)
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF(), saveat=tf/1000., reltol=1e-5, abstol=1e-5)
plot(sol; idxs=obs, legend=false, fmt=:png)
```
For these benchmarks we will be using the time-series error with these saving
points.
## Generate Test Solution
```julia
@time sol = solve(oprob, CVODE_BDF(), abstol=1/10^14, reltol=1/10^14)
test_sol = TestSolution(sol);
```
## Setups
#### Sets plotting defaults
```julia
default(legendfontsize=7,framestyle=:box,gridalpha=0.3,gridlinewidth=2.5)
```
#### Declares a plotting helper function
```julia
function plot_settings(wp)
times = vcat(map(wp -> wp.times, wp.wps)...)
errors = vcat(map(wp -> wp.errors, wp.wps)...)
xlimit = 10 .^ (floor(log10(minimum(errors))), ceil(log10(maximum(errors))))
ylimit = 10 .^ (floor(log10(minimum(times))), ceil(log10(maximum(times))))
return xlimit,ylimit
end
```
#### Sets tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (6:10)
reltols = 1.0 ./ 10.0 .^ (6:10);
```
## Implicit Work-Precision Diagrams
Benchmarks for implicit solvers.
#### Declare solvers (using default linear solver)
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>CVODE_BDF(linear_solver=:LapackDense)),
Dict(:alg=>CVODE_Adams()),
Dict(:alg=>TRBDF2()),
Dict(:alg=>QNDF()),
Dict(:alg=>FBDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rosenbrock23()),
Dict(:alg=>Rodas4()),
Dict(:alg=>Rodas5P())
];
```
#### Plot Work-Precision Diagram (using default linear solver)
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=100)
names = ["lsoda" "CVODE_BDF" "CVODE_BDF (Lapack Dense)" "CVODE_Adams" "TRBDF2" "QNDF" "FBDF" "KenCarp4" "Rosenbrock23" "Rodas4" "Rodas5P"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using GMRES linear solver)
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES)),
Dict(:alg=>TRBDF2(linsolve=KrylovJL_GMRES())),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES())),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES())),
Dict(:alg=>KenCarp4(linsolve=KrylovJL_GMRES())),
Dict(:alg=>Rosenbrock23(linsolve=KrylovJL_GMRES())),
Dict(:alg=>Rodas4(linsolve=KrylovJL_GMRES())),
Dict(:alg=>Rodas5P(linsolve=KrylovJL_GMRES()))
];
```
#### Plot Work-Precision Diagram (using GMRES linear solver)
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=100)
names = ["lsoda" "CVODE_BDF (GMRES)" "TRBDF2 (GMRES)" "QNDF (GMRES)" "FBDF (GMRES)" "KenCarp4 (GMRES)" "Rosenbrock23 (GMRES)" "Rodas4 (GMRES)" "Rodas5P (GMRES)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using sparse jacobian)
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>CVODE_BDF(linear_solver=:KLU)),
Dict(:alg=>TRBDF2(linsolve=KLUFactorization())),
Dict(:alg=>QNDF(linsolve=KLUFactorization())),
Dict(:alg=>FBDF(linsolve=KLUFactorization())),
Dict(:alg=>KenCarp4(linsolve=KLUFactorization())),
Dict(:alg=>Rosenbrock23(linsolve=KLUFactorization())),
Dict(:alg=>Rodas4(linsolve=KLUFactorization())),
Dict(:alg=>Rodas5P(linsolve=KLUFactorization()))
];
```
#### Plot Work-Precision Diagram (using sparse jacobian)
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(sparsejacprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=100)
names = ["CVODE_BDF (KLU, sparse jac)" "TRBDF2 (KLU, sparse jac)" "QNDF (KLU, sparse jac)" "FBDF (KLU, sparse jac)" "KenCarp4 (KLU, sparse jac)" "Rosenbrock23 (KLU, sparse jac)" "Rodas4 (KLU, sparse jac)" "Rodas5P (KLU, sparse jac)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Explicit Work-Precision Diagram
Benchmarks for explicit solvers.
#### Declare solvers
We designate the solvers we wish to use, this also includes lsoda and CVODE.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_Adams()),
Dict(:alg=>Tsit5()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern6()),
Dict(:alg=>Vern7()),
Dict(:alg=>Vern8()),
Dict(:alg=>Vern9()),
Dict(:alg=>ROCK4())
];
```
#### Plot Work-Precision Diagram
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_Adams" "Tsit5" "BS5" "VCABM" "Vern6" "Vern7" "Vern8" "Vern9" "ROCK4"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Additional explicit solvers
One additional explicit solver, `ROCK2`, performs noticeably worse as compared to the other ones.
```julia
setups = [Dict(:alg=>ROCK2())];
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["ROCK2"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Summary of results
Finally, we compute a single diagram comparing the various solvers used.
#### Declare solvers
We designate the solvers we wish to compare.
```julia
setups = [
Dict(:alg=>lsoda(), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES), :prob_choice => 1),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES()), :prob_choice => 2),
Dict(:alg=>QNDF(linsolve=KLUFactorization()), :prob_choice => 2),
Dict(:alg=>BS5(), :prob_choice => 1),
Dict(:alg=>Vern6(), :prob_choice => 1),
Dict(:alg=>ROCK4(), :prob_choice => 1)
];
```
#### Plot Work-Precision Diagram
For these, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet([oprob,sparsejacprob],abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=[test_sol,test_sol],maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_BDF (GMRES)" "QNDF (GMRES)" "QNDF (KLU)" "BS5" "Vern6" "ROCK4"]
colors = [:seagreen1 :darkgreen :deepskyblue1 :deepskyblue4 :thistle2 :lightslateblue :purple4]
markershapes = [:star4 :rect :hexagon :octagon :star8 :rtriangle :square]
xlimit,ylimit = plot_settings(wp)
xlimit = xlimit .* [0.95,1/0.95]; ylimit = ylimit .* [0.95,1/0.95];
plot(wp;label=names,left_margin=10Plots.mm,right_margin=10Plots.mm,xlimit=xlimit,ylimit=ylimit,xticks=[1e-9,1e-8,1e-7,1e-6,1e-5,1e-4,1e-3,1e-2],yticks=[1e-2,1e-1],color=colors,markershape=markershapes,legendfontsize=15,tickfontsize=15,guidefontsize=15, legend=:topright, lw=20, la=0.8, markersize=20,markerstrokealpha=1.0, markerstrokewidth=1.5, gridalpha=0.3, gridlinewidth=7.5,size=(1100,1000))
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 11811 | ---
title: Fceri_gamma2 Work-Precision Diagrams
author: Torkel Loman
---
The following benchmark is of 356 ODEs with 3749 terms that describe a stiff
chemical reaction network. This fceri_gamma2 model was used as a benchmark model in [Gupta et
al.](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC6013266/). It describes high-affinity human IgE receptor signalling [Faeder et
al.](https://www.jimmunol.org/content/170/7/3769.long). We use
[`ReactionNetworkImporters`](https://github.com/isaacsas/ReactionNetworkImporters.jl)
to load the BioNetGen model files as a
[Catalyst](https://github.com/SciML/Catalyst.jl) model, and then use
[ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl) to convert the
Catalyst network model to ODEs.
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools,
LinearSolve
gr()
const to = TimerOutput()
tf = 150.0
# generate ModelingToolkit ODEs
@timeit to "Parse Network" prnbng = loadrxnetwork(BNGNetwork(), joinpath(@__DIR__, "Models/fceri_gamma2.net"))
show(to)
rn = prnbng.rn
obs = [eq.lhs for eq in observed(rn)]
@timeit to "Create ODESys" osys = convert(ODESystem, rn)
show(to)
tspan = (0.,tf)
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
@timeit to "ODEProb No Jac" oprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[])
show(to)
oprob_sparse = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[]; sparse=true);
```
```julia
@show numspecies(rn) # Number of ODEs
@show numreactions(rn) # Apprx. number of terms in the ODE
@show length(parameters(rn)); # Number of Parameters
```
## Time ODE derivative function compilation
As compiling the ODE derivative functions has in the past taken longer than
running a simulation, we first force compilation by evaluating these functions
one time.
```julia
u = ModelingToolkit.varmap_to_vars(nothing, species(rn); defaults=ModelingToolkit.defaults(rn))
du = copy(u)
p = ModelingToolkit.varmap_to_vars(nothing, parameters(rn); defaults=ModelingToolkit.defaults(rn))
@timeit to "ODE rhs Eval1" sparsejacprob.f(du,u,p,0.)
sparsejacprob.f(du,u,p,0.)
```
We also time the ODE rhs function with BenchmarkTools as it is more accurate
given how fast evaluating `f` is:
```julia
@btime sparsejacprob.f($du,$u,$p,0.)
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF(), saveat=tf/1000., reltol=1e-5, abstol=1e-5)
plot(sol; idxs=obs, legend=false, fmt=:png)
```
For these benchmarks we will be using the time-series error with these saving
points.
## Generate Test Solution
```julia
@time sol = solve(sparsejacprob, CVODE_BDF(linear_solver=:GMRES), reltol=1e-15, abstol=1e-15)
test_sol = TestSolution(sol);
```
## Setups
#### Sets plotting defaults
```julia
default(legendfontsize=7,framestyle=:box,gridalpha=0.3,gridlinewidth=2.5)
```
#### Declares a plotting helper function
```julia
function plot_settings(wp)
times = vcat(map(wp -> wp.times, wp.wps)...)
errors = vcat(map(wp -> wp.errors, wp.wps)...)
xlimit = 10 .^ (floor(log10(minimum(errors))), ceil(log10(maximum(errors))))
ylimit = 10 .^ (floor(log10(minimum(times))), ceil(log10(maximum(times))))
return xlimit,ylimit
end
```
#### Declare pre-conditioners
```julia
using IncompleteLU, LinearAlgebra
jaccache = sparsejacprob.f.jac(oprob.u0,oprob.p,0.0)
W = I - 1.0*jaccache
prectmp = ilu(W, τ = 50.0)
preccache = Ref(prectmp)
const τ1 = 5
function psetupilu(p, t, u, du, jok, jcurPtr, gamma)
if !jok
sparsejacprob.f.jac(jaccache,u,p,t)
jcurPtr[] = true
# W = I - gamma*J
@. W = -gamma*jaccache
idxs = diagind(W)
@. @view(W[idxs]) = @view(W[idxs]) + 1
# Build preconditioner on W
preccache[] = ilu(W, τ = τ1)
end
end
function precilu(z,r,p,t,y,fy,gamma,delta,lr)
ldiv!(z,preccache[],r)
end
const τ2 = 5
function incompletelu(W,du,u,p,t,newW,Plprev,Prprev,solverdata)
if newW === nothing || newW
Pl = ilu(convert(AbstractMatrix,W), τ = τ2)
else
Pl = Plprev
end
Pl,nothing
end;
```
#### Sets tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (5:8);
```
## Work-Precision Diagrams (CVODE and lsoda solvers)
#### Declare solvers.
```julia
setups = [
Dict(:alg=>lsoda(), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:LapackDense), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES,prec=precilu,psetup=psetupilu,prec_side=1), :prob_choice => 2),
Dict(:alg=>CVODE_BDF(linear_solver=:KLU), :prob_choice => 3)
];
```
#### Plot Work-Precision Diagram.
```julia
wp = WorkPrecisionSet([oprob,oprob_sparse,sparsejacprob],abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=[test_sol,test_sol,test_sol],maxiters=Int(1e9),numruns=10)
names = ["lsoda" "CVODE_BDF" "CVODE_BDF (LapackDense)" "CVODE_BDF (GMRES)" "CVODE_BDF (GMRES, iLU)" "CVODE_BDF (KLU, sparse jac)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Work-Precision Diagrams (various Julia solvers)
#### Declare solvers (using default linear solver).
```julia
setups = [
Dict(:alg=>TRBDF2(autodiff=false)),
Dict(:alg=>QNDF(autodiff=false)),
Dict(:alg=>FBDF(autodiff=false)),
Dict(:alg=>KenCarp4(autodiff=false))
];
```
#### Plot Work-Precision Diagram (using default linear solver).
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=10)
names = ["TRBDF2" "QNDF" "FBDF" "KenCarp4"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using GMRES linear solver).
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false)),
Dict(:alg=>KenCarp4(linsolve=KrylovJL_GMRES(),autodiff=false))
];
```
#### Plot Work-Precision Diagram (using GMRES linear solver).
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=10)
names = ["TRBDF2 (GMRES)" "QNDF (GMRES)" "FBDF (GMRES)" "KenCarp4 (GMRES)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using GMRES linear solver, with pre-conditioner).
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true)),
Dict(:alg=>KenCarp4(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true))
];
```
#### Plot Work-Precision Diagram (using GMRES linear solver, with pre-conditioner).
```julia
wp = WorkPrecisionSet(sparsejacprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=10)
names = ["TRBDF2 (GMRES, iLU)" "QNDF (GMRES, iLU)" "FBDF (GMRES, iLU)" "KenCarp4 (GMRES, iLU)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Declare solvers (using sparse jacobian)
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>TRBDF2(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>QNDF(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>FBDF(linsolve=KLUFactorization(),autodiff=false)),
Dict(:alg=>KenCarp4(linsolve=KLUFactorization(),autodiff=false))
];
```
#### Plot Work-Precision Diagram (using sparse jacobian)
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(sparsejacprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=10)
names = ["TRBDF2 (KLU, sparse jac)" "QNDF (KLU, sparse jac)" "FBDF (KLU, sparse jac)" "KenCarp4 (KLU, sparse jac)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Explicit Work-Precision Diagram
Benchmarks for explicit solvers.
#### Declare solvers
We designate the solvers we wish to use, this also includes lsoda and CVODE.
```julia
setups = [
Dict(:alg=>CVODE_Adams()),
Dict(:alg=>Tsit5()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern6()),
Dict(:alg=>Vern7()),
Dict(:alg=>Vern8()),
Dict(:alg=>Vern9())
];
```
#### Plot Work-Precision Diagram
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=10)
names = ["CVODE_Adams" "Tsit5" "BS5" "Vern6" "Vern7" "Vern8" "Vern9"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Summary of results
Finally, we compute a single diagram comparing the various solvers used.
#### Declare solvers
We designate the solvers we wish to compare.
```julia
setups = [
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES), :prob_choice => 1),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES,prec=precilu,psetup=psetupilu,prec_side=1), :prob_choice => 2),
Dict(:alg=>QNDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true), :prob_choice => 3),
Dict(:alg=>FBDF(linsolve=KrylovJL_GMRES(),autodiff=false,precs=incompletelu,concrete_jac=true), :prob_choice => 3),
Dict(:alg=>Tsit5())
];
```
#### Plot Work-Precision Diagram
For these, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet([oprob,oprob_sparse,sparsejacprob],abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=[test_sol,test_sol,test_sol],maxiters=Int(1e9),numruns=200)
names = ["CVODE_BDF (GMRES)" "CVODE_BDF (GMRES, iLU)" "QNDF (GMRES, iLU)" "FBDF (GMRES, iLU)" "Tsit5"]
colors = [:darkgreen :green :deepskyblue1 :dodgerblue2 :orchid2]
markershapes = [:rect :octagon :hexagon :rtriangle :ltriangle]
xlimit,ylimit = plot_settings(wp)
xlimit = xlimit .* [0.95,1/0.95]; ylimit = ylimit .* [0.95,1/0.95];
plot(wp;label=names,left_margin=10Plots.mm,right_margin=10Plots.mm,xlimit=xlimit,ylimit=ylimit,xticks=[1e-9,1e-8,1e-7,1e-6,1e-5,1e-4,1e-3,1e-2,1e-1],yticks=[1e-1,1e0,1e1,1e2],color=colors,markershape=markershapes,legendfontsize=15,tickfontsize=15,guidefontsize=15, legend=:topright, lw=20, la=0.8, markersize=20,markerstrokealpha=1.0, markerstrokewidth=1.5, gridalpha=0.3, gridlinewidth=7.5,size=(1100,1000))
```
```julia
echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 8424 | ---
title: Multisite2 Work-Precision Diagrams
author: Torkel Loman
---
The following benchmark is of 66 ODEs with 288 terms that describe a
chemical reaction network. This multisite2 model was used as a benchmark model in [Gupta et
al.](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC6013266/). We use
[`ReactionNetworkImporters`](https://github.com/isaacsas/ReactionNetworkImporters.jl)
to load the BioNetGen model files as a
[Catalyst](https://github.com/SciML/Catalyst.jl) model, and then use
[ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl) to convert the
Catalyst network model to ODEs.
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools,
LinearSolve
gr()
const to = TimerOutput()
tf = 2.0
# generate ModelingToolkit ODEs
@timeit to "Parse Network" prnbng = loadrxnetwork(BNGNetwork(), joinpath(@__DIR__, "Models/multisite2.net"))
show(to)
rn = prnbng.rn
obs = [eq.lhs for eq in observed(rn)]
@timeit to "Create ODESys" osys = convert(ODESystem, rn)
show(to)
tspan = (0.,tf)
@timeit to "ODEProb No Jac" oprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[])
show(to);
```
```julia
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
```
```julia
@show numspecies(rn) # Number of ODEs
@show numreactions(rn) # Apprx. number of terms in the ODE
@show length(parameters(rn)); # Number of Parameters
```
## Time ODE derivative function compilation
As compiling the ODE derivative functions has in the past taken longer than
running a simulation, we first force compilation by evaluating these functions
one time.
```julia
u = ModelingToolkit.varmap_to_vars(nothing, species(rn); defaults=ModelingToolkit.defaults(rn))
du = copy(u)
p = ModelingToolkit.varmap_to_vars(nothing, parameters(rn); defaults=ModelingToolkit.defaults(rn))
@timeit to "ODE rhs Eval1" oprob.f(du,u,p,0.)
@timeit to "ODE rhs Eval2" oprob.f(du,u,p,0.)
sparsejacprob.f(du,u,p,0.)
```
We also time the ODE rhs function with BenchmarkTools as it is more accurate
given how fast evaluating `f` is:
```julia
@btime oprob.f($du,$u,$p,0.)
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF(), saveat=tf/1000., reltol=1e-5, abstol=1e-5)
plot(sol; idxs=obs, legend=false, fmt=:png)
```
For these benchmarks we will be using the time-series error with these saving
points.
## Generate Test Solution
```julia
@time sol = solve(oprob, CVODE_BDF(), reltol=1e-15, abstol=1e-15)
test_sol = TestSolution(sol);
```
## Setups
#### Sets plotting defaults
```julia
default(legendfontsize=7,framestyle=:box,gridalpha=0.3,gridlinewidth=2.5)
```
#### Declares a plotting helper function
```julia
function plot_settings(wp)
times = vcat(map(wp -> wp.times, wp.wps)...)
errors = vcat(map(wp -> wp.errors, wp.wps)...)
xlimit = 10 .^ (floor(log10(minimum(errors))), ceil(log10(maximum(errors))))
ylimit = 10 .^ (floor(log10(minimum(times))), ceil(log10(maximum(times))))
return xlimit,ylimit
end
```
#### Sets tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (6:10)
reltols = 1.0 ./ 10.0 .^ (6:10);
```
## Work-Precision Diagram
We start by trying lsoda and CVODE solvers.
#### Declare solvers
We designate the solvers (and options) we wish to use.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>CVODE_BDF(linear_solver=:LapackDense)),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES))
];
```
#### Plot Work-Precision Diagram
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_BDF" "CVODE_BDF (LapackDense)" "CVODE_BDF (GMRES)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Implicit Work-Precision Diagram
Next, we try a couple of implicit Julia solvers.
#### Declare solvers
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>TRBDF2()),
Dict(:alg=>QNDF()),
Dict(:alg=>FBDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rosenbrock23()),
Dict(:alg=>Rodas4()),
Dict(:alg=>Rodas5P())
];
```
#### Plot Work-Precision Diagram
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=200)
names = ["TRBDF2" "QNDF" "FBDF" "KenCarp4" "Rosenbrock23" "Rodas4" "Rodas5P"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
Implicit methods doing poorly suggests it's non-stiff.
## Explicit Work-Precision Diagram
Benchmarks for explicit solvers.
#### Declare solvers
We designate the solvers we wish to use, this also includes lsoda and CVODE.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_Adams()),
Dict(:alg=>Tsit5()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern6()),
Dict(:alg=>Vern7()),
Dict(:alg=>Vern8()),
Dict(:alg=>Vern9()),
Dict(:alg=>ROCK4())
];
```
#### Plot Work-Precision Diagram
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_Adams" "Tsit5" "BS5" "VCABM" "Vern6" "Vern7" "Vern8" "Vern9" "ROCK4"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Additional explicit solvers
One additional explicit solver, `ROCK2`, performs noticeably worse as compared to the other ones.
```julia
setups = [Dict(:alg=>ROCK2())];
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["ROCK2"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Summary of results
Finally, we compute a single diagram comparing the various solvers used.
#### Declare solvers
We designate the solvers we wish to compare.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES)),
Dict(:alg=>QNDF()),
Dict(:alg=>FBDF()),
Dict(:alg=>Rodas5P()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern6()),
Dict(:alg=>ROCK4())
];
```
#### Plot Work-Precision Diagram
For these, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_BDF (GMRES)" "QNDF" "FBDF" "Rodas5P" "BS5" "VCABM" "Vern6" "ROCK4"]
colors = [:seagreen1 :darkgreen :deepskyblue1 :dodgerblue2 :blue :thistle2 :lightsteelblue2 :lightslateblue :purple4]
markershapes = [:star4 :rect :hexagon :rtriangle :heptagon :star8 :heptagon :rtriangle :square]
xlimit,ylimit = plot_settings(wp)
xlimit = xlimit .* [0.95,1/0.95]; ylimit = ylimit .* [0.95,1/0.95];
plot(wp;label=names,left_margin=10Plots.mm,right_margin=10Plots.mm,xlimit=xlimit,ylimit=ylimit,xticks=[1e-10,1e-9,1e-8,1e-7,1e-6,1e-5,1e-4,1e-3],yticks=[1e-3,1e-2,1e-1],color=colors,markershape=markershapes,legendfontsize=15,tickfontsize=15,guidefontsize=15, legend=:topright, lw=20, la=0.8, markersize=20,markerstrokealpha=1.0, markerstrokewidth=1.5, gridalpha=0.3, gridlinewidth=7.5,size=(1100,1000))
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 8394 | ---
title: Multistate Work-Precision Diagrams
author: Torkel Loman
---
The following benchmark is of 9 ODEs with 18 terms that describe a
chemical reaction network. This multistate model was used as a benchmark model in [Gupta et
al.](https://www.ncbi.nlm.nih.gov/pmc/articles/PMC6013266/). We use
[`ReactionNetworkImporters`](https://github.com/isaacsas/ReactionNetworkImporters.jl)
to load the BioNetGen model files as a
[Catalyst](https://github.com/SciML/Catalyst.jl) model, and then use
[ModelingToolkit](https://github.com/SciML/ModelingToolkit.jl) to convert the
Catalyst network model to ODEs.
```julia
using DiffEqBase, OrdinaryDiffEq, Catalyst, ReactionNetworkImporters,
Sundials, Plots, DiffEqDevTools, ODEInterface, ODEInterfaceDiffEq,
LSODA, TimerOutputs, LinearAlgebra, ModelingToolkit, BenchmarkTools
gr()
const to = TimerOutput()
tf = 20.0
# generate ModelingToolkit ODEs
@timeit to "Parse Network" prnbng = loadrxnetwork(BNGNetwork(), joinpath(@__DIR__, "Models/multistate.net"))
show(to)
rn = prnbng.rn
obs = [eq.lhs for eq in observed(rn)]
@timeit to "Create ODESys" osys = convert(ODESystem, rn)
show(to)
tspan = (0.,tf)
@timeit to "ODEProb No Jac" oprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[])
show(to);
```
```julia
@timeit to "ODEProb SparseJac" sparsejacprob = ODEProblem{true, SciMLBase.FullSpecialize}(osys, Float64[], tspan, Float64[], jac=true, sparse=true)
show(to)
```
```julia
@show numspecies(rn) # Number of ODEs
@show numreactions(rn) # Apprx. number of terms in the ODE
@show length(parameters(rn)); # Number of Parameters
```
## Time ODE derivative function compilation
As compiling the ODE derivative functions has in the past taken longer than
running a simulation, we first force compilation by evaluating these functions
one time.
```julia
u = ModelingToolkit.varmap_to_vars(nothing, species(rn); defaults=ModelingToolkit.defaults(rn))
du = copy(u)
p = ModelingToolkit.varmap_to_vars(nothing, parameters(rn); defaults=ModelingToolkit.defaults(rn))
@timeit to "ODE rhs Eval1" oprob.f(du,u,p,0.)
@timeit to "ODE rhs Eval2" oprob.f(du,u,p,0.)
sparsejacprob.f(du,u,p,0.)
```
We also time the ODE rhs function with BenchmarkTools as it is more accurate
given how fast evaluating `f` is:
```julia
@btime oprob.f($du,$u,$p,0.)
```
## Picture of the solution
```julia
sol = solve(oprob, CVODE_BDF(), saveat=tf/1000., reltol=1e-5, abstol=1e-5)
plot(sol; idxs=obs, legend=false, fmt=:png)
```
For these benchmarks we will be using the time-series error with these saving
points.
## Generate Test Solution
```julia
@time sol = solve(oprob, CVODE_BDF(), reltol=1e-15, abstol=1e-15)
test_sol = TestSolution(sol);
```
## Setups
#### Sets plotting defaults
```julia
default(legendfontsize=7,framestyle=:box,gridalpha=0.3,gridlinewidth=2.5)
```
#### Declares a plotting helper function
```julia
function plot_settings(wp)
times = vcat(map(wp -> wp.times, wp.wps)...)
errors = vcat(map(wp -> wp.errors, wp.wps)...)
xlimit = 10 .^ (floor(log10(minimum(errors))), ceil(log10(maximum(errors))))
ylimit = 10 .^ (floor(log10(minimum(times))), ceil(log10(maximum(times))))
return xlimit,ylimit
end
```
#### Sets tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (6:10)
reltols = 1.0 ./ 10.0 .^ (6:10);
```
## Work-Precision Diagram
We start by trying lsoda and CVODE solvers.
#### Declare solvers
We designate the solvers (and options) we wish to use.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>CVODE_BDF(linear_solver=:LapackDense)),
Dict(:alg=>CVODE_BDF(linear_solver=:GMRES))
];
```
#### Plot Work-Precision Diagram
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_BDF" "CVODE_BDF (LapackDense)" "CVODE_BDF (GMRES)"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Implicit Work-Precision Diagram
Next, we try a couple of implicit Julia solvers.
#### Declare solvers
We designate the solvers we wish to use.
```julia
setups = [
Dict(:alg=>TRBDF2()),
Dict(:alg=>QNDF()),
Dict(:alg=>FBDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rosenbrock23()),
Dict(:alg=>Rodas4()),
Dict(:alg=>Rodas5P())
];
```
#### Plot Work-Precision Diagram
Finally, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e12),dtmin=1e-18,numruns=200)
names = ["TRBDF2" "QNDF" "FBDF" "KenCarp4" "Rosenbrock23" "Rodas4" "Rodas5P"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
Implicit methods doing poorly suggests it's non-stiff.
## Explicit Work-Precision Diagram
Benchmarks for explicit solvers.
#### Declare solvers
We designate the solvers we wish to use, this also includes lsoda and CVODE.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>Tsit5()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern6()),
Dict(:alg=>Vern7()),
Dict(:alg=>Vern8()),
Dict(:alg=>Vern9()),
Dict(:alg=>ROCK4())
];
```
#### Plot Work-Precision Diagram
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "Tsit5" "BS5" "VCABM" "Vern6" "Vern7" "Vern8" "Vern9" "ROCK4"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
#### Additional explicit solvers
Two additional explicit solvers, `CVODE_Adams` and `ROCK2`, perform noticeably worse as compared to the other ones.
```julia
setups = [Dict(:alg=>CVODE_Adams()), Dict(:alg=>ROCK2())];
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["CVODE_Adams" "ROCK2"]
xlimit,ylimit = plot_settings(wp)
plot(wp;label=names,xlimit=xlimit,ylimit=ylimit)
```
## Summary of results
Finally, we compute a single diagram comparing the various solvers used.
#### Declare solvers
We designate the solvers we wish to compare.
```julia
setups = [
Dict(:alg=>lsoda()),
Dict(:alg=>CVODE_BDF()),
Dict(:alg=>QNDF()),
Dict(:alg=>KenCarp4()),
Dict(:alg=>Rodas5P()),
Dict(:alg=>Tsit5()),
Dict(:alg=>BS5()),
Dict(:alg=>VCABM()),
Dict(:alg=>Vern7())
];
```
#### Plot Work-Precision Diagram
For these, we generate a work-precision diagram for the selection of solvers.
```julia
wp = WorkPrecisionSet(oprob,abstols,reltols,setups;error_estimate=:l2,
saveat=tf/10000.,appxsol=test_sol,maxiters=Int(1e9),numruns=200)
names = ["lsoda" "CVODE_BDF" "QNDF" "KenCarp4" "Rodas5P" "Tsit5" "BS5" "VCABM" "Vern7"]
colors = [:seagreen1 :chartreuse1 :deepskyblue1 :lightskyblue :blue :orchid2 :thistle2 :lightsteelblue2 :mediumpurple1]
markershapes = [:star4 :circle :hexagon :star5 :heptagon :ltriangle :star8 :heptagon :star6]
xlimit,ylimit = plot_settings(wp)
xlimit = xlimit .* [0.95,1/0.95]; ylimit = ylimit .* [0.95,1/0.95];
plot(wp;label=names,left_margin=10Plots.mm,right_margin=10Plots.mm,xlimit=xlimit,ylimit=ylimit,xticks=[1e-12,1e-11,1e-10,1e-9,1e-8,1e-7,1e-6,1e-5,1e-4,1e-3,1e-2],yticks=[1e-3,1e-2],color=colors,markershape=markershapes,legendfontsize=15,tickfontsize=15,guidefontsize=15, legend=:topright, lw=20, la=0.8, markersize=20,markerstrokealpha=1.0, markerstrokewidth=1.5, gridalpha=0.3, gridlinewidth=7.5,size=(1100,1000))
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 6788 | ---
title: Chemical Akzo Nobel Differential-Algebraic Equation (DAE) Work-Precision Diagrams
author: Chris Rackauckas
---
```julia
using OrdinaryDiffEq, DiffEqDevTools, Sundials, ModelingToolkit, ODEInterfaceDiffEq,
Plots, DASSL, DASKR
using LinearAlgebra
ModelingToolkit.@parameters begin
k₁=18.7
k₂=0.58
k₃=0.09
k₄=0.42
kbig=34.4
kla=3.3
ks=115.83
po2=0.9
hen=737
end
@variables begin
t
y₁(t) = 0.444
y₂(t) = 0.00123
y₃(t) = 0.0
y₄(t) = 0.007
y₅(t) = 1.0
y₆(t) = 115.83*0.444*0.007 # ks*y₁*y₄
end
D = Differential(t)
r₁ = k₁ * (y₁^4.)*sqrt(abs(y₂))
r₂ = k₂ * y₃ * y₄
r₃ = k₂/kbig * y₁ * y₅
r₄ = k₃*y₁*(y₄^2)
r₅ = k₄*(y₆^2)*sqrt(abs(y₂))
fin = kla*(po2/hen-y₂)
eqs = [
D(y₁) ~ -2. * r₁ + r₂ - r₃ - r₄
D(y₂) ~ -0.5 * r₁ - r₄ - 0.5*r₅ + fin
D(y₃) ~ r₁ - r₂ + r₃
D(y₄) ~ -r₂ + r₃ - 2. * r₄
D(y₅) ~ r₂ - r₃ + r₅
0. ~ ks * y₁ * y₄ - y₆
]
ModelingToolkit.@named sys = ModelingToolkit.ODESystem(eqs)
tspan = (0.0, 180.0)
mmprob = ODEProblem(sys, [], tspan)
sol = solve(mmprob, Rodas4(),abstol=1/10^14,reltol=1/10^14)
odaeprob = ODAEProblem(structural_simplify(sys),[],tspan)
ode_ref_sol = solve(odaeprob,CVODE_BDF(),abstol=1/10^14,reltol=1/10^14);
du = mmprob.f(mmprob.u0,mmprob.p,0.0)
du0 = D.(states(sys)) .=> du
daeprob = DAEProblem(sys,du0,[],tspan)
ref_sol = solve(daeprob,IDA(),abstol=1/10^14,reltol=1/10^14);
probs = [mmprob,daeprob,odaeprob]
refs = [ref_sol,ref_sol,ode_ref_sol];
```
```julia
plot(ode_ref_sol, vars = [y₁,y₂,y₃,y₄,y₅,y₆])
```
## High Tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (2:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (3:5);
setups = [Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 2, :alg=>DASSL.dassl()),
Dict(:prob_choice => 2, :alg=>DASKR.daskr()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Timeseries Errors
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()), # too slow
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (2:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Low Tolerances
This is the speed at lower tolerances, measuring what's good when accuracy is needed.
```julia
abstols = 1.0 ./ 10.0 .^ (7:12)
reltols = 1.0 ./ 10.0 .^ (4:9)
setups = [Dict(:prob_choice => 1, :alg=>Rodas5()),
Dict(:prob_choice => 3, :alg=>Rodas5()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
DASKR fails at too low of tolerances, so pull back for a comparison.
```julia
abstols = 1.0 ./ 10.0 .^ (7:9)
reltols = 1.0 ./ 10.0 .^ (4:6)
setups = [Dict(:prob_choice => 1, :alg=>Rodas5()),
Dict(:prob_choice => 3, :alg=>Rodas5()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 2, :alg=>DASKR.daskr()),
]
gr()
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Conclusion
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 5482 | ---
title: OREGO Differential-Algebraic Equation (DAE) Work-Precision Diagrams
author: Chris Rackauckas
---
```julia
using OrdinaryDiffEq, DiffEqDevTools, Sundials, ModelingToolkit, ODEInterfaceDiffEq,
Plots, DASSL, DASKR
using LinearAlgebra
@variables t y1(t)=1.0 y2(t)=2.0 y3(t)=3.0
@parameters p1=77.27 p2=8.375e-6 p3=0.161
D = Differential(t)
eqs = [
D(y1) ~ p1*(y2+y1*(1-p2*y1-y2))
D(y2) ~ (y3-(1+y1)*y2)/p1
D(y3) ~ p3*(y1-y3)
]
@named sys = ODESystem(eqs)
simpsys = structural_simplify(sys)
mmprob = ODEProblem(sys,[],(0.0,30.0))
daeprob = DAEProblem(sys,[D(y1)=>77.26935286375,
D(y2)=>-0.012941633234114146,
D(y3)=>-0.322],[],(0.0,30.0))
odaeprob = ODAEProblem(simpsys,[],(0.0,30.0))
ref_sol = solve(daeprob,IDA(),abstol=1/10^14,reltol=1/10^14);
ode_ref_sol = solve(odaeprob,CVODE_BDF(),abstol=1/10^14,reltol=1/10^14);
probs = [mmprob,daeprob,odaeprob]
refs = [ref_sol,ref_sol,ode_ref_sol];
```
```julia
plot(ref_sol)
```
## High Tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (6:9)
reltols = 1.0 ./ 10.0 .^ (2:5);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;print_names=true,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (2:4);
setups = [Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 2, :alg=>DASSL.dassl()),
Dict(:prob_choice => 2, :alg=>DASKR.daskr()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Timeseries Errors
```julia
abstols = 1.0 ./ 10.0 .^ (6:9)
reltols = 1.0 ./ 10.0 .^ (2:5);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()), # too slow
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
gr()
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:9)
reltols = 1.0 ./ 10.0 .^ (2:5);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Low Tolerances
This is the speed at lower tolerances, measuring what's good when accuracy is needed.
```julia
abstols = 1.0 ./ 10.0 .^ (7:12)
reltols = 1.0 ./ 10.0 .^ (4:9)
setups = [Dict(:prob_choice => 1, :alg=>Rodas5()),
Dict(:prob_choice => 3, :alg=>Rodas5()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
gr()
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Conclusion
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 6178 | ---
title: ROBER Differential-Algebraic Equation (DAE) Work-Precision Diagrams
author: Chris Rackauckas
---
```julia
using OrdinaryDiffEq, DiffEqDevTools, Sundials, ModelingToolkit, ODEInterfaceDiffEq,
Plots, DASSL, DASKR
using LinearAlgebra
@variables t y₁(t)=1.0 y₂(t)=0.0 y₃(t)=0.0
@parameters k₁=0.04 k₂=3e7 k₃=1e4
D = Differential(t)
eqs = [
D(y₁) ~ -k₁*y₁ + k₃*y₂*y₃
D(y₂) ~ k₁*y₁ - k₃*y₂*y₃ - k₂*y₂^2
0 ~ y₁ + y₂ + y₃ - 1
]
@named sys = ODESystem(eqs)
simpsys = structural_simplify(sys)
mmprob = ODEProblem(sys,[],(0.0,1e5))
daeprob = DAEProblem(sys,[D(y₁)=>-0.04,
D(y₂)=>0.04,
D(y₃)=>0.0],[],(0.0,1e5))
odaeprob = ODAEProblem(simpsys,[],(0.0,1e5))
ref_sol = solve(daeprob,IDA(),abstol=1/10^14,reltol=1/10^14);
ode_ref_sol = solve(odaeprob,CVODE_BDF(),abstol=1/10^14,reltol=1/10^14);
probs = [mmprob,daeprob,odaeprob]
refs = [ref_sol,ref_sol,ode_ref_sol];
```
```julia
plot(ode_ref_sol, vars = [y₁,y₂,y₃])
```
## High Tolerances
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (2:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (3:5);
setups = [Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 2, :alg=>DASSL.dassl()),
Dict(:prob_choice => 2, :alg=>DASKR.daskr()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Timeseries Errors
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()), # too slow
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
abstols = 1.0 ./ 10.0 .^ (6:8)
reltols = 1.0 ./ 10.0 .^ (2:4);
setups = [Dict(:prob_choice => 1, :alg=>Rosenbrock23()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 3, :alg=>Rosenbrock23()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>CVODE_BDF()),
Dict(:prob_choice => 3, :alg=>TRBDF2()),
Dict(:prob_choice => 3, :alg=>KenCarp4()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Low Tolerances
This is the speed at lower tolerances, measuring what's good when accuracy is needed.
```julia
abstols = 1.0 ./ 10.0 .^ (7:12)
reltols = 1.0 ./ 10.0 .^ (4:9)
setups = [Dict(:prob_choice => 1, :alg=>Rodas5()),
Dict(:prob_choice => 3, :alg=>Rodas5()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
#Dict(:prob_choice => 1, :alg=>FBDF()),
Dict(:prob_choice => 1, :alg=>QNDF()),
Dict(:prob_choice => 1, :alg=>rodas()),
Dict(:prob_choice => 1, :alg=>radau()),
Dict(:prob_choice => 1, :alg=>RadauIIA5()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
]
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
```julia
wp = WorkPrecisionSet(probs,abstols,reltols,setups;error_estimate = :l2,
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
DASKR fails at too low of tolerances, so pull back for a comparison.
```julia
abstols = 1.0 ./ 10.0 .^ (7:9)
reltols = 1.0 ./ 10.0 .^ (4:6)
setups = [Dict(:prob_choice => 1, :alg=>Rodas5()),
Dict(:prob_choice => 3, :alg=>Rodas5()),
Dict(:prob_choice => 1, :alg=>Rodas4()),
Dict(:prob_choice => 3, :alg=>Rodas4()),
Dict(:prob_choice => 2, :alg=>DFBDF()),
Dict(:prob_choice => 2, :alg=>IDA()),
Dict(:prob_choice => 2, :alg=>DASKR.daskr()),
]
gr()
wp = WorkPrecisionSet(probs,abstols,reltols,setups;
save_everystep=false,appxsol=refs,maxiters=Int(1e5),numruns=10)
plot(wp)
```
### Conclusion
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 7328 | ---
title: Hénon-Heiles Energy Conservation
author: Sebastian Micluța-Câmpeanu, Chris Rackauckas
---
In this notebook we will study the energy conservation properties of several high-order methods
for the [Hénon-Heiles system](https://en.wikipedia.org/wiki/H%C3%A9non%E2%80%93Heiles_system).
We will se how the energy error behaves at very thight tolerances and how different techniques,
such as using symplectic solvers or manifold projections, benchmark against each other.
The Hamiltonian for this system is given by:
$$\mathcal{H}=\frac{1}{2}(p_1^2 + p_2^2) + \frac{1}{2}\left(q_1^2 + q_2^2 + 2q_1^2 q_2 - \frac{2}{3}q_2^3\right)$$
We will also compare the in place apporach with the out of place approach by using `Array`s
(for the in place version) and `StaticArrays` (for out of place versions).
In order to separate these two, we will use `iip` for the in-place names and `oop` for out of place ones.
```julia
using OrdinaryDiffEq, Plots, DiffEqCallbacks
using SciMLBenchmarks
using TaylorIntegration, LinearAlgebra, StaticArrays
gr(fmt=:png)
default(fmt=:png)
T(p) = 1//2 * norm(p)^2
V(q) = 1//2 * (q[1]^2 + q[2]^2 + 2q[1]^2 * q[2]- 2//3 * q[2]^3)
H(p,q, params) = T(p) + V(q)
function iip_dq(dq,p,q,params,t)
dq[1] = p[1]
dq[2] = p[2]
end
function iip_dp(dp,p,q,params,t)
dp[1] = -q[1] * (1 + 2q[2])
dp[2] = -q[2] - (q[1]^2 - q[2]^2)
end
const iip_q0 = [0.1, 0.]
const iip_p0 = [0., 0.5]
function oop_dq(p, q, params, t)
p
end
function oop_dp(p, q, params, t)
dp1 = -q[1] * (1 + 2q[2])
dp2 = -q[2] - (q[1]^2 - q[2]^2)
@SVector [dp1, dp2]
end
const oop_q0 = @SVector [0.1, 0.]
const oop_p0 = @SVector [0., 0.5]
function hamilton(du,u,p,t)
dq, q = @views u[3:4], du[3:4]
dp, p = @views u[1:2], du[1:2]
dp[1] = -q[1] * (1 + 2q[2])
dp[2] = -q[2] - (q[1]^2 - q[2]^2)
dq .= p
return nothing
end
function g(resid, u, p)
resid[1] = H([u[1],u[2]], [u[3],u[4]], nothing) - E
resid[2:4] .= 0
end
const cb = ManifoldProjection(g, nlopts=Dict(:ftol=>1e-13))
const E = H(iip_p0, iip_q0, nothing)
```
For the comparison we will use the following function
```julia
energy_err(sol) = map(i->H([sol[1,i], sol[2,i]], [sol[3,i], sol[4,i]], nothing)-E, 1:length(sol.u))
abs_energy_err(sol) = [abs.(H([sol[1,j], sol[2,j]], [sol[3,j], sol[4,j]], nothing) - E) for j=1:length(sol.u)]
function compare(mode=:inplace, all=true, plt=nothing; tmax=1e2)
if mode == :inplace
prob = DynamicalODEProblem(iip_dp, iip_dq, iip_p0, iip_q0, (0., tmax))
else
prob = DynamicalODEProblem(oop_dp, oop_dq, oop_p0, oop_q0, (0., tmax))
end
prob_linear = ODEProblem(hamilton, vcat(iip_p0, iip_q0), (0., tmax))
GC.gc()
(mode == :inplace && all) && @time sol1 = solve(prob, Vern9(), callback=cb, abstol=1e-14, reltol=1e-14)
GC.gc()
@time sol2 = solve(prob, KahanLi8(), dt=1e-2, maxiters=1e10)
GC.gc()
@time sol3 = solve(prob, SofSpa10(), dt=1e-2, maxiters=1e8)
GC.gc()
@time sol4 = solve(prob, Vern9(), abstol=1e-14, reltol=1e-14)
GC.gc()
@time sol5 = solve(prob, DPRKN12(), abstol=1e-14, reltol=1e-14)
GC.gc()
(mode == :inplace && all) && @time sol6 = solve(prob_linear, TaylorMethod(50), abstol=1e-20)
(mode == :inplace && all) && println("Vern9 + ManifoldProjection max energy error:\t"*
"$(maximum(abs_energy_err(sol1)))\tin\t$(length(sol1.u))\tsteps.")
println("KahanLi8 max energy error:\t\t\t$(maximum(abs_energy_err(sol2)))\tin\t$(length(sol2.u))\tsteps.")
println("SofSpa10 max energy error:\t\t\t$(maximum(abs_energy_err(sol3)))\tin\t$(length(sol3.u))\tsteps.")
println("Vern9 max energy error:\t\t\t\t$(maximum(abs_energy_err(sol4)))\tin\t$(length(sol4.u))\tsteps.")
println("DPRKN12 max energy error:\t\t\t$(maximum(abs_energy_err(sol5)))\tin\t$(length(sol5.u))\tsteps.")
(mode == :inplace && all) && println("TaylorMethod max energy error:\t\t\t$(maximum(abs_energy_err(sol6)))"*
"\tin\t$(length(sol6.u))\tsteps.")
if plt === nothing
plt = plot(xlabel="t", ylabel="Energy error")
end
(mode == :inplace && all) && plot!(sol1.t, energy_err(sol1), label="Vern9 + ManifoldProjection")
plot!(sol2.t, energy_err(sol2), label="KahanLi8", ls=mode==:inplace ? :solid : :dash)
plot!(sol3.t, energy_err(sol3), label="SofSpa10", ls=mode==:inplace ? :solid : :dash)
plot!(sol4.t, energy_err(sol4), label="Vern9", ls=mode==:inplace ? :solid : :dash)
plot!(sol5.t, energy_err(sol5), label="DPRKN12", ls=mode==:inplace ? :solid : :dash)
(mode == :inplace && all) && plot!(sol6.t, energy_err(sol6), label="TaylorMethod")
return plt
end
```
The `mode` argument choses between the in place approach
and the out of place one. The `all` parameter is used to compare only the integrators that support both
the in place and the out of place versions (we reffer here only to the 6 high order methods chosen bellow).
The `plt` argument can be used to overlay the results over a previous plot and the `tmax` keyword determines
the simulation time.
Note:
1. The `Vern9` method is used with `ODEProblem` because of performance issues with `ArrayPartition` indexing which manifest for `DynamicalODEProblem`.
2. The `NLsolve` call used by `ManifoldProjection` was modified to use `ftol=1e-13` in order to obtain a very low energy error.
Here are the results of the comparisons between the in place methods:
```julia
compare(tmax=1e2)
```
```julia
compare(tmax=1e3)
```
```julia
compare(tmax=1e4)
```
```julia
compare(tmax=5e4)
```
We can see that as the simulation time increases, the energy error increases. For this particular example
the energy error for all the methods is comparable. For relatively short simulation times,
if a highly accurate solution is required, the symplectic method is not recommended as
its energy error fluctuations are larger than for other methods.
An other thing to notice is the fact that the two versions of `Vern9` behave identically, as expected,
untill the energy error set by `ftol` is reached.
We will now compare the in place with the out of place versions. In the plots bellow we will use
a dashed line for the out of place versions.
```julia
function in_vs_out(;all=false, tmax=1e2)
println("In place versions:")
plt = compare(:inplace, all, tmax=tmax)
println("\nOut of place versions:")
plt = compare(:oop, false, plt; tmax=tmax)
end
```
First, here is a summary of all the available methods for `tmax = 1e3`:
```julia
in_vs_out(all=true, tmax=1e3)
```
Now we will compare the in place and the out of place versions, but only for the integrators
that are compatible with `StaticArrays`
```julia
in_vs_out(tmax=1e2)
```
```julia
in_vs_out(tmax=1e3)
```
```julia
in_vs_out(tmax=1e4)
```
```julia
in_vs_out(tmax=5e4)
```
As we see from the above comparisons, the `StaticArray` versions are significantly faster and use less memory.
The speedup provided for the out of place version is more proeminent at larger values for `tmax`.
We can see again that if the simulation time is increased, the energy error of the symplectic methods
is less noticeable compared to the rest of the methods.
The benchmarks were performed on a machine with
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 7443 | ---
title: Quadruple Boson Energy Conservation
author: Sebastian Micluța-Câmpeanu, Chris Rackauckas
---
In this notebook we will study the energy conservation properties of several high-order methods for a system with the following Hamiltonian:
$$\mathcal{H}\left(q_0,q_2,p_0,p_2\right) = \frac{A}{2} \left(p_0^2 + p_2^2 + q_0^2 + q_2^2\right) + \frac{B}{\sqrt{2}} q_0 \left(3q_2^2 - q_0^2\right) + \frac{D}{4} \left(q_0^2+q_2^2\right)^2$$
This Hamiltonian resembles the Hénon-Heiles one, but it has an additional fourth order term.
The aim of this benchmark is to see what happens with the energy error when highly accurate solutions are needed and how the results compare with the Hénon-Heiles case.
```julia
using OrdinaryDiffEq, Plots, DiffEqCallbacks, LinearAlgebra
using TaylorIntegration
using ParameterizedFunctions
using StaticArrays
gr()
default(fmt=:png)
T(p) = A / 2 * norm(p)^2
V(q) = A / 2 * (q[1]^2 + q[2]^2) + B / sqrt(2) * q[1] * (3 * q[2]^2 - q[1]^2) + D / 4 * (q[1]^2 + q[2]^2)^2
H(p, q, params) = T(p) + V(q)
const A, B, D = 1., 0.55, 0.4
function iip_dq(dq, p, q, params, t)
dq[1] = A * p[1]
dq[2] = A * p[2]
end
function iip_dp(dp, p, q, params, t)
dp[1] = -A * q[1] - 3 * B / sqrt(2) * (q[2]^2 - q[1]^2) - D * q[1] * (q[1]^2 + q[2]^2)
dp[2] = -q[2] * (A + 3 * sqrt(2) * B * q[1] + D * (q[1]^2 + q[2]^2))
end
const iip_q0 = [4.919080920016389, 2.836942666663649]
const iip_p0 = [0., 0.]
const iip_u0 = vcat(iip_p0,iip_q0)
function oop_dq(p, q, params, t)
p
end
function oop_dp(p, q, params, t)
dp1 = -A * q[1] - 3 * B / sqrt(2) * (q[2]^2 - q[1]^2) - D * q[1] * (q[1]^2 + q[2]^2)
dp2 = -q[2] * (A + 3 * sqrt(2) * B * q[1] + D * (q[1]^2 + q[2]^2))
@SVector [dp1, dp2]
end
const oop_q0 = @SVector [4.919080920016389, 2.836942666663649]
const oop_p0 = @SVector [0., 0.]
const oop_u0 = vcat(oop_p0,oop_q0)
function hamilton(z, params, t)
SVector(
-A * z[3] - 3 * B / sqrt(2) * (z[4]^2 - z[3]^2) - D * z[3] * (z[3]^2 + z[4]^2),
-z[4] * (A + 3 * sqrt(2) * B * z[3] + D * (z[3]^2 + z[4]^2)),
z[1],
z[2]
)
end
function g(resid, u, p)
resid[1] = H([u[1],u[2]],[u[3],u[4]],nothing) - E
resid[2:4] .= 0
end
const E = H(iip_p0, iip_q0, nothing)
const cb = ManifoldProjection(g, nlopts=Dict(:ftol=>1e-13));
```
For the comparison we will use the following function
```julia
energy_err(sol) = map(i->H([sol[1,i], sol[2,i]], [sol[3,i], sol[4,i]],nothing)-E, 1:length(sol.u))
abs_energy_err(sol) = [abs.(H([sol[1,j], sol[2,j]], [sol[3,j], sol[4,j]],nothing) - E) for j=1:length(sol.u)]
function compare(mode=:inplace, all=true, plt=nothing; tmax=1e2)
if mode == :inplace
prob = DynamicalODEProblem(iip_dp, iip_dq, iip_p0, iip_q0, (0., tmax))
else
prob = DynamicalODEProblem(oop_dp, oop_dq, oop_p0, oop_q0, (0., tmax))
end
prob_linear = ODEProblem(hamilton, vcat(iip_p0, iip_q0), (0., tmax))
GC.gc()
(mode == :inplace && all) && @time sol1 = solve(prob, Vern9(), callback=cb, abstol=1e-14, reltol=1e-14)
GC.gc()
@time sol2 = solve(prob, KahanLi8(), dt=1e-2, maxiters=1e10)
GC.gc()
@time sol3 = solve(prob, SofSpa10(), dt=1e-2, maxiters=1e8)
GC.gc()
@time sol4 = solve(prob, Vern9(), abstol=1e-14, reltol=1e-14)
GC.gc()
@time sol5 = solve(prob, DPRKN12(), abstol=1e-14, reltol=1e-14)
GC.gc()
(mode == :inplace && all) && @time sol6 = solve(prob_linear, TaylorMethod(50), abstol=1e-20)
(mode == :inplace && all) && println("Vern9 + ManifoldProjection max energy error:\t"*
"$(maximum(abs_energy_err(sol1)))\tin\t$(length(sol1.u))\tsteps.")
println("KahanLi8 max energy error:\t\t\t$(maximum(abs_energy_err(sol2)))\tin\t$(length(sol2.u))\tsteps.")
println("SofSpa10 max energy error:\t\t\t$(maximum(abs_energy_err(sol3)))\tin\t$(length(sol3.u))\tsteps.")
println("Vern9 max energy error:\t\t\t\t$(maximum(abs_energy_err(sol4)))\tin\t$(length(sol4.u))\tsteps.")
println("DPRKN12 max energy error:\t\t\t$(maximum(abs_energy_err(sol5)))\tin\t$(length(sol5.u))\tsteps.")
(mode == :inplace && all) && println("TaylorMethod max energy error:\t\t\t$(maximum(abs_energy_err(sol6)))"*
"\tin\t$(length(sol6.u))\tsteps.")
if plt == nothing
plt = plot(xlabel="t", ylabel="Energy error")
end
(mode == :inplace && all) && plot!(sol1.t, energy_err(sol1), label="Vern9 + ManifoldProjection")
plot!(sol2.t, energy_err(sol2), label="KahanLi8", ls=mode==:inplace ? :solid : :dash)
plot!(sol3.t, energy_err(sol3), label="SofSpa10", ls=mode==:inplace ? :solid : :dash)
plot!(sol4.t, energy_err(sol4), label="Vern9", ls=mode==:inplace ? :solid : :dash)
plot!(sol5.t, energy_err(sol5), label="DPRKN12", ls=mode==:inplace ? :solid : :dash)
(mode == :inplace && all) && plot!(sol6.t, energy_err(sol6), label="TaylorMethod")
return plt
end
```
The `mode` argument choses between the in place approach
and the out of place one. The `all` parameter is used to compare only the integrators that support both the in place and the out of place versions (we reffer here only to the 6 high order methods chosen bellow).
The `plt` argument can be used to overlay the results over a previous plot and the `tmax` keyword determines the simulation time.
Note:
1. The `Vern9` method is used with `ODEProblem` because of performance issues with `ArrayPartition` indexing which manifest for `DynamicalODEProblem`.
2. The `NLsolve` call used by `ManifoldProjection` was modified to use `ftol=1e-13` in order to obtain a very low energy error.
Here are the results of the comparisons between the in place methods:
```julia
compare(tmax=1e2)
```
```julia
compare(tmax=1e3)
```
```julia
compare(tmax=1e4)
```
```julia
compare(tmax=2e4)
```
As we can see from the above plots, we can achieve a very low energy error for long time simulation by manifold projection and with very high order Taylor methods. In comparison with the Hénon-Heiles system we see that as the Hamiltonian got more complex, the energy error for the other integration methods increased significantly.
We will now compare the in place with the out of place versions. In the plots bellow we will use a dashed line for the out of place versions.
```julia
function in_vs_out(;all=false, tmax=1e2)
println("In place versions:")
plt = compare(:inplace, all, tmax=tmax)
println("\nOut of place versions:")
plt = compare(:oop, false, plt; tmax=tmax)
end
```
First, here is a summary of all the available methods for `tmax = 1e3`:
```julia
in_vs_out(all=true, tmax=1e3)
```
Now we will compare the in place and the out of place versions, but only for the integrators that are compatible with `StaticArrays`
```julia
in_vs_out(tmax=1e2)
```
```julia
in_vs_out(tmax=1e3)
```
```julia
in_vs_out(tmax=1e4)
```
```julia
in_vs_out(tmax=2e4)
```
As we see from the above comparisons, the `StaticArray` versions are significantly faster and use less memory. The speedup provided for the out of place version is more proeminent at larger values for `tmax`.
We can see again that if the simulation time is increased, the energy error of the symplectic methods is less noticeable compared to the rest of the methods.
In comparison with the Henon-Heiles case, we see that the symplectic methods are more competitive with `DPRKN12`.
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 5946 | ---
title: Single Pedulum Comparison
author: Gen Kuroki (黒木玄), Chris Rackauckas
---
# Solving single pendulums by DifferentialEquations.jl
In this notebook, we shall solve the single pendulum equation:
$$\ddot q = -\sin q,$$
where $q$ means the angle.
Hamiltonian:
$$H(q,p) = \frac{1}{2}p^2 - \cos q + 1.$$
Canonical equation:
$$\dot q = p, \quad \dot p = - \sin q.$$
Initial condition:
$$q(0) = 0, \quad p(0) = 2k.$$
Exact solution:
$$q(t) = 2\arcsin(k\,\mathrm{sn}(t,k)).$$
Maximum of $q(t)$:
$$\sin(q_{\max}/2) = k, \quad q_{\max} = \max\{q(t)\}.$$
Define $y(t)$ by
$$y(t) = \sin(q(t)/2) = k\,\mathrm{sn}(t,k), \quad y_{\max} = k.$$
```julia
# Single pendulums shall be solved numerically.
#
using OrdinaryDiffEq, Elliptic, Printf, DiffEqPhysics, Statistics
sol2q(sol) = [sol.u[i][j] for i in 1:length(sol.u), j in 1:length(sol.u[1])÷2]
sol2p(sol) = [sol.u[i][j] for i in 1:length(sol.u), j in length(sol.u[1])÷2+1:length(sol.u[1])]
sol2tqp(sol) = (sol.t, sol2q(sol), sol2p(sol))
# The exact solutions of single pendulums can be expressed by the Jacobian elliptic functions.
#
sn(u, k) = Jacobi.sn(u, k^2) # the Jacobian sn function
# Use PyPlot.
#
using PyPlot
colorlist = [
"#1f77b4", "#ff7f0e", "#2ca02c", "#d62728", "#9467bd",
"#8c564b", "#e377c2", "#7f7f7f", "#bcbd22", "#17becf",
]
cc(k) = colorlist[mod1(k, length(colorlist))]
# plot the sulution of a Hamiltonian problem
#
function plotsol(sol::ODESolution)
local t, q, p
t, q, p = sol2tqp(sol)
local d = size(q)[2]
for j in 1:d
j_str = d > 1 ? "[$j]" : ""
plot(t, q[:,j], color=cc(2j-1), label="q$(j_str)", lw=1)
plot(t, p[:,j], color=cc(2j), label="p$(j_str)", lw=1, ls="--")
end
grid(ls=":")
xlabel("t")
legend()
end
# plot the solution of a Hamiltonian problem on the 2D phase space
#
function plotsol2(sol::ODESolution)
local t, q, p
t, q, p = sol2tqp(sol)
local d = size(q)[2]
for j in 1:d
j_str = d > 1 ? "[$j]" : ""
plot(q[:,j], p[:,j], color=cc(j), label="(q$(j_str),p$(j_str))", lw=1)
end
grid(ls=":")
xlabel("q")
ylabel("p")
legend()
end
# plot the energy of a Hamiltonian problem
#
function plotenergy(H, sol::ODESolution)
local t, q, p
t, q, p = sol2tqp(sol)
local energy = [H(q[i,:], p[i,:], nothing) for i in 1:size(q)[1]]
plot(t, energy, label="energy", color="red", lw=1)
grid(ls=":")
xlabel("t")
legend()
local stdenergy_str = @sprintf("%.3e", std(energy))
title(" std(energy) = $stdenergy_str", fontsize=10)
end
# plot the numerical and exact solutions of a single pendulum
#
# Warning: Assume q(0) = 0, p(0) = 2k. (for the sake of laziness)
#
function plotcomparison(k, sol::ODESolution)
local t, q, p
t, q, p = sol2tqp(sol)
local y = sin.(q/2)
local y_exact = k*sn.(t, k) # the exact solution
plot(t, y, label="numerical", lw=1)
plot(t, y_exact, label="exact", lw=1, ls="--")
grid(ls=":")
xlabel("t")
ylabel("y = sin(q(t)/2)")
legend()
local error_str = @sprintf("%.3e", maximum(abs.(y - y_exact)))
title("maximum(abs(numerical - exact)) = $error_str", fontsize=10)
end
# plot solution and energy
#
function plotsolenergy(H, integrator, Δt, sol::ODESolution)
local integrator_str = replace("$integrator", r"^[^.]*\." => "")
figure(figsize=(10,8))
subplot2grid((21,20), ( 1, 0), rowspan=10, colspan=10)
plotsol(sol)
subplot2grid((21,20), ( 1,10), rowspan=10, colspan=10)
plotsol2(sol)
subplot2grid((21,20), (11, 0), rowspan=10, colspan=10)
plotenergy(H, sol)
suptitle("===== $integrator_str, Δt = $Δt =====")
end
# Solve a single pendulum
#
function singlependulum(k, integrator, Δt; t0 = 0.0, t1 = 100.0)
local H(p,q,params) = p[1]^2/2 - cos(q[1]) + 1
local q0 = [0.0]
local p0 = [2k]
local prob = HamiltonianProblem(H, p0, q0, (t0, t1))
local integrator_str = replace("$integrator", r"^[^.]*\." => "")
@printf("%-25s", "$integrator_str:")
sol = solve(prob, integrator, dt=Δt)
@time local sol = solve(prob, integrator, dt=Δt)
sleep(0.1)
figure(figsize=(10,8))
subplot2grid((21,20), ( 1, 0), rowspan=10, colspan=10)
plotsol(sol)
subplot2grid((21,20), ( 1,10), rowspan=10, colspan=10)
plotsol2(sol)
subplot2grid((21,20), (11, 0), rowspan=10, colspan=10)
plotenergy(H, sol)
subplot2grid((21,20), (11,10), rowspan=10, colspan=10)
plotcomparison(k, sol)
suptitle("===== $integrator_str, Δt = $Δt =====")
end
```
## Tests
```julia
# Single pendulum
k = rand()
integrator = VelocityVerlet()
Δt = 0.1
singlependulum(k, integrator, Δt, t0=-20.0, t1=20.0)
```
```julia
# Two single pendulums
H(q,p,param) = sum(p.^2/2 .- cos.(q) .+ 1)
q0 = pi*rand(2)
p0 = zeros(2)
t0, t1 = -20.0, 20.0
prob = HamiltonianProblem(H, q0, p0, (t0, t1))
integrator = McAte4()
Δt = 0.1
sol = solve(prob, integrator, dt=Δt)
@time sol = solve(prob, integrator, dt=Δt)
sleep(0.1)
plotsolenergy(H, integrator, Δt, sol)
```
## Comparison of symplectic Integrators
```julia
SymplecticIntegrators = [
SymplecticEuler(),
VelocityVerlet(),
VerletLeapfrog(),
PseudoVerletLeapfrog(),
McAte2(),
Ruth3(),
McAte3(),
CandyRoz4(),
McAte4(),
CalvoSanz4(),
McAte42(),
McAte5(),
Yoshida6(),
KahanLi6(),
McAte8(),
KahanLi8(),
SofSpa10(),
]
k = 0.999
Δt = 0.1
for integrator in SymplecticIntegrators
singlependulum(k, integrator, Δt)
end
```
```julia
k = 0.999
Δt = 0.01
for integrator in SymplecticIntegrators[1:4]
singlependulum(k, integrator, Δt)
end
```
```julia
k = 0.999
Δt = 0.001
singlependulum(k, SymplecticEuler(), Δt)
```
```julia
k = 0.999
Δt = 0.0001
singlependulum(k, SymplecticEuler(), Δt)
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 2711 | ---
title: Diffusion Model
author: Samuel Isaacson, Chris Rackauckas
weave_options:
fig_ext : ".png"
---
```julia
using Catalyst, JumpProcesses, JumpProblemLibrary, Plots, Statistics, DataFrames
```
# Model and example solutions
Here we implement a 1D continuous time random walk approximation of diffusion
for $N$ lattice sites on $\left[0,1\right]$, with reflecting boundary conditions
at $x=0$ and $x=1$. Note that our goal is to benchmark the non-spatial
well-mixed stochastic simulation algorithms (SSAs), so we do not benchmark the
spatial SSAs too here.
```julia
N = 256
h = 1 / N
u0 = 10 * ones(Int64, N)
tf = .01
methods = (Direct(), FRM(), SortingDirect(), NRM(), DirectCR(), RSSA(), RSSACR(), Coevolve())
shortlabels = [string(leg)[15:end-2] for leg in methods]
jprob = JumpProblemLibrary.prob_jump_diffnetwork
rn = jprob.network(N)
prob = DiscreteProblem(rn, u0, (0.0, tf), [1 / (h*h)])
ploth = plot(reuse=false)
for (i,method) in enumerate(methods)
println("Benchmarking method: ", method)
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
sol = solve(jump_prob, SSAStepper(); saveat=tf/1000.)
plot!(ploth, sol.t, sol[Int(N//2),:], label=shortlabels[i])
end
plot!(ploth, title="Population at middle lattice site", xlabel="time")
```
# Benchmarking performance of the methods
```julia
function run_benchmark!(t, jump_prob, stepper)
sol = solve(jump_prob, stepper)
@inbounds for i in 1:length(t)
t[i] = @elapsed (sol = solve(jump_prob, stepper))
end
end
```
```julia
nsims = 50
benchmarks = Vector{Vector{Float64}}()
for method in methods
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
stepper = SSAStepper()
t = Vector{Float64}(undef, nsims)
run_benchmark!(t, jump_prob, stepper)
push!(benchmarks, t)
end
```
```julia
medtimes = Vector{Float64}(undef,length(methods))
stdtimes = Vector{Float64}(undef,length(methods))
avgtimes = Vector{Float64}(undef,length(methods))
for i in 1:length(methods)
medtimes[i] = median(benchmarks[i])
avgtimes[i] = mean(benchmarks[i])
stdtimes[i] = std(benchmarks[i])
end
df = DataFrame(names=shortlabels, medtimes=medtimes, relmedtimes=(medtimes/medtimes[1]),
avgtimes=avgtimes, std=stdtimes, cv=stdtimes./avgtimes)
```
# Plotting
```julia
sa = [string(round(mt,digits=4),"s") for mt in df.medtimes]
bar(df.names, df.relmedtimes, legend=:false)
scatter!(df.names, .05 .+ df.relmedtimes, markeralpha=0, series_annotations=sa)
ylabel!("median relative to Direct")
title!("256 Site 1D Diffusion CTRW")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 3110 | ---
title: Mendes Multistate Model
author: Samuel Isaacson, Chris Rackauckas
weave_options:
fig_ext : ".png"
---
Taken from Gupta and Mendes, *An Overview of Network-Based and -Free Approaches for Stochastic Simulation of Biochemical Systems*, Computation, 6 (9), 2018.
```julia
using Catalyst, JumpProcesses, JumpProblemLibrary, Plots, Statistics
fmt = :png
```
Our model is
```julia
jprob = JumpProblemLibrary.prob_jump_multistate
rn = jprob.network
reactions(rn)
```
# Plot solutions by each method
```julia
methods = (Direct(), FRM(), SortingDirect(), NRM(), DirectCR(), RSSA(), RSSACR(), Coevolve())
shortlabels = [string(leg)[15:end-2] for leg in methods]
tf = 10.0 * jprob.tstop
prob = DiscreteProblem(rn, jprob.u0, (0.0, tf), jprob.rates)
varlegs = ["A_P" "A_bound_P" "A_unbound_P" "RLA_P"]
@variables t S7(t) S8(t) S9(t)
varsyms = [
[S7,S8,S9],
[S9],
[S7,S8],
[S7]
]
varidxs = []
for vars in varsyms
push!(varidxs, [findfirst(isequal(sym),rn.states) for sym in vars])
end
```
```julia
p = []
for (i,method) in enumerate(methods)
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
sol = solve(jump_prob, SSAStepper(), saveat=tf/1000.)
solv = zeros(1001, 4)
for (i,varidx) in enumerate(varidxs)
solv[:,i] = sum(sol[varidx,:], dims=1)
end
if i < length(methods)
push!(p, plot(sol.t, solv, title=shortlabels[i], legend=false, format=fmt))
else
push!(p, plot(sol.t, solv, title=shortlabels[i], legend=false, format=fmt))
end
end
push!(p, plot((1:4)', framestyle = :none, legend=:inside, labels=varlegs))
plot(p..., layout=(5,2), format=fmt)
```
# Benchmarking performance of the methods
```julia
function run_benchmark!(t, jump_prob, stepper)
sol = solve(jump_prob, stepper)
@inbounds for i in 1:length(t)
t[i] = @elapsed (sol = solve(jump_prob, stepper))
end
end
```
```julia
nsims = 100
benchmarks = Vector{Vector{Float64}}()
for method in methods
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
stepper = SSAStepper()
time = Vector{Float64}(undef, nsims)
run_benchmark!(time, jump_prob, stepper)
push!(benchmarks, time)
end
```
```julia
medtimes = Vector{Float64}(undef,length(methods))
stdtimes = Vector{Float64}(undef,length(methods))
avgtimes = Vector{Float64}(undef,length(methods))
for i in 1:length(methods)
medtimes[i] = median(benchmarks[i])
avgtimes[i] = mean(benchmarks[i])
stdtimes[i] = std(benchmarks[i])
end
using DataFrames
df = DataFrame(names=shortlabels, medtimes=medtimes, relmedtimes=(medtimes/medtimes[1]),
avgtimes=avgtimes, std=stdtimes, cv=stdtimes./avgtimes)
sa = [text(string(round(mt,digits=3),"s"),:center,12) for mt in df.medtimes]
bar(df.names,df.relmedtimes,legend=:false, fmt=fmt)
scatter!(df.names, .05 .+ df.relmedtimes, markeralpha=0, series_annotations=sa, fmt=fmt)
ylabel!("median relative to Direct")
title!("Multistate Model")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 23208 | ---
title: Multivariate Hawkes Model
author: Guilherme Zagatti
weave_options:
fig_ext : ".png"
---
```julia
using JumpProcesses, Graphs, Statistics, BenchmarkTools, Plots
using OrdinaryDiffEq: Tsit5
fmt = :png
width_px, height_px = default(:size);
```
# Model and example solutions
Let a graph with $V$ nodes, then the multivariate Hawkes process is characterized by $V$ point processes such that the conditional intensity rate of node $i$ connected to a set of nodes $E_i$ in the graph is given by:
$$
\lambda_i^\ast (t) = \lambda + \sum_{j \in E_i} \sum_{t_{n_j} < t} \alpha \exp \left[-\beta (t - t_{n_j}) \right]
$$
This process is known as self-exciting, because the occurence of an event $ j $ at $t_{n_j}$ will increase the conditional intensity of all the processes connected to it by $\alpha$. The excited intensity then decreases at a rate proportional to $\beta$.
The conditional intensity of this process has a recursive formulation which can significantly speed the simulation. The recursive formulation for the univariate case is derived in Laub et al. [2]. We derive the compound case here. Let $t_{N_i} = \max \{ t_{n_j} < t \mid j \in E_i \}$ and
$$
\begin{split}
\phi_i^\ast (t)
&= \sum_{j \in E_i} \sum_{t_{n_j} < t} \alpha \exp \left[-\beta (t - t_{N_i} + t_{N_i} - t_{n_j}) \right] \\
&= \exp \left[ -\beta (t - t_{N_i}) \right] \sum_{j \in E_i} \sum_{t_{n_j} \leq t_{N_i}} \alpha \exp \left[-\beta (t_{N_i} - t_{n_j}) \right] \\
&= \exp \left[ -\beta (t - t_{N_i}) \right] \left( \alpha + \phi^\ast (t_{N_i}) \right)
\end{split}
$$
Then the conditional intensity can be re-written in terms of $\phi_i^\ast (t_{N_i})$
$$
\lambda_i^\ast (t) = \lambda + \phi_i^\ast (t) = \lambda + \exp \left[ -\beta (t - t_{N_i}) \right] \left( \alpha + \phi_i^\ast (t_{N_i}) \right)
$$
In Julia, we define a factory for the conditional intensity $\lambda_i$ which returns the brute-force or recursive versions of the intensity given node $i$ and network $g$.
```julia
function hawkes_rate(i::Int, g; use_recursion = false)
@inline @inbounds function rate_recursion(u, p, t)
λ, α, β, h, urate, ϕ = p
urate[i] = λ + exp(-β*(t - h[i]))*ϕ[i]
return urate[i]
end
@inline @inbounds function rate_brute(u, p, t)
λ, α, β, h, urate = p
x = zero(typeof(t))
for j in g[i]
for _t in reverse(h[j])
ϕij = α * exp(-β * (t - _t))
if ϕij ≈ 0
break
end
x += ϕij
end
end
urate[i] = λ + x
return urate[i]
end
if use_recursion
return rate_recursion
else
return rate_brute
end
end
```
Given the rate factory, we can create a jump factory which will create all the jumps in our model.
```julia
function hawkes_jump(i::Int, g; use_recursion = false)
rate = hawkes_rate(i, g; use_recursion)
urate = rate
@inbounds rateinterval(u, p, t) = p[5][i] == p[1] ? typemax(t) : 1 / (2*p[5][i])
@inbounds lrate(u, p, t) = p[1]
@inbounds function affect_recursion!(integrator)
λ, α, β, h, _, ϕ = integrator.p
for j in g[i]
ϕ[j] *= exp(-β*(integrator.t - h[j]))
ϕ[j] += α
h[j] = integrator.t
end
integrator.u[i] += 1
end
@inbounds function affect_brute!(integrator)
push!(integrator.p[4][i], integrator.t)
integrator.u[i] += 1
end
return VariableRateJump(
rate,
use_recursion ? affect_recursion! : affect_brute!;
lrate,
urate,
rateinterval,
)
end
function hawkes_jump(u, g; use_recursion = false)
return [hawkes_jump(i, g; use_recursion) for i = 1:length(u)]
end
```
We can then create a factory for Multivariate Hawkes `JumpProblem`s. We can define two types of `JumpProblem`s depending on the aggregator. The `Direct()` aggregator expects an `ODEProblem` since it cannot handle the `SSAStepper` with `VariableRateJump`s.
```julia
function f!(du, u, p, t)
du .= 0
nothing
end
function hawkes_problem(
p,
agg;
u = [0.0],
tspan = (0.0, 50.0),
save_positions = (false, true),
g = [[1]],
use_recursion = false,
)
oprob = ODEProblem(f!, u, tspan, p)
jumps = hawkes_jump(u, g; use_recursion)
jprob = JumpProblem(oprob, agg, jumps...; save_positions = save_positions)
return jprob
end
```
The `Coevolve()` aggregator knows how to handle the `SSAStepper`, so it accepts a `DiscreteProblem`.
```julia
function hawkes_problem(
p,
agg::Coevolve;
u = [0.0],
tspan = (0.0, 50.0),
save_positions = (false, true),
g = [[1]],
use_recursion = false,
)
dprob = DiscreteProblem(u, tspan, p)
jumps = hawkes_jump(u, g; use_recursion)
jprob =
JumpProblem(dprob, agg, jumps...; dep_graph = g, save_positions = save_positions)
return jprob
end
```
Lets solve the problems defined so far. We sample a random graph sampled from the Erdős-Rényi model. This model assumes that the probability of an edge between two nodes is independent of other edges, which we fix at $0.2$. For illustration purposes, we fix $V = 10$.
```julia
V = 10
G = erdos_renyi(V, 0.2, seed = 9103)
g = [neighbors(G, i) for i = 1:nv(G)]
```
We fix the Hawkes parameters at $\lambda = 0.5 , \alpha = 0.1 , \beta = 2.0$ which ensures the process does not explode.
```julia
tspan = (0.0, 50.0)
u = [0.0 for i = 1:nv(G)]
p = (0.5, 0.1, 2.0)
```
Now, we instantiate the problems, find their solutions and plot the results.
```julia
algorithms = Tuple{Any, Any, Bool, String}[
(Direct(), Tsit5(), false, "Direct (brute-force)"),
(Coevolve(), SSAStepper(), false, "Coevolve (brute-force)"),
(Direct(), Tsit5(), true, "Direct (recursive)"),
(Coevolve(), SSAStepper(), true, "Coevolve (recursive)"),
]
let fig = []
for (i, (algo, stepper, use_recursion, label)) in enumerate(algorithms)
if use_recursion
h = zeros(eltype(tspan), nv(G))
urate = zeros(eltype(tspan), nv(G))
ϕ = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, ϕ, urate)
else
h = [eltype(tspan)[] for _ = 1:nv(G)]
urate = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, urate)
end
jump_prob = hawkes_problem(_p, algo; u, tspan, g, use_recursion)
sol = solve(jump_prob, stepper)
push!(fig, plot(sol.t, sol[1:V, :]', title=label, legend=false, format=fmt))
end
fig = plot(fig..., layout=(2,2), format=fmt)
end
```
## Alternative libraries
We benchmark `JumpProcesses.jl` against `PiecewiseDeterministicMarkovProcesses.jl` and Python `Tick` library.
In order to compare with the `PiecewiseDeterministicMarkovProcesses.jl`, we need to reformulate our jump problem as a Piecewise Deterministic Markov Process (PDMP). In this setting, we need to describe how the conditional intensity changes with time which we derive below:
$$
\begin{split}
\frac{d \lambda_i^\ast (t)}{d t}
&= -\beta \sum_{j \in E_i} \sum_{t_{n_j} < t} \alpha \exp \left[-\beta (t - t_{n_j}) \right] \\
&= -\beta \left( \lambda_i^\ast (t) - \lambda \right)
\end{split}
$$
```julia
function hawkes_drate(dxc, xc, xd, p, t)
λ, α, β, _, _, g = p
for i = 1:length(g)
dxc[i] = -β * (xc[i] - λ)
end
end
```
Next, we need to define the intensity rate and the jumps according to library's specification.
```julia
function hawkes_rate(rate, xc, xd, p, t, issum::Bool)
λ, α, β, _, _, g = p
if issum
return sum(@view(xc[1:length(g)]))
end
rate[1:length(g)] .= @view xc[1:length(g)]
return 0.0
end
function hawkes_affect!(xc, xd, p, t, i::Int64)
λ, α, β, _, _, g = p
for j in g[i]
xc[i] += α
end
end
```
Finally, we create a factory for the Multivariate Hawkes `PDMPCHV` problem.
```julia
import LinearAlgebra: I
using PiecewiseDeterministicMarkovProcesses
const PDMP = PiecewiseDeterministicMarkovProcesses
struct PDMPCHV end
function hawkes_problem(
p,
agg::PDMPCHV;
u = [0.0],
tspan = (0.0, 50.0),
save_positions = (false, true),
g = [[1]],
use_recursion = true,
)
xd0 = Array{Int}(u)
xc0 = [p[1] for i = 1:length(u)]
nu = one(eltype(xd0)) * I(length(xd0))
jprob = PDMPProblem(hawkes_drate, hawkes_rate, hawkes_affect!, nu, xc0, xd0, p, tspan)
return jprob
end
push!(algorithms, (PDMPCHV(), CHV(Tsit5()), true, "PDMPCHV"));
```
The Python `Tick` library can be accessed with the `PyCall.jl`. We install the required Python dependencies with `Conda.jl` and define a factory for the Multivariate Hawkes `PyTick` problem.
```julia
const BENCHMARK_PYTHON = false
const REBUILD_PYCALL = false
struct PyTick end
if BENCHMARK_PYTHON
if REBUILD_PYCALL
using Pkg, Conda
# rebuild PyCall to ensure it links to the python provided by Conda.jl
ENV["PYTHON"] = ""
Pkg.build("PyCall")
# PyCall only works with Conda.ROOTENV
# tick requires python=3.8
Conda.add("python=3.8", Conda.ROOTENV)
Conda.add("numpy", Conda.ROOTENV)
Conda.pip_interop(true, Conda.ROOTENV)
Conda.pip("install", "tick", Conda.ROOTENV)
end
using PyCall
function hawkes_problem(
p,
agg::PyTick;
u = [0.0],
tspan = (0.0, 50.0),
save_positions = (false, true),
g = [[1]],
use_recursion = true,
)
λ, α, β = p
SimuHawkesSumExpKernels = pyimport("tick.hawkes")[:SimuHawkesSumExpKernels]
jprob = SimuHawkesSumExpKernels(
baseline = fill(λ, length(u)),
adjacency = [i in j ? α / β : 0.0 for j in g, i = 1:length(u), u = 1:1],
decays = [β],
end_time = tspan[2],
verbose = false,
force_simulation = true,
)
return jprob
end
push!(algorithms, (PyTick(), nothing, true, "PyTick"));
end
```
Now, we instantiate the problems, find their solutions and plot the results.
```julia
let fig = []
for (i, (algo, stepper, use_recursion, label)) in enumerate(algorithms[5:end])
if typeof(algo) <: PyTick
_p = (p[1], p[2], p[3])
jump_prob = hawkes_problem(_p, algo; u, tspan, g, use_recursion)
jump_prob.reset()
jump_prob.simulate()
t = tspan[1]:0.1:tspan[2]
N = [[sum(jumps .< _t) for _t in t] for jumps in jump_prob.timestamps]
push!(fig, plot(t, N, title=label, legend=false, format=fmt))
elseif typeof(algo) <: PDMPCHV
_p = (p[1], p[2], p[3], nothing, nothing, g)
jump_prob = hawkes_problem(_p, algo; u, tspan, g, use_recursion)
sol = solve(jump_prob, stepper)
push!(fig, plot(sol.time, sol.xd[1:V, :]', title=label, legend=false, format=fmt))
end
end
fig = plot(fig..., layout=(1,2), format=fmt, size=(width_px, height_px/2))
end
```
# Correctness: QQ-Plots
We check that the algorithms produce correct simulation by inspecting their QQ-plots. Point process theory says that transforming the simulated points using the compensator should produce points whose inter-arrival duration is distributed according to the exponential distribution (see Section 7.4 [1]).
The compensator of any point process is the integral of the conditional intensity $\Lambda_i^\ast(t) = \int_0^t \lambda_i^\ast(u) du$. The compensator for the Multivariate Hawkes process is defined below.
$$
\Lambda_i^\ast(t) = \lambda t + \frac{\alpha}{\beta} \sum_{j \in E_i} \sum_{t_{n_j} < t} ( 1 - \exp \left[-\beta (t - t_{n_j}) \right])
$$
```julia
function hawkes_Λ(i::Int, g, p)
@inline @inbounds function Λ(t, h)
λ, α, β = p
x = λ * t
for j in g[i]
for _t in h[j]
if _t >= t
break
end
x += (α / β) * (1 - exp(-β * (t - _t)))
end
end
return x
end
return Λ
end
function hawkes_Λ(g, p)
return [hawkes_Λ(i, g, p) for i = 1:length(g)]
end
Λ = hawkes_Λ(g, p)
```
We need a method for extracting the history from a simulation run. Below, we define such functions for each type of algorithm.
```julia
"""
Given an ODE solution `sol`, recover the timestamp in which events occurred. It
returns a vector with the history of each process in `sol`.
It assumes that `JumpProblem` was initialized with `save_positions` equal to
`(true, false)`, `(false, true)` or `(true, true)` such the system's state is
saved before and/or after the jump occurs; and, that `sol.u` is a
non-decreasing series that counts the total number of events observed as a
function of time.
"""
function histories(u, t)
_u = permutedims(reduce(hcat, u))
k = size(_u)[2]
# computes a mask that show when total counts change
mask = cat(fill(0.0, 1, k), _u[2:end, :] .- _u[1:end-1, :], dims = 1) .≈ 1
h = Vector{typeof(t)}(undef, k)
@inbounds for i = 1:k
h[i] = t[mask[:, i]]
end
return h
end
function histories(sol::S) where {S<:ODESolution}
# get u and permute the dimensions to get a matrix n x k with n obsevations and k processes.
if typeof(sol.u[1]) <: ExtendedJumpArray
u = map((u) -> u.u, sol.u)
else
u = sol.u
end
return histories(u, sol.t)
end
function histories(sol::S) where {S<:PDMP.PDMPResult}
return histories(sol.xd.u, sol.time)
end
function histories(sols)
map(histories, sols)
end
```
We also need to compute the quantiles of the empirical distribution given a history of events `hs`, the compensator `Λ` and the target quantiles `quant`.
```julia
import Distributions: Exponential
"""
Computes the empirical and expected quantiles given a history of events `hs`,
the compensator `Λ` and the target quantiles `quant`.
The history `hs` is a vector with the history of each process. Alternatively,
the function also takes a vector of histories containing the histories from
multiple runs.
The compensator `Λ` can either be an homogeneous compensator function that
equally applies to all the processes in `hs`. Alternatively, it accepts a
vector of compensator that applies to each process.
"""
function qq(hs, Λ, quant = 0.01:0.01:0.99)
_hs = apply_Λ(hs, Λ)
T = typeof(hs[1][1][1])
Δs = Vector{Vector{T}}(undef, length(hs[1]))
for k = 1:length(Δs)
_Δs = Vector{Vector{T}}(undef, length(hs))
for i = 1:length(_Δs)
_Δs[i] = _hs[i][k][2:end] .- _hs[i][k][1:end-1]
end
Δs[k] = reduce(vcat, _Δs)
end
empirical_quant = map((_Δs) -> quantile(_Δs, quant), Δs)
expected_quant = quantile(Exponential(1.0), quant)
return empirical_quant, expected_quant
end
"""
Compute the compensator `Λ` value for each timestamp recorded in history `hs`.
The history `hs` is a vector with the history of each process. Alternatively,
the function also takes a vector of histories containing the histories from
multiple runs.
The compensator `Λ` can either be an homogeneous compensator function that
equally applies to all the processes in `hs`. Alternatively, it accepts a
vector of compensator that applies to each process.
"""
function apply_Λ(hs::V, Λ) where {V<:Vector{<:Number}}
_hs = similar(hs)
@inbounds for n = 1:length(hs)
_hs[n] = Λ(hs[n], hs)
end
return _hs
end
function apply_Λ(k::Int, hs::V, Λ::A) where {V<:Vector{<:Vector{<:Number}},A<:Array}
@inbounds hsk = hs[k]
@inbounds Λk = Λ[k]
_hs = similar(hsk)
@inbounds for n = 1:length(hsk)
_hs[n] = Λk(hsk[n], hs)
end
return _hs
end
function apply_Λ(hs::V, Λ) where {V<:Vector{<:Vector{<:Number}}}
_hs = similar(hs)
@inbounds for k = 1:length(_hs)
_hs[k] = apply_Λ(hs[k], Λ)
end
return _hs
end
function apply_Λ(hs::V, Λ::A) where {V<:Vector{<:Vector{<:Number}},A<:Array}
_hs = similar(hs)
@inbounds for k = 1:length(_hs)
_hs[k] = apply_Λ(k, hs, Λ)
end
return _hs
end
function apply_Λ(hs::V, Λ) where {V<:Vector{<:Vector{<:Vector{<:Number}}}}
return map((_hs) -> apply_Λ(_hs, Λ), hs)
end
```
We can construct QQ-plots with a Plot recipe as following.
```julia
@userplot QQPlot
@recipe function f(x::QQPlot)
empirical_quant, expected_quant = x.args
max_empirical_quant = maximum(maximum, empirical_quant)
max_expected_quant = maximum(expected_quant)
upperlim = ceil(maximum([max_empirical_quant, max_expected_quant]))
@series begin
seriestype := :line
linecolor := :lightgray
label --> ""
(x) -> x
end
@series begin
seriestype := :scatter
aspect_ratio := :equal
xlims := (0.0, upperlim)
ylims := (0.0, upperlim)
xaxis --> "Expected"
yaxis --> "Empirical"
markerstrokewidth --> 0
markerstrokealpha --> 0
markersize --> 1.5
size --> (400, 500)
label --> permutedims(["quantiles $i" for i = 1:length(empirical_quant)])
expected_quant, empirical_quant
end
end
```
Now, we simulate all of the algorithms we defined in the previous Section $250$ times to produce their QQ-plots.
```julia
let fig = []
for (i, (algo, stepper, use_recursion, label)) in enumerate(algorithms)
if typeof(algo) <: PyTick
_p = (p[1], p[2], p[3])
elseif typeof(algo) <: PDMPCHV
_p = (p[1], p[2], p[3], nothing, nothing, g)
else
if use_recursion
h = zeros(eltype(tspan), nv(G))
ϕ = zeros(eltype(tspan), nv(G))
urate = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, urate, ϕ)
else
h = [eltype(tspan)[] for _ = 1:nv(G)]
urate = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, urate)
end
end
jump_prob = hawkes_problem(_p, algo; u, tspan, g, use_recursion)
runs = Vector{Vector{Vector{Number}}}(undef, 250)
for n = 1:length(runs)
if typeof(algo) <: PyTick
jump_prob.reset()
jump_prob.simulate()
runs[n] = jump_prob.timestamps
else
if ~(typeof(algo) <: PDMPCHV)
if use_recursion
h .= 0
ϕ .= 0
else
for _h in h empty!(_h) end
end
urate .= 0
end
runs[n] = histories(solve(jump_prob, stepper))
end
end
qqs = qq(runs, Λ)
push!(fig, qqplot(qqs..., legend = false, aspect_ratio = :equal, title=label, fmt=fmt))
end
fig = plot(fig..., layout = (3, 2), fmt=fmt, size=(width_px, 3*height_px/2))
end
```
# Benchmarking performance
In this Section we benchmark all the algorithms introduced in the first Section.
We generate networks in the range from $1$ to $95$ nodes and simulate the Multivariate Hawkes process $25$ units of time.
and simulate models in the range from $1$ to $95$ nodes for $25$ units of time. We fix the Hawkes parameters at $\lambda = 0.5 , \alpha = 0.1 , \beta = 5.0$ which ensures the process does not explode. We simulate $50$ trajectories with a limit of ten seconds to complete execution for each configuration.
```julia
tspan = (0.0, 25.0)
p = (0.5, 0.1, 5.0)
Vs = append!([1], 5:5:95)
Gs = [erdos_renyi(V, 0.2, seed = 6221) for V in Vs]
bs = Vector{Vector{BenchmarkTools.Trial}}()
for (algo, stepper, use_recursion, label) in algorithms
global _stepper = stepper
push!(bs, Vector{BenchmarkTools.Trial}())
_bs = bs[end]
for (i, G) in enumerate(Gs)
local g = [neighbors(G, i) for i = 1:nv(G)]
local u = [0.0 for i = 1:nv(G)]
if typeof(algo) <: PyTick
_p = (p[1], p[2], p[3])
elseif typeof(algo) <: PDMPCHV
_p = (p[1], p[2], p[3], nothing, nothing, g)
else
if use_recursion
global h = zeros(eltype(tspan), nv(G))
global urate = zeros(eltype(tspan), nv(G))
global ϕ = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, urate, ϕ)
else
global h = [eltype(tspan)[] for _ = 1:nv(G)]
global urate = zeros(eltype(tspan), nv(G))
_p = (p[1], p[2], p[3], h, urate)
end
end
global jump_prob = hawkes_problem(_p, algo; u, tspan, g, use_recursion)
trial = try
if typeof(algo) <: PyTick
@benchmark(
jump_prob.simulate(),
setup = (jump_prob.reset()),
samples = 50,
evals = 1,
seconds = 10,
)
else
if typeof(algo) <: PDMPCHV
@benchmark(
solve(jump_prob, _stepper),
setup = (),
samples = 50,
evals = 1,
seconds = 10,
)
else
if use_recursion
@benchmark(
solve(jump_prob, _stepper),
setup = (h .= 0; urate .= 0; ϕ .= 0),
samples = 50,
evals = 1,
seconds = 10,
)
else
@benchmark(
solve(jump_prob, _stepper),
setup = ([empty!(_h) for _h in h]; urate .= 0),
samples = 50,
evals = 1,
seconds = 10,
)
end
end
end
catch e
BenchmarkTools.Trial(
BenchmarkTools.Parameters(samples = 50, evals = 1, seconds = 10),
)
end
push!(_bs, trial)
if (nv(G) == 1 || nv(G) % 10 == 0)
median_time =
length(trial) > 0 ? "$(BenchmarkTools.prettytime(median(trial.times)))" :
"nan"
println("algo=$(label), V = $(nv(G)), length = $(length(trial.times)), median time = $median_time")
end
end
end
```
```julia
let fig = plot(
yscale = :log10,
xlabel = "V",
ylabel = "Time (ns)",
legend_position = :outertopright,
)
for (i, (algo, stepper, use_recursion, label)) in enumerate(algorithms)
_bs, _Vs = [], []
for (j, b) in enumerate(bs[i])
if length(b) == 50
push!(_bs, median(b.times))
push!(_Vs, Vs[j])
end
end
plot!(_Vs, _bs, label=label)
end
title!("Simulations, 50 samples: nodes × time")
end
```
# References
[1] D. J. Daley and D. Vere-Jones. An Introduction to the Theory of Point Processes: Volume I: Elementary Theory and Methods. Probability and Its Applications, An Introduction to the Theory of Point Processes. Springer-Verlag, 2 edition. doi:10.1007/b97277.
[2] Patrick J. Laub, Young Lee, and Thomas Taimre. The Elements of Hawkes Processes. Springer International Publishing. doi:10.1007/978-3-030-84639-8.
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 2478 | ---
title: Negative Feedback Gene Expression Model
author: Samuel Isaacson, Chris Rackauckas
weave_options:
fig_ext : ".png"
---
```julia
using JumpProcesses, Catalyst, JumpProblemLibrary, Plots, Statistics
import JumpProblemLibrary: prob_jump_dnarepressor
fmt = :png
```
Our model is
```julia
rn = prob_jump_dnarepressor.network
reactions(rn)
```
# Plot solutions by each method
```julia
methods = (Direct(), FRM(), SortingDirect(), NRM(), DirectCR(), RSSA(), RSSACR(), Coevolve())
shortlabels = [string(leg)[15:end-2] for leg in methods]
prob = prob_jump_dnarepressor.discrete_prob
tf = prob_jump_dnarepressor.tstop
ploth = plot(reuse=false)
for (i,method) in enumerate(methods)
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
sol = solve(jump_prob, SSAStepper(), saveat=tf/1000.)
plot!(ploth,sol.t, sol[3,:], label=shortlabels[i], format=fmt)
end
plot(ploth, title="Protein level", xlabel="time", format=fmt)
```
# Benchmarking performance of the methods
```julia
function run_benchmark!(t, jump_prob, stepper)
sol = solve(jump_prob, stepper)
@inbounds for i in 1:length(t)
t[i] = @elapsed (sol = solve(jump_prob, stepper))
end
end
```
```julia
nsims = 2000
benchmarks = Vector{Vector{Float64}}()
for method in methods
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
stepper = SSAStepper()
t = Vector{Float64}(undef,nsims)
run_benchmark!(t, jump_prob, stepper)
push!(benchmarks, t)
end
```
```julia
medtimes = Vector{Float64}(undef, length(methods))
stdtimes = Vector{Float64}(undef, length(methods))
avgtimes = Vector{Float64}(undef, length(methods))
for i in 1:length(methods)
medtimes[i] = median(benchmarks[i])
avgtimes[i] = mean(benchmarks[i])
stdtimes[i] = std(benchmarks[i])
end
println(medtimes/medtimes[1])
```
```julia
using DataFrames
df = DataFrame(names=shortlabels, medtimes=medtimes, relmedtimes=(medtimes/medtimes[1]),
avgtimes=avgtimes, std=stdtimes, cv=stdtimes./avgtimes)
sa = [text(string(round(mt,sigdigits=2),"s"),:center,10) for mt in df.medtimes]
bar(df.names,df.relmedtimes,legend=:false, fmt=fmt)
scatter!(df.names, .05 .+ df.relmedtimes, markeralpha=0, series_annotations=sa, fmt=fmt)
ylabel!("median relative to Direct")
title!("Negative Feedback Gene Expression Model")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 2853 | ---
title: Negative Feedback Marchetti Model
author: Samuel Isaacson, Chris Rackauckas
weave_options:
fig_ext : ".png"
---
```julia
using OrdinaryDiffEq, Catalyst, JumpProcesses, JumpProblemLibrary, Plots, Statistics
fmt = :png
```
# Model and example solutions
Here we implement the gene expression model from appendix A.6 of Marchetti,
Priami and Thanh, *Simulation Algorithms for Comptuational Systems Biology*,
Springer (2017).
```julia
jprob = JumpProblemLibrary.prob_jump_dnadimer_repressor
rnpar = jprob.rates
u0 = jprob.u0
tf = jprob.tstop
rn = jprob.network
reactions(rn)
```
```julia
u0f = [1000., 0., 0., 0., 0.]
odeprob = ODEProblem(rn, u0f, (0.,tf), rnpar)
solution = solve(odeprob, Tsit5())
plot(solution, format=fmt)
```
```julia
tf = 4000.
methods = (Direct(), FRM(), SortingDirect(), NRM(), DirectCR(), RSSA(), RSSACR(), Coevolve())
shortlabels = [string(leg)[15:end-2] for leg in methods]
prob = prob = DiscreteProblem(rn, u0, (0.0, tf), rnpar)
ploth = plot(reuse=false)
p = []
for (i,method) in enumerate(methods)
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
sol = solve(jump_prob, SSAStepper(), saveat=tf/1000.)
plot!(ploth, sol.t, sol[3,:], label=shortlabels[i], format=fmt)
push!(p, plot(sol, title=shortlabels[i], format=fmt))
end
plot(ploth, title="Protein level", xlabel="time", format=fmt)
```
```julia
plot(p[end])
```
# Benchmarking performance of the methods
```julia
function run_benchmark!(t, jump_prob, stepper)
sol = solve(jump_prob, stepper)
@inbounds for i in 1:length(t)
t[i] = @elapsed (sol = solve(jump_prob, stepper))
end
end
```
```julia
nsims = 200
benchmarks = Vector{Vector{Float64}}()
for method in methods
jump_prob = JumpProblem(rn, prob, method, save_positions=(false, false))
stepper = SSAStepper()
t = Vector{Float64}(undef, nsims)
run_benchmark!(t, jump_prob, stepper)
push!(benchmarks, t)
end
```
```julia
medtimes = Vector{Float64}(undef, length(methods))
stdtimes = Vector{Float64}(undef, length(methods))
avgtimes = Vector{Float64}(undef, length(methods))
for i in 1:length(methods)
medtimes[i] = median(benchmarks[i])
avgtimes[i] = mean(benchmarks[i])
stdtimes[i] = std(benchmarks[i])
end
using DataFrames
df = DataFrame(names=shortlabels, medtimes=medtimes, relmedtimes=(medtimes/medtimes[1]),
avgtimes=avgtimes, std=stdtimes, cv=stdtimes./avgtimes)
sa = [text(string(round(mt,digits=3),"s"),:center,12) for mt in df.medtimes]
bar(df.names,df.relmedtimes,legend=:false, fmt=fmt)
scatter!(df.names, .05 .+ df.relmedtimes, markeralpha=0, series_annotations=sa, fmt=fmt)
ylabel!("median relative to Direct")
title!("Marchetti Gene Expression Model")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 8607 | ---
title: Spatial Signaling Model from Sanft and Othmer (2015)
author: Vasily Ilin and Samuel Isaacson
weave_options:
fig_ext : ".png"
---
```julia
using Catalyst, JumpProcesses, BenchmarkTools, Plots, Random
```
# Model description and setup
Here we implement the model from [^1] (8 species and 12 reactions) for different
mesh sizes, and benchmark the performance of JumpProcesses.jl's spatial
stochastic simulation alorithms (SSAs). Below, the value `N` will denote the
number of subvolumes along one dimension of a cubic grid, representing the
reaction volume. In [^1] this value ranges from 20 to 60.
We first define some helper functions to convert concentration units into number
units, as needed for spatial SSAs.
```julia
invmicromolar_to_cubicmicrometer(invconcen) = invconcen / (6.02214076e2)
micromolar_to_invcubicmicrometer(concen) = (6.02214076e2) * concen
```
Next we create a well-mixed model with the desired chemistry
```julia
rn = @reaction_network begin
k₁, EA --> EA + A
k₁, EB --> EB + B
(ka,kd), EA + B <--> EAB
(ka,kd), EAB + B <--> EAB₂
(ka,kd), EB + A <--> EBA
(ka,kd), EBA + A <--> EBA₂
k₄, A --> ∅
k₄, B --> ∅
end k₁ ka kd k₄
```
Let's next make a function to calculate the spatial transport rates, mesh/graph
that will represent our domain, and initial condition. We use a cubic lattice of
size `N` by `N` by `N` with reflecting boundary conditions
```julia
# domain_len is the physical length of each side of the cubic domain
# units should be in μm (6.0 or 12.0 in Sanft)
# D is the diffusivity in units of (μm)^2 s⁻¹
function transport_model(rn, N; domain_len = 6.0, D = 1.0, rng = Random.default_rng())
# topology
h = domain_len / N
dims = (N, N, N)
num_nodes = prod(dims)
# Cartesian grid with reflecting BC at boundaries
grid = CartesianGrid(dims)
# Cartesian grid hopping rate to neighbors
hopping_rate = D / h^2
# this indicates we have a uniform rate of D/h^2 along each edge at each site
hopping_constants = hopping_rate * ones(numspecies(rn))
# figure out the indices of species EA and EB
@unpack EA, EB = rn
EAidx = findfirst(isequal(EA), species(rn))
EBidx = findfirst(isequal(EB), species(rn))
# spatial initial condition
# initial concentration of 12.3 nM = 12.3 * 1e-3 μM
num_molecules = trunc(Int, micromolar_to_invcubicmicrometer(12.3*1e-3) * (domain_len^3))
u0 = zeros(Int, 8, num_nodes)
rand_EA = rand(rng, 1:num_nodes, num_molecules)
rand_EB = rand(rng, 1:num_nodes, num_molecules)
for i in 1:num_molecules
u0[EAidx, rand_EA[i]] += 1
u0[EBidx, rand_EB[i]] += 1
end
grid, hopping_constants, h, u0
end
```
Finally, let's make a function to setup the well-mixed model from the reaction
model in a cube of side length `h`:
```julia
function wellmixed_model(rn, u0, end_time, h)
kaval = invmicromolar_to_cubicmicrometer(46.2) / h^3
setdefaults!(rn, [:k₁ => 150, :ka => kaval, :kd => 3.82, :k₄ => 6.0])
# well-mixed initial condition corresponding to the spatial initial condition
u0wm = sum(u0, dims = 2)
dprobwm = DiscreteProblem(rn, u0wm, (0.0, end_time))
jprobwm = JumpProblem(rn, dprobwm, Direct(), save_positions = (false,false))
majumps = jprobwm.massaction_jump
majumps, dprobwm, jprobwm, u0wm
end
```
# Model Solution
Let's look at one example to check our model seems reasonable. We'll plot the
total number of molecules in the system to verify we get around 28,000
molecules, as reported in Sanft [^1], when using a domain length of 6 μm.
```julia
end_time = 3.0
grid, hopping_constants, h, u0 = transport_model(rn, 60)
majumps, dprobwm, jprobwm, u0wm = wellmixed_model(rn, u0, end_time, 6.0)
sol = solve(jprobwm, SSAStepper(); saveat = end_time/200)
Ntot = [sum(u) for u in sol.u]
plt = plot(sol.t, Ntot, label="Well-mixed", ylabel="Total Number of Molecules",
xlabel="time")
# spatial model
majumps, dprobwm, jprobwm, u0wm = wellmixed_model(rn, u0, end_time, h)
dprob = DiscreteProblem(u0, (0.0, end_time), copy(dprobwm.p))
jprob = JumpProblem(dprob, DirectCRDirect(), majumps; hopping_constants,
spatial_system = grid, save_positions = (false, false))
spatial_sol = solve(jprob, SSAStepper(); saveat = end_time/200)
Ntot = [sum(vec(u)) for u in spatial_sol.u]
plot!(plt, spatial_sol.t, Ntot, label="Spatial",
title="Steady-state number of molecules is $(Ntot[end])")
```
# Benchmarking performance of the methods
We can now run the solvers and record the performance with `BenchmarkTools`.
Let's first create a `DiscreteCallback` to terminate simulations once we reach
`10^8` events:
```julia
@Base.kwdef mutable struct EventCallback
n::Int = 0
end
function (ecb::EventCallback)(u, t, integ)
ecb.n += 1
ecb.n == 10^8
end
function (ecb::EventCallback)(integ)
# save the final state
terminate!(integ)
nothing
end
```
We next create a function to run and return our benchmarking results.
```julia
function benchmark_and_save!(bench_dict, end_times, Nv, algs, domain_len)
@assert length(end_times) == length(Nv)
# callback for terminating simulations
ecb = EventCallback()
cb = DiscreteCallback(ecb, ecb)
for (end_time, N) in zip(end_times, Nv)
names = ["$s"[1:end-2] for s in algs]
grid, hopping_constants, h, u0 = transport_model(rn, N; domain_len)
# we create a well-mixed model within a domain of the size of *one* voxel, h
majumps, dprobwm, jprobwm, u0wm = wellmixed_model(rn, u0, end_time, h)
# the spatial problem
dprob = DiscreteProblem(u0, (0.0, end_time), copy(dprobwm.p))
@show N
# benchmarking and saving
benchmarks = Vector{BenchmarkTools.Trial}(undef, length(algs))
# callback for terminating simulations
for (i, alg) in enumerate(algs)
name = names[i]
println("benchmarking $name")
jp = JumpProblem(dprob, alg, majumps, hopping_constants=hopping_constants,
spatial_system = grid, save_positions=(false,false))
b = @benchmarkable solve($jp, SSAStepper(); saveat = $(dprob.tspan[2]), callback) setup = (callback = deepcopy($cb)) samples = 10 seconds = 3600
bench_dict[name, N] = run(b)
end
end
end
```
Finally, let's make a function to plot the benchmarking data.
```julia
function fetch_and_plot(bench_dict, domain_len)
names = unique([key[1] for key in keys(bench_dict)])
Nv = sort(unique([key[2] for key in keys(bench_dict)]))
plt1 = plot()
plt2 = plot()
medtimes = [Float64[] for i in 1:length(names)]
for (i,name) in enumerate(names)
for N in Nv
try
push!(medtimes[i], median(bench_dict[name, N]).time/1e9)
catch
break
end
end
len = length(medtimes[i])
plot!(plt1, Nv[1:len], medtimes[i], marker = :hex, label = name, lw = 2)
plot!(plt2, (Nv.^3)[1:len], medtimes[i], marker = :hex, label = name, lw = 2)
end
plot!(plt1, xlabel = "number of sites per edge", ylabel = "median time in seconds",
xticks = Nv, legend = :bottomright)
plot!(plt2, xlabel = "total number of sites", ylabel = "median time in seconds",
xticks = (Nv.^3, string.(Nv.^3)), legend = :bottomright)
plot(plt1, plt2; size = (1200,800), legendtitle = "SSAs",
plot_title="3D RDME, domain length = $domain_len", left_margin=5Plots.mm)
end
```
We are now ready to run the benchmarks and plot the results. We start with a
domain length of `12` μm, analogous to Fig. 6 in [^1]:
```julia
bench_dict = Dict{Tuple{String, Int}, BenchmarkTools.Trial}()
algs = [NSM(), DirectCRDirect()]
Nv = [20, 30, 40, 50, 60, 90, 120, 240, 360]
end_times = 20000.0 * ones(length(Nv))
domain_len = 12.0
benchmark_and_save!(bench_dict, end_times, Nv, algs, domain_len)
```
```julia
plt=fetch_and_plot(bench_dict, domain_len)
```
We next consider a domain of length `6` μm, analogous to Fig. 7 in [^1].
```julia
bench_dict = Dict{Tuple{String, Int}, BenchmarkTools.Trial}()
domain_len = 6.0
benchmark_and_save!(bench_dict, end_times, Nv, algs, domain_len)
```
```julia
plt=fetch_and_plot(bench_dict, domain_len)
```
# References
[^1]: Sanft, Kevin R and Othmer, Hans G. *Constant-complexity stochastic simulation algorithm with optimal binning*. J. Chem. Phys., 143(7), 11 pp. (2015).
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder], WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 20011 | ---
title: Filament Work-Precision Diagrams
author: dextorious, Chris Rackauckas
---
# Filament Benchmark
In this notebook we will benchmark a real-world biological model from a paper entitled [Magnetic dipole with a flexible tail as a self-propelling microdevice](https://doi.org/10.1103/PhysRevE.85.041502). This is a system of PDEs representing a Kirchhoff model of an elastic rod, where the equations of motion are given by the Rouse approximation with free boundary conditions.
## Model Implementation
First we will show the full model implementation. It is not necessary to understand the full model specification in order to understand the benchmark results, but it's all contained here for completeness. The model is highly optimized, with all internal vectors pre-cached, loops unrolled for efficiency (along with `@simd` annotations), a pre-defined Jacobian, matrix multiplications are all in-place, etc. Thus this model is a good stand-in for other optimized PDE solving cases.
The model is thus defined as follows:
```julia
using OrdinaryDiffEq, ODEInterfaceDiffEq, Sundials, DiffEqDevTools, LSODA
using LinearAlgebra
using Plots
gr()
```
```julia
const T = Float64
abstract type AbstractFilamentCache end
abstract type AbstractMagneticForce end
abstract type AbstractInextensibilityCache end
abstract type AbstractSolver end
abstract type AbstractSolverCache end
```
```julia
struct FerromagneticContinuous <: AbstractMagneticForce
ω :: T
F :: Vector{T}
end
mutable struct FilamentCache{
MagneticForce <: AbstractMagneticForce,
InextensibilityCache <: AbstractInextensibilityCache,
SolverCache <: AbstractSolverCache
} <: AbstractFilamentCache
N :: Int
μ :: T
Cm :: T
x :: SubArray{T,1,Vector{T},Tuple{StepRange{Int,Int}},true}
y :: SubArray{T,1,Vector{T},Tuple{StepRange{Int,Int}},true}
z :: SubArray{T,1,Vector{T},Tuple{StepRange{Int,Int}},true}
A :: Matrix{T}
P :: InextensibilityCache
F :: MagneticForce
Sc :: SolverCache
end
```
```julia
struct NoHydroProjectionCache <: AbstractInextensibilityCache
J :: Matrix{T}
P :: Matrix{T}
J_JT :: Matrix{T}
J_JT_LDLT :: LinearAlgebra.LDLt{T, SymTridiagonal{T}}
P0 :: Matrix{T}
NoHydroProjectionCache(N::Int) = new(
zeros(N, 3*(N+1)), # J
zeros(3*(N+1), 3*(N+1)), # P
zeros(N,N), # J_JT
LinearAlgebra.LDLt{T,SymTridiagonal{T}}(SymTridiagonal(zeros(N), zeros(N-1))),
zeros(N, 3*(N+1))
)
end
```
```julia
struct DiffEqSolverCache <: AbstractSolverCache
S1 :: Vector{T}
S2 :: Vector{T}
DiffEqSolverCache(N::Integer) = new(zeros(T,3*(N+1)), zeros(T,3*(N+1)))
end
```
```julia
function FilamentCache(N=20; Cm=32, ω=200, Solver=SolverDiffEq)
InextensibilityCache = NoHydroProjectionCache
SolverCache = DiffEqSolverCache
tmp = zeros(3*(N+1))
FilamentCache{FerromagneticContinuous, InextensibilityCache, SolverCache}(
N, N+1, Cm, view(tmp,1:3:3*(N+1)), view(tmp,2:3:3*(N+1)), view(tmp,3:3:3*(N+1)),
zeros(3*(N+1), 3*(N+1)), # A
InextensibilityCache(N), # P
FerromagneticContinuous(ω, zeros(3*(N+1))),
SolverCache(N)
)
end
```
```julia
function stiffness_matrix!(f::AbstractFilamentCache)
N, μ, A = f.N, f.μ, f.A
@inbounds for j in axes(A, 2), i in axes(A, 1)
A[i, j] = j == i ? 1 : 0
end
@inbounds for i in 1 : 3
A[i,i] = 1
A[i,3+i] = -2
A[i,6+i] = 1
A[3+i,i] = -2
A[3+i,3+i] = 5
A[3+i,6+i] = -4
A[3+i,9+i] = 1
A[3*(N-1)+i,3*(N-3)+i] = 1
A[3*(N-1)+i,3*(N-2)+i] = -4
A[3*(N-1)+i,3*(N-1)+i] = 5
A[3*(N-1)+i,3*N+i] = -2
A[3*N+i,3*(N-2)+i] = 1
A[3*N+i,3*(N-1)+i] = -2
A[3*N+i,3*N+i] = 1
for j in 2 : N-2
A[3*j+i,3*j+i] = 6
A[3*j+i,3*(j-1)+i] = -4
A[3*j+i,3*(j+1)+i] = -4
A[3*j+i,3*(j-2)+i] = 1
A[3*j+i,3*(j+2)+i] = 1
end
end
rmul!(A, -μ^4)
nothing
end
```
```julia
function update_separate_coordinates!(f::AbstractFilamentCache, r)
N, x, y, z = f.N, f.x, f.y, f.z
@inbounds for i in 1 : length(x)
x[i] = r[3*i-2]
y[i] = r[3*i-1]
z[i] = r[3*i]
end
nothing
end
function update_united_coordinates!(f::AbstractFilamentCache, r)
N, x, y, z = f.N, f.x, f.y, f.z
@inbounds for i in 1 : length(x)
r[3*i-2] = x[i]
r[3*i-1] = y[i]
r[3*i] = z[i]
end
nothing
end
function update_united_coordinates(f::AbstractFilamentCache)
r = zeros(T, 3*length(f.x))
update_united_coordinates!(f, r)
r
end
```
```julia
function initialize!(initial_conf_type::Symbol, f::AbstractFilamentCache)
N, x, y, z = f.N, f.x, f.y, f.z
if initial_conf_type == :StraightX
x .= range(0, stop=1, length=N+1)
y .= 0
z .= 0
else
error("Unknown initial configuration requested.")
end
update_united_coordinates(f)
end
```
```julia
function magnetic_force!(::FerromagneticContinuous, f::AbstractFilamentCache, t)
# TODO: generalize this for different magnetic fields as well
N, μ, Cm, ω, F = f.N, f.μ, f.Cm, f.F.ω, f.F.F
F[1] = -μ * Cm * cos(ω*t)
F[2] = -μ * Cm * sin(ω*t)
F[3*(N+1)-2] = μ * Cm * cos(ω*t)
F[3*(N+1)-1] = μ * Cm * sin(ω*t)
nothing
end
```
```julia
struct SolverDiffEq <: AbstractSolver end
function (f::FilamentCache)(dr, r, p, t)
@views f.x, f.y, f.z = r[1:3:end], r[2:3:end], r[3:3:end]
jacobian!(f)
projection!(f)
magnetic_force!(f.F, f, t)
A, P, F, S1, S2 = f.A, f.P.P, f.F.F, f.Sc.S1, f.Sc.S2
# implement dr = P * (A*r + F) in an optimized way to avoid temporaries
mul!(S1, A, r)
S1 .+= F
mul!(S2, P, S1)
copyto!(dr, S2)
return dr
end
```
```julia
function jacobian!(f::FilamentCache)
N, x, y, z, J = f.N, f.x, f.y, f.z, f.P.J
@inbounds for i in 1 : N
J[i, 3*i-2] = -2 * (x[i+1]-x[i])
J[i, 3*i-1] = -2 * (y[i+1]-y[i])
J[i, 3*i] = -2 * (z[i+1]-z[i])
J[i, 3*(i+1)-2] = 2 * (x[i+1]-x[i])
J[i, 3*(i+1)-1] = 2 * (y[i+1]-y[i])
J[i, 3*(i+1)] = 2 * (z[i+1]-z[i])
end
nothing
end
```
```julia
function projection!(f::FilamentCache)
# implement P[:] = I - J'/(J*J')*J in an optimized way to avoid temporaries
J, P, J_JT, J_JT_LDLT, P0 = f.P.J, f.P.P, f.P.J_JT, f.P.J_JT_LDLT, f.P.P0
mul!(J_JT, J, J')
LDLt_inplace!(J_JT_LDLT, J_JT)
ldiv!(P0, J_JT_LDLT, J)
mul!(P, P0', J)
subtract_from_identity!(P)
nothing
end
```
```julia
function subtract_from_identity!(A)
lmul!(-1, A)
@inbounds for i in 1 : size(A,1)
A[i,i] += 1
end
nothing
end
```
```julia
function LDLt_inplace!(L::LinearAlgebra.LDLt{T,SymTridiagonal{T}}, A::Matrix{T}) where {T<:Real}
n = size(A,1)
dv, ev = L.data.dv, L.data.ev
@inbounds for (i,d) in enumerate(diagind(A))
dv[i] = A[d]
end
@inbounds for (i,d) in enumerate(diagind(A,-1))
ev[i] = A[d]
end
@inbounds @simd for i in 1 : n-1
ev[i] /= dv[i]
dv[i+1] -= abs2(ev[i]) * dv[i]
end
L
end
```
# Investigating the model
Let's take a look at what results of the model look like:
```julia
function run(::SolverDiffEq; N=20, Cm=32, ω=200, time_end=1., solver=TRBDF2(autodiff=false), reltol=1e-6, abstol=1e-6)
f = FilamentCache(N, Solver=SolverDiffEq, Cm=Cm, ω=ω)
r0 = initialize!(:StraightX, f)
stiffness_matrix!(f)
prob = ODEProblem(ODEFunction(f, jac=(J, u, p, t)->(mul!(J, f.P.P, f.A); nothing)), r0, (0., time_end))
sol = solve(prob, solver, dense=false, reltol=reltol, abstol=abstol)
end
```
This method runs the model with the `TRBDF2` method and the default parameters.
```julia
sol = run(SolverDiffEq())
plot(sol,vars = (0,25))
```
The model quickly falls into a highly oscillatory mode which then dominates throughout the rest of the solution.
# Work-Precision Diagrams
Now let's build the problem and solve it once at high accuracy to get a reference solution:
```julia
N=20
f = FilamentCache(N, Solver=SolverDiffEq)
r0 = initialize!(:StraightX, f)
stiffness_matrix!(f)
prob = ODEProblem(f, r0, (0., 0.01))
sol = solve(prob, Vern9(), reltol=1e-14, abstol=1e-14)
test_sol = TestSolution(sol);
```
## Omissions
```julia;eval=false
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => Rosenbrock23(autodiff=false)),
Dict(:alg => Rodas4(autodiff=false)),
Dict(:alg => radau()),
Dict(:alg=>Exprb43(autodiff=false)),
Dict(:alg=>Exprb32(autodiff=false)),
Dict(:alg=>ImplicitEulerExtrapolation(autodiff=false)),
Dict(:alg=>ImplicitDeuflhardExtrapolation(autodiff=false)),
Dict(:alg=>ImplicitHairerWannerExtrapolation(autodiff=false)),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
Rosenbrock23, Rodas4, Exprb32, Exprb43, extrapolation methods, and Rodas5 do
not perform well at all and are thus dropped from future tests. For reference,
they are in the 10^(2.5) range in for their most accurate run (with
ImplicitEulerExtrapolation takes over a day to run, and had to be prematurely
stopped), so about 500x slower than CVODE_BDF and
thus make the benchmarks take forever. It looks like `radau` fails on this
problem with high tolerance so its values should be ignored since it exits
early. It is thus removed from the next sections.
The EPIRK methods currently do not work on this problem
```julia
sol = solve(prob, EPIRK4s3B(autodiff=false), dt=2^-3)
```
but would be called like:
```julia;eval=false
abstols=1 ./10 .^(3:5)
reltols=1 ./10 .^(3:5)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => HochOst4(),:dts=>2.0.^(-3:-1:-5)),
Dict(:alg => EPIRK4s3B(),:dts=>2.0.^(-3:-1:-5)),
Dict(:alg => EXPRB53s3(),:dts=>2.0.^(-3:-1:-5)),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
## High Tolerance (Low Accuracy)
### Endpoint Error
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => BS3()),
Dict(:alg => Tsit5()),
Dict(:alg => ImplicitEuler(autodiff=false)),
Dict(:alg => Trapezoid(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => rodas()),
Dict(:alg => dop853()),
Dict(:alg => lsoda()),
Dict(:alg => ROCK2()),
Dict(:alg => ROCK4()),
Dict(:alg => ESERK5())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => ImplicitEuler(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => ABDF2(autodiff=false)),
Dict(:alg => QNDF(autodiff=false)),
Dict(:alg => RadauIIA5(autodiff=false)),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => CVODE_BDF(linear_solver=:GMRES)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false,linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false,linsolve=LinSolveGMRES())),
];
names = [
"CVODE-BDF",
"CVODE-BDF (GMRES)",
"TRBDF2",
"TRBDF2 (GMRES)",
"KenCarp4",
"KenCarp4 (GMRES)",
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; names=names, appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
### Timeseries Error
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => Trapezoid(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => rodas()),
Dict(:alg => lsoda()),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => ROCK2()),
Dict(:alg => ROCK4()),
Dict(:alg => ESERK5())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
Timeseries errors seem to match final point errors very closely in this problem,
so these are turned off in future benchmarks.
(Confirmed in the other cases)
### Dense Error
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => ROCK2()),
Dict(:alg => ROCK4()),
Dict(:alg => ESERK5())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false, dense_errors = true, error_estimate=:L2)
plot(wp)
```
Dense errors seem to match timeseries errors very closely in this problem, so
these are turned off in future benchmarks.
(Confirmed in the other cases)
## Low Tolerance (High Accuracy)
```julia
abstols=1 ./10 .^(6:12)
reltols=1 ./10 .^(6:12)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => Vern7()),
Dict(:alg => Vern9()),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => dop853()),
Dict(:alg => ROCK4())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
```julia
abstols=1 ./10 .^(6:12)
reltols=1 ./10 .^(6:12)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => radau()),
Dict(:alg => RadauIIA5(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno5(autodiff=false)),
Dict(:alg => KenCarp5(autodiff=false)),
Dict(:alg => lsoda()),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
### Timeseries Error
```julia;eval=false
abstols=1 ./10 .^(6:12)
reltols=1 ./10 .^(6:12)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => radau()),
Dict(:alg => RadauIIA5(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno5(autodiff=false)),
Dict(:alg => KenCarp5(autodiff=false)),
Dict(:alg => lsoda()),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false, error_estimate = :l2)
plot(wp)
```
### Dense Error
```julia;eval=false
abstols=1 ./10 .^(6:12)
reltols=1 ./10 .^(6:12)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => radau()),
Dict(:alg => RadauIIA5(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno5(autodiff=false)),
Dict(:alg => KenCarp5(autodiff=false)),
Dict(:alg => lsoda()),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false, dense_errors=true, error_estimate = :L2)
plot(wp)
```
# No Jacobian Work-Precision Diagrams
In the previous cases the analytical Jacobian is given and is used by the solvers. Now we will solve the same problem without the analytical Jacobian.
Note that the pre-caching means that the model is not compatible with autodifferentiation by ForwardDiff. Thus all of the native Julia solvers are set to `autodiff=false` to use DiffEqDiffTools.jl's numerical differentiation backend. We'll only benchmark the methods that did well before.
```julia
N=20
f = FilamentCache(N, Solver=SolverDiffEq)
r0 = initialize!(:StraightX, f)
stiffness_matrix!(f)
prob = ODEProblem(ODEFunction(f, jac=nothing), r0, (0., 0.01))
sol = solve(prob, Vern9(), reltol=1e-14, abstol=1e-14)
test_sol = TestSolution(sol.t, sol.u);
```
## High Tolerance (Low Accuracy)
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => BS3()),
Dict(:alg => Tsit5()),
Dict(:alg => ImplicitEuler(autodiff=false)),
Dict(:alg => Trapezoid(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => rodas()),
Dict(:alg => dop853()),
Dict(:alg => lsoda())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => BS3()),
Dict(:alg => Tsit5()),
Dict(:alg => ImplicitEuler(autodiff=false)),
Dict(:alg => Trapezoid(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => rodas()),
Dict(:alg => dop853()),
Dict(:alg => lsoda()),
Dict(:alg => ROCK2()),
Dict(:alg => ROCK4()),
Dict(:alg => ESERK5())
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
```julia
abstols=1 ./10 .^(3:8)
reltols=1 ./10 .^(3:8)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => CVODE_BDF(linear_solver=:GMRES)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false,linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false,linsolve=LinSolveGMRES())),
];
names = [
"CVODE-BDF",
"CVODE-BDF (GMRES)",
"TRBDF2",
"TRBDF2 (GMRES)",
"KenCarp4",
"KenCarp4 (GMRES)",
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; names=names, appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
## Low Tolerance (High Accuracy)
```julia
abstols=1 ./10 .^(6:12)
reltols=1 ./10 .^(6:12)
setups = [
Dict(:alg => CVODE_BDF()),
Dict(:alg => radau()),
Dict(:alg => RadauIIA5(autodiff=false)),
Dict(:alg => TRBDF2(autodiff=false)),
Dict(:alg => Kvaerno3(autodiff=false)),
Dict(:alg => KenCarp3(autodiff=false)),
Dict(:alg => Kvaerno4(autodiff=false)),
Dict(:alg => KenCarp4(autodiff=false)),
Dict(:alg => Kvaerno5(autodiff=false)),
Dict(:alg => KenCarp5(autodiff=false)),
Dict(:alg => lsoda()),
];
wp = WorkPrecisionSet(prob, abstols, reltols, setups; appxsol=test_sol,
maxiters=Int(1e6), verbose = false)
plot(wp)
```
## Conclusion
Sundials' `CVODE_BDF` does the best in this test. When the Jacobian is given, the ESDIRK methods `TRBDF2` and `KenCarp3` are able to do almost as well as it until `<1e-6` error is needed. When Jacobians are not given, Sundials is the fastest without competition.
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 9072 | ---
title: Allen_Cahn FDM Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the Burgers equation using FDM.
```julia
# Define the linear and nonlinear terms
function lin_term(N)
dx = 1/(N + 1)
du = 1/2 * ones(N - 1) # super diagonal
dl = -1/2 * ones(N - 1) # lower diagonal
DiffEqArrayOperator(0.01*(1/dx) * diagm(-1 => dl, 1 => du))
end
function nl_term(N)
function (du,u,p,t)
du .= u .- u.^3
end
end
# Construct the problem
function allen_cahn(N)
f1 = lin_term(N)
f2 = nl_term(N)
dx = 1 / (N + 1)
xs = (1:N) * dx
f0 = x -> .53*x + .47*sin(-1.5*pi*x) - x
u0 = f0.(xs)
prob = SplitODEProblem(f1, f2, u0, (0.0, 1.0))
xs, prob
end;
```
Reference solution using Vern9 is below:
```julia
xs, prob = allen_cahn(100)
sol = solve(prob, RadauIIA5(autodiff=false); abstol=1e-14, reltol=1e-14, dt=1e-4, adaptive=false)
test_sol = TestSolution(sol);
tslices = [0.0 0.25 0.50 0.75 1.]
ys = hcat((sol(t) for t in tslices)...)
labels = ["t = $t" for t in tslices]
plot(xs, ys, label=labels)
```
Linear solvers
```julia
const LS_Dense = LinSolveFactorize(lu)
```
## High tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNLF2(), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, low order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => CNLF2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=5), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=20), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler (caching)", "NorsettEuler (m=5)", "NorsettEuler (m=20)",
"ETDRK2 (caching)", "ETDRK2 (m=5)", "ETDRK2 (m=20)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers)]
labels = ["CNAB2 (dense linsolve)" "CNAB2 (Krylov linsolve)" "ETDRK2 (m=5)"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3()),
Dict(:alg => KenCarp4()),
Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense))]
labels = hcat("KenCarp3", "KenCarp4", "KenCarp5", "ARKODE3", "ARKODE4", "ARKODE5")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, medium order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES))]
labels = ["KenCarp3" "KenCarp4" "KenCarp5" "ARKODE3" "ARKODE4" "ARKODE5"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK3 (m=5)", "ETDRK4 (caching)",
"ETDRK4 (m=5)", "HochOst4 (caching)", "HochOst4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense)),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES)),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("KenCarp5 (dense linsolve)", "ARKODE (dense linsolve)", "KenCarp5 (Krylov linsolve)",
"ARKODE (Krylov linsolve)", "ETDRK3 (m=5)", "ETDRK4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 9109 | ---
title: Allen-Cahn Pseudospectral Methods Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the Allen-Cahn equation using Chebyshev spectral methods.
```julia
function cheb(N)
N==0 && return (0,1)
x = cos.(pi*(0:N)/N)
c = [2; ones(N-1,1); 2].*(-1).^(0:N)
X = hcat([x for i in 1:N+1]...)
dX = X-X'
D = (c*(1 ./c)')./(dX+I) # off-diagonal entries
D = D .- Diagonal(vec(sum(D,dims=2))) # diagonal entries
D,x
end
N = 128
ChebD2,x = cheb(N)
xx = x
x = x[2:N]
w = .53*x + .47*sin.(-1.5*pi*x) - x # use w = u-x to make BCs homogeneous
u = [1;w+x;-1]
ϵ=0.01
D2=ϵ*(ChebD2^2)[2:N, 2:N]
function allen_cahn(du,u,x,t)
@. du = (u + x) - (u + x)^3
end
```
Reference solution using RadauIIA5 is below:
```julia
prob = SplitODEProblem(DiffEqArrayOperator(D2), allen_cahn, w, (0.0,5.0), x)
sol = solve(prob, RadauIIA5(autodiff=false); reltol=1e-14,abstol=1e-14)
test_sol = TestSolution(sol)
tslices=[0.0 1.0 2.0 3.0 5.0]
ys=hcat(([1;x.+sol(t);-1] for t in tslices)...)
labels=["t=$t" for t in tslices]
plot(xx,ys,label=labels)
```
## High tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (5:8)
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(), :dts => 5e-3 * multipliers),
Dict(:alg => CNLF2(), :dts => 5e-4 * multipliers),
Dict(:alg => SBDF2(), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp1 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true,names=labels,
numruns=5,seconds=5,
save_everystop=false,appxsol=test_sol,maxiters=Int(1e5));
plot(wp1,label=labels,markershape=:auto,title="IMEX methods, dense linsolve, low order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 5e-3 * multipliers),
Dict(:alg => CNLF2(linsolve=LinSolveGMRES()), :dts => 5e-4 * multipliers),
Dict(:alg => SBDF2(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp1 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp1, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=5), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=20), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler (caching)", "NorsettEuler (m=5)", "NorsettEuler (m=20)",
"ETDRK2 (caching)", "ETDRK2 (m=5)", "ETDRK2 (m=20)")
@time wp2 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp2, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(), :dts => 5e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 5e-3 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = ["CNAB2 (dense linsolve)" "CNAB2 (Krylov linsolve)" "ETDRK2 (m=5)"]
@time wp3 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp3, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (dense/band linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3()),
Dict(:alg => KenCarp4()),
Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1))]
labels = hcat("KenCarp3", "KenCarp4", "KenCarp5",
"ARKODE3", "ARKODE4", "ARKODE5")
@time wp4 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp4, label=labels, markershape=:auto, title="IMEX methods, dense/band linsolve, medium order")
```
1.IMEX methods (krylov linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES))]
labels = ["KenCarp3" "KenCarp4" "KenCarp5" "ARKODE3" "ARKODE4" "ARKODE5"]
@time wp4 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp4, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK3 (m=5)", "ETDRK4 (caching)",
"ETDRK4 (m=5)", "HochOst4 (caching)", "HochOst4 (m=5)")
@time wp5 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp5, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense)),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES)),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("KenCarp5 (dense linsolve)", "ARKODE (dense linsolve)", "KenCarp5 (Krylov linsolve)",
"ARKODE (Krylov linsolve)", "ETDRK3 (m=5)", "ETDRK4 (m=5)")
@time wp6 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp6, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 9190 | ---
title: Burgers FDM Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the Burgers equation using FDM.
```julia
function lin_term(N, ϵ)
dx = 1/(N + 1)
d = -2 * ones(N) # main diagonal
du = ones(N - 1) # off diagonal
DiffEqArrayOperator((ϵ/dx^2) * diagm(-1 => du, 0 => d, 1 => du))
end
function nl_term(N)
dx = 1/(N + 1)
du = ones(N - 1) # super diagonal
dl = -ones(N - 1) # lower diagonal
D = (-1/(4*dx)) * diagm(-1 => dl, 1 => du)
tmp = zeros(N)
function (du,u,p,t)
@. tmp = u^2
mul!(du, D, tmp)
end
end
# Construct the problem
function burgers(N, ϵ)
f1 = lin_term(N, ϵ)
f2 = nl_term(N)
dx = 1 / (N + 1)
xs = (1:N) * dx
μ0 = 0.3; σ0 = 0.05
f0 = x -> exp(-(x - μ0)^2 / (2 * σ0^2))
u0 = f0.(xs)
prob = SplitODEProblem(f1, f2, u0, (0.0, 1.0))
xs, prob
end;
```
Reference solution using Vern9 is below:
```julia
xs, prob = burgers(512, 1e-3)
sol = solve(prob, Vern9(); abstol=1e-14, reltol=1e-14)
test_sol = TestSolution(sol);
tslices = [0.0 0.25 0.50 0.75 1.00]
ys = hcat((sol(t) for t in tslices)...)
labels = ["t = $t" for t in tslices]
plot(xs, ys, label=labels)
```
## High tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNLF2(), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, low order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => CNLF2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(linsolve=LinSolveGMRES()), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=5), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=20), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers)]
#Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers)) matrix contains Inf or NaN
#Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers) matrix contains Inf or NaN
labels = hcat("NorsettEuler (caching)", "NorsettEuler (m=5)", "NorsettEuler (m=20)",
"ETDRK2 (caching)")#, "ETDRK2 (m=5)"), "ETDRK2 (m=20)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers)]
labels = ["CNAB2 (dense linsolve)" "CNAB2 (Krylov linsolve)" "ETDRK2 (m=5)"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (8:13)
reltols = 0.1 .^ (5:10)
setups = [Dict(:alg => KenCarp3()),
Dict(:alg => KenCarp4()),
Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense))]
labels = hcat("KenCarp3", "KenCarp4", "KenCarp5", "ARKODE3", "ARKODE4", "ARKODE5")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, medium order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (8:13)
reltols = 0.1 .^ (5:10)
setups = [Dict(:alg => KenCarp3(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES))]
labels = ["KenCarp3" "KenCarp4" "KenCarp5" "ARKODE3" "ARKODE4" "ARKODE5"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK3 (m=5)", "ETDRK4 (caching)",
"ETDRK4 (m=5)", "HochOst4 (caching)", "HochOst4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (8:13)
reltols = 0.1 .^ (5:10)
multipliers = 0.5 .^ (0:5)
setups = [Dict(:alg => KenCarp4()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense)),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES)),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("KenCarp4 (dense linsolve)", "ARKODE (dense linsolve)", "KenCarp4 (Krylov linsolve)",
"ARKODE (Krylov linsolve)", "ETDRK3 (m=5)", "ETDRK4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));#162s
plot(wp, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 6115 | ---
title: Burgers Pseudospectral Methods Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the Burgers equation using Fourier spectral methods.
```julia
S = Fourier()
n = 512
x = points(S, n)
D2 = Derivative(S,2)[1:n,1:n]
D = (Derivative(S) → S)[1:n,1:n]
T = ApproxFun.plan_transform(S, n)
Ti = ApproxFun.plan_itransform(S, n)
û₀ = T*cos.(cos.(x.-0.1))
A = 0.03*D2
tmp = similar(û₀)
p = (D,D2,T,Ti,tmp,similar(tmp))
function burgers_nl(dû,û,p,t)
D,D2,T,Ti,u,tmp = p
mul!(tmp, D, û)
mul!(u, Ti, tmp)
mul!(tmp, Ti, û)
@. tmp = tmp*u
mul!(u, T, tmp)
@. dû = - u
end
```
Reference solution using Rodas5 is below:
```julia
prob = SplitODEProblem(DiffEqArrayOperator(Diagonal(A)), burgers_nl, û₀, (0.0,5.0), p)
sol = solve(prob, Rodas5(autodiff=false); reltol=1e-12,abstol=1e-12)
test_sol = TestSolution(sol)
tslices=[0.0 1.0 2.0 3.0 5.0]
ys=hcat((Ti*sol(t) for t in tslices)...)
labels=["t=$t" for t in tslices]
plot(x,ys,label=labels)
```
## High tolerances
```julia
diag_linsolve=LinSolveFactorize(W->let tmp = tmp
for i in 1:size(W, 1)
tmp[i] = W[i, i]
end
Diagonal(tmp)
end)
```
## In-family comparisons
1.IMEX methods (diagonal linear solver)
```julia
abstols = 0.1 .^ (5:8)
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=diag_linsolve), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers),
Dict(:alg => CNLF2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers),
Dict(:alg => SBDF2(linsolve=diag_linsolve), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp1 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true,names=labels,
numruns=5,seconds=5,
save_everystop=false,appxsol=test_sol,maxiters=Int(1e5));
plot(wp1,label=labels,markershape=:auto,title="IMEX methods, diagonal linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler",
"ETDRK2 (caching)")
@time wp2 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp2, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = ["CNAB2 (diagonal linsolve)" "ETDRK2"]
@time wp3 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp3, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (band linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1))]
labels = hcat("ARKODE3", "ARKODE4", "ARKODE5")
@time wp4 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp4, label=labels, markershape=:auto, title="IMEX methods, band linsolve, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK4 (caching)",
"HochOst4 (caching)")
@time wp5 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp5, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers)]
labels = hcat("ARKODE (nondiagonal linsolve)", "ETDRK3 ()", "ETDRK4 ()")
#"ARKODE (Krylov linsolve)")
@time wp6 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp6, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 9412 | ---
title: KdV FDM Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the KdV equation using FDM.
```julia
# Define the linear and nonlinear terms
function lin_term(N)
dx = 1/(N + 1)
du = ones(N-1) # off diagonal
du2 = -ones(N-1) # off diagonal
d = (1/2) * ones(N-2)
d2 = (-1/2) * ones(N-2)
diag=-2 * ones(N)
DiffEqArrayOperator(5e-5*(1/dx^3) * diagm(-2 => d2, -1 => du, 1 => du2, 2 => d))
end
function nl_term(N)
dx = 1/(N + 1)
du = ones(N - 1) # super diagonal
dl = -ones(N - 1) # lower diagonal
D = (0.2/dx) * diagm(-1 => dl, 1 => du)
tmp = zeros(N)
function (du,u,p,t)
@. tmp = u^2
mul!(du, D, tmp)
end
end
# Construct the problem
function kdv(N)
f1 = lin_term(N)
f2 = nl_term(N)
dx = 1 / (N + 1)
xs = (1:N) * dx
μ0 = 0.3; σ0 = 0.05
f0 = x -> 0.9*exp(-(x - μ0)^2 / (2 * σ0^2))
u0 = f0.(xs)
prob = SplitODEProblem(f1, f2, u0, (0.0, 1.0))
xs, prob
end;
```
Reference solution using Tsit5 is below:
```julia
xs, prob = kdv(200)
sol = solve(prob, Tsit5(); abstol=1e-11, reltol=1e-11, dt=1e-7, adptive=false)
test_sol = TestSolution(sol);
tslices = [0.0 0.25 0.50 0.75 1.00]
ys = hcat((sol(t) for t in tslices)...)
labels = ["t = $t" for t in tslices]
fn=plot(xs, ys, label=labels)
```
Linear solvers
```julia
const LS_Dense = LinSolveFactorize(lu)
```
## High tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(), :dts => 1e-5 * multipliers),
Dict(:alg => CNLF2(), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, low order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [#Dict(:alg => IMEXEuler(linsolve=LinSolveGMRES()), :dts => 1e-5 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-5 * multipliers),
Dict(:alg => CNLF2(linsolve=LinSolveGMRES()), :dts => 1e-5 * multipliers),
Dict(:alg => SBDF2(linsolve=LinSolveGMRES()), :dts => 1e-4 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-6 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=5), :dts => 1e-3 * multipliers),
#Dict(:alg => NorsettEuler(krylov=true, m=20), :dts => 1e-3 * multipliers), matrix contains Infs or NaNs
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler (caching)", "NorsettEuler (m=5)",# "NorsettEuler (m=20)",
"ETDRK2 (caching)", "ETDRK2 (m=5)"), "ETDRK2 (m=20)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(), :dts => 1e-5 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-5 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers)]
labels = ["CNAB2 (dense linsolve)" "CNAB2 (Krylov linsolve)" "ETDRK2 (m=5)"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3()),
Dict(:alg => KenCarp4()),
Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense))]
labels = hcat("KenCarp3", "KenCarp4", "KenCarp5", "ARKODE3", "ARKODE4", "ARKODE5")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, medium order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES))]
labels = ["KenCarp3" "KenCarp4" "KenCarp5" "ARKODE3" "ARKODE4" "ARKODE5"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
#Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),matrix contains Infs or NaNs
Dict(:alg => ETDRK4(), :dts => 1e-3 * multipliers),
#Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers),matrix contains Infs or NaNs
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers)]
#Dict(:alg => HochOst4(krylov=true, m=5), :dts => 1e-2 * multipliers)] matrix contains Infs or NaNs
labels = hcat("ETDRK3 (caching)", "ETDRK4 (caching)",# "ETDRK3 (m=5)", "ETDRK4 (m=5)"
"HochOst4 (caching)")#, "HochOst4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense)),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES)),
Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-3 * multipliers)]
labels = hcat("KenCarp5 (dense linsolve)", "ARKODE (dense linsolve)", "KenCarp5 (Krylov linsolve)",
"ARKODE (Krylov linsolve)", "ETDRK3 (m=5)", "ETDRK4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 5947 | ---
title: KdV Pseudospectral Methods Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the Burgers equation using Fourier spectral methods.
```julia
S = Fourier()
n = 512
x = points(S, n)
D2 = Derivative(S,2)[1:n,1:n]
D = (Derivative(S) → S)[1:n,1:n]
T = ApproxFun.plan_transform(S, n)
Ti = ApproxFun.plan_itransform(S, n)
D3 = (Derivative(S,3) → S)[1:n,1:n]
û₀ = T*cos.(x)
δ=0.022
tmp = similar(û₀)
p = (D,T,Ti,similar(tmp),tmp)
function kdv(dû,û,p,t)
D,T,Ti,u,tmp = p
mul!(u,D,û)
mul!(tmp,Ti,u)
mul!(u,Ti,û)
@. tmp=u*tmp
mul!(u,T,tmp)
@.dû = 6*u
end
```
Reference solution using RadauIIA5 is below:
```julia
prob = SplitODEProblem(DiffEqArrayOperator(-D3), kdv, û₀, (0.0,5.0), p)
sol = solve(prob, RadauIIA5(autodiff=false); reltol=1e-14,abstol=1e-14)
test_sol = TestSolution(sol)
tslices=[0.0 1.0 2.0 3.0 5.0]
ys=hcat((Ti*sol(t) for t in tslices)...)
labels=["t=$t" for t in tslices]
plot(x,ys,label=labels)
```
## High tolerances
## In-family comparisons
1.IMEX methods (diagonal linear solver)
```julia
abstols = 0.1 .^ (5:8)
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=diag_linsolve), :dts => 1e-5 * multipliers),
Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-7 * multipliers),
Dict(:alg => CNLF2(linsolve=diag_linsolve), :dts => 5e-7 * multipliers),
Dict(:alg => SBDF2(linsolve=diag_linsolve), :dts => 1e-5 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp1 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true,names=labels,
numruns=5,seconds=5,
save_everystop=false,appxsol=test_sol,maxiters=Int(1e5));
plot(wp1,label=labels,markershape=:auto,title="IMEX methods, diagonal linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler",
"ETDRK2 (caching)")
@time wp2 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp2, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-5 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = ["CNAB2 (diagonal linsolve)" "ETDRK2"]
@time wp3 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp3, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (band linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1))]
labels = hcat("ARKODE3", "ARKODE4", "ARKODE5")
@time wp4 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp4, label=labels, markershape=:auto, title="IMEX methods, band linsolve, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK4 (caching)",
"HochOst4 (caching)")#,"ETDRK4 (m=5)" "ETDRK3 (m=5)" "HochOst4 (m=5)")
@time wp5 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp5, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers)]
labels = hcat("ARKODE (nondiagonal linsolve)", "ETDRK3 ()", "ETDRK4 ()")
@time wp6 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp6, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 9466 | ---
title: KS FDM Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the KS equation using FDM.
```julia
# Define the linear and nonlinear terms
function lin_term(N)
#which is -(D2+D4)
dx = 1/(N + 1)
d2 = (-2) * ones(N) # main diagonal
du2 = ones(N - 1) # off diagonal
d4 = 6 * ones(N) # main diagonal
du4 = (-4) * ones(N - 1) # off diagonal
duu4 = ones(N - 2)
DiffEqArrayOperator(-0.0004*((1/dx^2) * diagm(-1 => du2, 0 => d2, 1 => du2)
+(1/dx^4) * diagm(-2 => duu4, -1 => du4, 0 => d4, 1 => du4, 2 => duu4)))
end
function nl_term(N)
dx = 1/(N + 1)
du = ones(N - 1) # super diagonal
dl = -ones(N - 1) # lower diagonal
D = (-0.2/(4*dx)) * diagm(-1 => dl, 1 => du)
tmp = zeros(N)
function (du,u,p,t)
@. tmp = u^2
mul!(du, D, tmp)
end
end
# Construct the problem
function ks(N)
f1 = lin_term(N)
f2 = nl_term(N)
dx = 1 / (N + 1)
xs = (1:N) * dx
μ0 = 0.3; σ0 = 0.05
f0 = x -> 0.6*exp(-(x - μ0)^2 / (2 * σ0^2))
u0 = f0.(xs)
prob = SplitODEProblem(f1, f2, u0, (0.0, 1.0))
xs, prob
end;
```
Reference solution using RadauIIA5 is below:
```julia
xs, prob = ks(200)
sol = solve(prob, RadauIIA5(autodiff=false); abstol=1e-14, reltol=1e-14)
test_sol = TestSolution(sol);
tslices = [0.0 0.25 0.50 0.75 1.]
ys = hcat((sol(t) for t in tslices)...)
labels = ["t = $t" for t in tslices]
plot(xs, ys, label=labels)
```
Linear solvers
```julia
const LS_Dense = LinSolveFactorize(lu)
```
## High tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNLF2(), :dts => 1e-4 * multipliers),
Dict(:alg => SBDF2(), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, low order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=LinSolveGMRES()), :dts => 1e-9 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-9 * multipliers),
Dict(:alg => CNLF2(linsolve=LinSolveGMRES()), :dts => 1e-9 * multipliers),
Dict(:alg => SBDF2(linsolve=LinSolveGMRES()), :dts => 1e-9 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, Krylov linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=5), :dts => 1e-3 * multipliers),
Dict(:alg => NorsettEuler(krylov=true, m=20), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK2(krylov=true, m=20), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler (caching)", "NorsettEuler (m=5)", "NorsettEuler (m=20)",
"ETDRK2 (caching)", "ETDRK2 (m=5)", "ETDRK2 (m=20)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(), :dts => 1e-4 * multipliers),
Dict(:alg => CNAB2(linsolve=LinSolveGMRES()), :dts => 1e-9 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-3 * multipliers)]
labels = ["CNAB2 (dense linsolve)" "CNAB2 (Krylov linsolve)" "ETDRK2 (m=5)"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (dense linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3()),
Dict(:alg => KenCarp4()),
Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Dense)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense))]
labels = hcat("KenCarp3", "KenCarp4", "KenCarp5", "ARKODE3", "ARKODE4", "ARKODE5")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, dense linsolve, medium order")
```
1.IMEX methods (Krylov linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => KenCarp3(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp4(linsolve=LinSolveGMRES())),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:GMRES)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES))]
labels = ["KenCarp3" "KenCarp4" "KenCarp5" "ARKODE3" "ARKODE4" "ARKODE5"]
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="IMEX methods, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK3 (m=5)", "ETDRK4 (caching)",
"ETDRK4 (m=5)", "HochOst4 (caching)", "HochOst4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => KenCarp5()),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Dense)),
Dict(:alg => KenCarp5(linsolve=LinSolveGMRES())),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:GMRES)),
Dict(:alg => ETDRK3(krylov=true, m=5), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(krylov=true, m=5), :dts => 1e-2 * multipliers)]
labels = hcat("KenCarp5 (dense linsolve)", "ARKODE (dense linsolve)", "KenCarp5 (Krylov linsolve)",
"ARKODE (Krylov linsolve)", "ETDRK3 (m=5)", "ETDRK4 (m=5)")
@time wp = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 6154 | ---
title: KS Pseudospectral Methods Work-Precision Diagrams
author: HAO HAO
---
```julia
using ApproxFun, OrdinaryDiffEq, Sundials
using DiffEqDevTools
using LinearAlgebra
using Plots; gr()
```
Here is the kuramoto_sivashinsky equation using Fourier spectral methods.
```julia
S = Fourier()
n = 512
x = points(S, n)
D2 = Derivative(S,2)[1:n,1:n]
D = (Derivative(S) → S)[1:n,1:n]
T = ApproxFun.plan_transform(S, n)
Ti = ApproxFun.plan_itransform(S, n)
D4 = Derivative(S,4)[1:n,1:n]
û₀ = T*(cos.(x./16).*(1 .+ sin.(x./2.04)))
tmp=similar(û₀)
q = (D,T,Ti,tmp,similar(tmp),similar(tmp))
function kuramoto_sivashinsky(dû,û,q,t)
D,T,Ti,tmp,u,uc = q
mul!(u, D, û)
mul!(tmp, Ti, u)
mul!(u, Ti, û)
@. tmp=tmp*u
mul!(u,T, tmp)
#mul!(uc, D2, û)
@. dû = - u
end
```
Reference solution using Rodas5 is below:
```julia
prob = SplitODEProblem(DiffEqArrayOperator(-Diagonal(D4+D2)), kuramoto_sivashinsky, û₀, (0.0,5.0), q)
sol = solve(prob,RadauIIA5(autodiff=false); reltol=1e-14,abstol=1e-14)
test_sol = TestSolution(sol)
tslices=[0.0 1.0 2.0 3.0 5.0]
ys=hcat((Ti*sol(t) for t in tslices)...)
labels=["t=$t" for t in tslices]
plot(x,ys,label=labels)
```
## High tolerances
```julia
diag_linsolve=LinSolveFactorize(W->let tmp = tmp
for i in 1:size(W, 1)
tmp[i] = W[i, i]
end
Diagonal(tmp)
end)
```
## In-family comparisons
1.IMEX methods (diagonal linear solver)
```julia
abstols = 0.1 .^ (5:8)
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => IMEXEuler(linsolve=diag_linsolve), :dts => 1e-3 * multipliers),
Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers),
Dict(:alg => CNLF2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers),
Dict(:alg => SBDF2(linsolve=diag_linsolve), :dts => 1e-3 * multipliers)]
labels = ["IMEXEuler" "CNAB2" "CNLF2" "SBDF2"]
@time wp1 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true,names=labels,
numruns=5,seconds=5,
save_everystop=false,appxsol=test_sol,maxiters=Int(1e5));
plot(wp1,label=labels,markershape=:auto,title="IMEX methods, diagonal linsolve, low order")
```
2. ExpRK methods
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => NorsettEuler(), :dts => 1e-3 * multipliers),
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = hcat("NorsettEuler",
"ETDRK2 (caching)")
@time wp2 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp2, label=labels, markershape=:auto, title="ExpRK methods, low order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (5:8) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (1:4)
multipliers = 0.5 .^ (0:3)
setups = [Dict(:alg => CNAB2(linsolve=diag_linsolve), :dts => 5e-3 * multipliers)
Dict(:alg => ETDRK2(), :dts => 1e-2 * multipliers)]
labels = ["CNAB2 (diagonal linsolve)" "ETDRK2"]
@time wp3 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp3, label=labels, markershape=:auto, title="Between family, low orders")
```
## Low tolerances
## In-family comparisons
1.IMEX methods (band linear solver)
```julia
abstols = 0.1 .^ (7:13)
reltols = 0.1 .^ (4:10)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=3, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=4, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1))]
labels = hcat("ARKODE3", "ARKODE4", "ARKODE5")
@time wp4 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp4, label=labels, markershape=:auto, title="IMEX methods, band linsolve, medium order")
```
2.ExpRK methods
```julia
abstols = 0.1 .^ (7:11) # all fixed dt methods so these don't matter much
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers),
Dict(:alg => HochOst4(), :dts => 1e-2 * multipliers)]
labels = hcat("ETDRK3 (caching)", "ETDRK4 (caching)",
"HochOst4 (caching)")
@time wp5 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp5, label=labels, markershape=:auto, title="ExpRK methods, medium order")
```
## Between family comparisons
```julia
abstols = 0.1 .^ (7:11)
reltols = 0.1 .^ (4:8)
multipliers = 0.5 .^ (0:4)
setups = [Dict(:alg => ARKODE(Sundials.Implicit(), order=5, linear_solver=:Band, jac_upper=1, jac_lower=1)),
Dict(:alg => ETDRK3(), :dts => 1e-2 * multipliers),
Dict(:alg => ETDRK4(), :dts => 1e-2 * multipliers)]
labels = hcat("ARKODE (nondiagonal linsolve)", "ETDRK3 ()", "ETDRK4 ()")
@time wp6 = WorkPrecisionSet(prob,abstols,reltols,setups;
print_names=true, names=labels,
numruns=5, error_estimate=:l2,
save_everystep=false, appxsol=test_sol, maxiters=Int(1e5));
plot(wp6, label=labels, markershape=:auto, title="Between family, medium order")
```
```julia, echo = false
using SciMLBenchmarks
SciMLBenchmarks.bench_footer(WEAVE_ARGS[:folder],WEAVE_ARGS[:file])
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |
|
[
"MIT"
] | 0.1.3 | f4076dd5a103010d48bb6c4e50c5526f6622fa96 | docs | 5035 | ---
title: Burgers FDM Work-Precision Diagrams with Various MethodOfLines Methods
author: Alex Jones
---
This benchmark is for the MethodOfLines package, which is an automatic PDE discretization package.
It is concerned with comparing the performance of various discretization methods for the Burgers equation.
```julia
using MethodOfLines, DomainSets, OrdinaryDiffEq, ModelingToolkit, DiffEqDevTools, LinearAlgebra,
LinearSolve, Plots
```
Here is the burgers equation with a Dirichlet and Neumann boundary conditions,
```julia
# pdesys1 has Dirichlet BCs, pdesys2 has Neumann BCs
const N = 30
@parameters x t
@variables u(..)
Dx = Differential(x)
Dt = Differential(t)
x_min = 0.0
x_max = 1.0
t_min = 0.0
t_max = 20.0
solver = FBDF()
analytic_u(p, t, x) = x / (t + 1)
analytic = [u(t, x) ~ analytic_u([], t, x)]
eq = Dt(u(t, x)) ~ -u(t, x) * Dx(u(t, x))
bcs1 = [u(0, x) ~ x,
u(t, x_min) ~ analytic_u([], t, x_min),
u(t, x_max) ~ analytic_u([], t, x_max)]
bcs2 = [u(0, x) ~ x,
Dx(u(t, x_min)) ~ 1 / (t + 1),
Dx(u(t, x_max)) ~ 1 / (t + 1)]
domains = [t ∈ Interval(t_min, t_max),
x ∈ Interval(x_min, x_max)]
@named pdesys1 = PDESystem(eq, bcs1, domains, [t, x], [u(t, x)], analytic=analytic)
@named pdesys2 = PDESystem(eq, bcs2, domains, [t, x], [u(t, x)], analytic=analytic)
```
Here is a uniform discretization with the Upwind scheme:
```julia
discupwind1 = MOLFiniteDifference([x => N], t, advection_scheme=UpwindScheme())
discupwind2 = MOLFiniteDifference([x => N-1], t, advection_scheme=UpwindScheme(), grid_align=edge_align)
```
Here is a uniform discretization with the WENO scheme:
```julia
discweno1 = MOLFiniteDifference([x => N], t, advection_scheme=WENOScheme())
discweno2 = MOLFiniteDifference([x => N-1], t, advection_scheme=WENOScheme(), grid_align=edge_align)
```
Here is a non-uniform discretization with the Upwind scheme, using tanh (nonuniform WENO is not implemented yet):
```julia
gridnu1 = chebyspace(domains[2], N)
gridnu2 = chebyspace(domains[2], N-1)
discnu1 = MOLFiniteDifference([x => gridnu1], t, advection_scheme=UpwindScheme())
discnu2 = MOLFiniteDifference([x => gridnu2], t, advection_scheme=UpwindScheme(), grid_align=edge_align)
```
Here are the problems for pdesys1:
```julia
probupwind1 = discretize(pdesys1, discupwind1; analytic=pdesys1.analytic_func)
probupwind2 = discretize(pdesys1, discupwind2; analytic=pdesys1.analytic_func)
probweno1 = discretize(pdesys1, discweno1; analytic=pdesys1.analytic_func)
probweno2 = discretize(pdesys1, discweno2; analytic=pdesys1.analytic_func)
probnu1 = discretize(pdesys1, discnu1; analytic=pdesys1.analytic_func)
probnu2 = discretize(pdesys1, discnu2; analytic=pdesys1.analytic_func)
probs1 = [probupwind1, probupwind2, probnu1, probnu2, probweno1, probweno2]
```
## Work-Precision Plot for Burgers Equation, Dirichlet BCs
```julia
dummy_appxsol = [nothing for i in 1:length(probs1)]
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:alg => solver, :prob_choice => 1),
Dict(:alg => solver, :prob_choice => 2),
Dict(:alg => solver, :prob_choice => 3),
Dict(:alg => solver, :prob_choice => 4),
Dict(:alg => solver, :prob_choice => 5),
Dict(:alg => solver, :prob_choice => 6),]
names = ["Uniform Upwind, center_align", "Uniform Upwind, edge_align", "Nonuniform Upwind, center_align",
"Nonuniform Upwind, edge_align", "WENO, center_align", "WENO, edge_align"];
wp = WorkPrecisionSet(probs1, abstols, reltols, setups; names=names,
save_everystep=false, appxsol = dummy_appxsol, maxiters=Int(1e5),
numruns=10, wrap=Val(false))
plot(wp)
```
Here are the problems for pdesys2:
```julia
probupwind1 = discretize(pdesys2, discupwind1; analytic=pdesys2.analytic_func)
probupwind2 = discretize(pdesys2, discupwind2; analytic=pdesys2.analytic_func)
probweno1 = discretize(pdesys2, discweno1; analytic=pdesys2.analytic_func)
probweno2 = discretize(pdesys2, discweno2; analytic=pdesys2.analytic_func)
probnu1 = discretize(pdesys2, discnu1; analytic=pdesys2.analytic_func)
probnu2 = discretize(pdesys2, discnu2; analytic=pdesys2.analytic_func)
probs2 = [probupwind1, probupwind2, probnu1, probnu2, probweno1, probweno2]
```
## Work-Precision Plot for Burgers Equation, Neumann BCs
```julia
abstols = 1.0 ./ 10.0 .^ (5:8)
reltols = 1.0 ./ 10.0 .^ (1:4);
setups = [Dict(:alg => solver, :prob_choice => 1),
Dict(:alg => solver, :prob_choice => 2),
Dict(:alg => solver, :prob_choice => 3),
Dict(:alg => solver, :prob_choice => 4),
Dict(:alg => solver, :prob_choice => 5),
Dict(:alg => solver, :prob_choice => 6),]
names = ["Uniform Upwind, center_align", "Uniform Upwind, edge_align", "Nonuniform Upwind, center_align",
"Nonuniform Upwind, edge_align", "WENO, center_align", "WENO, edge_align"];
wp = WorkPrecisionSet(probs2, abstols, reltols, setups; names=names,
save_everystep=false, maxiters=Int(1e5),
numruns=10, wrap=Val(false))
plot(wp)
```
| SciMLBenchmarks | https://github.com/SciML/SciMLBenchmarks.jl.git |